[SOLVED] Bash ‘Permission Denied’ Error
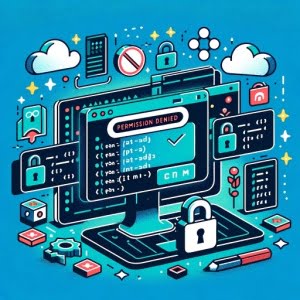
Ever found yourself stuck with a ‘permission denied’ error in bash? Like a stubborn lock refusing to budge, bash can deny access if you don’t have the right key. Many users encounter this issue, but don’t worry, we’re here to help.
Think of bash permissions as a security guard – they ensure only authorized users can perform certain actions. When they work well, they keep your system secure. But when they don’t, they can lead to frustrating ‘permission denied’ errors.
In this guide, we’ll walk you through the process of solving the ‘permission denied’ error in bash, from understanding why it occurs to how to fix it. We’ll cover everything from the basics of file permissions to more advanced techniques, as well as alternative approaches.
Let’s dive in and start unlocking the ‘permission denied’ error in bash!
TL;DR: Why Am I Getting a ‘Permission Denied’ Error in Bash?
The
'permission denied'
error in bash typically occurs when you try to execute a file or command without the necessary permissions. To fix it, you can use the'chmod'
with the syntaxchmod [option] [permissions] filename
.
Here’s a simple example:
chmod +x filename
This command gives the user execute permission for the file, allowing it to run.
Here’s a simple example:
ls -l script.sh
chmod +x script.sh
ls -l script.sh
# Output:
# -rw-r--r-- 1 user group 0 Jan 1 00:00 script.sh
# -rwxr-xr-x 1 user group 0 Jan 1 00:00 script.sh
In this example, we first use the ls -l
command to view the permissions of the file script.sh
. The output -rw-r--r--
shows that the user has read and write permissions, but not execute permissions. After running chmod +x script.sh
, the permissions change to -rwxr-xr-x
, indicating that the user now has execute permissions.
This is a basic way to resolve the ‘permission denied’ error in bash, but there’s much more to learn about file permissions and other potential solutions. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding the ‘chmod’ Command
- Digging Deeper: Understanding Different Types of Permissions
- Exploring Alternatives: ‘sudo’ and Changing Ownership
- Tackling Common Bash Permission Issues
- Decoding File Permissions in Unix-like Systems
- The Bigger Picture: File Permissions and System Security
- Wrapping Up: Unraveling the ‘Bash Permission Denied’ Error
Understanding the ‘chmod’ Command
The first step in solving the ‘permission denied’ error in bash is understanding the ‘chmod’ command. This command allows you to change the permissions of a file or directory. The term ‘chmod’ stands for ‘change mode’, and it’s used to define the way a file can be accessed.
In bash, the ‘chmod’ command follows this basic syntax:
chmod options permissions filename
Here, ‘options’ are optional modifiers that change the behavior of the command, ‘permissions’ specify the new permissions, and ‘filename’ is the name of the file or directory you want to modify.
One of the most common uses of ‘chmod’ is to give the user execute permissions on a file. This is done with the ‘+x’ permission. Here’s an example:
ls -l script.sh
chmod +x script.sh
ls -l script.sh
# Output:
# -rw-r--r-- 1 user group 0 Jan 1 00:00 script.sh
# -rwxr-xr-x 1 user group 0 Jan 1 00:00 script.sh
In this example, we first use the ls -l
command to view the permissions of the file script.sh
. The output -rw-r--r--
shows that the user has read and write permissions, but not execute permissions. After running chmod +x script.sh
, the permissions change to -rwxr-xr-x
, indicating that the user now has execute permissions.
While the ‘chmod’ command is powerful and can solve many ‘permission denied’ errors, it’s important to use it responsibly. Changing the permissions of a file can have serious security implications, especially if you’re giving execute permissions to a file from an untrusted source. Always make sure you understand the implications of the changes you’re making before you run the command.
Digging Deeper: Understanding Different Types of Permissions
In bash, there are three basic types of permissions: read (r), write (w), and execute (x). Each file and directory has a set of these permissions for the user that owns it (u), the group that owns it (g), and others (o). Understanding these permissions is crucial to resolving the ‘permission denied’ error.
Read, Write, and Execute: What Do They Mean?
Let’s break down what each permission type means:
- Read (r): The file can be opened and its content viewed. For a directory, this means you can list its contents.
- Write (w): The file can be modified. For a directory, this means you can add, remove, or rename files within it.
