Bash ‘&&’ Operator: Chaining Commands in Shell Script
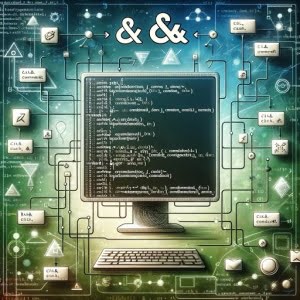
Are you finding it difficult to execute multiple commands in a sequence in Bash? You’re not alone. Many developers find themselves in a similar situation, but there’s a method in Bash that can make this process smooth and efficient.
Think of the '&&'
operator in Bash as a strict supervisor, ensuring that each task is completed successfully before moving on to the next. This command allows you to execute multiple commands in a sequence, but only if the previous command executes successfully.
In this guide, we’ll walk you through the basics to advanced usage of the ‘&&’ command in Bash. We’ll cover everything from the basic use of ‘&&’, its advanced usage scenarios, alternative approaches, and even troubleshooting common issues.
So, let’s dive in and start mastering the ‘&&’ command in Bash!
TL;DR: How Do I Use the ‘&&’ Operator in Bash?
The
'&&'
operator in Bash allows for the execution of multiple commands in sequence, but only if the previous command executes successfully. It can be used between two commands, such asmkdir new_directory && cd new_directory
. It’s like a strict supervisor, ensuring each task is completed before moving on to the next.
Here’s a quick example:
mkdir new_directory && cd new_directory
# Output:
# (Assuming no error, your terminal's prompt will now show you're in 'new_directory')
In this example, we first create a new directory with mkdir new_directory
, then navigate into it with cd new_directory
. The ‘&&’ command ensures that the second command (cd new_directory
) only runs if the first command (mkdir new_directory
) executes successfully.
This is just a basic usage of the ‘&&’ command in Bash. There’s much more to learn about it, including advanced usage scenarios and alternatives. Continue reading for a more detailed understanding.
Table of Contents
Understanding the Basics of Bash && Command
The ‘&&’ command in Bash is a logical operator that allows you to execute multiple commands in sequence. The key feature of this command is that the next command in the sequence will only execute if the previous command has executed successfully. In other words, if the first command fails, the second command won’t run.
Here’s a simple example of how the ‘&&’ command works:
echo 'Hello, Bash' && echo 'You have mastered the basic use of &&'
# Output:
# 'Hello, Bash'
# 'You have mastered the basic use of &&'
In this example, the first command echo 'Hello, Bash'
prints ‘Hello, Bash’ to the terminal. The second command echo 'You have mastered the basic use of &&'
only runs because the first command was successful. If the first command had failed for any reason, the second command would not have executed.
Advantages of Using &&
The ‘&&’ command can make your scripts more efficient and error-proof. By ensuring that each command only runs if the previous command was successful, you can prevent many common errors that occur when a command depends on the success of a previous command.
Potential Pitfalls
While the ‘&&’ command is incredibly useful, it’s important to remember that it only checks if the previous command was successful. It does not check if the output of the previous command is correct or as expected. Therefore, it’s still crucial to test your scripts thoroughly and ensure your commands are producing the correct output.
Bash && Command: Beyond the Basics
As we delve deeper into the world of Bash scripting, the ‘&&’ command proves to be even more useful. It’s not just about executing two commands; ‘&&’ can be used with numerous commands, allowing you to create complex scripts with multiple dependencies.
Using && in Scripts
Take a look at this intermediate-level example where we create a directory, navigate into it, create a file, and then write to that file – all in one line:
mkdir intermediate_directory && cd intermediate_directory && touch hello.txt && echo 'Hello, Intermediate Level!' > hello.txt
# Output:
# (Assuming no error, 'Hello, Intermediate Level!' will be written in 'hello.txt' inside 'intermediate_directory')
In this example, we execute four commands in sequence. Each command only runs if the previous command was successful. This ensures that we don’t attempt to navigate into a directory that wasn’t created, or write to a file that doesn’t exist.
