AWK If Statement Guide | Command Line Conditional Logic
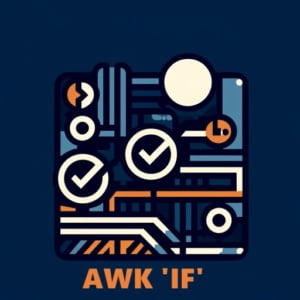
Text processing is a common task for us while programming at IOFLOOD. Often we prefer to use AWK, as well at the ‘if’ statements as they allow us to execute specific actions based on defined conditions. In today’s article, we’ll focus on AWK’s ‘if’ statement, providing practical examples and step-by-step instructions to assist our bare metal cloud customers and fellow developers in effectively using conditional logic in AWK.
This guide will help you understand and master the use of ‘if’ statements in AWK. We’ll explore the basic syntax, delve into more advanced concepts, and even discuss common issues and their solutions.
So, let’s dive in and start mastering ‘if’ statements in AWK!
TL;DR: How Do I Use the ‘If’ Statement in AWK?
In AWK, you use the
'if'
statement to test a condition. If the condition is true, AWK will execute a block of code. The basic syntax for an ‘if’ statement is,awk '{if (condition) <action>}' file.txt
.
Here’s a simple example:
echo '10' | awk '{if ($1 > 5) print "Number is greater than 5";}'
# Output:
# 'Number is greater than 5'
In this example, we’re using the ‘echo’ command to pass the number 10 to AWK. The ‘if’ statement in the AWK script checks if the number (represented by $1
) is greater than 5. Since 10 is indeed greater than 5, AWK executes the code within the curly braces, printing ‘Number is greater than 5’.
This is just a basic use of the ‘if’ statement in AWK. There’s much more to learn about controlling the flow of your AWK scripts. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
Understanding AWK ‘If’ Statements
The ‘if’ statement in AWK is a conditional statement that tests whether a condition is true or false. If the condition is true, AWK executes a specific block of code. If it’s false, AWK will skip over the code block and continue with the rest of the script. This functionality is crucial for controlling the flow of your AWK scripts.
Here’s the basic syntax of the ‘if’ statement in AWK:
awk '{if (condition) action}'
In this syntax, ‘condition’ is the condition you want to test, and ‘action’ is the code you want AWK to execute if the condition is true.
Let’s look at a simple example of how to use the ‘if’ statement in AWK:
echo '15' | awk '{if ($1 <= 20) print "Number is less than or equal to 20";}'
# Output:
# 'Number is less than or equal to 20'
In this example, we’re passing the number 15 to AWK. The ‘if’ statement in the AWK script checks if this number (represented by $1
) is less than or equal to 20. Since 15 is indeed less than or equal to 20, AWK executes the code within the curly braces, printing ‘Number is less than or equal to 20’.
Advantages and Pitfalls of ‘If’ Statements
The ‘if’ statement in AWK is a powerful tool that offers several advantages. It allows you to control the flow of your AWK scripts, making decisions based on specific conditions. This functionality can greatly enhance your script’s flexibility and adaptability.
However, ‘if’ statements also come with potential pitfalls. One common issue is forgetting to use proper syntax, such as missing curly braces or parentheses. Another pitfall is not clearly defining your conditions, which can lead to unexpected results. Always ensure you’re using clear, precise conditions and proper syntax when working with ‘if’ statements in AWK.
Advanced ‘If’ Statement Usage in AWK
As you become more comfortable with ‘if’ statements in AWK, you can start to explore more complex uses. Two important concepts to understand are nested ‘if’ statements and the use of ‘else’ and ‘else if’.
Nested ‘If’ Statements in AWK
Nested ‘if’ statements are ‘if’ statements within another ‘if’ statement. This allows you to test multiple conditions in a specific order.
Here’s an example of a nested ‘if’ statement in AWK:
echo '15' | awk '{if ($1 <= 20) {if ($1 >= 10) print "Number is between 10 and 20";}}'
# Output:
# 'Number is between 10 and 20'
In this example, we’re passing the number 15 to AWK. The outer ‘if’ statement checks if this number (represented by $1
) is less than or equal to 20. If this condition is true (which it is), AWK moves on to the inner ‘if’ statement, which checks if the number is greater than or equal to 10. Since both conditions are true, AWK executes the code within the innermost curly braces, printing ‘Number is between 10 and 20’.
Using ‘Else’ and ‘Else If’ in AWK
In addition to ‘if’, AWK also provides ‘else’ and ‘else if’ for more complex decision making. ‘Else’ allows you to specify a block of code to be executed if the ‘if’ condition is false, while ‘else if’ allows you to test another condition if the first ‘if’ condition is false.
