JavaScript .map() Function: Transforming Data in Arrays
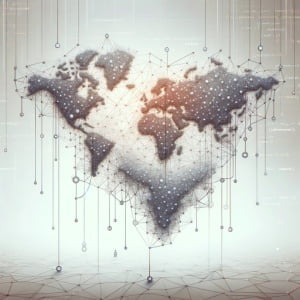
Ever found yourself struggling to understand the map function in JavaScript? You’re not alone. Many developers find themselves puzzled when it comes to handling this powerful tool. Think of the map function in JavaScript as a factory assembly line, transforming each element in an array according to your specifications.
The map function is a powerful way to transform arrays in JavaScript, making it a popular choice for data manipulation in various applications.
In this guide, we’ll walk you through the basics and advanced uses of the map function in JavaScript. We’ll cover everything from the basic syntax and usage, to more complex applications and even alternative approaches. We’ll also discuss common issues and their solutions.
So, let’s dive in and start mastering the JavaScript map function!
TL;DR: How Do I Use the Map Function in JavaScript?
The
map
function in JavaScript is used to create a new array by applying a function to each element of an existing array, with the syntaxconst squares = existingArray.map([mapping function]);
. It’s a powerful tool for transforming data in JavaScript applications.
Here’s a simple example:
const numbers = [1, 2, 3];
const squares = numbers.map(x => x * x);
console.log(squares);
// Output:
// [1, 4, 9]
In this example, we have an array of numbers. We use the map function to create a new array (squares
), where each element is the square of the corresponding element in the original array. The map function takes a callback function as an argument, which is applied to each element of the original array. In this case, the callback function is x => x * x
, which squares each number.
This is just a basic way to use the map function in JavaScript, but there’s much more to learn about this powerful tool. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Map Function in JavaScript
- Advanced Uses of JavaScript Map Function
- Exploring Alternatives to JavaScript Map
- Common Pitfalls and Solutions in Using JavaScript Map
- Understanding Arrays and Callback Functions in JavaScript
- How Does Map Fit into Functional Programming?
- Leveraging JavaScript Map in Larger Applications
- Wrapping Up: Mastering the Map Function in JavaScript
Understanding the Map Function in JavaScript
The map function is a method built into the Array object in JavaScript. It creates a new array by applying a function to each element of an existing array. It’s a powerful tool for data transformation in JavaScript applications.
Here’s the basic syntax of the map function:
const newArray = oldArray.map(function callback(currentValue, index, array)) {
// return element for newArray
};
In this syntax:
oldArray
is the original array.callback
is a function that is applied to each element of the oldArray. This function can take three arguments:currentValue
is the current element being processed in the array.index
(optional) is the index of the current element being processed in the array.array
(optional) is the array map was called upon.
newArray
is the new array resulting from the map function.
Let’s look at a simple example to demonstrate the basic usage of the map function in JavaScript:
const numbers = [1, 2, 3];
const doubled = numbers.map(num => num * 2);
console.log(doubled);
// Output:
// [2, 4, 6]
In this example, we have an array of numbers. We use the map function to create a new array (doubled
), where each element is twice the corresponding element in the original array. The map function takes a callback function (num => num * 2
) as an argument, which is applied to each element of the original array. The result is a new array where each element is the double of the original element.
This is the basic usage of the map function in JavaScript. It’s a powerful tool for transforming arrays, but it’s also flexible and can be used in more complex scenarios, as we will see in the next sections.
Advanced Uses of JavaScript Map Function
The map function in JavaScript is not limited to simple transformations. It can also handle more complex scenarios, such as mapping over nested arrays or using map with other array methods like filter and reduce. Let’s take a look at these advanced uses.
Mapping Over Nested Arrays
Nested arrays, also known as multi-dimensional arrays, are arrays that contain other arrays. When handling nested arrays, you can use the map function to transform each sub-array. Here’s an example:
const nestedNumbers = [[1, 2], [3, 4], [5, 6]];
const flattened = nestedNumbers.map(subArray => subArray.reduce((a, b) => a + b));
console.log(flattened);
// Output:
// [3, 7, 11]
In this example, the map function is applied to nestedNumbers
, which is an array containing three sub-arrays. The callback function passed to map uses the reduce method to add up the numbers in each sub-array. The result is a new array (flattened
) where each element is the sum of the numbers in the corresponding sub-array.