Combining && with Other Commands
The ‘&&’ command can also be used in combination with other commands, such as ‘||’, which executes the next command if the previous command fails. This allows you to handle both success and failure scenarios in your scripts.
Here’s an example:
echo 'This will run' && (false || echo 'This will also run')
# Output:
# 'This will run'
# 'This will also run'
In this example, even though the command ‘false’ fails, the echo command after the ‘||’ still runs. This is because ‘||’ executes the next command if the previous command fails, providing a way to handle failures in your scripts.
The ‘&&’ command in Bash is a powerful tool that can greatly enhance your scripts when used effectively. With a good understanding of this command, you’re well on your way to becoming a Bash scripting expert.
Exploring Alternatives to Bash && Command
While the ‘&&’ command is a powerful tool in Bash scripting, it’s not the only method to execute commands in sequence. There are alternative approaches that offer different behaviors and can be more suitable in certain scenarios. Let’s explore two of these alternatives: the ‘||’ and ‘;’ commands.
The ‘||’ Command: Execute on Failure
The ‘||’ command in Bash is a logical OR operator. It will execute the next command if the previous command fails. This is the opposite of ‘&&’, which only executes the next command if the previous command is successful.
Here’s an example of how the ‘||’ command works:
false || echo 'The previous command failed'
# Output:
# 'The previous command failed'
In this example, the command ‘false’ will always fail. Therefore, the echo command after the ‘||’ operator will execute, printing ‘The previous command failed’ to the terminal.
The ‘;’ Command: Execute Regardless
The ‘;’ command in Bash allows you to execute multiple commands in sequence, regardless of whether the previous command was successful or not.
Here’s an example of how the ‘;’ command works:
false ; echo 'This command will run regardless'
# Output:
# 'This command will run regardless'
In this example, even though the ‘false’ command fails, the echo command after the ‘;’ operator still runs, printing ‘This command will run regardless’ to the terminal.
Choosing the Right Command
While ‘&&’, ‘||’, and ‘;’ all allow you to execute multiple commands in sequence, they offer different behaviors that can be more or less suitable depending on your needs. ‘&&’ is ideal when you want to ensure each command only runs if the previous command was successful. ‘||’ is useful for handling failure scenarios, and ‘;’ allows you to run multiple commands regardless of their success or failure.
Understanding these commands and when to use them can make your Bash scripts more robust and efficient.
Common Issues and Solutions with Bash && Command
Like any other command in Bash, using ‘&&’ can sometimes lead to unexpected results or errors. Let’s discuss some common issues that you may encounter when using the ‘&&’ command and how to troubleshoot them.
Command Execution Failure
One of the most common issues is when a command fails to execute successfully, preventing subsequent commands in the sequence from running. This is actually the intended behavior of ‘&&’, but it can be problematic if the failure wasn’t anticipated.
Suppose you have the following sequence of commands:
cd non_existent_directory && echo 'This will not print'
# Output:
# cd: non_existent_directory: No such file or directory
In this example, the cd
command tries to navigate into a directory that doesn’t exist, causing it to fail. As a result, the echo
command after the ‘&&’ doesn’t run.
Handling Command Failure
To handle such scenarios, you can use the ‘||’ command to provide a fallback command that will execute if a command fails. Here’s how you can modify the previous example to handle the failure:
cd non_existent_directory || echo 'Failed to change directory'
# Output:
# 'Failed to change directory'
In this updated example, the echo
command after the ‘||’ will run if the cd
command fails, providing a way to handle the failure.
Considerations When Using &&
When using the ‘&&’ command, it’s important to anticipate potential failures and handle them appropriately. Remember that ‘&&’ will only execute the next command if the previous command was successful. If a command might fail, consider providing a fallback command using ‘||’.
Mastering the ‘&&’ command in Bash involves not only understanding how to use it, but also how to troubleshoot common issues and handle potential failures.
Bash Scripting and Command Execution Basics
To fully grasp the power and utility of the ‘&&’ command in Bash, it’s crucial to understand the fundamentals of Bash scripting and command execution.
Understanding Bash Scripting
Bash, or the Bourne Again SHell, is a popular command-line interpreter. It allows users to interact with their operating system by typing commands into a terminal. Bash scripting is the art of stringing together these commands to automate tasks, manipulate data, and create new functionalities.
