AWK Substring Function | Unix String Manipulation Guide
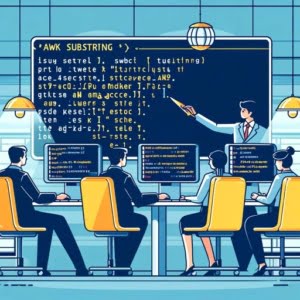
Developing scripts for text processing tasks at IOFLOOD often requires utilizing specialized functions like the substring function in AWK. We regularly use the function to extract specific portions of strings based on defined positions and lengths, for consistent and precise data manipulation. In this guide, we’ll share our tips and tricks on the usage of the substring function in AWK, to empower dedicated server hosting customers and fellow developers.
In this guide, we’ll walk you through the process of using the AWK substring function, from the basics to more advanced techniques. We’ll cover everything from simple string extraction to complex uses with regular expressions or in combination with other AWK functions. We’ll also discuss alternative approaches for string manipulation in AWK, common pitfalls, and their solutions.
Let’s dive in and start mastering the AWK substring function!
TL;DR: How Do I Use the Substring Function in AWK?
The AWK substring function is used to extract a specific part of a string. The syntax is
substring($0, start, length)
, where$0
is the string,start
is the position where the substring starts, andlength
is the length of the substring.
Here’s a simple example:
echo 'Hello, World!' | awk '{print substr($0, 8, 5)}'
# Output:
# 'World'
In this example, we’re using the AWK substring function to extract the word ‘World’ from the string ‘Hello, World!’. The substring starts at the 8th character and has a length of 5 characters.
This is just a basic way to use the AWK substring function, but there’s much more to learn about string manipulation in AWK. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with AWK Substring
The AWK substring function is a powerful tool for text manipulation. It allows you to extract a specific part of a string, which can be incredibly useful in many scenarios, such as parsing log files or processing user input.
Let’s look at a simple example to understand how it works:
echo 'The quick brown fox jumps over the lazy dog' | awk '{print substr($0, 5, 5)}'
# Output:
# 'quick'
In this example, we’re using the AWK substring function to extract the word ‘quick’ from the string. The substring function takes three parameters: the string, the start position, and the length of the substring. Here, the string is $0
(which represents the entire line), the start position is 5
, and the length is 5
.
This is a basic usage of the AWK substring function. It’s simple but powerful, and it’s a great starting point for learning more complex string manipulation techniques in AWK.
Benefits of AWK Substring
The AWK substring function is a versatile tool with many benefits. It allows you to extract precise information from your data, which can be essential in data analysis or text processing tasks. The function is flexible and can be used in a wide range of scenarios.
Potential Pitfalls
While the AWK substring function is incredibly useful, there are a few potential pitfalls to be aware of. For example, if the start position is beyond the end of the string, or if the length is longer than the remaining part of the string from the start position, the function will return an empty string. It’s important to ensure that your start position and length are within the bounds of the string to avoid unexpected results.
Advanced AWK Substring Techniques
As you become more comfortable with the AWK substring function, you can start to explore more complex uses. This includes using the function with regular expressions, or in combination with other AWK functions. Let’s delve into these advanced techniques.
AWK Substring with Regular Expressions
Regular expressions (regex) are a powerful tool for pattern matching in strings. They can be used with the AWK substring function to extract specific patterns from your data.
Here’s an example:
awk '{match($0, /quick ([a-z]+)/, arr); print arr[1]}' <<< 'The quick brown fox jumps over the lazy dog'
# Output:
# 'brown'
In this example, we’re using the match
function to find the word ‘quick’ followed by a space and a sequence of lowercase letters. The matched string is stored in the arr
array, and we’re printing the first element of the array, which is the sequence of lowercase letters following ‘quick’.
Combining AWK Substring with Other Functions
The AWK substring function can also be used in combination with other AWK functions to achieve more complex text manipulation tasks. For instance, you can use the length
function to get the length of the substring.
Here’s an example:
awk '{print length(substr($0, 5, 5))}' <<< 'The quick brown fox jumps over the lazy dog'
# Output:
# '5'
In this example, we’re using the length
function to get the length of the substring extracted by the substr
function. The output is 5
, which is the length of the word ‘quick’.
These advanced techniques can significantly enhance your text processing capabilities with AWK. They allow you to perform complex string manipulations and extract precise information from your data.
Exploring Alternative Methods in AWK
While the AWK substring function is a powerful tool for string manipulation, AWK also offers other functions that can be used for similar purposes. Let’s explore some of these alternatives, such as the split
and gsub
functions.
AWK Split Function
The split
function in AWK is used to split a string into an array of substrings. It’s a great tool when you need to break down a string into smaller parts.
Here’s an example:
awk '{split($0, arr, " "); print arr[2]}' <<< 'The quick brown fox jumps over the lazy dog'
# Output:
# 'quick'
In this example, we’re using the split
function to split the string into an array arr
using a space as the delimiter. We then print the second element of the array, which is the word ‘quick’.
AWK Gsub Function
The gsub
function in AWK is used to replace all occurrences of a pattern in a string. It can be used for more complex string manipulation tasks.
Here’s an example:
awk '{gsub(/fox/, "cat"); print $0}' <<< 'The quick brown fox jumps over the lazy dog'
# Output:
# 'The quick brown cat jumps over the lazy dog'
In this example, we’re using the gsub
function to replace all occurrences of the word ‘fox’ with the word ‘cat’ in the string.
Deciding Between AWK Substring and Alternatives
Choosing between the AWK substring function and its alternatives depends on your specific needs. If you need to extract a specific part of a string, the substr
function is the way to go. If you need to split a string into an array of substrings, the split
function is a better choice. If you need to replace all occurrences of a pattern in a string, the gsub
function is your best bet.
