‘expect’ Linux Command: Mastering Automation in Linux
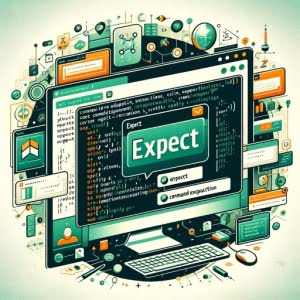
Are you finding it challenging to automate interactive applications in Linux? You’re not alone. Many developers struggle with this task, but there’s a tool that can make this process a breeze. Like a puppet master controlling his marionettes, the ‘expect’ command in Linux can control interactive applications.
This guide will walk you through the basics to advanced usage of the ‘expect’ command. We’ll explore the ‘expect’ command’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the ‘expect’ command in Linux!
TL;DR: What is the ‘expect’ command in Linux?
The
'expect'
command is a powerful tool in Linux used to automate interactive applications. The basic syntax is as follows,expect "pattern" { action }
. It can be used to script and automate tasks that require user interaction.
Here’s a simple example:
#!/usr/bin/expect
spawn ssh user@host
expect "password:"
send "mypassword
"
interact
# Output:
# This script will automatically log in to a remote host via SSH.
In this example, we’ve used the ‘expect’ command to automate the login process to a remote host via SSH. The script spawns an SSH session, waits for the password prompt, sends the password, and then allows further interaction.
This is just a basic usage of the ‘expect’ command in Linux, but there’s much more to learn about automating interactive applications. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with the ‘expect’ Command
- Advanced Usage of the ‘expect’ Command in Linux
- Exploring Alternative Methods for Automation
- Troubleshooting Common ‘expect’ Command Issues
- The Fundamentals of the ‘expect’ Command
- ‘Expect’ Command in Real-World Applications
- Wrapping Up: Linux Automation with the ‘expect’ Command
Getting Started with the ‘expect’ Command
The ‘expect’ command in Linux is a powerful tool for automating interactive applications. It’s built on the Tcl (Tool Command Language) and allows you to automate tasks that would typically require human interaction.
Syntax of ‘expect’ Command
The basic syntax of the ‘expect’ command is as follows:
expect "pattern" { action }
In this syntax, ‘expect’ waits for the ‘pattern’, and when it matches, it performs the ‘action’.
Simple ‘expect’ Script Example
Let’s look at a simple ‘expect’ script. Suppose you want to automate a task that requires user interaction, such as logging into a remote server via SSH:
#!/usr/bin/expect
spawn ssh user@your_server
expect "password:"
send "your_password
"
expect "$ "
send "ls
"
expect "$ "
interact
# Output:
# This script will log into a remote server, run the 'ls' command, and then allow further interaction.
In this script, ‘spawn’ starts a new process (ssh session in this case). The ‘expect’ command waits for the ‘password:’ prompt, and then ‘send’ provides the password. After logging in, it expects the shell prompt (‘$ ‘), sends the ‘ls’ command, and waits for the prompt again before allowing further interaction.
Advantages and Potential Pitfalls
The ‘expect’ command can be a game-changer when it comes to automating tasks in Linux. It can save you a lot of time and make your scripts more efficient. However, it’s crucial to handle sensitive information like passwords carefully. In our example, the password is written directly in the script, which can be a security risk. In real-world scenarios, consider safer methods to deal with sensitive information.
Advanced Usage of the ‘expect’ Command in Linux
As you become more familiar with the ‘expect’ command, you’ll discover that its true power lies in its advanced features. The ‘expect’ command’s flexibility allows it to handle more complex automation tasks, such as handling timeouts or multi-line responses.
Before we delve into these advanced usage scenarios, let’s familiarize ourselves with some of the command-line arguments or flags that can modify the behavior of the ‘expect’ command. Here’s a table with some commonly used ‘expect’ command arguments.
