Using Bash Script Arguments: Script Variables Guide
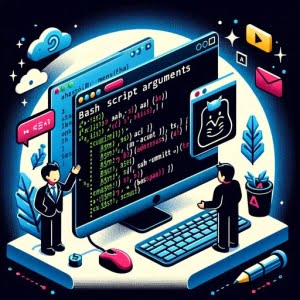
Are you finding it difficult to handle bash script arguments? You’re not alone. Many developers find themselves in a maze when it comes to managing bash script arguments. Think of bash script arguments as a courier service – they deliver information from one point to another within your scripts, making them a powerful tool for scripting and automation.
This guide will help you understand how to pass and use arguments in your bash scripts. We’ll explore bash script arguments’ core functionality, delve into their advanced features, and even discuss common issues and their solutions. So, let’s dive in and start mastering bash script arguments!
TL;DR: Using Arguments in Bash Script
Arguments can be passed to a bash script after the script name, such as
./myscript.sh World
and are accessed inside the script using special variables like$1
,$2
, etc. Here’s a simple example:
#!/bin/bash
echo "Hello, $1!"
You can run this script with an argument like this: ./myscript.sh World
and it will output: Hello, World!
.
In this example, we’ve created a simple bash script that accepts one argument. The $1
inside the script is a special variable that represents the first argument passed to the script. When we run the script with ./myscript.sh World
, the $1
is replaced with World
, and the script outputs Hello, World!
.
This is just a basic way to use arguments in a bash script, but there’s much more to learn about managing arguments efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basics of Bash Script Arguments
- Diving Deeper: Special Variables and Shift Command
- Exploring Alternative Input Methods in Bash Scripts
- Overcoming Common Bash Script Argument Hurdles
- Bash Scripting and The Power of Arguments
- Expanding Your Horizons: Bash Script Arguments in Larger Contexts
- Wrapping Up: Mastering Bash Script Arguments
Basics of Bash Script Arguments
Bash script arguments are a fundamental part of bash scripting. They allow you to pass information into your scripts, making them more flexible and reusable. Let’s break down the basics of how to pass arguments to a bash script and how to access these arguments within the script.
Passing Arguments to a Bash Script
You can pass arguments to a bash script by typing them after the script’s name when running it. Each argument should be separated by a space. Here’s an example:
./myscript.sh arg1 arg2 arg3
In this example, we’re running a script named myscript.sh
and passing three arguments to it: arg1
, arg2
, and arg3
.
Accessing Arguments Within a Script
Inside the script, you can access these arguments using special variables. $1
represents the first argument, $2
the second, and so on. Let’s look at an example:
#!/bin/bash
echo "First argument: $1"
echo "Second argument: $2"
If you run this script with ./myscript.sh arg1 arg2
, it will output:
# Output:
# First argument: arg1
# Second argument: arg2
This basic use of bash script arguments is straightforward and effective for simple scripts. However, it does have some limitations. For instance, it can become confusing and difficult to manage if you have many arguments. In our next section, we’ll explore more advanced usage scenarios that can help you handle such situations.
Diving Deeper: Special Variables and Shift Command
As you gain more experience handling bash script arguments, you’ll encounter situations that require more than just $1
, $2
, etc. Bash scripting provides special variables and commands to help you manage these scenarios.
Unveiling Special Variables
Bash scripting has a few special variables that can help manage arguments in more complex scripts:
$0
: This variable represents the name of the script itself.$#
: This variable holds the number of arguments passed to the script.$@
: This is an array-like construct of all arguments passed to the script.
Let’s see these special variables in action:
#!/bin/bash
echo "Script Name: $0"
echo "Total Arguments: $#"
echo "All Arguments: $@"
If you run this script with ./myscript.sh arg1 arg2 arg3
, it will output:
# Output:
# Script Name: ./myscript.sh
# Total Arguments: 3
# All Arguments: arg1 arg2 arg3
The Shift Command
The shift
command is another handy tool for managing arguments in bash scripts. It ‘shifts’ all arguments to the left. For instance, if you use shift
, $2
becomes $1
, $3
becomes $2
, and so on. The original first argument is discarded.
