CSS Alignment | How to Center and Vertical Align Text and Divs
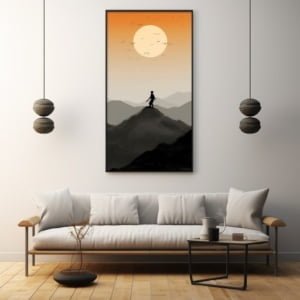
Imagine trying to hang a picture in the middle of a wall. If it’s too far to the left or right, or too high or low, it just doesn’t look right. The same principle applies when you’re designing a webpage. Whether it’s a logo, an image, or a block of text, getting that element to sit perfectly in the center is crucial for a balanced and aesthetically pleasing layout. However, centering elements in CSS can be surprisingly complex, especially for those new to web design.
In the early days of HTML, you might have relied on the <center>
tag to do the job. But that’s now deprecated and best avoided. Instead, we turn to CSS, a more powerful and flexible tool, to achieve our centering goals.
This blog post is here to demystify centering in CSS. We’ll explore multiple methods, each backed up with examples, to help you master the art of centering. Whether your challenge is to center a div, text, or both, we’ve got you covered.
TL;DR: How do I center elements in CSS?
To center a block-level element horizontally, you can set the left and right margins to ‘auto’, and define a width for the element. For vertical centering, you can use the CSS ‘transform’ property, along with ‘position: absolute’. To center a div both horizontally and vertically, you can combine these techniques.
Example of centering elements in CSS:
.center-element {
margin: auto;
position: absolute;
top: 0; left: 0; bottom: 0; right: 0;
}
Table of Contents
- Understanding Div
- How to Center a Div in CSS?
- Centering a Div Horizontally
- Centering a Div Vertically
- Centering a Div Both Horizontally and Vertically
- Responsiveness and Centering
- Using the Position, Top, Left, and Margin Properties
- Using the Position, Top, Left, and Transform Properties
- Weighing the Pros and Cons
- Understanding Flexbox
- Centering with Flexbox
- The Flexbox Approach
- Simplifying Centering with the ‘flex’ Keyword
- Weighing the Pros and Cons of Flexbox
- Centering Text Horizontally
- Centering Text Vertically
- Centering Text Both Horizontally and Vertically
- The Significance of Text Alignment
Understanding Div
Before we embark on our centering journey, let’s first familiarize ourselves with the key player in this process – the ‘div’ element. In HTML, ‘div’ is short for ‘division’ and serves as a container element. It’s often used to group other HTML elements together, allowing us to apply CSS styles to multiple elements simultaneously. This grouping plays a pivotal role in structuring our web page, much like how a bookshelf helps organize books.
But why is centering these ‘div’ elements so crucial? The answer lies in the visual impact it has on your website. Think of your website as a room you’re decorating. If the furniture (your web elements) is haphazardly placed, the room can appear chaotic and uninviting. However, properly centered and aligned elements create a sense of balance and aesthetic appeal, enhancing the user experience. A misaligned logo or off-center text can be as jarring as a crooked painting on a wall, making your website appear unprofessional and distracting to your users.
But don’t let these challenges deter you. Understanding how to center elements in CSS is a valuable skill, akin to mastering the art of interior design. It opens the door to more advanced layout techniques, enabling you to create more dynamic, responsive, and attractive web designs. So, let’s roll up our sleeves and delve into the intricacies of centering in CSS.
How to Center a Div in CSS?
Now that we understand the importance of centering, let’s get to the heart of the matter – how do we center a div in CSS? The path to perfect alignment involves various techniques, each suited to different layout requirements and constraints. Let’s explore these methods one by one.
Centering a Div Horizontally
Picture trying to place a book exactly in the middle of a bookshelf. The simplest way to achieve this with a block-level element in CSS is by setting the left and right margins to ‘auto’, and defining a width for the element. Here’s the code for it:
.center-div {
margin-left: auto;
margin-right: auto;
width: 50%;
}
This CSS rule will center the div with a class of ‘center-div’ within its parent element, much like positioning our book in the center of the shelf.
