How-To Check if a Bash Environment Variable is Set
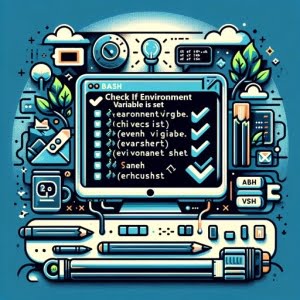
Are you finding it challenging to check if an environment variable is set in your Bash script? You’re not alone. Many developers find themselves puzzled when it comes to handling environment variables in Bash, but we’re here to help.
Think of checking environment variables in Bash as a detective looking for clues – it’s all about knowing where to look and what to look for. These variables can be crucial in controlling the behavior of your scripts, making them an essential part of Bash scripting.
In this guide, we’ll unravel the mystery of how to detect the presence of environment variables in Bash, from the basics to more advanced techniques. We’ll cover everything from using test operators to check if an environment variable is set, handling special cases, to dealing with multiple environment variables.
So, let’s dive in and start mastering how to check if an environment variable is set in Bash!
TL;DR: How Do I Check if an Environment Variable is Set in Bash?
In Bash, you can check if an environment variable is set using the
'-z'
or'-n'
test operators, with the syntax-z "${VAR}"
or-n "${VAR}"
. These operators help you determine whether a variable is unset or if it’s set to some string.
Here’s a simple example:
VAR='Hello, World!'
if [ -z "${VAR}" ]; then
echo "VAR is unset or set to the empty string"
else
echo "VAR is set to some string"
fi
# Output:
# VAR is set to some string
In this example, we’ve set the variable VAR
to ‘Hello, World!’. We then use the ‘-z’ test operator to check if VAR
is unset or set to an empty string. Since VAR
is set, the else clause executes, and we see the output ‘VAR is set to some string’.
This is a basic way to check if an environment variable is set in Bash, but there’s much more to learn about handling environment variables effectively. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Basic Use: Checking Environment Variables in Bash
- Advanced Techniques: Dealing with Special Cases in Bash
- Alternative Methods: Beyond Basic Bash Checks
- Troubleshooting Bash: Handling Common Issues
- Understanding Environment Variables in Bash
- Exploring the Relevance of Environment Variables in Bash
- Wrapping Up: Checking Bash Environment Variables
Basic Use: Checking Environment Variables in Bash
In Bash, checking if an environment variable is set is straightforward once you understand the ‘-z’ and ‘-n’ test operators. These operators are used in conditional expressions and provide a simple way to check the status of an environment variable.
The ‘-z’ and ‘-n’ Test Operators
The ‘-z’ test operator returns true if the length of the string is zero. In other words, it checks if a variable is unset or set to an empty string. On the other hand, the ‘-n’ operator returns true if the string’s length is non-zero, meaning it checks if a variable is set to some string.
Here’s an example of how to use these operators:
MY_VARIABLE=''
if [ -z "${MY_VARIABLE}" ]; then
echo "MY_VARIABLE is unset or set to the empty string"
else
echo "MY_VARIABLE is set to some string"
fi
# Output:
# MY_VARIABLE is unset or set to the empty string
In this example, we’ve set MY_VARIABLE
to an empty string. We then use the ‘-z’ test operator to check if MY_VARIABLE
is unset or set to an empty string. Since MY_VARIABLE
is set to an empty string, the ‘-z’ test returns true, and we see the output ‘MY_VARIABLE is unset or set to the empty string’.
Advantages and Potential Pitfalls
The advantage of using these operators is their simplicity and readability. However, one potential pitfall is that they only check if a variable is set or not. They don’t check the value of the variable. If you need to check the value of a variable, you’ll need to use other methods, which we’ll cover in the advanced use section.
Advanced Techniques: Dealing with Special Cases in Bash
Once you’ve mastered the basics, you can begin to explore more advanced scenarios when checking if an environment variable is set in Bash. These might include checking for non-empty strings, handling default values, and dealing with multiple environment variables.
Checking for Non-Empty Strings
The ‘-n’ operator checks if a variable is set to some string, but what if you want to ensure that the variable is set to a non-empty string? Here’s how you can do that:
MY_VARIABLE='Hello, World!'
if [ -n "${MY_VARIABLE}" ]; then
echo "MY_VARIABLE is set to a non-empty string"
else
echo "MY_VARIABLE is unset or set to the empty string"
fi
# Output:
# MY_VARIABLE is set to a non-empty string
In this example, MY_VARIABLE
is set to ‘Hello, World!’. The ‘-n’ operator checks if MY_VARIABLE
is set to a non-empty string. Since MY_VARIABLE
is set to a non-empty string, the ‘-n’ test returns true, and we see the output ‘MY_VARIABLE is set to a non-empty string’.