Combining Map with Other Array Methods
The map function can be used in combination with other array methods, such as filter and reduce, to perform more complex transformations. For example, you might want to filter an array to remove certain elements, and then use map to transform the remaining elements. Here’s an example:
const numbers = [1, 2, 3, 4, 5, 6];
const oddSquares = numbers.filter(num => num % 2 !== 0).map(num => num * num);
console.log(oddSquares);
// Output:
// [1, 9, 25]
In this example, the filter method is first used to remove even numbers from the numbers
array. The resulting array is then passed to the map function, which squares each number. The result is a new array (oddSquares
) that contains the squares of the odd numbers from the original array.
These are just a few examples of the advanced uses of the map function in JavaScript. By understanding these concepts, you can leverage the power of map to handle a wide range of data transformation tasks in your JavaScript applications.
Exploring Alternatives to JavaScript Map
While the map function is a powerful tool for transforming arrays in JavaScript, there are other methods you can use to achieve similar results. In this section, we’ll discuss three alternatives: using a for loop, the forEach method, and the reduce method. We’ll provide code examples for each and discuss their pros and cons.
Using a For Loop
The for loop is a basic control flow statement that can be used to iterate over an array and transform its elements. Here’s an example:
const numbers = [1, 2, 3];
let doubled = [];
for (let i = 0; i < numbers.length; i++) {
doubled[i] = numbers[i] * 2;
}
console.log(doubled);
// Output:
// [2, 4, 6]
In this example, we use a for loop to iterate over the numbers
array and double each number. The result is stored in the doubled
array. While this approach gives you more control over the iteration process, it requires more code and is less declarative than using map.
Using the forEach Method
The forEach method is another way to iterate over an array and perform a function on each element. Here’s an example:
const numbers = [1, 2, 3];
let doubled = [];
numbers.forEach((num, index) => {
doubled[index] = num * 2;
});
console.log(doubled);
// Output:
// [2, 4, 6]
In this example, we use the forEach method to iterate over the numbers
array and double each number. The result is stored in the doubled
array. The forEach method is more declarative than a for loop, but unlike map, it doesn’t return a new array, so you need to push the results into a new array manually.
Using the Reduce Method
The reduce method can also be used to transform an array by applying a function to each element and accumulating the results. Here’s an example:
const numbers = [1, 2, 3];
const doubled = numbers.reduce((acc, num) => {
acc.push(num * 2);
return acc;
}, []);
console.log(doubled);
// Output:
// [2, 4, 6]
In this example, we use the reduce method to iterate over the numbers
array and double each number. The result is stored in the doubled
array. The reduce method is powerful and flexible, but it can be more difficult to understand and use correctly.
These are just a few alternatives to the map function in JavaScript. Each has its pros and cons, and the best choice depends on your specific needs and the complexity of your data transformation task.
Common Pitfalls and Solutions in Using JavaScript Map
While the map function is an incredibly useful tool, it’s not without its subtleties that can lead to unexpected results. Here we’ll discuss a couple of common issues you might encounter when using the map function in JavaScript, and how to solve them.
Forgetting to Return a Value
One common mistake when using the map function is forgetting to return a value from the callback function. When this happens, the map function will create a new array filled with undefined
values. Here’s an example:
const numbers = [1, 2, 3];
const result = numbers.map(num => {
num * 2;
});
console.log(result);
// Output:
// [undefined, undefined, undefined]
In this example, the callback function multiplies each number by 2, but it doesn’t return the result. Therefore, the map function has nothing to put in the new array, and it fills it with undefined
values. To fix this issue, make sure to return a value from the callback function:
const numbers = [1, 2, 3];
const result = numbers.map(num => {
return num * 2;
});
console.log(result);
// Output:
// [2, 4, 6]
Using Map on Non-Iterable Types
Another common mistake is trying to use the map function on data types that are not iterable, such as numbers or undefined. This will result in a TypeError. Here’s an example:
const num = 42;
const result = num.map(num => num * 2);
// Output:
// TypeError: num.map is not a function
In this example, we’re trying to use the map function on a number, which is not iterable. The map function is a method of the Array object, so it can only be used on arrays. To fix this issue, make sure to use map on an array:
const numbers = [42];
const result = numbers.map(num => num * 2);
console.log(result);
// Output:
// [84]
These are just a couple of common issues you might encounter when using the map function in JavaScript. By understanding these pitfalls and how to avoid them, you can use the map function more effectively in your code.
Understanding Arrays and Callback Functions in JavaScript
Before diving deeper into the map function, let’s take a step back and understand two fundamental concepts in JavaScript: arrays and callback functions. These concepts are the building blocks for understanding how map works.
