How To Set Environment Variables: Bash Command Guide
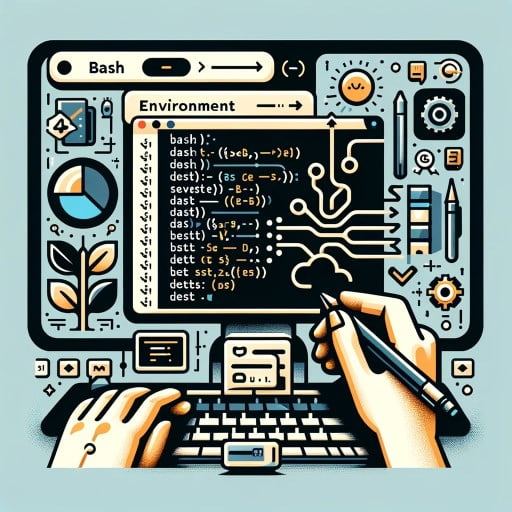
Are you finding it difficult to set environment variables in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling environment variables in Bash, but we’re here to help.
Think of environment variables as the settings of your Bash shell – allowing us to customize our shell environment, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of setting environment variables in Bash, from their creation, manipulation, and usage. We’ll cover everything from the basics of environment variables to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Set an Environment Variable in Bash?
Setting an environment variable in Bash is straightforward. You can use the
export
command followed by the variable name and its value like this:export VARNAME="value"
.
Here’s a simple example:
export USERNAME="Anton"
echo $USERNAME
# Output:
# Anton
In this example, we set the environment variable USERNAME
to the value Anton
using the export
command. Then, we use the echo
command to print the value of the USERNAME
environment variable. The echo
command is followed by $USERNAME
, which is how we access the value of the USERNAME
environment variable in Bash.
This is a basic way to set an environment variable in Bash, but there’s much more to learn about managing environment variables effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Using the export Command in Bash
- Understanding the Scope and Lifespan of Environment Variables
- Setting Environment Variables in a Script
- Making Environment Variables Available in Child Processes
- Using the env Command in Bash
- Modifying the .bashrc or .bash_profile Files
- Common Issues and Solutions With Bash Environment Variables
- Understanding Environment Variables and the Bash Shell
- The Role of Environment Variables in Bash
- Importance of Environment Variables in Linux/Unix Systems
- The Use Cases of Bash Environment Variables
- Wrapping Up: Mastering Bash Environment Variables
Using the export
Command in Bash
The export
command in Bash is used to set environment variables. The format of the command is straightforward: you start with the word export
, followed by the variable name, an equals sign, and the value you want to set for the variable. Here’s a simple example:
export GREETING="Hello, World!"
echo $GREETING
# Output:
# Hello, World!
In this example, we set the environment variable GREETING
to the value Hello, World!
. Then, we use the echo
command to print the value of the GREETING
environment variable.
It’s important to note that the environment variables you set in this way are only available in the current shell and its child processes. If you open a new shell or a new terminal window, the variable will not be available.
Understanding the Scope and Lifespan of Environment Variables
The scope of an environment variable refers to where the variable is available. When you set an environment variable using the export
command, the variable is available in the current shell and any child processes started from that shell.
The lifespan of an environment variable refers to how long the variable exists. In Bash, an environment variable exists until it is explicitly unset with the unset
command, or until the shell in which it was set exits.
Here’s an example of how to unset an environment variable:
export GREETING="Hello, World!"
echo $GREETING
unset GREETING
echo $GREETING
# Output:
# Hello, World!
#
In this example, we first set the GREETING
environment variable, then print its value. Next, we unset the GREETING
variable, and finally, we try to print its value again. As you can see from the output, after the GREETING
variable is unset, it no longer has a value.
Setting Environment Variables in a Script
Setting environment variables in a script is similar to setting them in a shell. You use the export
command in the script just like you would in a shell. However, remember that the environment variables set in a script are only available within that script and any child processes it spawns.
