AWK Regex Usage Guide | Pattern Matching in Linux/Unix
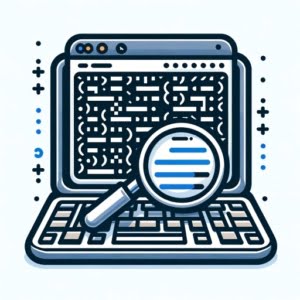
When scripting complex text processing tasks at IOFLOOD, understanding how to use regular expressions (regex) in AWK can help tremendously. In today’s article, we’ll dive into the usage of regex in AWK, providing practical examples and detailed explanations to assist our cloud server hosting customers and fellow developers in harnessing the power of regex for advanced text processing and scripting.
In this guide, we’ll walk you through the process of using regular expressions in AWK, from their creation, manipulation, and usage. We’ll cover everything from the basics of AWK regex to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use Regex in AWK?
To use regex in AWK, you can use the basic syntax,
awk '/pattern/' file.txt
. This allows AWK to match lines in a file or input that contain the pattern enclosed in the slashes.
Here’s a simple example:
echo 'hello' | awk '/^hel/ {print $0}'
# Output:
# 'hello'
In this example, we’re using the echo
command to create a string ‘hello’, and then we pipe this string into AWK. The AWK command searches for lines starting with the pattern “hel” (^hel). Since the input string “hello” starts with “hel,” the entire line “hello” is printed as the output.
This is just a basic way to use regex in AWK, but there’s much more to learn about pattern scanning and processing language. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
The Basics of AWK Regex
Regex, or regular expressions, are a powerful tool in AWK’s arsenal. They provide a way to match complex patterns in a text file or input. Let’s delve into the basics of using regex in AWK.
Creating and Using Simple Regex in AWK
To create a regex in AWK, you need to enclose your pattern within forward slashes (/
). AWK will then match lines that correspond to this pattern. Here’s a simple example of how you can use regex in AWK:
echo 'Hello, World!' | awk '/World/ {print $0}'
# Output:
# 'Hello, World!'
In this example, we’re using the echo
command to create a string ‘Hello, World!’, and then we pipe this string into AWK. The AWK command is looking for lines that match the regex pattern ‘World’, and when it finds a match, it prints the entire line (print $0
). In this case, the output is ‘Hello, World!’ because our string matches the regex pattern.
Advantages of Using AWK Regex
The use of AWK regex offers several advantages. It allows for flexible and powerful pattern matching, which can be especially useful when working with large and complex text files. Additionally, AWK’s syntax for regex is relatively straightforward, making it accessible for beginners.
Potential Pitfalls and How to Avoid Them
While AWK regex is powerful, there are some common pitfalls that beginners might encounter. One of these is forgetting to enclose the regex pattern within forward slashes. Without these, AWK will not recognize it as a regex pattern. Another common mistake is not properly escaping special characters in your regex pattern. Remember that characters like ‘.’, ‘*’, and ‘+’ have special meanings in regex and need to be escaped with a backslash (\
) if you want to match them literally.
In the next section, we’ll delve into more complex uses of regex in AWK, including different regex patterns and flags.
Advanced Regex Techniques in AWK
As you become more comfortable with basic AWK regex, you may find yourself needing more complex pattern matching. Let’s explore some advanced uses of regex in AWK, including different patterns and flags.
Utilizing Different Regex Patterns
Regex patterns can be as simple or as complex as you need them to be. For instance, you can use a period (‘.’) to match any single character, or an asterisk (‘*’) to match zero or more of the preceding character. Here’s an example:
echo 'Hello, World!' | awk '/o.*W/ {print $0}'
# Output:
# 'Hello, World!'
In this example, the regex pattern ‘o.*W’ matches any line that has an ‘o’ followed by any number of characters, and then a ‘W’. Since our string ‘Hello, World!’ fits this pattern, the entire line is printed.
