AWK If-Else Statements | Conditional Execution in Unix
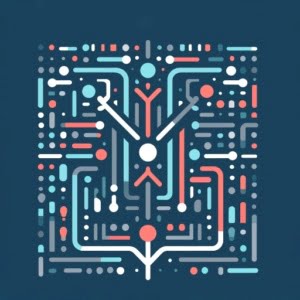
When working with AWK for text processing automation at IOFLOOD, if-else statements are a staple technique for conditional logic. Utilizing our notes and processes, we have formulated this article on utilizing AWK’s if-else statements. Our hope is that by utilizing this resource, our dedicated hosting customers and fellow developers will gain the insights necessary to implement if-else logic in AWK for their data processing needs.
In this guide, we’ll walk you through the process of using if-else statements in AWK, from the basics to more advanced techniques. We’ll cover everything from writing simple if-else statements, handling complex conditions, to alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering if-else statements in AWK!
TL;DR: How Do I Use If-Else Statements in AWK?
In AWK, you use if-else statements to control the flow of your program based on certain conditions. They are used with the syntax,
awk '{if (first_condition) <action_if_true>; else <action_if_false>}' file.txt
.
Here’s a simple example:
awk '{if ($1 > 10) print $1; else print $0}' file.txt
# Output:
# If the first field is greater than 10, it will print the first field. Otherwise, it will print the whole line.
In this example, we’re using AWK’s if-else statement to check if the first field ($1
) of each line in file.txt
is greater than 10. If it is, AWK prints the first field. If it’s not, AWK prints the whole line.
This is a basic way to use if-else statements in AWK, but there’s much more to learn about controlling the flow of your AWK scripts. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
The Basic Use of If-Else Statements
The if-else statement in AWK is a conditional statement that allows your program to perform different actions based on whether a certain condition is true or false. It’s a fundamental part of any programming language, and AWK is no exception.
Syntax of If-Else Statements in AWK
awk '{if (condition) action1; else action2}' file
In this syntax, condition
is the condition that you’re checking. If this condition is true, AWK performs action1
. If it’s false, AWK performs action2
.
Let’s look at a simple example:
awk '{if ($1 == "Anton") print "Hello, " $1; else print "Who are you?"}' file.txt
# Output:
# If the first field is "Anton", it will print "Hello, Anton". Otherwise, it will print "Who are you?".
In this example, we’re using an if-else statement to check if the first field of each line in file.txt
is “Anton”. If it is, AWK prints “Hello, Anton”. If it’s not, AWK prints “Who are you?”.
Benefits and Potential Pitfalls of If-Else Statements
If-else statements are extremely powerful as they allow your AWK scripts to make decisions based on certain conditions. However, they can also lead to complex and hard-to-read code if not used carefully.
One common pitfall is not using braces {}
to group your actions. If your action1
or action2
consists of more than one command, you must use braces to group them. Otherwise, AWK will only consider the first command as part of your if-else statement.
In the next section, we’ll dive deeper into more complex uses of if-else statements in AWK, such as nested if-else statements and using logical operators.
Advanced Use of If-Else Statements
As you become more comfortable with if-else statements in AWK, you can start exploring more complex uses. Two such advanced techniques involve nested if-else statements and the use of logical operators.
Nested If-Else Statements in AWK
Nested if-else statements are when an if-else statement is placed inside another if-else statement. This allows you to check for multiple conditions and make more complex decisions.
Here’s an example:
awk '{if ($1 == "Anton") print "Hello, " $1; else if ($1 == "John") print "Hey, " $1; else print "Who are you?"}' file.txt
# Output:
# If the first field is "Anton", it will print "Hello, Anton". If it's "John", it will print "Hey, John". Otherwise, it will print "Who are you?".
In this example, we’re using a nested if-else statement to check if the first field of each line in file.txt
is “Anton” or “John”. Depending on the name, AWK prints a different greeting.
