Using AWK ‘split’ Function | Field Separation Techniques
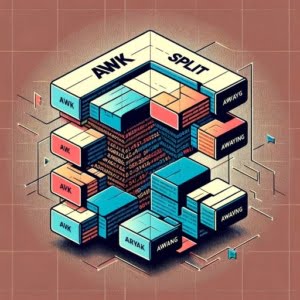
Exploring text processing functionalities at IOFLOOD often involves testing practical usage cases of specialized functions like ‘split’ in AWK. Through our experience we have found that the ‘split’ function divides strings into arrays, based on delimiters, which enables us to easily handle data parsing tasks. In today’s article, we’ll explore into the usage of the ‘split’ function in AWK, to equip our dedicated cloud service customers and fellow developers with the knowledge needed for data parsing in Unix/Linux environments.
In this guide, we’ll walk you through the process of using the ‘split’ function in AWK, from the basics to more advanced techniques. We’ll cover everything from simple string splitting, handling different delimiters, to dealing with multi-line records and even troubleshooting common issues.
Let’s dive in and start mastering the AWK ‘split’ function!
TL;DR: How Do I Use the ‘split’ Function in AWK?
The
'split'
function in AWK is a powerful tool that allows you to divide a string into pieces based on a specified delimiter. It is used with the basic syntax,awk '{split($0, array, "delimiter"); print array[index]}' file.txt
.
Here’s a simple example:
echo 'Hello World' | awk '{split($0,a," "); print a[1]}'
# Output:
# 'Hello'
In this example, we use the ‘split’ function to divide the string ‘Hello World’ into two pieces, ‘Hello’ and ‘World’. The function takes three arguments: the string to split, an array to store the pieces, and a delimiter to split the string. In this case, we use a space as the delimiter. The ‘split’ function divides the string at each space and stores the pieces in the array ‘a’. We then print the first piece, ‘Hello’.
This is just a basic way to use the ‘split’ function in AWK, but there’s much more to learn about string splitting and data processing. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with AWK ‘split’
The ‘split’ function in AWK is a fundamental tool for text processing and data manipulation. It provides a simple and efficient way to break a string into smaller parts, making it easier to handle and analyze.
Let’s take a closer look at how this function works.
Breaking Down the ‘split’ Function
The ‘split’ function in AWK takes three arguments: the string you want to split, an array to store the split parts, and a delimiter to determine where to split the string.
Here’s a simple example to illustrate how it works:
echo 'Learn AWK Split Function' | awk '{split($0,a," "); print a[2]}'
# Output:
# 'AWK'
In this example, we use the ‘split’ function to divide the string ‘Learn AWK Split Function’ into four pieces: ‘Learn’, ‘AWK’, ‘Split’, and ‘Function’. We specify a space as the delimiter, so the function splits the string at each space and stores the pieces in the array ‘a’. We then print the second piece, ‘AWK’.
Advantages and Pitfalls of the ‘split’ Function
The ‘split’ function is a versatile tool for text processing. It allows you to break down a string into smaller parts, making it easier to analyze and manipulate the data. This can be particularly useful when dealing with large text files or complex data structures.
However, there are a few potential pitfalls to be aware of. The ‘split’ function can only split strings based on a single character or a regular expression. If you need to split a string based on multiple characters or a complex pattern, you may need to use a different method.
Additionally, the ‘split’ function does not change the original string. It creates a new array with the split parts, leaving the original string intact. This can be an advantage if you need to preserve the original data, but it can also consume more memory if you’re working with large strings or large arrays.
Advanced Uses of ‘split’ Function
As you become more comfortable with the ‘split’ function in AWK, you can start to explore more complex usage scenarios. Let’s take a look at how you can use different delimiters and handle multi-line records.
Using Different Delimiters
By default, the ‘split’ function uses a space as the delimiter. However, you can specify any character or regular expression as the delimiter. For example, you can split a string at each comma, colon, or even each letter.
Here’s an example of how to use a comma as the delimiter:
echo 'apple,banana,cherry' | awk '{split($0,a,", "); print a[1], a[2], a[3]}'
# Output:
# 'apple' 'banana' 'cherry'
In this example, we split the string ‘apple,banana,cherry’ into three pieces: ‘apple’, ‘banana’, and ‘cherry’. We specify a comma as the delimiter, so the function splits the string at each comma.
Handling Multi-line Records
The ‘split’ function can also handle multi-line records. This can be particularly useful when dealing with large text files or complex data structures.