Here’s an example of a script that sets an environment variable:
#!/bin/bash
export GREETING="Hello, Script!"
echo $GREETING
# Output:
# Hello, Script!
In this script, we set the GREETING
environment variable to the value Hello, Script!
. We then print the value of the GREETING
environment variable.
Making Environment Variables Available in Child Processes
When you set an environment variable in a shell or a script, that variable is available in the current shell or script and any child processes. A child process is a process that is started by another process, the parent process.
Here’s an example of a child process accessing an environment variable set by its parent process:
#!/bin/bash
export PARENT_VAR="Hello, Child!"
bash -c 'echo $PARENT_VAR'
# Output:
# Hello, Child!
In this script, we set the PARENT_VAR
environment variable to the value Hello, Child!
. We then start a child process with the bash -c
command. The child process prints the value of the PARENT_VAR
environment variable.
As you can see from the output, the child process is able to access the PARENT_VAR
environment variable set by its parent process.
Using the env
Command in Bash
The env
command is another way to set environment variables in Bash. This command is often used to set environment variables for a single command execution without affecting the current shell environment.
Here’s an example of how to use the env
command:
env GREETING="Hello, env!" bash -c 'echo $GREETING'
# Output:
# Hello, env!
In this example, we use the env
command to set the GREETING
environment variable to the value Hello, env!
. We then start a child process with the bash -c
command. The child process prints the value of the GREETING
environment variable.
As you can see from the output, the GREETING
environment variable is available in the child process. However, it is not available in the parent process because we used the env
command to set it.
Modifying the .bashrc
or .bash_profile
Files
Another way to set environment variables in Bash is by modifying the .bashrc
or .bash_profile
files. These files are executed when a new shell is started, so any environment variables set in these files will be available in all new shells.
Here’s an example of how to add an environment variable to the .bashrc
file:
echo 'export WELCOME="Hello, .bashrc!"' >> ~/.bashrc
source ~/.bashrc
echo $WELCOME
# Output:
# Hello, .bashrc!
In this example, we add a line to the .bashrc
file that exports the WELCOME
environment variable with the value Hello, .bashrc!
. We then source the .bashrc
file to make the new environment variable available in the current shell. Finally, we print the value of the WELCOME
environment variable.
As you can see from the output, the WELCOME
environment variable is available in the current shell after sourcing the .bashrc
file. It will also be available in any new shells that are started.
Note: Modifying the
.bashrc
or.bash_profile
files is a more permanent way to set environment variables. However, it should be done with caution because it can affect the behavior of all new shells.
Common Issues and Solutions With Bash Environment Variables
While setting environment variables in Bash is a common task, it’s not without its challenges. Let’s discuss some common issues you might encounter and their solutions.
Bash Environment Variables Not Available in Child Processes
One common issue is that environment variables set in a parent process aren’t available in child processes. This usually happens when the environment variable is set without using the export
command.
For example:
GREETING="Hello, World!"
bash -c 'echo $GREETING'
# Output:
#
In this example, we set the GREETING
environment variable in the parent process, but when we try to access it in the child process, it’s not available. This is because we didn’t use the export
command to set the GREETING
environment variable.
The solution is to use the export
command to set the environment variable, as shown in the following example:
export GREETING="Hello, World!"
bash -c 'echo $GREETING'
# Output:
# Hello, World!
As you can see, when we use the export
command, the GREETING
environment variable is available in the child process.
Bash Environment Variables Not Persisting Across Sessions
Another common issue is that environment variables set in one terminal session are not available in another. This is because environment variables set using the export
command are only available in the current shell and its child processes.
To make an environment variable available in all new shells, you can add the export
command to the .bashrc
or .bash_profile
file, as we discussed in the Modifying the .bashrc
or .bash_profile
Files section.
These are just a few of the common issues you might encounter when setting environment variables in Bash. Remember, understanding the scope and lifespan of environment variables is key to using them effectively.
Understanding Environment Variables and the Bash Shell
An environment variable is a dynamic-named value that can affect the way running processes will behave on a computer. They are part of the environment in which a process runs.
