Bash Shell Options: A Guide to Using Modifier Flags
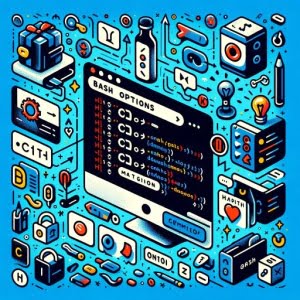
Ever felt overwhelmed by the plethora of options available in Bash? You’re not alone. Many developers find themselves in a maze when it comes to handling Bash options, but we’re here to help.
Think of Bash options as a toolbox – a toolbox that equips your command line with a wide range of capabilities, making Bash a powerful tool for various tasks.
In this guide, we’ll walk you through the process of using Bash options, from the basics to more advanced techniques. We’ll cover everything from enabling simple Bash options to understanding their impact on your Bash environment, as well as alternative approaches.
Let’s get started and master Bash options!
TL;DR: What Are Bash Options and How Do I Use Them?
Bash options are flags like
-a
,-b
,-i
, and-b
. They are used to modify the behavior of your Bash shell. For instance, the'-i'
option starts Bash in interactive mode and the'-b'
option reads commands in script, but does not execute them (syntax check).
Here’s a simple example:
bash -i
In this example, we’ve used the ‘-i’ option with the bash command to start an interactive Bash session. This is just a basic usage of Bash options, but there are many more options with different functionalities.
Dive in for a comprehensive guide on Bash options and their practical applications. We’ll cover everything from basic to advanced usage, troubleshooting, and even alternative approaches.
Table of Contents
- Understanding Bash Options: A Beginner’s Guide
- Diving Deeper: Intermediate Bash Options
- Exploring Alternatives: Bash Without Options
- Troubleshooting Common Bash Option Issues
- Bash Shell: The Foundation for Bash Options
- Enhancing Your Scripting Skills with Bash Options
- Wrapping Up: Option Flags and the Bash Shell
Understanding Bash Options: A Beginner’s Guide
Bash options, also known as shell options, are settings that change the behavior of your Bash shell. They can be set or unset to enable or disable specific features in the shell. To understand why they are important, let’s take a look at a simple but commonly used Bash option: the ‘-e’ option.
The ‘-e’ option tells the shell to exit immediately if any invoked command exits with a non-zero status, which is the convention for indicating errors in Unix-like operating systems. It’s a handy option for scripting, as it helps to catch errors and bugs early.
Here’s a simple demonstration:
#!/bin/bash
set -e
echo 'This will run'
command_that_does_not_exist
echo 'This will not'
# Output:
# This will run
# ./script.sh: line 7: command_that_does_not_exist: command not found
In this script, we first enable the ‘-e’ option with the ‘set’ command. We then try to run a command that doesn’t exist. Because of the ‘-e’ option, the script exits immediately after the failed command, and the final ‘echo’ command doesn’t get a chance to run.
This is a simple example, but it illustrates the power of Bash options. By using them effectively, you can customize your Bash environment and make your scripts more robust and efficient.
Diving Deeper: Intermediate Bash Options
As you become more comfortable with Bash options, you can start exploring more advanced ones that offer greater control and customization of your Bash environment. Let’s take a look at a couple of these options.
The ‘-v’ Option
The ‘-v’ option, short for verbose, instructs Bash to print shell input lines as they are read. This is particularly useful for debugging purposes as it provides a detailed account of what’s happening behind the scenes.
#!/bin/bash
set -v
echo 'Hello, world!'
# Output:
# echo 'Hello, world!'
# Hello, world!
In the above script, we’ve enabled verbose mode with set -v
. When we run the script, it first prints the command echo 'Hello, world!'
before executing it and printing Hello, world!
. This gives us a clear picture of what commands are being run.
The ‘-x’ Option
Another useful option for debugging is the ‘-x’ option. When enabled, Bash will print each command that is going to be executed to the terminal before it is executed.
#!/bin/bash
set -x
echo 'Hello, world!'
# Output:
# + echo 'Hello, world!'
# Hello, world!
In this script, we’ve enabled the ‘-x’ option with set -x
. This option is similar to ‘-v’, but it also prints the results of variable expansions and command substitutions. This makes it even more powerful for debugging complex scripts.
These are just a couple of the many advanced Bash options available. By understanding and utilizing these options, you can greatly enhance your scripting abilities and make your Bash environment more suited to your needs.
Exploring Alternatives: Bash Without Options
While Bash options provide a powerful way to customize your Bash environment, there are alternative ways to achieve similar results. Let’s explore a couple of these alternatives.
Error Handling Without ‘-e’
Remember the ‘-e’ option we discussed earlier? It’s a handy tool for error handling, but we can achieve similar results with careful scripting.
#!/bin/bash
echo 'This will run'
command_that_does_not_exist || exit 1
echo 'This will not'
# Output:
# This will run
# ./script.sh: line 4: command_that_does_not_exist: command not found
In this script, we use the ‘||’ operator to specify that the script should exit if the preceding command fails. It’s a more manual approach, but it gives you finer control over error handling.
Debugging Without ‘-v’ or ‘-x’
The ‘-v’ and ‘-x’ options are great for debugging, but you can also use strategic ‘echo’ statements to track the flow of your script.
#!/bin/bash
echo 'Running command...'
echo 'Hello, world!'
# Output:
# Running command...
# Hello, world!
In this script, we use an ‘echo’ statement to indicate that a command is about to be run. This can be a simple but effective way to keep track of what’s happening in your script.