Here’s a simple Bash script example:
#!/bin/bash
# This is a comment
echo 'Hello, Bash scripting!'
# Output:
# 'Hello, Bash scripting!'
In this script, we use the echo
command to print ‘Hello, Bash scripting!’ to the terminal. The first line #!/bin/bash
is called a shebang and it tells the system to execute the script using Bash.
Command Execution in Bash
In Bash, commands are executed in the order they are written, one after the other. However, the ‘&&’ command allows you to control this order based on whether a command is successful or not.
Here’s an example to illustrate this:
echo 'This will run' && echo 'This will also run'
# Output:
# 'This will run'
# 'This will also run'
In this example, the second echo
command only runs because the first echo
command was successful. If the first command had failed for any reason, the second command would not have run.
Understanding these fundamentals of Bash scripting and command execution is key to mastering the ‘&&’ command and using it effectively in your scripts.
The ‘&&’ Command: The Key to Efficient Bash Scripts
The ‘&&’ command is not just a tool for executing a couple of commands in sequence. When working on larger scripts or projects, this command becomes a crucial part of writing efficient and error-proof code.
The Relevance of && in Larger Scripts
In larger scripts, there are often multiple commands that need to be executed in a specific order, with later commands relying on the success of earlier ones. The ‘&&’ command allows you to ensure that each command only runs if all previous commands have been successful, preventing errors and unexpected behavior.
Here’s an example of how the ‘&&’ command can be used in a larger script:
#!/bin/bash
cd project_directory && git pull && npm install && npm start
# Output:
# (Assuming no error, your project will be updated and started)
In this script, we navigate into the project directory, update the project from a git repository, install any necessary dependencies, and start the project. Each command only runs if all previous commands were successful, ensuring that we don’t attempt to start the project if any previous step failed.
Exploring Related Concepts
If you’re interested in further exploring the world of Bash scripting, a related concept to the ‘&&’ command is conditional execution. In Bash, you can use if
, else
, and elif
statements to execute commands based on certain conditions. This can be a powerful tool in combination with the ‘&&’ command, allowing you to create complex scripts with multiple dependencies and conditions.
Further Resources for Mastering Bash &&
For those who want to dive deeper into the ‘&&’ command and Bash scripting in general, here are some resources that provide a wealth of information:
- GNU Bash Manual: This is the official manual for Bash and provides a comprehensive overview of all its features.
Bash Scripting Guide on LinuxCommand.org: This guide covers all the basics of Bash scripting, including the use of ‘&&’.
Advanced Bash-Scripting Guide: This guide goes beyond the basics and covers advanced topics in Bash scripting.
Recap: Bash && Operator Guide
In this comprehensive guide, we’ve navigated the intricacies of the ‘&&’ command in Bash, a powerful tool that allows for the execution of multiple commands in sequence, but only if the previous command executes successfully.
We embarked on our journey with the basics, learning how to use the ‘&&’ command to execute simple sequences of commands. As we delved deeper, we explored more complex uses of ‘&&’, such as using it in scripts and combining it with other commands like ‘||’.
Tackling common challenges was a key part of our journey, as we discussed issues like command execution failure and provided solutions to handle these scenarios. We also broadened our horizons by looking at alternative approaches to executing commands in sequence, like the ‘||’ and ‘;’ commands.
Method | Executes Next Command If… | Ideal For |
---|---|---|
&& | Previous command was successful | Ensuring each command only runs if the previous command was successful |
| Previous command failed | Providing a fallback command if a command fails | ||
; | Regardless of whether the previous command was successful or not | Running multiple commands without considering their success or failure |
Whether you’re a beginner just starting out with Bash scripting, or an experienced developer looking to refine your skills, we hope this guide has shed light on the ‘&&’ command and its effective usage in your scripts.
The ‘&&’ command is a key tool in writing efficient and error-proof Bash scripts. With the knowledge you’ve gained from this guide, you’re now equipped to use this command to its fullest potential. Happy scripting!