Here’s an example of how to split a multi-line record:
echo -e 'apple\nbanana\ncherry' | awk '{split($0,a,"\n"); print a[1], a[2], a[3]}'
# Output:
# 'apple' 'banana' 'cherry'
In this example, we split a multi-line record into three pieces: ‘apple’, ‘banana’, and ‘cherry’. We specify a newline character (‘\n’) as the delimiter, so the function splits the record at each newline.
These advanced techniques can open up new possibilities for text processing and data manipulation with the AWK ‘split’ function.
Alternate Tools: Split Strings in AWK
While the ‘split’ function is a powerful tool in AWK, it’s not the only way to divide strings. Let’s explore some alternative methods, like using the ‘gsub’ function or the ‘FS’ variable.
Using the ‘gsub’ Function
The ‘gsub’ function in AWK can replace all occurrences of a pattern in a string. You can use it to replace a delimiter with a newline character, effectively splitting the string into multiple lines.
Here’s an example:
echo 'apple,banana,cherry' | awk '{gsub(",","\n"); print}'
# Output:
# 'apple'
# 'banana'
# 'cherry'
In this example, we use the ‘gsub’ function to replace each comma in the string ‘apple,banana,cherry’ with a newline character. This splits the string into three lines.
The ‘gsub’ function provides a flexible way to manipulate strings, but it changes the original string, unlike the ‘split’ function. This could be a drawback if you need to preserve the original data.
Using the ‘FS’ Variable
The ‘FS’ variable in AWK stands for ‘Field Separator’. It specifies the character or regular expression that separates fields in a record. By changing the ‘FS’ variable, you can split a string into fields based on a specified delimiter.
Here’s an example of how to use the ‘FS’ variable:
echo 'apple:banana:cherry' | awk 'BEGIN {FS=":"} {print $1, $2, $3}'
# Output:
# 'apple' 'banana' 'cherry'
In this example, we set the ‘FS’ variable to a colon. This tells AWK to split the string ‘apple:banana:cherry’ into fields at each colon.
The ‘FS’ variable provides a simple way to split strings, especially when dealing with structured data like CSV files. However, it only affects the way AWK reads records, not the way it prints them. To change the output delimiter, you need to use the ‘OFS’ (Output Field Separator) variable.
Each of these methods has its benefits and drawbacks, and the best one to use depends on your specific needs. Whether you choose the ‘split’ function, the ‘gsub’ function, or the ‘FS’ variable, AWK provides a versatile toolkit for string splitting and data processing.
Troubleshooting AWK ‘split’ Function
As with any tool, you may encounter some challenges when using the AWK ‘split’ function. Let’s explore some common issues and their solutions.
Handling Special Characters
Special characters, like backslashes or quotes, can cause unexpected behavior when splitting strings. This is because AWK interprets these characters as part of the syntax, not as part of the string.
To handle special characters, you can use the escape character (‘\’). This tells AWK to treat the following character as a literal character, not a special character.
Here’s an example of how to split a string with a backslash:
echo 'apple\banana\cherry' | awk '{split($0,a,"\\"); print a[1], a[2], a[3]}'
# Output:
# 'apple' 'banana' 'cherry'
In this example, we use two backslashes (‘\\’) as the delimiter. The first backslash is the escape character, and the second backslash is the literal character. This allows us to split the string at each backslash.
Dealing with Empty Fields
When splitting a string, you may end up with empty fields. This can happen if there are multiple delimiters in a row, or if the string starts or ends with a delimiter.
To handle empty fields, you can check the length of each field before using it. If the length is zero, you can skip the field or replace it with a default value.
Here’s an example of how to handle empty fields:
echo 'apple,,cherry' | awk '{split($0,a,", "); for(i in a) if (length(a[i]) != 0) print a[i]}'
# Output:
# 'apple'
# 'cherry'
In this example, we split the string ‘apple,,cherry’ into three fields: ‘apple’, an empty field, and ‘cherry’. We then print each field only if its length is not zero, effectively skipping the empty field.
These are just a few of the issues you may encounter when using the AWK ‘split’ function. With a bit of practice and troubleshooting, you can overcome these challenges and use the ‘split’ function effectively.
AWK’s String Handling Capabilities
AWK, an acronym for the creators Aho, Weinberger, and Kernighan, is a powerful text processing language. It’s particularly adept at handling strings, making it a go-to tool for tasks involving text files or data streams.