For example, a running process can query the value of the TEMP
environment variable to discover a suitable location to store temporary files, or the HOME
variable to find the directory structure owned by the user running the process.
echo $HOME
# Output:
# /home/username
In the above example, the echo
command is used to print the value of the HOME
environment variable, which typically holds the path of the current user’s home directory.
The Role of Environment Variables in Bash
Bash, an acronym for Bourne Again Shell, is a Unix shell and command language. It’s widely used as the default shell for most Linux distributions. A shell is a program that takes your commands from the keyboard and gives them to the operating system to perform.
In the Bash shell, environment variables serve several purposes. They can be used to hold temporary data for shell scripts, influence the behavior of software, and set specific preferences. They are a fundamental part of the Bash shell environment.
Importance of Environment Variables in Linux/Unix Systems
Environment variables provide a simple way to share configuration settings between multiple applications and processes in Linux. They are used by the shell to keep track of important system information, and many applications use them to obtain information about the system, such as the operating system’s name, the number of CPUs, the current user’s home directory, and more.
For instance, the PATH
environment variable is used by the shell to determine the executable search path. With a properly set PATH
variable, you can run executables from any directory without having to specify the full path to the executable.
echo $PATH
# Output:
# /usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
In the example above, the echo
command is used to print the value of the PATH
environment variable, which holds a colon-separated list of directories in which the shell looks for executable files.
In summary, understanding environment variables and how to manipulate them in Bash is a crucial skill for managing Linux and Unix systems.
The Use Cases of Bash Environment Variables
Environment variables in Bash are not just limited to setting and retrieving data. They play a crucial role in shell scripting and system administration, acting as global variables that can be accessed by any child process of the shell.
Essential in Shell Scripting
In shell scripting, environment variables can be used to store temporary data for use in scripts, to customize the shell environment, and to pass data and set options for programs.
Here’s an example of a shell script that uses environment variables:
#!/bin/bash
export USER_NAME="John Doe"
echo "Hello, $USER_NAME. Welcome to our system!"
# Output:
# Hello, John Doe. Welcome to our system!
In this script, we set the USER_NAME
environment variable to John Doe
and then use that variable in a greeting message.
Pivotal in System Administration
In system administration, environment variables are often used to define system-wide settings, such as the system’s PATH
, the locale and language settings, and the location of temporary files.
For instance, the PATH
environment variable, which holds a list of directories that the shell should search when trying to execute a command, is a crucial environment variable in system administration.
echo $PATH
# Output:
# /usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin
Further Resources for Bash Environment Variable Mastery
Mastering the use of environment variables in Bash can greatly enhance your efficiency in shell scripting and system administration. Here are some resources to help you dive deeper:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and offers a deep dive into all aspects of Bash, including environment variables.
- Linux Command Library: This is a great resource for learning about different Bash commands, including commands for manipulating environment variables.
- The Linux Documentation Project Guides: This site offers a variety of guides on Linux topics, including Bash scripting and environment variables.
Wrapping Up: Mastering Bash Environment Variables
In this comprehensive guide, we’ve explored the ins and outs of setting environment variables in Bash. Whether you’re just getting started with Bash or you’re an experienced developer looking for a refresher, we’ve covered everything from the basics to more advanced techniques.
We started with the basics, learning how to use the export
command to set environment variables. We then delved into more advanced topics, such as setting environment variables in a script and making them available in child processes.
We also explored alternative approaches to setting environment variables in Bash, such as using the env
command or modifying the .bashrc
or .bash_profile
files. We provided practical examples and discussed the advantages and disadvantages of each method.
Along the way, we tackled common issues you might encounter when setting environment variables in Bash, such as variables not being available in child processes, and provided solutions to these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Scope | Persistence |
---|---|---|
export command | Current shell and child processes | Until shell exits |
env command | Single command execution | Until command exits |
Modifying .bashrc or .bash_profile | All new shells | Permanent |
We hope this guide has given you a deeper understanding of how to set environment variables in Bash. Understanding and effectively using environment variables is a crucial skill when working with Linux and Unix systems. Now, you’re well equipped to handle environment variables in Bash like a pro. Happy scripting!