Argument | Description | Example |
---|---|---|
-b | Enables binary mode. | expect -b '{print "binary mode"}' |
-d | Enables debug mode. | expect -d script.exp |
-f | Specifies a file that contains ‘expect’ script. | expect -f script.exp |
-c | Executes the following argument as an ‘expect’ command. | expect -c 'spawn ssh user@host' |
-i | Specifies the spawn id. | expect -i $spawn_id 'expect "*"' |
-D | Sets the debug level. | expect -D 1 script.exp |
-v | Prints the version of ‘expect’. | expect -v |
-n | Specifies the number of characters for ‘expect’ to read. | expect -n 5 'expect "*"' |
-t | Sets the timeout for ‘expect’ command. | expect -t 10 'expect "*"' |
-r | Enables raw mode. | expect -r '{print "raw mode"}' |
Now that we have a basic understanding of ‘expect’ command line arguments, let’s dive deeper into the advanced use of ‘expect’.
Handling Timeouts
One of the advanced features of ‘expect’ is the ability to handle timeouts. This is particularly useful when you’re dealing with network operations that may take an unpredictable amount of time. Here’s an example:
#!/usr/bin/expect
set timeout 10
spawn ssh user@your_server
expect {
"password:" {
send "your_password
"
expect "$ "
send "ls
"
expect "$ "
interact
}
timeout {
send_user "Connection timed out
"
}
}
# Output:
# This script will log into a remote server, run the 'ls' command, and then allow further interaction. If the connection takes longer than 10 seconds, it will print a timeout message.
In this script, we’ve set a timeout of 10 seconds. If the connection takes longer than that, the script will print a timeout message.
Handling Multi-line Responses
Another advanced feature of ‘expect’ is the ability to handle multi-line responses. This is useful when you’re dealing with commands that return multiple lines of output. Here’s an example:
#!/usr/bin/expect
spawn ssh user@your_server
expect "password:"
send "your_password
"
expect "$ "
send "ls -l
"
expect -re "(.*)$ "
puts "Output: $expect_out(1,string)"
interact
# Output:
# This script will log into a remote server, run the 'ls -l' command, capture the multi-line output, and then print it. It then allows further interaction.
In this script, we’ve used the ‘-re’ flag with ‘expect’ to handle regular expressions. We’ve captured the multi-line output of the ‘ls -l’ command and printed it.
These are just a few examples of the advanced usage of the ‘expect’ command in Linux. As you can see, ‘expect’ is a powerful tool for automating interactive applications, capable of handling a wide range of scenarios.
Exploring Alternative Methods for Automation
While the ‘expect’ command offers a powerful way to automate interactive applications in Linux, it’s not the only tool available. Let’s explore some alternative methods that can help you automate tasks, such as ‘autoexpect’ and Python’s ‘pexpect’ library.
Automating Tasks with ‘autoexpect’
‘autoexpect’ is a tool that generates ‘expect’ script from a session’s typescript. It watches your interactions with an application and creates a script that can replay those interactions. Here’s how you can use ‘autoexpect’:
autoexpect -f my_script.exp ssh user@your_server
# Follow the prompts and interact with your application.
# Output:
# 'autoexpect' will create a script named 'my_script.exp' that replays your interactions.
In this example, ‘autoexpect’ generates an ‘expect’ script that automates your SSH login process. The script can be edited and reused as needed.
Using Python’s ‘pexpect’ Library
If you’re a Python enthusiast, you’ll be glad to know that there’s a Python library for automating interactive applications: ‘pexpect’. This library allows your Python script to spawn child applications, control them, and respond to expected patterns in their output. Here’s a simple example:
import pexpect
child = pexpect.spawn('ssh user@your_server')
child.expect('password:')
child.sendline('your_password')
child.expect('$')
child.sendline('ls')
child.expect('$')
print(child.before)
child.interact()
# Output:
# This Python script will log into a remote server, run the 'ls' command, print the output, and then allow further interaction.
In this script, we’ve used ‘pexpect’ to automate the SSH login process, run a command, and print its output.
Comparing ‘expect’, ‘autoexpect’, and ‘pexpect’
While all three methods can automate interactive applications, they each have their strengths. ‘expect’ is powerful and flexible, but it may require some time to learn. ‘autoexpect’ can generate ‘expect’ scripts automatically, making it a great time-saver. ‘pexpect’ brings the power of Python to automation, making it a good choice for Python developers.
