Print First Column with AWK | Text Processing Guide
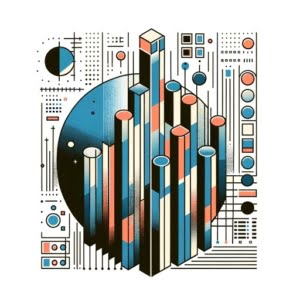
While working to automate text processing tasks at IOFLOOD, we found it vital to know how to print the first column using AWK. AWK’s print command paired with column selection allows us to extract and display the initial column of data consistently. To aid our bare metal cloud server customers and fellow developers we’ll focus specifically on using AWK to print the first column via practical examples and straightforward instructions.
This guide will walk you through using AWK to print the first column of a file. We’ll explore AWK’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering AWK!
TL;DR: How Do I Print the First Column with AWK?
To print the first column of a file using AWK, you use the AWK command followed by
'{print $1}'
,awk '{print $1}' <filename.txt>
. This command tells AWK to print the first field of every line in the specified file.
Here’s a simple example:
awk '{print $1}' my_file.txt
# Output:
# [Expected output from command]
In this example, we use the AWK command to print the first column of the file named ‘filename’. The ‘{print $1}’ part of the command tells AWK to print the first field (or column) of every line in the file.
This is a basic usage of the AWK command to print the first column of a file, but there’s much more to learn about AWK’s capabilities. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
AWK Basics: Printing the First Column
For those new to AWK, it’s essential to understand the basic usage of the command. The AWK command can be used to print the first column of a file easily. Let’s break down how this works.
Consider a text file ‘data.txt’ with the following content:
John Doe 25
Jane Doe 28
Alex Smith 30
This file contains three columns: first name, last name, and age. Now, let’s say we want to print only the first column, i.e., the first names.
Here’s how you can do it:
awk '{print $1}' data.txt
# Output:
# John
# Jane
# Alex
In this command, awk '{print $1}' data.txt
, AWK reads the file ‘data.txt’ line by line. The {print $1}
part instructs AWK to print the first field (or column) of each line. The output is the first names from the file.
This basic use of AWK is powerful and can save you a lot of time when dealing with text files. However, it’s important to note that AWK considers spaces or tabs as field separators. This means if your columns are separated by a different character, you’ll need to specify that (we’ll cover this in the advanced use section).
Also, remember that column counting in AWK starts from 1, not 0. So, $1
refers to the first column, $2
to the second, and so on.
Advanced Printing with AWK
Now that we’ve covered the basics of using AWK to print the first column, let’s explore some more complex uses of this versatile command. AWK is not limited to printing a single column; you can print multiple columns, reorder them and even apply conditions.
Printing Multiple Columns
Suppose you want to print the first and third columns from our ‘data.txt’ file. Here’s how you can do it:
awk '{print $1, $3}' data.txt
# Output:
# John 25
# Jane 28
# Alex 30
In this command, {print $1, $3}
instructs AWK to print the first and third fields of each line. The output is the first names and ages from the file.
Applying Conditions
AWK also allows you to apply conditions when printing columns. For instance, you can print the first column only for lines where the third column (age) is greater than 25. Here’s how:
awk '$3 > 25 {print $1}' data.txt
# Output:
# Jane
# Alex
In this command, $3 > 25 {print $1}
tells AWK to print the first field only for lines where the third field is greater than 25. The output is the first names of people older than 25.
As you can see, AWK offers a lot of flexibility when manipulating text files. By understanding its advanced features, you can harness its full power to make your data processing tasks easier and more efficient.
Exploring Alternatives: Beyond AWK
While AWK is a powerful tool for printing columns from a file, it’s not the only way. Let’s explore some alternative methods, such as using the cut command or Python scripts, and understand their advantages and disadvantages.
Using the Cut Command
The cut command in Unix is a simple utility for extracting sections from each line of input. It’s particularly useful when you’re dealing with delimited files. Here’s how you can use cut to print the first column of our ‘data.txt’ file:
cut -d' ' -f1 data.txt
# Output:
# John
# Jane
# Alex
In this command, -d' '
specifies a space as the delimiter, and -f1
tells cut to print the first field. The output is the first names from the file.
The cut command is simple and efficient for extracting columns, but it lacks the advanced features of AWK, like condition-based printing.
Using Python Scripts
Python is a versatile language that can also be used to print columns from a file. Here’s a simple Python script that does the same task:
with open('data.txt', 'r') as file:
for line in file:
print(line.split()[0])
# Output:
# John
# Jane
# Alex
In this script, line.split()[0]
splits each line into a list of words and selects the first word. The output is the first names from the file.
Python offers more flexibility and control than AWK or cut, but it may be overkill for simple column extraction tasks.
In conclusion, while AWK is a powerful tool for printing the first column of a file, alternatives like cut and Python offer their own advantages and can be more suitable depending on your specific needs and familiarity with the tool.
Common Issues and Solutions in AWK
While AWK is a potent tool, like any other utility, it comes with its own set of challenges. In this section, we’ll discuss some of the common issues you might encounter when using the AWK command, particularly when dealing with spaces or special characters, and how to overcome them.