Centering a Div Vertically
Vertical centering, on the other hand, can be a bit more complex. A common method involves using the CSS ‘transform’ property, along with ‘position: absolute’. It’s like adjusting the height of the shelf to align the book perfectly. Here’s an example:
.center-div {
position: absolute;
top: 50%;
transform: translateY(-50%);
}
Centering a Div Both Horizontally and Vertically
To center a div both horizontally and vertically, you can combine the techniques above. It’s like finding the sweet spot for our book both vertically and horizontally on the shelf. Here’s how:
.center-div {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 50%;
}
However, there’s a caveat. When you use ‘left: 50%’, it moves the left edge of the div to the center of the parent element, which might not be what you want. To compensate for this, you can use ‘margin-right: -50%’ to pull the div back into the correct position.
Responsiveness and Centering
Just as a bookshelf might be viewed from different angles and distances, your website will be viewed on a variety of screen sizes. This means that your centering method needs to be responsive. The ‘transform’ property is particularly useful here, as it adjusts according to the size of the element itself, rather than its parent. This means it will keep your div centered, no matter the screen size.
In summary, centering a div in CSS can be achieved in various ways, and the method you choose will depend on your specific needs. Whether you’re centering horizontally, vertically, or both, CSS offers the tools you need to get your website elements perfectly aligned.
Having explored how to center a single div, let’s now move on to a more complex scenario – centering a div within another div. It’s a common situation in web design, much like placing a smaller book perfectly centered on a larger one on a bookshelf. There are several traditional methods you can use to achieve this.
Using the Position, Top, Left, and Margin Properties
One approach to center a child div inside a parent div involves the position, top, left, and margin properties in CSS. The parent div needs to have a relative position, while the child div should have an absolute position. It’s like setting a fixed spot on the larger book (parent div) for the smaller book (child div) to sit on. Here’s an example:
.parent {
position: relative;
}
.child {
position: absolute;
top: 50%;
left: 50%;
margin-left: -50px; /* Half the width of the child div */
margin-top: -50px; /* Half the height of the child div */
}
In this scenario, the child div will always stay in the center of the parent div, regardless of the size of the parent div.
Using the Position, Top, Left, and Transform Properties
Another method involves using the transform property in addition to position, top, and left. This method is more flexible and doesn’t require you to know the dimensions of the child div. It’s like having an adjustable spot on the larger book for the smaller book to sit on. Here’s how you can do it:
.parent {
position: relative;
}
.child {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
In this case, the transform property moves the child div back by half of its own width and height, effectively centering it within the parent div.
Weighing the Pros and Cons
Both methods have their strengths and weaknesses. The first method is simple and effective if you know the dimensions of the child div. However, it can be cumbersome if the dimensions of the child div are dynamic or unknown.
The second method is more flexible and doesn’t require you to know the dimensions of the child div. However, it requires a deeper understanding of the transform property and how it interacts with other CSS properties.
In conclusion, centering a div within a div using traditional methods is like a game of chess. It requires a good understanding of CSS properties and their interactions. By mastering these methods, you gain a deeper understanding of CSS and the ability to create more complex layouts with ease.
While traditional methods of centering a div within a div have their merits, there’s a modern, streamlined approach that you might find more efficient – Flexbox. It’s like having a state-of-the-art tool in your web design toolbox that simplifies the task of arranging your ‘books’ (web elements) on the ‘shelf’ (web page).
Understanding Flexbox
Flexbox, also known as the Flexible Box Layout, is a CSS module that provides a more efficient way to layout, align, and distribute space among items in a container, even when their size is unknown or dynamic.
Here’s a basic example of a Flexbox layout:
.flex-container {
display: flex;
justify-content: center;
align-items: center;
}
It’s a powerful and flexible tool that can make your CSS centering tasks a breeze.