Each of these functions has its own benefits and drawbacks, and understanding these can help you make the right decision for your text processing tasks.
Troubleshooting Substrings in AWK
As with any programming function, you may encounter some challenges or obstacles when using the AWK substring function. Let’s discuss some common issues you might face and how to solve them.
Out-of-Bounds Substring
One common issue is trying to extract a substring that’s out-of-bounds. When the start position is beyond the end of the string or the length is longer than the remaining part of the string from the start position, the AWK substring function will return an empty string.
awk '{print substr($0, 50, 5)}' <<< 'The quick brown fox jumps over the lazy dog'
# Output:
# ''
In this example, we’re trying to extract a substring starting from the 50th character. However, the string is only 44 characters long, so the function returns an empty string.
Incorrectly Specified Start or Length
Another common issue is incorrectly specifying the start position or length. Remember, the start position is the position in the string where the substring starts, and the length is the length of the substring. Both should be positive integers.
awk '{print substr($0, -5, 5)}' <<< 'The quick brown fox jumps over the lazy dog'
# Output:
# 'Error: start position cannot be negative'
In this example, we’re trying to extract a substring with a negative start position, which is not allowed. The function returns an error message.
Handling Special Characters
Special characters in the string can sometimes cause unexpected results. For example, if you’re trying to extract a substring that includes a newline character, the function might not behave as expected.
awk '{print substr($0, 5, 5)}' <<< $'The quick\nbrown fox jumps over the lazy dog'
# Output:
# 'quick'
In this example, we’re trying to extract a substring from a string that includes a newline character. The function still works as expected, but the output might not be what you intended.
Understanding these potential pitfalls can help you use the AWK substring function more effectively and avoid common errors.
AWK Programming: A Closer Look
AWK is a powerful programming language designed for text processing and typically used as a data extraction and reporting tool. It’s a standard feature of most Unix-like operating systems, but it’s also available for other platforms.
Understanding AWK’s String Handling Capabilities
One of AWK’s key strengths is its robust string handling capabilities. It provides a suite of functions for manipulating strings, with the substr
function being one of the most commonly used.
awk '{print substr($0, 1, 3)}' <<< 'Hello, World!'
# Output:
# 'Hel'
In this example, we’re using the substr
function to extract the first three characters of the string ‘Hello, World!’. The output is ‘Hel’. AWK’s string handling functions like substr
allow you to perform complex text processing tasks with relative ease.
Delving into Substrings in AWK
A substring is a part of a string. In AWK, you can extract a substring from a string using the substr
function. This function takes a string, a start position, and a length, and it returns the substring that starts at the specified position and has the specified length.
awk '{print substr($0, 8, 5)}' <<< 'Hello, World!'
# Output:
# 'World'
In this example, we’re extracting the substring ‘World’ from the string ‘Hello, World!’. The substr
function starts at the 8th character and extracts a substring of length 5.
Understanding the fundamentals of AWK and the concept of substrings is crucial to effectively using the substr
function and other string handling functions in AWK.
Further Learning: AWK Substrings
The AWK substring function is not just a standalone tool; it’s a part of a larger toolkit for text processing and data analysis. You can integrate it into larger scripts or projects, and it often works in tandem with other AWK functions.
Integrating AWK Substring in Larger Scripts
In a larger script, you might use the AWK substring function to extract specific parts of your data for further processing. For example, you might extract timestamps from log entries, or usernames from email addresses.
awk -F: '{print substr($1, 1, 5)}' /etc/passwd
# Output:
# 'root'
# 'daemo'
# 'bin'
# 'sys'
# 'sync'
In this example, we’re using the AWK substring function in a script that processes the /etc/passwd
file, which contains user account information. The script extracts the first five characters of each username.
Complementary AWK Functions
The AWK substring function often works hand in hand with other AWK functions. For example, you might use the length
function to determine the length of the substring, or the index
function to find the position of the substring in the string.
awk '{print length(substr($0, 1, 5))}' <<< 'Hello, World!'
# Output:
# '5'
In this example, we’re using the length
function to determine the length of the substring extracted by the substr
function. The output is 5
, which is the length of the word ‘Hello’.
Further Resources for Mastering AWK
If you’re interested in learning more about AWK and its powerful functions, here are a few resources to explore:
- GNU AWK User’s Guide: This comprehensive guide covers all aspects of AWK, including its string handling functions.
The AWK Programming Language: This book, written by the creators of AWK, provides a deep dive into the language and its capabilities.
AWK Tutorial by TutorialsPoint: This online tutorial provides a step-by-step guide to learning AWK, including its string handling functions.
Recap: Handling Substrings with AWK
In this comprehensive guide, we’ve delved into the world of AWK, focusing on the powerful substring function that allows for precise text manipulation.
We kicked off with the basics, learning how to use the AWK substring function in its simplest form. We then ventured into more advanced territory, exploring complex uses of the function, such as using it with regular expressions or in combination with other AWK functions.
Along the way, we tackled common challenges you might face when using the AWK substring function, such as out-of-bounds substrings and incorrectly specified start or length parameters, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to text manipulation in AWK, comparing the substring function with other functions like split
and gsub
. Here’s a quick comparison of these functions:
Function | Use Case | Complexity |
---|---|---|
substr | Extracting specific parts of a string | Moderate |
split | Splitting a string into an array of substrings | Low |
gsub | Replacing all occurrences of a pattern in a string | High |
Whether you’re just starting out with AWK or you’re looking to level up your text manipulation skills, we hope this guide has given you a deeper understanding of the AWK substring function and its capabilities.
With its balance of precision and flexibility, the AWK substring function is a powerful tool for text manipulation. Now, you’re well equipped to enjoy those benefits. Happy coding!