AWK’s String Processing Power
One of AWK’s strengths is its ability to process strings. It can read and write strings, concatenate them, search for patterns, and of course, split them into parts. This makes it a versatile tool for tasks like data extraction, report generation, and even some types of data analysis.
echo 'AWK is a powerful string processing tool' | awk '{print $1, $5, $6}'
# Output:
# 'AWK string processing'
In this example, AWK reads the string ‘AWK is a powerful string processing tool’ and prints the first, fifth, and sixth words, demonstrating its ability to handle and manipulate strings.
The ‘split’ Function: A Key Player in String Handling
Among AWK’s string handling capabilities, the ‘split’ function stands out. It’s one of AWK’s built-in functions specifically designed for string manipulation. The ‘split’ function can divide a string into an array of substrings based on a specified delimiter.
This function is particularly useful when you need to dissect a string into parts for further processing. Whether you’re parsing a log file, processing user input, or manipulating data, the ‘split’ function is an essential tool in your AWK toolkit.
echo 'apple-banana-cherry' | awk '{split($0,a,"-"); print a[1], a[2], a[3]}'
# Output:
# 'apple' 'banana' 'cherry'
In this example, we use the ‘split’ function to divide the string ‘apple-banana-cherry’ into three parts: ‘apple’, ‘banana’, and ‘cherry’. We specify a hyphen as the delimiter, so the function splits the string at each hyphen.
These powerful string handling capabilities, combined with the flexibility and simplicity of the ‘split’ function, make AWK an invaluable tool for text processing and data manipulation.
AWK ‘split’: Beyond String Splitting
While the ‘split’ function in AWK is primarily used for dividing strings, its applications extend far beyond this basic use case. It plays a crucial role in various data processing tasks, file handling operations, and more. Let’s delve into these broader applications and related concepts you might want to explore.
AWK ‘split’ in Data Processing
Data processing often involves parsing and manipulating text files or data streams. Here, the ‘split’ function becomes a valuable ally. By dividing strings into manageable parts, it enables more effective data analysis and extraction.
echo 'name:John,age:30,city:NY' | awk '{split($0,a,", "); for(i in a) {split(a[i],b,":"); print b[1],"=",b[2]}}'
# Output:
# 'name = John'
# 'age = 30'
# 'city = NY'
In this example, we’re processing a data string that contains a person’s name, age, and city, separated by commas. The ‘split’ function is used twice: first to divide the string into individual data points, and then to separate each data point into a key-value pair.
File Handling with AWK ‘split’
When dealing with file handling tasks, the ‘split’ function can be used to parse file paths, extract file names, or process file contents.
echo '/home/user/document.txt' | awk -F/ '{print $NF}'
# Output:
# 'document.txt'
In this example, we’re extracting the file name from a file path. The ‘split’ function, represented here by the -F option, divides the file path into parts using the slash as a delimiter. The $NF variable then prints the last part, which is the file name.
Exploring Related Concepts
As you continue to master the ‘split’ function, you may want to explore related concepts like regular expressions in AWK and field separation. These concepts can further enhance your string manipulation and data processing skills.
Further Resources for Mastering AWK ‘split’
To deepen your understanding of AWK and the ‘split’ function, consider exploring these resources:
- GNU AWK User’s Guide: A comprehensive guide to AWK, including detailed information about the ‘split’ function.
The AWK Programming Language: A book by AWK’s creators, providing insights into the language’s capabilities and use cases.
AWK Tutorial by TutorialsPoint: A step-by-step tutorial covering the basics of AWK and its functions, including ‘split’.
Recap: AWK ‘split’ Usage Guide
In this comprehensive guide, we’ve journeyed through the intricate world of AWK’s ‘split’ function, a powerful tool for string manipulation and data processing tasks.
We embarked our journey with the basics, learning how to use the ‘split’ function to divide strings into manageable parts. We then ventured into more advanced territory, exploring complex usage scenarios, such as using different delimiters and handling multi-line records.
Along the way, we tackled common challenges you might face when using the ‘split’ function, such as handling special characters and dealing with empty fields, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to splitting strings in AWK, comparing the ‘split’ function with other methods like using the ‘gsub’ function or the ‘FS’ variable. Here’s a quick comparison of these methods:
Method | Flexibility | Memory Efficiency | Complexity |
---|---|---|---|
‘split’ Function | High | Moderate | Low |
‘gsub’ Function | Moderate | Low | Moderate |
‘FS’ Variable | Low | High | Low |
Whether you’re just starting out with AWK or you’re looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of the ‘split’ function and its capabilities.
With its balance of flexibility, memory efficiency, and simplicity, the ‘split’ function is a powerful tool for string manipulation in AWK. Now, you’re well equipped to tackle any string splitting tasks that come your way. Happy coding!