Here’s an example of using ‘else’ and ‘else if’ in AWK:
echo '25' | awk '{if ($1 <= 20) print "Number is less than or equal to 20"; else if ($1 <= 30) print "Number is between 21 and 30"; else print "Number is greater than 30";}'
# Output:
# 'Number is between 21 and 30'
In this example, we’re passing the number 25 to AWK. The ‘if’ statement checks if this number (represented by $1
) is less than or equal to 20. Since this condition is false, AWK moves on to the ‘else if’ statement, which checks if the number is less than or equal to 30. Since this condition is true, AWK executes the code within these curly braces, printing ‘Number is between 21 and 30’. If both conditions had been false, AWK would have executed the code within the ‘else’ block, printing ‘Number is greater than 30’.
Other AWK Control Flow Tools
While ‘if’ statements are a powerful tool for controlling the flow of your AWK scripts, they’re not the only tool available. Two other methods for controlling flow in AWK scripts are ‘while’ loops and ‘for’ loops. These tools can offer more flexibility in certain situations.
The ‘While’ Loop in AWK
The ‘while’ loop in AWK executes a block of code as long as a specific condition is true. Here’s an example of a ‘while’ loop that prints the numbers 1 through 5:
echo | awk '{i=1; while(i<=5) {print i; i++;}}'
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we initialize a variable i
with a value of 1. The ‘while’ loop checks if i
is less than or equal to 5. If this condition is true (which it is initially), AWK executes the code within the curly braces, printing the current value of i
and then incrementing i
by 1. This process continues until i
is greater than 5, at which point the ‘while’ loop stops executing.
The ‘For’ Loop in AWK
The ‘for’ loop in AWK is another tool for controlling flow. It’s particularly useful when you want to execute a block of code a specific number of times. Here’s an example of a ‘for’ loop that prints the numbers 1 through 5:
echo | awk '{for(i=1; i<=5; i++) print i;}'
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we’re using a ‘for’ loop to print the numbers 1 through 5. The ‘for’ loop initializes a variable i
with a value of 1, checks if i
is less than or equal to 5, and increments i
by 1 after each loop iteration. The code within the curly braces (printing the current value of i
) is executed for each iteration of the loop.
Weighing the Benefits and Drawbacks
While ‘while’ and ‘for’ loops offer more flexibility in certain situations, they also come with potential pitfalls. For example, ‘while’ and ‘for’ loops can lead to infinite loops if not used carefully. Also, ‘if’ statements are generally simpler and easier to understand, especially for beginners. However, by understanding and using all these tools effectively, you can greatly enhance the functionality and flexibility of your AWK scripts.
Troubleshooting AWK ‘If’ Statements
While ‘if’ statements in AWK are incredibly useful, they can also be a common source of errors, especially for beginners. Let’s discuss some typical issues you might encounter and how to resolve them.
Syntax Errors
Syntax errors are common when using ‘if’ statements in AWK. These can be caused by missing parentheses or curly braces, or by incorrect usage of operators.
For example, consider the following code:
echo '10' | awk '{if $1 > 5 print "Number is greater than 5";}'
This code will result in a syntax error because the condition within the ‘if’ statement is not enclosed in parentheses. The correct syntax should be:
echo '10' | awk '{if ($1 > 5) print "Number is greater than 5";}'
# Output:
# 'Number is greater than 5'
Logical Errors
Logical errors occur when your script doesn’t behave as expected due to incorrect logic. For example, you might accidentally use the less than operator (“), leading to unexpected results.
Consider the following code:
echo '10' | awk '{if ($1 < 5) print "Number is less than 5";}'
This code will not produce any output because the number 10 is not less than 5. The logic of the ‘if’ statement is incorrect. If you wanted to check if the number is greater than 5, you should use the greater than operator (>
):
echo '10' | awk '{if ($1 > 5) print "Number is greater than 5";}'
# Output:
# 'Number is greater than 5'
Best Practices
When using ‘if’ statements in AWK, here are some best practices to follow:
- Always enclose your conditions in parentheses.
- Use curly braces to define the block of code to be executed if the condition is true.
- Be clear and precise with your conditions to avoid logical errors.
- Regularly test your scripts to ensure they’re behaving as expected.
By understanding these common errors and following these best practices, you can use ‘if’ statements in AWK more effectively and avoid common pitfalls.
Unpacking AWK and the ‘If’ Statement
AWK is a powerful text processing language named after its inventors—Aho, Weinberger, and Kernighan. It’s particularly adept at handling tasks such as data extraction, report generation, and text transformation. One of the keys to AWK’s power is its ability to control the flow of scripts, which is where the ‘if’ statement comes into play.
