Splitting Strings in Bash: Techniques and Examples
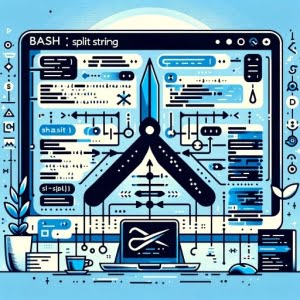
Are you finding it challenging to split strings in Bash? You’re not alone. Many developers grapple with this task, but there’s are tools that can make this process a breeze.
Bash can divide a string into smaller parts based on a delimiter. These parts can be used for a variety of tasks, making string splitting a versatile and handy tool for various tasks.
This guide will walk you through the process of splitting strings in Bash, from basic usage to advanced techniques. We’ll cover everything from the basics of string splitting to more advanced techniques, as well as alternative approaches.
Let’s get started and master string splitting in Bash!
TL;DR: How Do I Split a String in Bash?
In Bash, you can use the
Internal Field Separator (IFS)
to split a string. The IFS is a special shell variable used for splitting a string into words.
Here’s a simple example:
IFS=' ' read -ra ADDR <<< "Hello World"
for i in "${ADDR[@]}"; do echo "$i"; done
# Output:
# Hello
# World
In this example, we set the IFS variable to a space (‘ ‘), then read the string “Hello World” into an array ADDR. The -ra
option tells read to split the string into an array. We then loop over the array and print each element, resulting in the words ‘Hello’ and ‘World’ printed on separate lines.
This is just a basic way to split a string in Bash. There’s much more to learn about string manipulation in Bash, including dealing with different delimiters and multi-character delimiters. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Splitting Strings in Bash: The Basics
- Pitfalls to Avoid
- Handling Different Delimiters
- Dealing with Multi-Character Delimiters
- Best Practices
- Exploring Alternative Methods for String Splitting in Bash
- Troubleshooting Common Issues in Bash String Splitting
- Understanding Bash String Manipulation and IFS
- The Impact of String Splitting in Bash
- Wrapping Up: Mastering String Splitting in Bash
Splitting Strings in Bash: The Basics
In Bash, the Internal Field Separator (IFS) is a key player when it comes to splitting strings. The IFS is a special variable that Bash uses to split a string into words. By default, it’s set to a whitespace, but you can change it to any character you like.
Let’s take a look at a basic example of how to use the IFS to split a string in Bash:
IFS=',' read -ra NAMES <<< "John,Doe,Jane,Smith"
for i in "${NAMES[@]}"; do echo "$i"; done
# Output:
# John
# Doe
# Jane
# Smith
In this example, we set the IFS variable to a comma (‘,’). We then read the string “John,Doe,Jane,Smith” into an array called NAMES. The -ra
option tells the read
command to split the string into an array. We then loop over the array and print each element, resulting in the names ‘John’, ‘Doe’, ‘Jane’, and ‘Smith’ printed on separate lines.
This method is quite straightforward and works well for most scenarios. However, it’s important to remember that the IFS will reset to its default value after the read
command. If you need to use the same IFS value in other parts of your script, you’ll need to set it again.
Pitfalls to Avoid
While using IFS to split strings in Bash is generally reliable, there are a few potential pitfalls to be aware of. For example, if your string contains multiple consecutive delimiters, Bash will treat them as a single delimiter. This can lead to unexpected results if you’re not careful.
For instance, consider the following string: “John,,Jane”. If you try to split this string using the IFS method described above, you’ll end up with an array of two elements: ‘John’ and ‘Jane’. The empty field between the two commas is ignored.
IFS=',' read -ra NAMES <<< "John,,Jane"
for i in "${NAMES[@]}"; do echo "$i"; done
# Output:
# John
# Jane
As you can see, the empty field was not included in the output. This is something to keep in mind when splitting strings in Bash.
Handling Different Delimiters
In Bash, you can use any character as a delimiter to split a string. This flexibility allows for a wide range of possibilities. Let’s take a look at an example where we use a colon (‘:’) as the delimiter:
IFS=':' read -ra PATH <<< "$PATH"
for i in "${PATH[@]}"; do echo "$i"; done
# Output:
# /usr/local/sbin
# /usr/local/bin
# /usr/sbin
# /usr/bin
# /sbin
# /bin
In this example, we’re splitting the PATH
environment variable, which is a string that contains a list of directories separated by colons. Each directory is then printed on a separate line.