Using Regex Flags in AWK
AWK also supports the use of regex flags, which can modify the behavior of your regex patterns. For instance, the ‘i’ flag makes your pattern case-insensitive. Here’s how you can use it:
echo 'Hello, World!' | awk 'BEGIN{IGNORECASE=1} /world/ {print $0}'
# Output:
# 'Hello, World!'
In this example, we’re using the BEGIN
block to set IGNORECASE
to 1, which makes our regex pattern case-insensitive. This means that ‘world’, ‘World’, and ‘WORLD’ would all match our pattern.
Mastering advanced AWK regex techniques can greatly improve your text processing abilities. In the next section, we’ll look at alternative approaches to using regex in AWK.
Alternate Pattern Matching Tools
While AWK’s regex is a powerful tool for , it’s not the only option available. There are other commands and functions that can accomplish similar tasks. Let’s explore some of these alternatives and when you might want to use them.
Using AWK’s Built-In String Functions
AWK comes with a variety of built-in string functions that can be used as alternatives to regex. For instance, you can use the index()
function to find the position of a substring within a string. Here’s an example:
echo 'Hello, World!' | awk '{print index($0, "World")}'
# Output:
# 8
In this example, we’re using index()
to find the position of ‘World’ in our string. The output is ‘8’, indicating that ‘World’ starts at the 8th character of our string.
Leveraging grep for Pattern Matching
Another alternative to AWK regex is the grep
command, which is specifically designed for pattern matching. Here’s how you can use it:
echo 'Hello, World!' | grep -o 'World'
# Output:
# 'World'
In this example, we’re using grep
with the ‘-o’ option to print only the parts of our string that match the pattern ‘World’. The output is ‘World’, as that’s the part of our string that matches our pattern.
Deciding Between AWK Regex and Alternatives
When deciding between using AWK regex or an alternative approach, consider the complexity of your task and the tools you’re most comfortable with. AWK regex is powerful and flexible, making it a great choice for complex pattern matching. However, for simpler tasks or if you’re more comfortable with another tool, using an alternative might be the better choice.
In the next section, we’ll discuss common troubleshooting tips and considerations when working with AWK regex.
Troubleshooting Errors: AWK Regex
Like with any programming tool, using AWK regex can sometimes lead to errors or obstacles. Let’s go over some of the most common issues you might encounter and how to solve them, along with tips for best practices and optimization.
Handling Special Characters in AWK Regex
One common issue when working with AWK regex is handling special characters. These characters have a particular meaning in regex and need to be escaped with a backslash (\
) to be taken literally. Forgetting to do so can lead to unexpected results. Here’s an example:
echo 'Hello, World!' | awk '/Hello, World!/ {print $0}'
# Output:
# (no output)
In this example, we’re trying to match the string ‘Hello, World!’ exactly, but we’re not getting any output. That’s because the comma (‘,’) and the exclamation mark (‘!’) are special characters in regex and need to be escaped. Here’s the corrected command:
echo 'Hello, World!' | awk '/Hello\, World\!/ {print $0}'
# Output:
# 'Hello, World!'
Avoiding Overuse of AWK Regex
While AWK regex is a powerful tool, it’s also easy to overuse. Over-reliance on regex can make your AWK scripts hard to read and maintain, especially for more complex patterns. As a best practice, consider using AWK’s built-in string functions or other alternatives for simpler tasks.
Optimizing AWK Regex for Performance
When working with large files, the performance of your AWK scripts can become a concern. One way to optimize your AWK regex is by using the most specific pattern possible. This reduces the amount of backtracking AWK has to do, speeding up your script.
As you continue to use AWK regex, you’ll likely encounter your own unique challenges. However, with these troubleshooting tips and considerations in mind, you’ll be well-equipped to tackle them.
Fundamentals of AWK and Regex
To fully appreciate the power of AWK regex, it’s important to understand the fundamentals of both AWK and regular expressions. Let’s delve deeper into these concepts and discuss some related commands and broader ideas.