- Execute (x): The file can be run as a program. For a directory, this means you can access items within it.
These permissions can be represented numerically: read is 4, write is 2, and execute is 1. Therefore, if a file has permissions of ‘7’, it means it has read (4), write (2), and execute (1) permissions.
Applying Different Permissions
You can use the ‘chmod’ command to apply different permissions. For example, to give the user read, write, and execute permissions (7), and the group and others only read and execute permissions (5), you would use the following command:
chmod 755 filename
Let’s see this in action:
ls -l script.sh
chmod 755 script.sh
ls -l script.sh
# Output:
# -rw-r--r-- 1 user group 0 Jan 1 00:00 script.sh
# -rwxr-xr-x 1 user group 0 Jan 1 00:00 script.sh
In this example, the original permissions for script.sh
are -rw-r--r--
. After running chmod 755 script.sh
, the permissions change to -rwxr-xr-x
, indicating that the user now has read, write, and execute permissions, and the group and others have read and execute permissions.
Understanding and using these permissions effectively will help you avoid the ‘permission denied’ error and maintain the security of your system. Remember, the key is to give just enough permissions to accomplish the task, but not so much that you open up security vulnerabilities.
Exploring Alternatives: ‘sudo’ and Changing Ownership
While ‘chmod’ is a powerful tool for managing file permissions, it’s not the only way to address the ‘permission denied’ error in bash. Other methods such as using the ‘sudo’ command or changing the file’s owner can also be effective.
Super User Do: The ‘sudo’ Command
The ‘sudo’ command allows you to run programs with the security privileges of another user (by default, the superuser). This can be useful when you need to execute a file that you don’t have permissions for.
Here’s an example of how to use ‘sudo’ to run a script:
ls -l script.sh
sudo ./script.sh
# Output:
# -rw-r--r-- 1 root root 0 Jan 1 00:00 script.sh
# [Output of script.sh]
In this case, the ‘script.sh’ file is owned by ‘root’ and doesn’t have execute permissions for other users. By using ‘sudo’, we can still run the script despite the ‘permission denied’ error.
Caution: ‘sudo’ is a powerful command that can have significant effects on your system. Always use it with care, and only when necessary.
Changing Ownership: The ‘chown’ Command
Another way to deal with ‘permission denied’ errors is to change the owner of the file. The ‘chown’ command allows you to do this.
Here’s an example of how to use ‘chown’ to change the owner of a file to the current user:
ls -l script.sh
sudo chown $USER:$USER script.sh
ls -l script.sh
# Output:
# -rw-r--r-- 1 root root 0 Jan 1 00:00 script.sh
# -rw-r--r-- 1 user user 0 Jan 1 00:00 script.sh
In this example, the ‘script.sh’ file originally belongs to the ‘root’ user. After running sudo chown $USER:$USER script.sh
, the file is now owned by the current user, allowing you to modify its permissions as needed.
These alternative methods can be powerful tools in your arsenal for dealing with ‘permission denied’ errors. However, as with all powerful tools, they should be used responsibly to avoid unintended consequences.
Tackling Common Bash Permission Issues
While dealing with file permissions in bash, you may encounter a few common issues. Let’s discuss these problems and provide some solutions and workarounds.
‘Operation not permitted’
This error typically occurs when you’re trying to modify a file or directory that you don’t have write permissions for. One way to solve this is by using the ‘chmod’ command to give yourself write permissions.
Here’s an example:
ls -l document.txt
chmod u+w document.txt
ls -l document.txt
# Output:
# -r--r--r-- 1 user group 0 Jan 1 00:00 document.txt
# -rw-r--r-- 1 user group 0 Jan 1 00:00 document.txt
In this example, the ‘document.txt’ file initially has read-only permissions for all users. After running chmod u+w document.txt
, the user now has write permissions, as indicated by the -rw-r--r--
output.
‘No such file or directory’
This error message means that the file or directory you’re trying to access doesn’t exist, or the path to the file or directory is incorrect. Make sure the file or directory exists and that you’re in the correct directory. You can use the ‘ls’ command to list the contents of the current directory and verify the file’s existence.
Here’s an example:
ls
# Output:
# document.txt script.sh
In this example, running ls
shows that ‘document.txt’ and ‘script.sh’ are in the current directory. If you were trying to access a file that isn’t listed, you would receive a ‘No such file or directory’ error.
These are just a few of the common issues you may encounter when dealing with file permissions in bash. By understanding these problems and knowing how to solve them, you’ll be well-equipped to tackle the ‘permission denied’ error.