Here’s an example of how shift
works:
#!/bin/bash
echo "First argument before shift: $1"
shift
echo "First argument after shift: $1"
If you run this script with ./myscript.sh arg1 arg2
, it will output:
# Output:
# First argument before shift: arg1
# First argument after shift: arg2
The shift
command is particularly useful when you’re dealing with a large number of arguments and you want to process them one at a time.
However, while these features offer greater flexibility, they can also make your scripts more complex and harder to read if not used wisely. It’s important to balance the use of these advanced features with the readability and maintainability of your scripts.
Exploring Alternative Input Methods in Bash Scripts
While bash script arguments are a powerful tool, they’re not the only way to feed input into your scripts. Other methods, such as reading from standard input or using a configuration file, can offer more flexibility in certain scenarios.
Reading from Standard Input
Standard input (stdin) is a method that allows your script to accept input while it’s running. This can be useful when you’re dealing with sensitive information that you don’t want to include in your command line history, such as passwords.
Here’s an example of reading from stdin in a bash script:
#!/bin/bash
echo "Please enter your name:"
read name
echo "Hello, $name!"
When you run this script, it will prompt you to enter your name and then greet you:
# Output:
# Please enter your name:
# [You type your name and press enter]
# Hello, Your Name!
Using a Configuration File
Another alternative is to use a configuration file to store your script’s input. This can be particularly useful when you have a large number of inputs, or when you want to reuse the same inputs across multiple scripts.
Here’s an example of a bash script reading from a configuration file:
#!/bin/bash
source config.sh
echo "Hello, $name!"
And here’s what config.sh
might look like:
# config.sh
name="Your Name"
When you run the script, it will output:
# Output:
# Hello, Your Name!
These alternative approaches can offer more flexibility and security than bash script arguments in certain scenarios. However, they also come with their own drawbacks. Reading from stdin can make your script interactive, which might not be desirable in all situations. Using a configuration file can make your scripts more complex and harder to manage, especially if you have many configuration files. As always, it’s important to choose the right tool for the job.
Overcoming Common Bash Script Argument Hurdles
Like any coding practice, working with bash script arguments can come with its share of challenges. Let’s explore some common errors, their solutions, and some tips for best practices and optimization.
Unhandled Arguments
A common issue is failing to handle all the arguments passed to a script. This can lead to unexpected behavior if your script is relying on certain arguments to function correctly.
Consider this script:
#!/bin/bash
echo "Hello, $1!"
If you run this script without any arguments (./myscript.sh
), it will output:
# Output:
# Hello, !
To avoid this, you can check the number of arguments passed to your script using the $#
special variable and handle the situation appropriately. Here’s an improved version of the script:
#!/bin/bash
if [ $# -eq 0 ]
then
echo "No arguments supplied!"
else
echo "Hello, $1!"
fi
Now if you run the script without any arguments, it will output:
# Output:
# No arguments supplied!
Argument Overload
While bash scripts can handle a large number of arguments, there is a limit. The exact limit depends on your system, but it’s generally around 100,000 arguments. If you try to pass more arguments than your system can handle, you’ll receive an error: bash: ./myscript.sh: Argument list too long
.
If you’re dealing with a large number of arguments, you might want to consider alternative approaches, such as reading from a file or using an array.
Best Practices and Optimization
When working with bash script arguments, it’s important to write clear and readable code. Use meaningful argument names, handle missing arguments gracefully, and limit the number of arguments to a manageable amount. If you’re dealing with a large number of arguments, consider using arrays or reading from a file.
Remember, the goal is to write scripts that are not only functional but also easy to understand and maintain.
Bash Scripting and The Power of Arguments
Bash scripting is a fundamental aspect of system administration and automation. It allows you to automate repetitive tasks, manage system configurations, and even create your own tools. But what makes bash scripts truly powerful and flexible are arguments.
