How to Compare Bash Strings: Operators and Commands
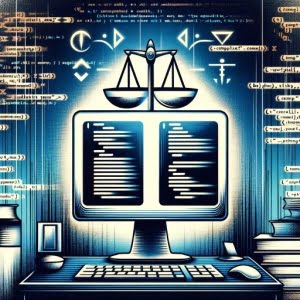
Are you finding it challenging to compare strings in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling string comparisons in Bash, but we’re here to help.
Think of Bash’s string comparison as a precise scale – allowing us to measure and compare data in a versatile and handy way, providing a powerful tool for various scripting tasks.
In this guide, we’ll walk you through the process of comparing strings in Bash, from their basic comparison, manipulation, and usage. We’ll cover everything from the basics of string comparison to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Compare Strings in Bash?
In Bash, you can compare strings using the
'=='
operator within an ‘if’ statement. For instance, you can check if two strings are equal or not.
Here’s a simple example:
string1="Hello"
string2="World"
if [ "$string1" == "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
# Output:
# Strings are not equal.
In this example, we have two strings: ‘Hello’ and ‘World’. We use the '=='
operator within an ‘if’ statement to compare these strings. Since ‘Hello’ and ‘World’ are not the same, the output is ‘Strings are not equal.’
This is a basic way to compare strings in Bash, but there’s much more to learn about string comparisons, including advanced techniques and alternative approaches. Continue reading for a more detailed exploration.
Table of Contents
- Bash String Comparison: The '==' Operator
- Advanced String Comparisons in Bash
- Alternative Methods for Bash String Comparison
- Troubleshooting Bash String Comparisons
- Understanding Bash’s String Data Type and Comparison Operators
- The Relevance of String Comparisons in Bash Scripting
- Wrapping Up: Mastering String Comparisons in Bash
Bash String Comparison: The '=='
Operator
At the heart of Bash string comparison is the '=='
operator. This operator checks if two strings are equal. It’s a fundamental tool for comparing strings in Bash and is typically used within an ‘if’ statement.
Let’s take a look at a different example:
string1="Bash"
string2="bash"
if [ "$string1" == "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
# Output:
# Strings are not equal.
In this example, we’re comparing ‘Bash’ and ‘bash’. Even though they look similar, the '=='
operator considers these strings as different due to the case sensitivity in Bash. Hence, the output is ‘Strings are not equal.’
The '=='
operator is straightforward and easy to use, making it a great starting point for beginners. However, it’s important to note that Bash is case-sensitive, which can lead to unexpected results if you’re not careful. Always ensure that the strings you’re comparing are in the same case, or use appropriate methods to handle case sensitivity.
Advanced String Comparisons in Bash
As you become more comfortable with basic string comparisons, you can start exploring more complex operations. Two such operations are comparing string lengths and using regular expressions.
Comparing String Lengths
Sometimes, you might need to compare the lengths of two strings rather than their content. Bash provides the ${#string}
syntax for this purpose. Let’s see how it works:
string1="Hello"
string2="World"
if [ ${#string1} -eq ${#string2} ]; then
echo "Strings are of equal length."
else
echo "Strings are of different lengths."
fi
# Output:
# Strings are of equal length.
In this example, we’re comparing the lengths of ‘Hello’ and ‘World’. Both strings have 5 characters, so the output is ‘Strings are of equal length.’
Using Regular Expressions
Bash also supports regular expressions, which offer powerful pattern matching capabilities. Here’s an example:
string="Hello World"
if [[ $string =~ ^Hello ]]; then
echo "String starts with 'Hello'."
else
echo "String does not start with 'Hello'."
fi
# Output:
# String starts with 'Hello'.
In this example, we’re using the =~
operator to check if ‘Hello World’ starts with ‘Hello’. Since it does, the output is ‘String starts with ‘Hello’.’
These advanced techniques allow for more complex string comparisons. However, they also require a deeper understanding of Bash and regular expressions.