What are Arrays?
In JavaScript, an array is a single variable used to store different elements. It’s an ordered list of values that you refer to with a name and an index. For example, here’s how you can create an array in JavaScript:
const fruits = ['apple', 'banana', 'cherry'];
console.log(fruits[0]);
// Output:
// 'apple'
In this example, fruits
is an array that contains three elements. fruits[0]
refers to the first element of the array, which is ‘apple’.
What are Callback Functions?
A callback function is a function passed into another function as an argument. This allows the function to call the callback function at a suitable time. The beauty of callback functions is that they allow you to control the flow of your program.
Here’s an example of a callback function:
function greet(name, callback) {
console.log('Hello ' + name);
callback();
}
greet('Alice', function() {
console.log('Callback function called!');
});
// Output:
// 'Hello Alice'
// 'Callback function called!'
In this example, the greet
function takes two arguments: a name and a callback function. It first prints a greeting message, and then it calls the callback function.
How Does Map Fit into Functional Programming?
Functional programming is a programming paradigm where programs are constructed by applying and composing functions. It is a declarative type of programming. The key to understanding functional programming is understanding functions as first-class entities that can be used in the same way as any other entity.
The map function is a perfect example of functional programming in JavaScript. It takes a function as an argument and applies it to each element of an array. The result is a new array that contains the results of applying the function. This makes map a pure function: it does not modify the original array, and for the same input, it will always produce the same output.
Understanding these fundamentals of JavaScript can help you better understand and make use of the map function in your code.
Leveraging JavaScript Map in Larger Applications
The map function in JavaScript is not just for simple data transformations. Its power and flexibility make it a key tool in larger JavaScript applications, especially in front-end frameworks like React and Vue. Here, we’ll discuss the relevance of the map function in these contexts.
Map in React
In React, a popular JavaScript library for building user interfaces, the map function is often used to render lists of components. For example, you might have an array of objects representing users, and you want to display a list of User components.
Here’s how you could use the map function in React:
const users = [{ name: 'Alice' }, { name: 'Bob' }, { name: 'Charlie' }];
function App() {
return (
<div>
{users.map(user => <User name={user.name} />)}
</div>
);
}
// Output:
// A list of User components is rendered, one for each user in the users array.
In this example, the map function is used to transform the users
array into an array of User components. Each User component is rendered with a name
prop corresponding to the name
property of the user object.
Map in Vue
Vue.js, another popular JavaScript framework for building user interfaces, also leverages the power of the map function. In Vue, you might use the map function in combination with the v-for directive to render a list of elements based on an array.
Here’s an example of using the map function in Vue:
const app = new Vue({
el: '#app',
data: {
numbers: [1, 2, 3]
},
computed: {
doubledNumbers() {
return this.numbers.map(number => number * 2);
}
}
});
// Output:
// The doubledNumbers computed property returns a new array where each number is doubled.
In this Vue instance, the map function is used in a computed property to create a new array based on the numbers
data property. The new array is created by doubling each number in numbers
.
Further Resources for Mastering JavaScript Map
To learn more about the map function and its uses in JavaScript, check out these resources:
- Mozilla Developer Network’s guide on Array.prototype.map()
- JavaScript.Info’s article on Array methods, including map
- Eloquent JavaScript’s chapter on Higher-Order Functions, which covers map
These resources provide in-depth explanations and examples of the map function in JavaScript, helping you to deepen your understanding and mastery of this powerful tool.
Wrapping Up: Mastering the Map Function in JavaScript
In this comprehensive guide, we’ve explored the ins and outs of the map function, a powerful tool in JavaScript for transforming arrays.
We began with the basics, understanding what the map function is and how to use it in its simplest form. We then delved deeper into more complex uses, such as mapping over nested arrays and combining map with other array methods like filter and reduce. We also tackled common pitfalls when using the map function and how to avoid them.
In addition, we explored alternative methods to transform arrays, such as using a for loop, the forEach method, and the reduce method. Each of these methods has its strengths and weaknesses, and the best choice depends on the specific needs of your task.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Map | Declarative, returns a new array | Requires understanding of callback functions |
For loop | More control over iteration | Requires more code, less declarative |
forEach | Declarative, easy to understand | Doesn’t return a new array |
Reduce | Powerful and flexible | Can be difficult to understand |
Whether you’re just starting out with JavaScript or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the map function and its alternatives. With its balance of simplicity, flexibility, and power, the map function is a key tool for data transformation in JavaScript. Happy coding!