While these alternative approaches can be useful, they require more manual intervention and can be more error-prone. Bash options offer a more automated and reliable way to customize your Bash environment, but understanding these alternatives can give you more flexibility and control over your scripts.
Troubleshooting Common Bash Option Issues
While Bash options can be incredibly useful, they can also lead to unexpected behaviors if not used properly. Let’s look at some common issues that may arise and how to troubleshoot them.
Unintended Script Exit with ‘-e’
The ‘-e’ option can cause your script to exit unexpectedly if a command fails that you didn’t expect to fail. Consider the following example:
#!/bin/bash
set -e
command_that_might_fail
echo 'This might not run'
# Output:
# ./script.sh: line 5: command_that_might_fail: command not found
In this script, the ‘command_that_might_fail’ command does indeed fail, causing the script to exit immediately due to the ‘-e’ option. The ‘echo’ command never gets executed. If this is not the intended behavior, you might need to handle errors more explicitly.
Excessive Output with ‘-v’ or ‘-x’
While the ‘-v’ and ‘-x’ options can be useful for debugging, they can also produce a lot of output, which can make it difficult to find the information you’re looking for. If you’re finding the output to be too verbose, you might want to consider using strategic ‘echo’ statements or logging to a file instead.
Forgetting to Unset Options
Once an option is set, it remains in effect until it is explicitly unset. This can lead to unexpected behavior if you forget to unset an option. It’s a good practice to unset options when you’re done using them.
#!/bin/bash
set -e
command_that_might_fail || true
echo 'This will run'
# Output:
# This will run
In this script, we use ‘|| true’ to effectively disable the ‘-e’ option for the ‘command_that_might_fail’ command. Even though the command fails, the script does not exit and the ‘echo’ command gets executed.
Remember, Bash options are powerful tools, but they should be used carefully and thoughtfully. With a good understanding of these options and potential issues, you can write robust and efficient Bash scripts.
Bash Shell: The Foundation for Bash Options
Before diving deeper into Bash options, it’s important to understand the environment in which these options operate: the Bash shell.
Bash, short for Bourne Again SHell, is a command processor that allows users to interact with operating systems. It’s a powerful tool that interprets and executes your commands, scripts, and even manages files and processes.
The Role of Bash Shell
The Bash shell serves as the interface between you and your operating system. When you type a command in the terminal, Bash interprets that command and communicates with the operating system to execute it. The results are then displayed back to you in the terminal.
echo "Hello, World!"
# Output:
# Hello, World!
In the above code block, the echo
command is interpreted by the Bash shell, which then instructs the operating system to print ‘Hello, World!’ in the terminal.
Bash Options in Context
Bash options come into play when you want to modify the behavior of the Bash shell. They allow you to customize how Bash interprets and executes your commands, providing you with greater control and flexibility over your Bash environment.
Understanding the Bash shell and its functionalities provides the necessary context for understanding Bash options. With this foundation, you can better appreciate the power and utility of Bash options.
Enhancing Your Scripting Skills with Bash Options
Understanding and effectively using Bash options can significantly improve your scripting skills and efficiency in the Bash environment. By customizing your Bash shell’s behavior, you can tailor your working environment to better suit your needs and preferences.
Boosting Efficiency with Bash Options
Bash options can help you write more robust scripts by providing control over error handling and debugging. For instance, the ‘-e’ option allows your script to exit immediately upon encountering an error, preventing further execution of potentially harmful commands.
#!/bin/bash
set -e
command_that_fails
echo 'This will not run'
# Output:
# ./script.sh: line 5: command_that_fails: command not found
In this script, the ‘command_that_fails’ command causes the script to exit immediately due to the ‘-e’ option, preventing the ‘echo’ command from running. This can help you catch and fix errors in your scripts more efficiently.
Expanding Your Knowledge: Further Resources for Bash Mastery
If you’re interested in diving deeper into Bash and its functionalities, here are some resources that you might find useful:
- GNU Bash Manual: This is the official manual for Bash, providing a comprehensive overview of its features and functionalities.
Bash Guide for Beginners: This guide provides a detailed introduction to Bash for beginners, covering everything from basic commands to scripting.
Advanced Bash-Scripting Guide: For those who are more comfortable with Bash, this guide dives into more advanced topics, including scripting and shell programming.
Remember, mastering Bash options is just one part of becoming proficient in Bash. There’s a whole world of command line utilities, scripting techniques, and other features to explore. So keep learning, keep experimenting, and keep scripting!
Wrapping Up: Option Flags and the Bash Shell
In this comprehensive guide, we’ve delved into the world of Bash options, a powerful tool for customizing your Bash shell and enhancing your scripting abilities.
We embarked on this journey with the basics, understanding what Bash options are and how to use them. We then ventured into more advanced territory, exploring complex Bash options and how they can be used to customize your Bash environment.
Along the way, we tackled common challenges you might face when using Bash options, such as unintended script exit and excessive output, providing you with solutions and workarounds for each issue. We also looked at alternative approaches to using Bash options, giving you a broader perspective and more flexibility in your scripting.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Bash Options | Powerful, customizable | Can lead to unexpected behaviors if not used properly |
Manual Error Handling | Fine control over error handling | More manual intervention, can be more error-prone |
Strategic Echo Statements | Simple, easy to use | Less detailed than ‘-v’ or ‘-x’ |
Whether you’re just starting out with Bash options or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of Bash options and their capabilities.
With Bash options, you can tailor your Bash environment to your needs, making your scripts more robust and efficient. Keep exploring, keep learning, and happy scripting!