Centering with Flexbox
One of the key advantages of Flexbox is its ability to center elements both vertically and horizontally with ease. It’s like having a magical tool that perfectly aligns your books on the shelf with minimal effort. Here’s how you can do it:
.parent {
display: flex;
justify-content: center; /* aligns items horizontally */
align-items: center; /* aligns items vertically */
}
With just a few lines of CSS, you can center a child div within a parent div, regardless of the size of the child div.
The Flexbox Approach
Flexbox is a modern layout model that simplifies the process of aligning and distributing space among items in a container. However, it’s worth noting that Flexbox is not supported in Internet Explorer 10 and earlier versions. So, if you need to support these older browsers, you might need to stick with the traditional methods or use a fallback.
Simplifying Centering with the ‘flex’ Keyword
In CSS level 3, the ‘flex’ keyword was introduced, which further simplifies the process of centering.
Here’s how you can use the ‘flex’ keyword to center an element:
.center-element {
display: flex;
justify-content: center;
align-items: center;
}
It allows you to control the direction, alignment, order, and size of items within a container, minimizing text overlap and maximizing responsiveness.
Weighing the Pros and Cons of Flexbox
Flexbox offers a number of advantages over traditional methods. It’s more flexible, it’s easier to use, and it can handle both horizontal and vertical centering simultaneously. However, it does have its downsides. The syntax can be a bit tricky to grasp at first, and as mentioned earlier, it’s not supported in older browsers.
In conclusion, if you’re dealing with a complex layout or need a more flexible and responsive solution, Flexbox is a fantastic tool to have in your CSS toolkit. It may not be suitable for every situation, but when it works, it’s like having a magic wand that aligns everything perfectly on your web page.
Having mastered the art of centering divs, it’s time to turn our attention towards centering text within a div. This is another frequently encountered task in CSS, one that can significantly influence your website’s readability and aesthetics, much like the placement of words on a page in a book.
Centering Text Horizontally
Just as a well-placed title on a book cover catches the eye, horizontal text centering is crucial in CSS. This is a straightforward task. All you need to do is use the ‘text-align’ property with the value of ‘center’. Here’s an example:
.center-text {
text-align: center;
}
This CSS rule will center the text within the div with a class of ‘center-text’, making your text as neatly aligned as a well-written book title.
Centering Text Vertically
Vertical text centering, on the other hand, can be a bit more complex. It’s akin to aligning the text perfectly on the spine of a book. One method is to use the ‘line-height’ property. This technique works well when you’re working with a single line of text. Here’s how you can do it:
.center-text {
line-height: 200px; /* This should be the same as the height of your div */
}
Centering Text Both Horizontally and Vertically
To center text both horizontally and vertically within a div, you can combine the techniques above. However, if you’re working with multiple lines of text, you’ll need a different approach. This is where the ‘display: table’ and ‘display: table-cell’ properties come into play. Here’s an example:
.parent {
display: table;
}
.center-text {
display: table-cell;
text-align: center;
vertical-align: middle;
}
In this case, the parent div is displayed as a table, and the child div (which contains the text) is displayed as a table cell. This allows you to use the ‘vertical-align: middle’ property to center the text vertically, just like aligning the words perfectly on the spine of a book.
The Significance of Text Alignment
Just as the alignment of text in a book impacts its readability, proper text alignment in web design is crucial for a good user experience. It not only makes your content more readable but also influences the overall aesthetics of your website. Misaligned or off-center text can be distracting and can make your website appear unprofessional, much like a poorly printed book. Conversely, well-aligned and centered text can enhance the visual appeal of your site and make your content easier to read.
In conclusion, while centering in CSS might seem as complex as arranging books on a shelf at first, understanding the different methods and knowing when to use them can make the task much more manageable. With these techniques in your CSS toolkit, you’re well on your way to creating more professional, aesthetically pleasing, and user-friendly web designs, much like a well-arranged bookshelf that appeals to every reader’s eye.