Decoding File Permissions in Unix-like Systems
Before we dive deeper into solving the ‘bash permission denied’ error, it’s essential to understand the concept of file permissions in Unix-like operating systems, including Linux and macOS. These permissions are a fundamental part of the system’s security, determining who can read, write, or execute a file.
Three Types of Permissions: Read, Write, and Execute
In Unix-like systems, each file and directory has three types of permissions:
- Read (r): Allows a user to read the contents of a file or list the contents of a directory.
- Write (w): Allows a user to modify a file or directory, including creating, deleting, and renaming files.
- Execute (x): Allows a user to run a file as a program or script, or access a directory and its subdirectories.
Here’s an example of how these permissions are displayed:
ls -l document.txt
# Output:
# -rw-r--r-- 1 user group 0 Jan 1 00:00 document.txt
In the output -rw-r--r--
, the first character -
indicates the type of file (a -
for regular files, d
for directories). The next three characters rw-
represent the user’s permissions (read and write in this case), the next three r--
represent the group’s permissions (read only), and the final three r--
represent others’ permissions (read only).
Understanding User, Group, and Others
In Unix-like systems, permissions are defined for three types of users:
- User (u): The owner of the file.
- Group (g): Other users who are members of the file’s group.
- Others (o): Everyone else.
Understanding these fundamentals of file permissions will help you troubleshoot and resolve the ‘bash permission denied’ error more effectively.
The Bigger Picture: File Permissions and System Security
Understanding and properly managing file permissions is more than just a way to avoid the ‘bash permission denied’ error. It plays a crucial role in system administration and security. By controlling who can read, write, and execute files, you can protect sensitive data and prevent unauthorized changes to your system.
User and Group Ownership
In Unix-like systems, each file and directory is associated with a user (the owner) and a group. By changing the owner or group of a file, you can control who has access to it. This is an important aspect of system administration, particularly in multi-user environments.
Special Permissions: suid, sgid, and Sticky Bit
In addition to the basic read, write, and execute permissions, Unix-like systems also support special permissions such as suid (Set User ID), sgid (Set Group ID), and the sticky bit. These permissions provide additional control over how files are accessed and executed, offering another layer of security.
For example, a file with the suid permission will run with the permissions of the file’s owner, rather than the user who ran it. This can be useful for programs that need to perform administrative tasks, but it can also pose a security risk if not used carefully.
Further Resources for Mastering Bash Permissions
If you want to dive deeper into the world of file permissions and bash, here are some resources that you might find useful:
- GNU Bash Manual: This is the official manual for bash, offering a comprehensive guide to its features and capabilities.
- The Linux Command Line: This book by William Shotts provides a detailed introduction to the command line and covers a wide range of topics, including file permissions.
- Linux File Permissions Tutorial: This tutorial offers a detailed explanation of file permissions, along with practical examples and exercises.
Wrapping Up: Unraveling the ‘Bash Permission Denied’ Error
In this all-encompassing guide, we’ve dissected the ‘bash permission denied’ error, a common stumbling block for many bash users. We’ve shed light on the reasons behind this error and provided you with practical solutions to overcome it.
We embarked on our journey with an introduction to the ‘chmod’ command, the primary tool in our arsenal to tackle this error. We then delved deeper into the world of file permissions, exploring the read, write, and execute permissions, and how to apply them using ‘chmod’.
We didn’t stop there. We ventured into alternative approaches to resolve this error. We discussed the ‘sudo’ command, a powerful tool that allows you to execute commands with superuser privileges, and the ‘chown’ command, which lets you change the ownership of a file.
Method | Pros | Cons |
---|---|---|
chmod | Changes file permissions, widely used | Misuse can lead to security risks |
sudo | Executes commands with superuser privileges | Potential for system damage if misused |
chown | Changes file ownership | Can lead to security issues if not used carefully |
We also tackled common issues you might encounter while dealing with file permissions, such as ‘Operation not permitted’ and ‘No such file or directory’, providing you with solutions and workarounds for each issue.
Finally, we took a step back to look at the bigger picture, discussing the fundamental concept of file permissions in Unix-like systems and its significance in system administration and security.
Whether you’re a bash beginner encountering the ‘permission denied’ error for the first time, or an experienced user looking for a refresher, we hope this guide has equipped you with the knowledge and tools to effectively handle file permissions in bash. Now, you’re well-prepared to confront the ‘bash permission denied’ error head-on. Happy coding!