Choosing the right method depends on your needs and familiarity with these tools. Regardless of the method you choose, automating interactive applications can significantly improve your productivity and efficiency.
Troubleshooting Common ‘expect’ Command Issues
Even with the best tools, you might encounter some roadblocks. The ‘expect’ command is no exception. However, understanding common issues and their solutions can make your journey smoother. Let’s discuss some of these problems and how to overcome them.
Dealing with Unmet Dependencies
When you try to use the ‘expect’ command, you might encounter an error message indicating unmet dependencies. This usually means that the ‘expect’ package isn’t installed on your system. Here’s how you can install it:
sudo apt-get update
sudo apt-get install expect
# Output:
# The system will update the package lists and install the 'expect' package.
In this example, we’ve updated the package lists and installed the ‘expect’ package using the apt package manager. If you’re using a different package manager, replace ‘apt-get’ with the appropriate command.
Handling Incorrect Script Syntax
If your ‘expect’ script isn’t working as expected, you might have a syntax error. Here’s an example of a common syntax mistake and how to correct it:
# Incorrect syntax
#!/usr/bin/expect
spawn ssh user@your_server
expect "password"
send "your_password
"
expect "$ "
send "ls
"
expect "$ "
interact
# Correct syntax
#!/usr/bin/expect
spawn ssh user@your_server
expect "password:"
send "your_password
"
expect "$ "
send "ls
"
expect "$ "
interact
# Output:
# The first script will fail because the 'expect' command is missing the colon (:) after 'password'. The second script corrects this mistake.
In this example, the first script fails because the ‘expect’ command is missing the colon (:) after ‘password’. The second script corrects this mistake.
Other Considerations
Remember to handle sensitive information like passwords carefully. Avoid hardcoding passwords into your scripts. Instead, consider safer methods such as reading from a secure file or using environment variables.
Also, bear in mind that while the ‘expect’ command is powerful, it’s not suitable for every task. For complex or large-scale tasks, consider using a more robust automation tool or scripting language.
The Fundamentals of the ‘expect’ Command
To truly master the ‘expect’ command in Linux, it’s crucial to understand its underlying mechanics and concepts. The ‘expect’ command is built on the Tcl (Tool Command Language) and uses a few core concepts to automate interactive applications: ‘spawn’, ‘expect’, and ‘send’.
The Tcl Scripting Language
Tcl, or Tool Command Language, is a dynamic programming language. It’s known for its simplicity and power, making it an excellent choice for scripting tasks. The ‘expect’ command uses Tcl as its scripting language, allowing you to use Tcl’s features and syntax in your ‘expect’ scripts.
Here’s a simple Tcl script:
set x 10
set y 20
set sum [expr $x + $y]
puts "The sum is $sum"
# Output:
# The sum is 30
In this script, we’ve declared two variables, x and y, and calculated their sum using the ‘expr’ command. We then printed the sum using the ‘puts’ command.
The ‘spawn’ Command
The ‘spawn’ command is used to start a new process that ‘expect’ can interact with. This could be an SSH session, a telnet session, or any other interactive application.
Here’s an example of the ‘spawn’ command:
#!/usr/bin/expect
spawn ssh user@your_server
# Output:
# This script will start a new SSH session.
In this script, the ‘spawn’ command starts a new SSH session.
The ‘expect’ Command
The ‘expect’ command waits for a specific pattern from the spawned process. When the pattern matches, ‘expect’ performs an action.
Here’s an example of the ‘expect’ command:
#!/usr/bin/expect
spawn ssh user@your_server
expect "password:"
# Output:
# This script will start a new SSH session and wait for the password prompt.
In this script, the ‘expect’ command waits for the password prompt from the SSH session.
The ‘send’ Command
The ‘send’ command sends input to the spawned process. This could be a password, a command, or any other input.
Here’s an example of the ‘send’ command:
#!/usr/bin/expect
spawn ssh user@your_server
expect "password:"
send "your_password
"
# Output:
# This script will start a new SSH session, wait for the password prompt, and then send the password.
In this script, the ‘send’ command sends the password to the SSH session after the ‘expect’ command detects the password prompt.