Dealing with Multi-Character Delimiters
What if you need to split a string based on a multi-character delimiter? For instance, let’s say you have a string where fields are separated by ‘||’. In Bash, you can handle this by using a loop and the read
command again:
INPUT="John||Doe||Jane||Smith"
while IFS='||' read -ra NAME; do
for i in "${NAME[@]}"; do
echo "$i"
done
done <<< "$INPUT"
# Output:
# John
# Doe
# Jane
# Smith
In this example, we first define a string INPUT
with fields separated by ‘||’. We then use a while
loop to read from this string. Inside the loop, we use the read
command with the -ra
option to split the string into an array. Each element of the array is then printed on a separate line.
Best Practices
When splitting strings in Bash, it’s important to remember a few best practices. First, always quote your variables to prevent word splitting and pathname expansion. Second, if you’re changing the IFS, it’s good practice to save its original value and restore it afterwards. This prevents any unintended side effects in other parts of your script. Finally, always test your scripts with different inputs to ensure they handle edge cases correctly.
Exploring Alternative Methods for String Splitting in Bash
While the Internal Field Separator (IFS) is a powerful tool for string splitting in Bash, there are other commands at your disposal that can provide more flexibility or better suit certain scenarios. Let’s explore a few of these alternatives, namely the awk
, cut
, and sed
commands.
Splitting Strings with awk
awk
is a versatile programming language designed for text processing. It’s particularly good at processing structured text, making it a great tool for string splitting. Here’s an example:
echo "John,Doe,Jane,Smith" | awk -F ',' '{print $1,$2,$3,$4}'
# Output:
# John Doe Jane Smith
In this example, we use the -F
option to specify a comma (‘,’) as the field separator. We then print each field on a separate line. It’s important to note that awk
treats each line as a separate record, making it great for processing multi-line input.
Using cut
to Split Strings
The cut
command is a simple and efficient way to split a string in Bash. It’s particularly useful when you’re dealing with delimited text files or logs. Here’s how you can use it:
echo "John,Doe,Jane,Smith" | cut -d ',' -f 1-4
# Output:
# John
# Doe
# Jane
# Smith
In this example, we use the -d
option to specify a comma (‘,’) as the delimiter, and the -f
option to specify the fields we want to print. Unlike awk
, cut
can only use a single character as a delimiter.
Splitting Strings with sed
sed
is a stream editor used to perform basic text transformations. It’s powerful and flexible, making it a good choice for complex string manipulations. Here’s an example of how to use sed
to split a string:
echo "John,Doe,Jane,Smith" | sed 's/,/
/g'
# Output:
# John
# Doe
# Jane
# Smith
In this example, we use the s
command to replace each comma (‘,’) with a newline (‘
‘). The g
at the end tells sed
to apply the substitution globally to the entire input.
It’s important to note that while awk
, cut
, and sed
can be more powerful than using IFS, they also come with their own complexities and potential pitfalls. As always, choose the tool that best fits your specific needs.
Troubleshooting Common Issues in Bash String Splitting
While splitting strings in Bash is a common task, it can sometimes present unexpected challenges. Let’s discuss some of these common issues and how to resolve them.
Handling Empty Fields
One common issue is the handling of empty fields. For example, if you have a string like ‘John,,Doe’, you might expect to get an array with three elements: ‘John’, an empty string, and ‘Doe’. However, as we discussed earlier, Bash treats multiple consecutive delimiters as a single delimiter, resulting in only two elements.
IFS=',' read -ra NAMES <<< "John,,Doe"
for i in "${NAMES[@]}"; do echo "$i"; done
# Output:
# John
# Doe
To handle this, you can use a loop and the read
command:
INPUT="John,,Doe"
while IFS=',' read -ra NAME; do
for i in "${NAME[@]}"; do
echo "$i"
done
done <<< "$INPUT"
# Output:
# John
#
# Doe
In this example, we use a while
loop to read from the string. Inside the loop, we use the read
command with the -ra
option to split the string into an array. Each element of the array is then printed on a separate line, including the empty field.
Dealing with Special Characters
Another common issue is dealing with special characters. For example, if your string contains a backslash (‘\’), you might run into unexpected behavior. This is because the backslash is an escape character in Bash.
IFS='\' read -ra PATHS <<< "path\to\file"
for i in "${PATHS[@]}"; do echo "$i"; done
# Output:
# pathtofile
In this example, we try to split the string 'path\to\file'
using a backslash as the delimiter. However, the backslash is interpreted as an escape character, and the string is not split as expected.
To resolve this, you can use double backslashes (‘\’) in your string:
IFS='\\' read -ra PATHS <<< "path\\to\\file"
for i in "${PATHS[@]}"; do echo "$i"; done
# Output:
# path
# to
# file
In this corrected example, we use double backslashes in both the string and the IFS. This allows the string to be split as expected.
While these are just a few examples, always remember to test your scripts with different inputs to ensure they handle edge cases correctly.