The Role of ‘If’ in AWK
The ‘if’ statement is a fundamental part of many programming languages, and AWK is no exception. In AWK, the ‘if’ statement tests a condition and, based on the result, decides the next course of action. If the condition is true, AWK executes a specific block of code. If it’s false, AWK skips the block and moves on.
Here’s an example:
echo '5' | awk '{if ($1 != 10) print "Number is not 10";}'
# Output:
# 'Number is not 10'
In this example, we’re passing the number 5 to AWK. The ‘if’ statement checks if this number (represented by $1
) is not equal to 10. As the condition is true (5 is not equal to 10), AWK executes the code within the curly braces, printing ‘Number is not 10’.
The Importance of Flow Control in Scripts
Flow control is essential in scripting and programming. It allows your script to make decisions based on specific conditions, leading to more dynamic and flexible code. Without flow control, your scripts would always follow the same linear path, which is often not desirable or efficient.
The ‘if’ statement is one of the most basic forms of flow control. By mastering ‘if’ statements in AWK, you can create scripts that adapt to different inputs and situations, making your AWK programming more robust and versatile.
Practical Uses of AWK Statements
As you start to work on larger scripts or projects in AWK, you’ll find that ‘if’ statements become even more crucial. They’re not just useful for simple scripts—they’re also a key part of more complex AWK programs.
Let’s consider an example where you have a file with multiple columns of data, and you want to process this data based on certain conditions. Here, ‘if’ statements can be used to check each line of the file and perform different actions depending on the data.
awk '{if ($1 > $2) print $1 " is greater than " $2; else if ($1 < $2) print $1 " is less than " $2; else print $1 " is equal to " $2;}' data.txt
This command will go through each line in data.txt
, compare the values in the first and second columns, and print a message indicating whether the first value is greater than, less than, or equal to the second value.
Exploring Related AWK Topics
As you continue to learn AWK and use ‘if’ statements, you’ll often find yourself dealing with related topics. Two such topics are arrays and built-in variables.
Arrays in AWK allow you to store multiple values in a single variable. This can be particularly useful when you’re dealing with large amounts of data. You can use ‘if’ statements in conjunction with arrays to perform tasks based on specific conditions.
Built-in variables in AWK, such as NF
(the number of fields in a record) and NR
(the current record number), can also be used with ‘if’ statements to control the flow of your scripts based on the structure and content of your data.
Further Resources for Mastering AWK ‘If’ Statements
To deepen your understanding of ‘if’ statements in AWK and related topics, here are some additional resources you might find helpful:
- GNU AWK User’s Guide: This comprehensive guide covers all aspects of AWK, including ‘if’ statements, arrays, and built-in variables.
The AWK Programming Language by Aho, Kernighan, and Weinberger: This book, written by the creators of AWK, provides an in-depth look at the language and its features.
AWK Tutorial by TutorialsPoint: This online tutorial offers a step-by-step approach to learning AWK, with separate sections dedicated to ‘if’ statements and other control flow tools.
Recap: ‘If’ Statements in AWK
In this comprehensive guide, we’ve delved into the world of AWK, focusing on the usage of ‘if’ statements to control the flow of scripts. This essential tool, akin to a traffic light, governs the execution of your scripts based on specific conditions.
We started with the basics, understanding the syntax and usage of the ‘if’ statement in AWK. We then delved deeper, exploring nested ‘if’ statements and the use of ‘else’ and ‘else if’ for more complex decision-making scenarios. We also discussed common errors when using ‘if’ statements and provided solutions to help you avoid these pitfalls.
In the expert level, we ventured beyond ‘if’ statements, exploring other flow control tools in AWK, such as ‘while’ and ‘for’ loops. We also touched on related topics, such as arrays and built-in variables, and how they can be used in conjunction with ‘if’ statements.
Here’s a quick recap of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘If’ Statement | Controls flow based on conditions, easy to understand | Can lead to complex nested structures |
‘While’ Loop | Executes code as long as condition is true | Can lead to infinite loops if not used carefully |
‘For’ Loop | Executes code a specific number of times, useful for iterating over arrays | Requires careful setup and initialization |
Whether you’re just starting out with AWK or you’re looking to deepen your understanding, we hope this guide has helped you master ‘if’ statements and other control flow tools in AWK.
The ability to control the flow of your scripts is a powerful tool that can make your AWK programming more robust and flexible. With this knowledge, you’re well-equipped to write efficient and adaptable AWK scripts. Happy coding!