Alternative Methods for Bash String Comparison
As you delve deeper into Bash scripting, you’ll discover multiple ways to compare strings. Two such methods are using the ‘test’ command and the ‘[[…]]’ syntax. Both of these methods provide a more flexible approach to string comparison.
Using the ‘test’ Command
The ‘test’ command is a built-in function in Bash that evaluates conditional expressions. One of its many uses is string comparison. Let’s see it in action:
testString="Hello"
if test "$testString" = "Hello"; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
# Output:
# Strings are equal.
In this example, we use the ‘test’ command to compare the string ‘Hello’ with another string ‘Hello’. Since both strings are equal, the output is ‘Strings are equal.’
The ‘[[…]]’ Syntax
Bash also provides the ‘[[…]]’ syntax for string comparison. This method is more modern and provides several advantages over the traditional ‘[…]’ syntax, including the ability to handle empty strings and special characters more effectively.
string1="Hello"
string2=""
if [[ -z "$string2" ]]; then
echo "String is empty."
else
echo "String is not empty."
fi
# Output:
# String is empty.
In this example, we’re using the ‘-z’ operator within the ‘[[…]]’ syntax to check if ‘string2’ is empty. Since ‘string2’ is indeed empty, the output is ‘String is empty.’
These alternative approaches offer more flexibility and robustness when comparing strings in Bash. However, they may require a more advanced understanding of Bash scripting.
Troubleshooting Bash String Comparisons
While Bash string comparisons are generally straightforward, there are a few common issues you might encounter. Let’s discuss these issues and provide some solutions.
Dealing with Special Characters
Special characters in Bash, like spaces or symbols, can sometimes cause unexpected results during string comparisons. To handle these special characters, you should enclose your strings in double quotes (“”). Let’s see an example:
string1="Hello World"
string2="Hello World"
if [ "$string1" == "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
# Output:
# Strings are equal.
In this example, we’re comparing ‘Hello World’ and ‘Hello World’. By enclosing our strings in double quotes, we ensure that the space between ‘Hello’ and ‘World’ is treated as part of the string, resulting in the output ‘Strings are equal.’
Handling Whitespace
Bash treats whitespace (spaces, tabs, and newlines) as a separator between items. If you’re comparing strings with leading or trailing whitespace, you might get unexpected results. To avoid this, you can use the ‘trim’ command to remove leading and trailing whitespace. Here’s an example:
string1=" Hello World "
string2="Hello World"
trimmedString1=$(echo "$string1" | xargs)
if [ "$trimmedString1" == "$string2" ]; then
echo "Strings are equal."
else
echo "Strings are not equal."
fi
# Output:
# Strings are equal.
In this example, ‘string1’ has leading and trailing spaces, while ‘string2’ does not. We use the ‘xargs’ command to trim ‘string1’, and then compare it with ‘string2’. The output is ‘Strings are equal.’
By understanding these common issues and their solutions, you can handle string comparisons in Bash more effectively.
Understanding Bash’s String Data Type and Comparison Operators
Before diving deeper into string comparisons, it’s crucial to grasp the fundamental concepts underlying these operations. This involves understanding Bash’s string data type and its comparison operators.
Bash’s String Data Type
In Bash, a string is a sequence of characters. Unlike some other programming languages, Bash doesn’t have separate data types for integers, floats, or booleans. Everything is a string, even if it looks like a number.
Let’s illustrate this with a quick example:
string="123"
if [[ "$string" =~ ^[0-9]+$ ]]; then
echo "String contains only numbers."
else
echo "String contains non-numeric characters."
fi
# Output:
# String contains only numbers.
In this example, we define ‘123’ as a string, even though it consists only of numbers. We then use a regular expression to check if the string contains only numbers. The output is ‘String contains only numbers.’
Bash’s Comparison Operators
Bash provides several operators for comparing strings, including '=='
, ‘!=’, ‘<‘, and ‘>’. These operators check for equality, inequality, less than, and greater than conditions, respectively.