Using Logical Operators in AWK If-Else Statements
Logical operators allow you to check multiple conditions at once. AWK supports the &&
(and) and ||
(or) logical operators.
Here’s an example of using the &&
operator:
awk '{if ($1 == "Anton" && $2 == "Smith") print "Hello, " $1 " " $2; else print "Who are you?"}' file.txt
# Output:
# If the first field is "Anton" and the second field is "Smith", it will print "Hello, Anton Smith". Otherwise, it will print "Who are you?".
In this example, the if-else statement checks if the first field is “Anton” and the second field is “Smith”. If both conditions are true, AWK prints a greeting. If not, AWK prints “Who are you?”.
These advanced techniques allow you to write more versatile and efficient AWK scripts. However, they can also lead to more complex and harder-to-read code. Therefore, it’s important to use them judiciously and always prioritize code readability.
Alternatives to If-Else in AWK
While if-else statements are a fundamental way to control program flow in AWK, they are not the only method. For more advanced control, you can use alternative approaches such as the ternary operator or switch statements.
The Ternary Operator in AWK
The ternary operator is a shorthand way of writing an if-else statement. It’s a one-liner that checks a condition and performs an action based on whether the condition is true or false.
Here’s the syntax of the ternary operator in AWK:
awk '{print (condition ? "true action" : "false action")}' file
Let’s see an example:
awk '{print ($1 == "Anton" ? "Hello, " $1 : "Who are you?")}' file.txt
# Output:
# If the first field is "Anton", it will print "Hello, Anton". Otherwise, it will print "Who are you?".
In this example, the ternary operator checks if the first field is “Anton”. If it is, AWK prints a greeting. If it’s not, AWK prints “Who are you?”.
Switch Statements in AWK
AWK also supports switch statements, which are a more readable alternative to nested if-else statements when you have multiple conditions to check.
Here’s the syntax of switch statements in AWK:
awk '{switch ($1) {case "value1": action1; break; case "value2": action2; break; default: default_action;}}' file
Let’s see an example:
awk '{switch ($1) {case "Anton": print "Hello, " $1; break; case "John": print "Hey, " $1; break; default: print "Who are you?";}}' file.txt
# Output:
# If the first field is "Anton", it will print "Hello, Anton". If it's "John", it will print "Hey, John". Otherwise, it will print "Who are you?".
In this example, the switch statement checks the first field of each line. Depending on the name, AWK prints a different greeting.
While these alternative approaches can make your AWK scripts more concise and readable, they also have their downsides. The ternary operator can lead to hard-to-read code if overused, and switch statements can be overkill for simple conditions. Therefore, it’s important to choose the right tool for the job and always prioritize code readability.
Troubleshooting AWK If-Else
While if-else statements in AWK are a powerful tool, they can also lead to common issues, especially syntax errors. Understanding these issues and how to resolve them is key to writing effective AWK scripts.
Syntax Errors in AWK If-Else Statements
One of the most common issues when using if-else statements in AWK is syntax errors. These can occur due to missing or misplaced braces {}
, parentheses ()
, or semicolons ;
.
For example, consider this incorrect AWK script:
awk '{if $1 == "Anton" print "Hello, " $1; else print "Who are you?"}' file.txt
# Output:
# syntax error at source line 1
# context is
# >>> {if $1 == "Anton" print <<< "Hello, " $1; else print "Who are you?"}
# awk: bailing out at source line 1
This script results in a syntax error because the condition in the if statement is not enclosed in parentheses. Here’s the corrected script:
awk '{if ($1 == "Anton") print "Hello, " $1; else print "Who are you?"}' file.txt
# Output:
# If the first field is "Anton", it will print "Hello, Anton". Otherwise, it will print "Who are you?".
By simply adding parentheses around the condition, we’ve resolved the syntax error.
Best Practices for AWK If-Else Statements
Here are some tips to avoid common issues when using if-else statements in AWK:
- Always enclose your conditions in parentheses
()
. - Use braces
{}
to group your actions, especially if they consist of more than one command. - Place your else statement on the same line as the closing brace of the if statement to avoid unexpected behavior.
