Multiple Condition If Statements: Or ‘||’ Operator in Bash
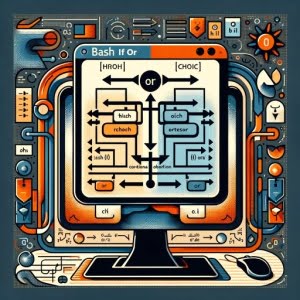
Ever found yourself tangled up in ‘if’ and ‘or’ statements in Bash? You’re not alone. Many developers find these conditional statements a bit challenging. But think of ‘if’ and ‘or’ statements in Bash like a traffic cop directing traffic – they control the flow of your script, making them essential tools in your Bash scripting toolkit.
These statements are the cornerstone of decision making in Bash scripts. They allow your scripts to make decisions and take actions based on specific conditions, making your scripts more flexible and powerful.
In this guide, we’ll walk you through the process of mastering ‘if’ and ‘or’ statements in Bash, from the basics to more advanced techniques. We’ll cover everything from writing simple ‘if’ and ‘or’ conditions, handling complex scenarios with nested ‘if’ statements, to troubleshooting common issues and even discussing alternative approaches.
So, let’s dive in and start mastering ‘if’ and ‘or’ statements in Bash!
TL;DR: How Do I Use ‘Or’ in ‘If’ Statements in Bash?
'If'
and'or'
statements in Bash are used to control script execution based on conditions.Or
is represented by the||
operator, and is used with the syntax,if [ {firstCondition} ] || [ {secondCondition} ]
. You can use them to test whether certain conditions are true and then perform different actions based on the results.
Here’s a simple example:
var=15
if [ $var -gt 10 ] || [ $var -lt 5 ]
then
echo 'Condition met'
fi
# Output:
# 'Condition met'
In this example, we have a variable var
set to 15. The ‘if’ statement checks if var
is greater than 10 or less than 5. If either of these conditions is true, it echoes ‘Condition met’. Since var
is 15, which is greater than 10, the condition is met, and ‘Condition met’ is printed.
This is a basic way to use ‘if’ and ‘or’ statements in Bash, but there’s much more to learn about controlling script flow. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Basic Use of ‘If’ and ‘Or’ Statements in Bash
- Advanced ‘If’ and ‘Or’ Usage in Bash
- Exploring Alternative Methods for Script Flow Control
- Common Issues and Solutions with ‘If’ and ‘Or’ Statements
- Unveiling the Concepts Behind ‘If’ and ‘Or’ Statements
- Expanding Your Bash Scripting Skills: Beyond ‘If’ and ‘Or’
- Wrapping Up: Mastering ‘If’ and ‘Or’ Statements in Bash
Understanding Basic Use of ‘If’ and ‘Or’ Statements in Bash
The ‘if’ and ‘or’ statements in Bash are fundamental for controlling script flow based on conditions. They are used to test whether certain conditions are true and then perform different actions based on the results.
A Simple Example of ‘If’ and ‘Or’ Statements
Let’s start with a basic example:
var=7
if [ $var -eq 7 ] || [ $var -eq 10 ]
then
echo 'Variable matches'
fi
# Output:
# 'Variable matches'
In this example, we have a variable var
set to 7. The ‘if’ statement checks if var
is equal to 7 or 10. If either of these conditions is true, it echoes ‘Variable matches’. As var
is 7, which matches one of our conditions, ‘Variable matches’ is printed.
Advantages and Potential Pitfalls
The advantage of using ‘if’ and ‘or’ statements is that they allow your scripts to make decisions and take actions based on specific conditions, making your scripts more flexible and powerful. However, there are a few potential pitfalls to be aware of.
One common mistake is not understanding that ‘or’ in Bash is represented by ‘||’. Another is not enclosing each condition in square brackets. Also, remember that spaces are crucial in Bash. Failing to include them can lead to syntax errors. For example, [ $var -eq 7]
would be incorrect – it should be [ $var -eq 7 ]
.
Understanding these basics of ‘if’ and ‘or’ statements in Bash is the first step towards writing more complex scripts.
Advanced ‘If’ and ‘Or’ Usage in Bash
As you become more comfortable with ‘if’ and ‘or’ statements in Bash, you can start to explore more complex uses. This includes nested ‘if’ statements and combining ‘or’ with ‘and’ operators.