Understanding AWK
AWK is a versatile programming language designed for text processing. It’s particularly adept at handling structured text data, like tables or databases. AWK reads input line by line, and performs actions on each line based on its content.
Grasping Regular Expressions
Regular expressions, or regex, are a method of representing patterns in text data. They’re used in various programming languages, including AWK, to search, edit, or manipulate text. Regex can range from simple patterns, like a specific word, to complex patterns that match a variety of different text structures.
The Power of AWK Regex
The combination of AWK and regex forms a powerful tool for text processing. AWK’s ability to read and manipulate text data, combined with regex’s flexible pattern matching, allows for precise and efficient text processing. Here’s an example of how AWK regex can be used to match lines with a specific number of characters:
echo -e 'Hello \nWorld' | awk 'length($0) == 5 {print $0}'
# Output:
# Hello
# World
In this example, we’re using the length()
function to match lines that have exactly 5 characters. The lines that match this criteria are ‘Hello’ and ‘World’, so these are printed as the output.
Related Commands and Broader Ideas
AWK and regex are part of a broader ecosystem of text processing tools in Unix-like operating systems. Commands like grep
, sed
, and perl
also use regex and can be used in combination with AWK for more complex text processing tasks. Understanding AWK and regex can also provide a foundation for learning these related tools.
In the next section, we’ll discuss how the application of regex in AWK can be used in larger scripts or projects.
Script Usages of AWK Regex
As you become more proficient in using AWK regex, you’ll find it’s not just useful for small tasks. It can be a powerful tool in larger scripts and projects, providing flexible and efficient text processing capabilities.
Integrating AWK Regex in Scripts
AWK, with its regex capabilities, can be seamlessly integrated into larger shell scripts. It can handle complex tasks like parsing log files, transforming data formats, generating reports, and more. Here’s an example of how AWK regex can be used in a script to process a log file:
# Assume we have a log file with entries in the format: 'DATE: EVENT'
awk '/ERROR/ {print $0}' /var/log/mylogfile.log
# Output:
# (Lines from the log file that contain 'ERROR')
This script uses AWK regex to scan a log file and print out any lines that contain the word ‘ERROR’. This can be useful for quickly identifying issues in your logs.
AWK and Related Commands
AWK often works in tandem with other commands for efficient text processing. Commands like grep
for filtering, sed
for substitution, and sort
for ordering lines often accompany AWK in scripts. By combining these commands, you can build powerful text processing pipelines.
Further Resources for Mastering AWK Regex
To continue your journey in mastering AWK regex, consider these additional resources:
- GNU Awk User’s Guide: This guide from the GNU project provides comprehensive information about AWK, including its regex capabilities.
The AWK Programming Language: This book by AWK’s original creators offers in-depth insights and practical examples.
Regular-Expressions.info: A valuable resource for understanding and mastering regular expressions in various languages, including AWK.
In the next section, we’ll consolidate the information and important points discussed in this article.
Wrapping Up: Mastering AWK Regex
In this comprehensive guide, we’ve navigated the world of AWK regex, a powerful tool for text processing in AWK.
We began with the basics, understanding how to create and use simple regex patterns in AWK. We then advanced to more complex uses of regex, including different patterns and flags. We also discussed alternative approaches to using regex in AWK, such as its built-in string functions and the grep
command.
Along the way, we tackled common challenges you might face when using AWK regex, such as handling special characters and optimizing your scripts for performance. We also provided solutions and best practices to help you overcome these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Basic AWK Regex | Moderate | Low | Simple pattern matching |
Advanced AWK Regex | High | High | Complex pattern matching |
AWK String Functions | Low | Low | Simple text manipulation |
grep Command | High | Moderate | Filtering lines based on a pattern |
Whether you’re just starting out with AWK regex or you’re looking to enhance your text processing skills, we hope this guide has given you a deeper understanding of AWK regex and its capabilities.
With its blend of flexibility and power, AWK regex is an indispensable tool for text processing in Unix-like operating systems. Happy coding!