Handling Default Values
In some cases, you might want to assign a default value to a variable if it’s not set. Bash provides a way to do that using the :-
syntax:
DEFAULT_VALUE='Default'
VALUE=${MY_VARIABLE:-$DEFAULT_VALUE}
echo $VALUE
# Output:
# Default
In this example, if MY_VARIABLE
is unset or empty, VALUE
will be set to the DEFAULT_VALUE
, which is ‘Default’.
Dealing with Multiple Environment Variables
If you need to check multiple environment variables, you can use a loop. Here’s an example:
VARIABLES=('VAR1' 'VAR2' 'VAR3')
for VAR in "${VARIABLES[@]}"; do
if [ -z "${!VAR}" ]; then
echo "$VAR is unset or set to the empty string"
else
echo "$VAR is set to some string"
fi
done
# Output (assuming VAR1 is set, VAR2 is unset, and VAR3 is set to an empty string):
# VAR1 is set to some string
# VAR2 is unset or set to the empty string
# VAR3 is unset or set to the empty string
This script loops over the VARIABLES
array and checks each variable using the ‘-z’ operator. The !
before VAR
in ${!VAR}
is Bash’s indirect reference syntax, which allows you to access the value of a variable whose name is stored in another variable.
Alternative Methods: Beyond Basic Bash Checks
While the ‘-z’ and ‘-n’ test operators are commonly used to check if an environment variable is set in Bash, there are other techniques that offer more flexibility and control. Let’s explore a couple of these alternatives: using the ‘isset’ command and the ‘[[ -v VAR ]]’ test.
Using the ‘isset’ Command
The ‘isset’ command is a powerful tool for checking if an environment variable is set. Here’s a simple example:
MY_VARIABLE='Hello, World!'
if isset MY_VARIABLE; then
echo "MY_VARIABLE is set"
else
echo "MY_VARIABLE is unset"
fi
# Output:
# MY_VARIABLE is set
In this example, the ‘isset’ command checks if MY_VARIABLE
is set. Since MY_VARIABLE
is set, the if clause executes, and we see the output ‘MY_VARIABLE is set’.
The ‘[[ -v VAR ]]’ Test
Another alternative is to use the ‘[[ -v VAR ]]’ test, which returns true if VAR
is set. Here’s an example:
MY_VARIABLE=''
if [[ -v MY_VARIABLE ]]; then
echo "MY_VARIABLE is set"
else
echo "MY_VARIABLE is unset"
fi
# Output:
# MY_VARIABLE is set
In this example, even though MY_VARIABLE
is set to an empty string, the ‘-v’ test returns true because MY_VARIABLE
is set.
Comparing the Alternatives
Both the ‘isset’ command and the ‘[[ -v VAR ]]’ test provide more control than the ‘-z’ and ‘-n’ operators. They allow you to check if a variable is set without considering its value. However, they might be less readable to someone unfamiliar with these commands.
As always, the best method depends on your specific needs. If readability is a priority, you might prefer the ‘-z’ and ‘-n’ operators. If you need more control, the ‘isset’ command or the ‘[[ -v VAR ]]’ test might be better choices.
Troubleshooting Bash: Handling Common Issues
When working with environment variables in Bash, you might encounter some common issues. These could range from dealing with special characters in variable names to unexpected behavior due to variable scope. Let’s discuss these issues and provide solutions and workarounds.
Special Characters in Variable Names
One common issue is dealing with special characters in variable names. Bash variable names can contain letters, numbers, and underscores, but special characters can cause problems.
Consider the following example:
MY-VARIABLE='Hello, World!'
echo $MY-VARIABLE
# Output:
# -VARIABLE: command not found
In this example, the hyphen in MY-VARIABLE
is interpreted as a minus sign, leading to an error. To avoid this issue, stick to letters, numbers, and underscores when naming your variables.
Unexpected Behavior Due to Variable Scope
Another common issue is unexpected behavior due to variable scope. In Bash, variables can be local, environment, or shell variables, and their scope can affect how they behave.
Consider this example:
function test() {
LOCAL_VAR='Local'
echo $LOCAL_VAR
}
test
echo $LOCAL_VAR
# Output:
# Local
#
In this example, LOCAL_VAR
is a local variable within the test
function. It’s accessible within the function, but not outside of it. That’s why the second echo
command doesn’t print anything.