By understanding these fundamental concepts and how they work together, you can start to see the power and flexibility of the ‘expect’ command in Linux. With a bit of practice, you’ll be able to automate a wide range of interactive applications.
‘Expect’ Command in Real-World Applications
The ‘expect’ command isn’t just a theoretical tool; it has practical applications in real-world scenarios. From automated testing to remote server management, ‘expect’ can be a valuable asset in a developer’s toolkit.
Automated Testing with ‘expect’
One of the most common uses of the ‘expect’ command is in automated testing. By scripting interactive tasks, developers can automate the testing of their applications. This can save a significant amount of time and ensure consistent testing procedures.
Here’s a basic example of how you might use ‘expect’ in automated testing:
#!/usr/bin/expect
spawn ./your_test_script
expect {
"Test Passed" {
send_user "Test Passed
"
}
"Test Failed" {
send_user "Test Failed
"
exit 1
}
}
# Output:
# This script will run a test script and check the output. If the test passes, it prints 'Test Passed'. If the test fails, it prints 'Test Failed' and exits with a non-zero status.
In this script, we’re running a test script and checking its output. If the test passes, we print ‘Test Passed’. If the test fails, we print ‘Test Failed’ and exit with a non-zero status, indicating an error.
Remote Server Management with ‘expect’
Another practical use of the ‘expect’ command is in remote server management. By automating SSH login and command execution, ‘expect’ can streamline the management of remote servers.
Here’s an example of how you might use ‘expect’ to automate a routine task on a remote server:
#!/usr/bin/expect
spawn ssh user@your_server
expect "password:"
send "your_password
"
expect "$ "
send "sudo apt-get update
"
expect "[sudo] password for user:"
send "your_password
"
expect "$ "
interact
# Output:
# This script will log into a remote server, run 'sudo apt-get update' to update the package lists, and then allow further interaction.
In this script, we’re logging into a remote server and running ‘sudo apt-get update’ to update the package lists. We’re then allowing further interaction, so you can continue managing the server.
Further Resources for Mastering the ‘expect’ Command
If you want to delve deeper into the ‘expect’ command and its applications, here are some resources to explore:
- Exploring Expect: A Tcl-based Toolkit for Automating Interactive Applications – A book by Don Libes, the creator of ‘expect’.
- The Tcl Programming Language – A comprehensive guide to Tcl, the scripting language used by ‘expect’.
- Advanced Bash-Scripting Guide – A section on ‘expect’ in the Advanced Bash-Scripting Guide on The Linux Documentation Project.
These resources provide a wealth of information on ‘expect’, Tcl, and scripting in Linux. Whether you’re a beginner looking to learn the basics or an experienced developer seeking advanced techniques, these resources can help you on your journey to mastering the ‘expect’ command in Linux.
Wrapping Up: Linux Automation with the ‘expect’ Command
In this comprehensive guide, we’ve explored the ‘expect’ command in Linux, a powerful tool for automating interactive applications. We’ve seen how to use ‘expect’ from the basics to more advanced usage, and we’ve discussed common issues and their solutions.
We began with the basics, learning how to use ‘expect’ to automate simple tasks like logging into a remote server via SSH. We then delved into more advanced usage, such as handling timeouts and multi-line responses. Along the way, we tackled common challenges you might face when using ‘expect’, such as unmet dependencies and incorrect script syntax, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to automation, comparing ‘expect’ with other methods such as ‘autoexpect’ and Python’s ‘pexpect’ library. Here’s a quick comparison of these methods:
Method | Flexibility | Learning Curve | Language |
---|---|---|---|
‘expect’ | High | Moderate | Tcl |
‘autoexpect’ | Moderate | Low | Tcl |
‘pexpect’ | High | Moderate | Python |
Whether you’re just starting out with ‘expect’ or you’re looking to level up your automation skills, we hope this guide has given you a deeper understanding of ‘expect’ and its capabilities.
With its balance of flexibility, power, and control, the ‘expect’ command in Linux is a valuable tool in any developer’s toolkit. Keep practicing, keep exploring, and you’ll be an ‘expect’ master in no time. Happy scripting!