The Role of Arguments in Bash Scripts
Arguments in bash scripts are like inputs to a function in programming. They provide a way to make your scripts dynamic and reusable. Instead of hardcoding certain values or inputs, you can pass them as arguments when you run your script.
Consider this simple script that greets a user:
#!/bin/bash
echo "Hello, User!"
This script will always output Hello, User!
. But what if you want to greet different users? That’s where arguments come in.
#!/bin/bash
echo "Hello, $1!"
Now, you can run ./myscript.sh Alice
to greet Alice, or ./myscript.sh Bob
to greet Bob. The script has become more dynamic and reusable thanks to the use of arguments.
The Importance of Arguments in Scripting and Automation
Arguments are a crucial component in scripting and automation. They allow you to write flexible scripts that can handle different situations. For instance, you could write a script that takes a filename as an argument and performs some operation on that file. You could then run this script on any file, making it a reusable tool in your toolbox.
In summary, arguments are a powerful feature of bash scripting that allow you to write dynamic, flexible, and reusable scripts. Understanding how to use arguments effectively is a key skill in bash scripting and automation.
Expanding Your Horizons: Bash Script Arguments in Larger Contexts
Bash script arguments are not just for small, isolated scripts. They play a crucial role in larger scripts and projects as well. Understanding how to use arguments effectively can greatly enhance your scripting skills and open up a world of possibilities in automation and system administration.
Integrating Arguments in Complex Scripts
In complex scripts, bash arguments can be used to control the script’s behavior, select different options, or input data. For example, you might have a script that performs different tasks based on the arguments provided. Here’s a simple illustration:
#!/bin/bash
case $1 in
start)
echo "Starting..."
;;
stop)
echo "Stopping..."
;;
*)
echo "Usage: $0 start|stop"
;;
esac
In this script, the argument determines whether the script will output ‘Starting…’ or ‘Stopping…’. If no argument or an unrecognized argument is provided, the script outputs a usage message.
Complementary Topics to Bash Script Arguments
As you delve deeper into bash scripting, you’ll find that arguments often go hand-in-hand with other topics. For instance, you might find yourself using arguments in conjunction with control structures (like if statements and loops), commands (like grep and awk), and even other scripts. Exploring these topics can help you make the most of bash script arguments.
Further Resources for Mastering Bash Script Arguments
If you’re interested in learning more about bash script arguments and related topics, here are some resources that you might find helpful:
- The GNU Bash Reference Manual: This is the official manual for bash, and it covers everything from basic syntax to advanced features like arguments.
The Linux Command Line by William Shotts: This is a comprehensive guide to the Linux command line, including bash scripting and arguments.
Baeldung – Use Command Line Arguments in Bash Script: This Baeldung article provides a clear explanation and practical examples of how to utilize command line arguments in Bash scripts.
By mastering bash script arguments and related topics, you can write powerful, flexible scripts that can handle a wide range of tasks and scenarios.
Wrapping Up: Mastering Bash Script Arguments
In this comprehensive guide, we’ve navigated the world of bash script arguments, a crucial aspect of bash scripting that equips you with the ability to write dynamic, flexible, and reusable scripts.
We began with the basics, understanding how to pass arguments to a bash script and access them within the script. We then delved into more advanced features, such as special variables and the shift command, which provide greater flexibility in handling arguments. We also explored alternative input methods, like reading from standard input and using a configuration file, offering more options for different scenarios.
Along the way, we tackled common challenges that you might encounter when working with bash script arguments, such as unhandled arguments and argument overload, providing solutions and best practices to overcome these hurdles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Bash Script Arguments | Dynamic, flexible, reusable | Can be confusing with many arguments |
Reading from stdin | Interactive, secure | Not suitable for non-interactive scenarios |
Using a configuration file | Handles large number of inputs, reusable across scripts | Can be complex with many configuration files |
Whether you’re just starting out with bash scripting or you’re looking to enhance your scripting skills, we hope this guide has given you a deeper understanding of bash script arguments and their powerful role in scripting and automation.
With this knowledge, you’re now well-equipped to tackle any task that requires the use of bash script arguments. Happy scripting!