Understanding the scope of your variables can help prevent unexpected behavior in your scripts.
Understanding Environment Variables in Bash
Before diving into how to check if an environment variable is set in Bash, it’s essential to understand what environment variables are and how they work in Bash.
What are Environment Variables?
Environment variables are dynamic-named values that can affect the running processes’ behavior on a computer. They exist in every operating system, and in Bash, they provide a simple way to share configuration settings between multiple applications and processes.
For example, the PATH
environment variable supplies a list of directories where the system looks for executable files. When you type a command in the terminal, the system uses these directories defined in the PATH
variable to find the required executable file.
echo $PATH
# Output:
# /usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
In this example, echo $PATH
prints the list of directories stored in the PATH
environment variable.
Types of Variables in Bash
In Bash, there are three types of variables: local variables, environment variables, and shell variables.
- Local Variables: These are defined in the current shell only and are not available to child processes.
- Environment Variables: These are available system-wide and are inherited by all spawned child processes.
- Shell Variables: These are similar to environment variables, but they are not exported to the environment of child processes unless explicitly told to do so.
Scope of Variables in Bash
The scope of a variable in Bash refers to its visibility within the script. Variables can have global scope (accessible anywhere in the script) or local scope (accessible only within a function where they are declared).
Here’s an example showing the scope of a local variable:
function test() {
LOCAL_VAR='Local'
echo $LOCAL_VAR
}
test
# Output:
# Local
In this example, LOCAL_VAR
is a local variable within the test
function. It’s accessible within the function, but not outside of it.
Exploring the Relevance of Environment Variables in Bash
Environment variables in Bash scripting are more than just storage containers for data. They play a crucial role in parameterizing scripts, storing configuration data, and more. Understanding how to check if an environment variable is set is just the tip of the iceberg when it comes to mastering Bash scripting.
Parameterizing Scripts with Environment Variables
Environment variables are a great way to parameterize your scripts. By using them, you can write scripts that behave differently based on the environment they are run in. For instance, you might have a script that connects to a database. By storing the database credentials in environment variables, you can use the same script to connect to different databases in different environments.
DB_USER=$1
DB_PASS=$2
echo "Connecting to the database as $DB_USER..."
# Output:
# Connecting to the database as admin...
In this example, the script takes two arguments: the database username and password. It stores these arguments in the DB_USER
and DB_PASS
environment variables and uses them to connect to the database.
Storing Configuration Data in Environment Variables
Environment variables are also an excellent place to store configuration data. This can include anything from system paths to feature flags, making them a flexible tool for configuring your scripts.
FEATURE_FLAG='true'
if [ $FEATURE_FLAG = 'true' ]; then
echo "Feature is enabled"
else
echo "Feature is disabled"
fi
# Output:
# Feature is enabled
In this example, the FEATURE_FLAG
environment variable is used to control whether a feature is enabled or disabled in the script.
Further Resources for Bash Scripting Mastery
Are you eager to dive deeper into Bash scripting and environment variables? Here are some resources that can help you on your journey:
- GNU Bash Manual: The official manual for Bash, packed with in-depth information about every aspect of the shell.
- Advanced Bash-Scripting Guide: A comprehensive guide to scripting in Bash, including detailed sections on variables.
- FreeCodeCamp’s Bash Scripting Tutorial: A beginner-friendly tutorial covering Bash scripting, Linux shell scripting, and command-line basics.
Wrapping Up: Checking Bash Environment Variables
In this comprehensive guide, we’ve journeyed through the process of checking if an environment variable is set in Bash. We’ve explored the significance of environment variables in Bash scripting and how they can influence the behavior of your scripts.
We began with the basics, learning how to use the ‘-z’ and ‘-n’ test operators to check if an environment variable is set. We then delved into more advanced techniques, such as handling special cases, dealing with multiple environment variables, and using alternative methods like the ‘isset’ command and the ‘[[ -v VAR ]]’ test.
Along the way, we tackled common issues you might encounter when checking for environment variables in Bash, such as dealing with special characters in variable names and unexpected behavior due to variable scope. We provided solutions and workarounds for each issue to help you navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Control | Readability |
---|---|---|---|
‘-z’ and ‘-n’ operators | High | Moderate | High |
‘isset’ command | Moderate | High | Moderate |
‘[[ -v VAR ]]’ test | Moderate | High | Moderate |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to check if an environment variable is set in Bash.
Understanding how to work with environment variables is a fundamental part of Bash scripting. With this knowledge, you’re well equipped to write more flexible and robust scripts. Happy scripting!