Nested ‘If’ Statements
Nested ‘if’ statements allow you to create more complex decision structures in your script. Here’s an example of how you might use a nested ‘if’ statement:
var=15
if [ $var -gt 10 ]
then
if [ $var -lt 20 ]
then
echo 'Variable is between 10 and 20'
fi
fi
# Output:
# 'Variable is between 10 and 20'
In this example, the outer ‘if’ statement checks if var
is greater than 10. If this condition is met, it moves on to the inner ‘if’ statement, which checks if var
is less than 20. If both conditions are met, it echoes ‘Variable is between 10 and 20’. Since var
is 15, which is between 10 and 20, the conditions are met and the message is printed.
Combining ‘Or’ with ‘And’ Operators
You can also combine ‘or’ with ‘and’ operators for more complex conditions. Here’s an example:
var=25
if [ $var -gt 20 ] && ([ $var -lt 30 ] || [ $var -eq 50 ])
then
echo 'Condition met'
fi
# Output:
# 'Condition met'
In this script, the ‘if’ statement checks if var
is greater than 20 and either less than 30 or equal to 50. If these conditions are met, it echoes ‘Condition met’. As var
is 25, which meets the conditions, the message is printed.
These advanced techniques allow you to control the flow of your script more precisely, enabling you to handle a wider range of situations.
Exploring Alternative Methods for Script Flow Control
While ‘if’ and ‘or’ statements are powerful tools for controlling script flow in Bash, they are not the only options. For more complex scripts, alternatives like ‘case’ statements and ‘select’ loops can be useful. Let’s explore these methods and how they compare to ‘if’ and ‘or’ statements.
Using ‘Case’ Statements in Bash
‘Case’ statements in Bash are a more compact way to write complex ‘if’-‘elif’-‘else’ statements. Here’s an example of how a ‘case’ statement might look:
var='B'
case $var in
'A')
echo 'Variable is A'
;;
'B')
echo 'Variable is B'
;;
*)
echo 'Variable is neither A nor B'
;;
esac
# Output:
# 'Variable is B'
In this script, the ‘case’ statement checks the value of var
. If var
is ‘A’, it echoes ‘Variable is A’. If var
is ‘B’, it echoes ‘Variable is B’. For any other value, it echoes ‘Variable is neither A nor B’. Since var
is ‘B’, ‘Variable is B’ is printed.
Controlling Script Flow with ‘Select’ Loops
‘Select’ loops in Bash provide a simple way to create menus. Here’s an example:
options=('A' 'B' 'Quit')
select opt in "${options[@]}"
do
case $opt in
'A')
echo 'You chose A'
;;
'B')
echo 'You chose B'
;;
'Quit')
break
;;
*)
echo 'Invalid option'
;;
esac
done
In this script, a menu is presented with the options ‘A’, ‘B’, and ‘Quit’. The ‘select’ loop waits for the user to choose an option. The ‘case’ statement then takes action based on the chosen option.
While ‘if’ and ‘or’ statements are fundamental to Bash scripting, ‘case’ statements and ‘select’ loops offer alternative methods for controlling script flow. They can be more suitable for certain scenarios and can make your scripts more readable and maintainable.
Common Issues and Solutions with ‘If’ and ‘Or’ Statements
Working with ‘if’ and ‘or’ statements in Bash is not always smooth sailing. You might encounter syntax errors, unexpected behavior, or other issues. In this section, we’ll discuss some common problems and how to solve them.
Syntax Errors
One of the most common issues when using ‘if’ and ‘or’ statements in Bash is syntax errors. These can occur if you forget to use square brackets, don’t include spaces around the brackets, or misuse the ‘||’ operator. Here’s an example of a syntax error and how to fix it:
var=10
if [$var -eq 10] || [$var -eq 20] # Incorrect syntax
then
echo 'Variable matches'
fi
In the script above, the ‘if’ statement will throw a syntax error because there are no spaces around the square brackets. Here’s the correct syntax:
var=10
if [ $var -eq 10 ] || [ $var -eq 20 ] # Correct syntax
then
echo 'Variable matches'
fi
# Output:
# 'Variable matches'
Unexpected Behavior
Unexpected behavior can occur if the conditions in your ‘if’ and ‘or’ statements aren’t what you expect. For example, you might mistakenly use the ‘&&’ operator instead of ‘||’, leading to different results. Always double-check your conditions and test your scripts to ensure they’re working as expected.
Other Considerations
When using ‘if’ and ‘or’ statements, it’s important to consider the order of your conditions. Bash evaluates conditions from left to right and will stop as soon as it finds a condition that meets the criteria. This can affect the outcome of your script if you’re not careful.
Understanding these common issues and their solutions can help you write more effective ‘if’ and ‘or’ statements in Bash, leading to more robust and reliable scripts.
Unveiling the Concepts Behind ‘If’ and ‘Or’ Statements
To truly master ‘if’ and ‘or’ statements in Bash, we need to delve deeper into the underlying concepts. Two key concepts we need to grasp are conditional execution and Boolean logic.