Understanding Bash String Manipulation and IFS
Bash, or the Bourne Again Shell, is a powerful command-line interpreter widely used in Unix-like operating systems. It provides a robust set of features for manipulating text, which is a common task in shell scripting. One of these features is the ability to split strings into arrays based on a delimiter, a process known as ‘string splitting’.
The Power of String Splitting in Bash
String splitting is a fundamental operation in many programming and scripting languages, and Bash is no exception. Whether you’re processing log files, parsing command-line arguments, or handling input data, being able to split a string into parts based on a delimiter can be incredibly useful.
In Bash, string splitting is often done using the Internal Field Separator (IFS). Let’s take a closer look at this concept.
Diving into the Internal Field Separator (IFS)
The IFS is a special variable in Bash that defines the character or characters used for word splitting. When Bash reads a line of input, it looks at the IFS value to determine where to split the line into words.
By default, the IFS is set to whitespace (spaces, tabs, and newlines), but you can change it to any character you like. This flexibility allows you to split strings based on whatever delimiter is relevant to your specific task.
Here’s an example of how you can change the IFS to split a string based on a comma:
IFS=',' read -ra NAMES <<< "John,Doe,Jane,Smith"
for i in "${NAMES[@]}"; do echo "$i"; done
# Output:
# John
# Doe
# Jane
# Smith
In this example, we first set the IFS to a comma (‘,’). We then use the read
command with the -ra
option to split the string “John,Doe,Jane,Smith” into an array. We then loop over the array and print each element, resulting in the names ‘John’, ‘Doe’, ‘Jane’, and ‘Smith’ printed on separate lines.
Understanding the role of the IFS in Bash string splitting is key to mastering this process. In the next sections, we’ll dig deeper into more advanced uses and potential pitfalls when splitting strings in Bash.
The Impact of String Splitting in Bash
String splitting is not just a mere operation in Bash; it’s a technique that has a profound impact on shell scripting and data processing. It’s a building block that, when combined with other Bash features, can lead to powerful scripts and command-line solutions.
Relevance in Shell Scripting
In shell scripting, string splitting is a common task. It’s often used when parsing command-line arguments, processing text files, or manipulating input data. By understanding how to effectively split strings in Bash, you can write more efficient and flexible scripts.
Significance in Data Processing
Data processing often involves manipulating and analyzing text data. Whether you’re dealing with CSV files, logs, or even raw text, being able to split strings based on a delimiter is a crucial skill. With Bash’s string splitting capabilities, you can quickly transform and prepare your data for further analysis.
Exploring Related Concepts
Once you’ve mastered string splitting in Bash, there are other related concepts that you might find interesting. Regular expressions, for example, offer a powerful way to match and manipulate strings based on patterns. Similarly, pattern matching in Bash allows you to check if a string matches a specific pattern, which can be useful in a variety of scenarios.
Further Resources for Bash Scripting Mastery
To deepen your understanding of Bash scripting and string manipulation, here are some resources that you might find useful:
- Advanced Bash-Scripting Guide: An in-depth exploration of the art of scripting with Bash. It covers everything from basics to advanced topics.
Bash Guide for Beginners: If you’re new to Bash or need a refresher on the basics, this guide is a great place to start.
Bash Academy: An online platform that offers interactive courses and exercises on Bash scripting.
By exploring these resources and practicing your skills, you’ll be well on your way to mastering string splitting and other Bash scripting techniques.
Wrapping Up: Mastering String Splitting in Bash
In this comprehensive guide, we’ve delved into the process of splitting strings in Bash, a powerful command-line interpreter. We’ve explored the role of the Internal Field Separator (IFS) and how it can be used to divide a string into smaller parts based on a delimiter, providing a robust solution for many scripting and data processing tasks.
We began with the basics, explaining how to use the IFS to split a string and highlighting some of the potential pitfalls. We then moved onto more advanced usage, discussing how to handle different delimiters and multi-character delimiters. We also explored alternative methods for splitting strings, such as using the awk
, cut
, and sed
commands.
Along the way, we tackled common issues you might encounter when splitting strings in Bash, such as handling empty fields and dealing with special characters. We provided solutions and workarounds for each issue, equipping you with the tools to overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
IFS | High | Low |
awk | High | Moderate |
cut | Moderate | Low |
sed | High | High |
Whether you’re new to Bash or an experienced user looking to deepen your understanding of string splitting, we hope this guide has been a valuable resource. The ability to split strings effectively in Bash is a powerful skill that can greatly enhance your scripting and data processing capabilities.
With this guide, you’re now well-equipped to handle a wide range of string splitting scenarios in Bash. Happy scripting!