- Use indentation and spacing to make your if-else statements more readable.
Remember, the key to effective AWK scripting is not just knowing how to write if-else statements, but also understanding how to troubleshoot and optimize them.
Conditional Statements & Control Flow
Before diving deeper into the specifics of AWK’s if-else statements, it’s crucial to grasp the fundamental concepts of conditional statements and control flow in programming.
Conditional Statements: The Building Blocks of Control Flow
Conditional statements are the backbone of control flow in any programming language. They allow a program to perform different actions based on whether a certain condition is true or false. The simplest form of a conditional statement is the if statement.
Here’s a simple example in Python, another programming language:
if 5 > 3:
print('5 is greater than 3')
# Output:
# '5 is greater than 3'
In this example, the program checks if 5 is greater than 3. Since this condition is true, it prints ‘5 is greater than 3’.
Control Flow in AWK: If-Else Statements
In AWK, if-else statements are a fundamental way to control the flow of your program. Much like a traffic signal, they direct the course of your AWK scripts based on certain conditions.
Here’s a simple example in AWK:
awk '{if ($1 > 3) print $1 " is greater than 3"}' file.txt
# Output:
# If the first field is greater than 3, it will print '[first field] is greater than 3'.
In this example, AWK checks if the first field of each line in file.txt
is greater than 3. If it is, AWK prints ‘[first field] is greater than 3’.
Understanding these fundamental concepts is key to mastering if-else statements in AWK and controlling the flow of your AWK scripts.
Practical Usages of AWK If-Else
As you become more proficient in using if-else statements in AWK, you’ll start to see their true power when used in larger scripts or programs. If-else statements can control complex workflows, making decisions based on multiple variables or conditions.
If-Else Statements in Complex AWK Scripts
Consider a script that processes a log file. The script could use if-else statements to handle different types of log entries differently. For example, it could print warnings to the console, write errors to a separate error log, and ignore informational entries.
Exploring Related Topics in AWK
As you continue to learn AWK, there are other topics that you might find interesting and useful. For example, learning about arrays in AWK can help you process and manipulate data more efficiently. Regular expressions, on the other hand, can help you match and extract data from strings in a flexible way.
Further Resources for Mastering AWK
To help you further your understanding and mastery of AWK and its if-else statements, here are a few resources that you might find helpful:
- GNU AWK User’s Guide: A comprehensive guide to AWK from the creators of the language.
Effective AWK Programming: A book by Arnold Robbins, a recognized expert in AWK.
Learn AWK with The AWK Programming Language: A book by the original authors of the AWK language, providing in-depth information and practical examples.
These resources provide a wealth of information and practical examples that can help you master if-else statements and other aspects of AWK.
Recap: If-Else Guide in AWK
In this comprehensive guide, we’ve explored the ins and outs of using if-else statements in AWK, a powerful tool for controlling the flow of your AWK scripts.
We began with the basics, learning how to write simple if-else statements in AWK. We then moved on to more advanced usage, such as nested if-else statements and using logical operators. Along the way, we’ve tackled common issues you might face when using if-else statements in AWK, such as syntax errors, providing you with solutions and best practices for each issue.
We also ventured beyond if-else statements, exploring alternative approaches to control program flow in AWK, such as the ternary operator and switch statements. Each method has its own strengths and weaknesses, and the best choice depends on your specific needs and the complexity of your AWK scripts.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
If-Else Statements | Powerful, versatile | Can lead to complex code |
Ternary Operator | Concise, one-liner | Can be hard to read if overused |
Switch Statements | More readable for multiple conditions | Can be overkill for simple conditions |
Whether you’re just starting out with AWK or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of if-else statements in AWK and how to use them effectively.
The ability to control the flow of your AWK scripts using if-else statements is a powerful tool in your scripting toolbox. Happy coding!