Conditional Execution in Bash
Conditional execution is a fundamental concept in Bash, and indeed in any programming or scripting language. It’s the principle that certain code is executed only if specific conditions are met. The ‘if’ statement in Bash is a primary tool for implementing conditional execution. Here’s an example:
var=20
if [ $var -eq 20 ]
then
echo 'Variable is 20'
fi
# Output:
# 'Variable is 20'
In this script, the ‘if’ statement checks if the variable var
is equal to 20. If this condition is met, it echoes ‘Variable is 20’.
Understanding Boolean Logic
Boolean logic, named after mathematician George Boole, is a form of algebra where values are either true or false. In Bash, ‘or’ is a Boolean operator that you can use in ‘if’ statements. The ‘or’ operator, represented by ‘||’, checks if either of the conditions is true. If either condition is true, the ‘if’ statement returns true. Here’s an example:
var=30
if [ $var -eq 20 ] || [ $var -eq 30 ]
then
echo 'Variable is 20 or 30'
fi
# Output:
# 'Variable is 20 or 30'
In this script, the ‘if’ statement checks if var
is equal to 20 or 30. As var
is 30, one of the conditions is met, so the ‘if’ statement returns true and ‘Variable is 20 or 30’ is printed.
Understanding these fundamental concepts of conditional execution and Boolean logic is crucial to mastering ‘if’ and ‘or’ statements in Bash. With these concepts in mind, you’ll be able to write more effective and reliable Bash scripts.
Expanding Your Bash Scripting Skills: Beyond ‘If’ and ‘Or’
Mastering ‘if’ and ‘or’ statements in Bash is just the beginning. These statements are fundamental, but Bash scripting offers a lot more to explore and learn. As you continue your journey, you’ll find that these concepts lay the groundwork for more advanced topics in Bash scripting.
Integrating ‘If’ and ‘Or’ in Larger Scripts
In larger scripts or projects, ‘if’ and ‘or’ statements play a critical role in controlling the flow of execution. They allow your scripts to make decisions based on various conditions, adding flexibility and power to your code. Whether it’s a simple script to automate a routine task or a complex script for system administration, ‘if’ and ‘or’ statements are indispensable.
Exploring Related Topics
Once you’re comfortable with ‘if’ and ‘or’ statements, there are other related topics to explore. For instance, Bash loops (such as ‘for’, ‘while’, and ‘until’) offer different ways to repeat certain operations. Bash functions allow you to group commands for specific tasks into reusable blocks. Script debugging is another essential skill, helping you identify and fix errors in your scripts.
Further Resources for Mastering Bash Scripting
To deepen your understanding of Bash scripting, here are some additional resources you might find helpful:
- GNU Bash Manual: The official manual for Bash, providing comprehensive information on its features.
- Conditions in Bash Scripting IF Statements – Pluralsight: This Pluralsight blog post provides insights into conditional statements in Bash scripting.
- Bash Guide from The Linux Documentation Project: A beginner-friendly guide covering the basics of Bash scripting.
By understanding ‘if’ and ‘or’ statements and exploring beyond, you’re well on your way to becoming proficient in Bash scripting.
Wrapping Up: Mastering ‘If’ and ‘Or’ Statements in Bash
In this comprehensive guide, we’ve delved into the world of ‘if’ and ‘or’ statements in Bash, exploring their utility in controlling script flow and decision-making processes.
We began with the basics, learning how to use ‘if’ and ‘or’ statements in simple scenarios. We then moved on to more advanced uses, such as nested ‘if’ statements and combining ‘or’ with ‘and’ operators. Along the way, we tackled common issues you might encounter when using ‘if’ and ‘or’ statements, providing solutions and workarounds for each problem.
We also explored alternative methods for controlling script flow, such as ‘case’ statements and ‘select’ loops. These alternatives, while not always necessary, can offer more flexibility and readability in certain scenarios.
Here’s a quick comparison of the methods we’ve discussed:
Method | Complexity | Flexibility | Use Case |
---|---|---|---|
‘If’ and ‘Or’ Statements | Low to Moderate | High | Simple to Intermediate Script Flow Control |
Nested ‘If’ Statements | Moderate | High | Complex Decision Structures |
‘Case’ Statements | Moderate | High | Compact ‘if’-‘elif’-‘else’ Statements |
‘Select’ Loops | High | High | Creating Menus |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of ‘if’ and ‘or’ statements and their capabilities.
Mastering these tools is a crucial step towards becoming proficient in Bash scripting. With these skills, you’ll be well-equipped to write effective and efficient scripts. Happy coding!