Here’s an example of using the ‘!=’ operator to check for inequality:
string1="Hello"
string2="World"
if [ "$string1" != "$string2" ]; then
echo "Strings are not equal."
else
echo "Strings are equal."
fi
# Output:
# Strings are not equal.
In this example, we’re comparing ‘Hello’ and ‘World’. Since these strings are not equal, the output is ‘Strings are not equal.’
Understanding Bash’s string data type and comparison operators is essential for mastering string comparisons. With this knowledge, you can create more efficient and effective Bash scripts.
The Relevance of String Comparisons in Bash Scripting
String comparisons are not just a theoretical concept but a practical tool used extensively in Bash scripting and automation tasks. Whether you’re writing a script to automate a routine task, parsing output from a command, or developing a complex program, chances are you’ll need to compare strings at some point.
Exploring Related Concepts
Beyond string comparisons, there are other related concepts in Bash that are worth exploring. These include string manipulation and the use of regular expressions.
String manipulation involves operations such as substring extraction, string replacement, and string splitting. These operations can be incredibly useful when working with text data in Bash scripts.
Regular expressions, on the other hand, offer powerful pattern matching capabilities. They can be used to check if a string matches a specific pattern, making them a valuable tool for tasks like data validation and parsing.
Here’s an example of using a regular expression to validate an email address in Bash:
email="[email protected]"
if [[ "$email" =~ ^[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}$ ]]; then
echo "Email is valid."
else
echo "Email is not valid."
fi
# Output:
# Email is valid.
In this example, we’re using a regular expression to check if ‘[email protected]’ is a valid email address. The regular expression checks for a sequence of alphanumeric characters, followed by an ‘@’ symbol, another sequence of alphanumeric characters, a ‘.’, and finally, two or more alphabetic characters. Since ‘[email protected]’ meets these criteria, the output is ‘Email is valid.’
Further Resources for Bash Scripting Mastery
To learn more about string comparisons and related concepts in Bash, here are a few resources you might find helpful:
- GNU Bash Manual: This is the official manual for Bash. It provides a comprehensive overview of Bash’s features and capabilities, including string comparisons.
Bash Guide for Beginners: This guide provides a gentle introduction to Bash scripting, making it a great resource for beginners.
Advanced Bash-Scripting Guide: This guide delves into more advanced topics in Bash scripting, including string comparisons and regular expressions.
Wrapping Up: Mastering String Comparisons in Bash
Throughout this comprehensive guide, we’ve unraveled the process of comparing strings in Bash, shedding light on the different techniques, their usage, potential pitfalls, and solutions.
We embarked on this journey with the basics, learning how to compare strings using the '=='
operator within an ‘if’ statement. We then delved into more advanced techniques such as comparing string lengths and using regular expressions for more complex comparisons.
We also explored alternative approaches, such as using the ‘test’ command and the ‘[[…]]’ syntax for a more flexible and robust string comparison. Along the way, we tackled common issues that you might encounter, such as special characters and whitespace handling, and provided solutions to help you navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
'==' Operator | Simple and easy to use | Case-sensitive, may lead to unexpected results |
Comparing String Lengths | Useful for specific use cases | Not suitable for content comparison |
Regular Expressions | Powerful pattern matching capabilities | Requires understanding of regular expressions |
‘test’ Command | Versatile, can handle more complex conditions | Requires more advanced Bash knowledge |
‘[[…]]’ Syntax | More robust, handles empty strings and special characters effectively | Requires more advanced Bash knowledge |
Whether you’re a beginner just starting out with Bash or an experienced developer looking to level up your scripting skills, we hope this guide has provided you with a deeper understanding of how to compare strings in Bash.
String comparisons are a fundamental part of Bash scripting, and mastering them will undoubtedly make your scripts more efficient and effective. Happy scripting!