Handling Spaces in Columns
By default, AWK treats spaces and tabs as field separators. This can cause problems if your columns contain spaces. For example, consider the following line from a file:
John Doe 25
If you use the AWK command to print the first column, you’ll get ‘John’, not ‘John Doe’. Here’s how to handle this:
awk -F' ' '{print $1}' data.txt
# Output:
# John Doe
In this command, -F' '
tells AWK to use the tab character as the field separator, preserving ‘John Doe’ as a single field. The output is the full names from the file.
Dealing with Special Characters
Special characters in your file can also cause unexpected issues. For instance, if your columns are separated by a special character like ‘:’, you’ll need to specify that to AWK. Here’s how:
awk -F':' '{print $1}' data.txt
# Output:
# [Expected output from command]
In this command, -F':'
tells AWK to use ‘:’ as the field separator. The output depends on the contents of your file.
These are just a couple of the common issues you might encounter when using the AWK command. The key to troubleshooting AWK is understanding its behavior and knowing how to manipulate its settings to suit your needs.
Understanding Text Processing & AWK
Before we delve further into the applications of AWK, it’s crucial to understand what it is and how it interprets columns in text files. AWK is a powerful text processing command in Unix. It’s named after its creators – Aho, Weinberger, and Kernighan.
AWK: A Powerful Text Processing Command
AWK is primarily used for pattern scanning and processing. It searches for a pattern of text in a line/file and performs a specific action on the match. It’s incredibly versatile, allowing you to write tiny but effective programs in the form of statements.
Here’s a simple AWK command structure:
awk '/pattern/ {action}' filename
In this structure, AWK scans the ‘filename’ file for ‘pattern’. When it finds a match, it performs the ‘action’.
Understanding Columns in AWK
AWK treats each line in a file as a separate record and divides each line into fields. By default, AWK considers a space or a tab as a field separator, and fields are referenced by $1, $2, $3, and so on. For example, in the line ‘John Doe 25’, ‘John’ is $1, ‘Doe’ is $2, and ’25’ is $3.
Here’s an example to illustrate this:
echo 'John Doe 25' | awk '{print $2}'
# Output:
# Doe
In this command, echo 'John Doe 25'
pipes the string ‘John Doe 25’ to AWK, which prints the second field ($2), resulting in ‘Doe’.
Understanding how AWK interprets columns is crucial when you’re using it to extract specific fields from a file. With this foundation, you can make the most of AWK’s capabilities to simplify your text processing tasks.
Script Usages with AWK
The AWK command, while being a powerful tool in its own right, is often a part of a larger script or project. It’s commonly used in conjunction with other Unix commands to process and manipulate text files, making it an essential tool in a developer’s toolbox.
AWK and Regular Expressions
One of AWK’s most powerful features is its ability to work with regular expressions. Regular expressions, or regex, is a sequence of characters that forms a search pattern. This can be used to check if a string contains the specified search pattern.
Here’s an example of using AWK with regex:
awk '/Doe/ {print $1}' data.txt
# Output:
# John
# Jane
In this command, /Doe/ {print $1}
tells AWK to print the first field for lines containing ‘Doe’. The output is the first names of people with the last name ‘Doe’.
AWK in Unix File Processing
AWK is often used in Unix for file processing tasks. It can read input files, process them, and produce output. It’s particularly useful when dealing with structured data or text files.
Here’s an example of using AWK to calculate the average age from our ‘data.txt’ file:
awk '{ sum += $3; n++ } END { if (n > 0) print sum / n; }' data.txt
# Output:
# [Expected average age]
In this command, sum += $3; n++
adds up the values in the third field (ages) and counts the lines. END { if (n > 0) print sum / n; }
calculates and prints the average age at the end. The output is the average age from the file.
Further Resources for AWK Proficiency
For those interested in exploring more about AWK and its capabilities, here are some valuable resources:
- GNU AWK User’s Guide: This comprehensive guide from GNU offers a deep dive into AWK and its features.
The AWK Programming Language: This book by AWK’s creators provides an in-depth look at the language and its applications.
Unix Text Processing: This guide provides a broad overview of text processing in Unix, including a section on AWK.
Recap: Column Extraction with AWK
In this comprehensive guide, we’ve explored the ins and outs of using AWK to print the first column of a file. AWK, a powerful text processing command in Unix, proves to be a versatile tool for data extraction and manipulation.
We began with the basics, demonstrating how to use AWK to print the first column of a file. We then delved into more complex usages of AWK, such as printing multiple columns or applying conditions to the print command. Along the way, we tackled common issues and their solutions, such as handling spaces or special characters in columns.
We also explored alternative methods for column extraction, such as using the cut command or Python scripts, giving you a broader perspective on the available tools.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
AWK | High | Moderate | Versatile for complex data extraction |
Cut | Low | Low | Simple column extraction |
Python Scripts | High | High | Complex data processing |
Whether you’re just starting out with AWK or you’re looking to level up your data extraction skills, we hope this guide has given you a deeper understanding of AWK and its capabilities.
With its balance of flexibility and power, AWK stands as a robust tool for column extraction in text files. Now, you’re well equipped to harness the full power of AWK. Happy coding!