How to Use jq Online for Efficient JSON Processing
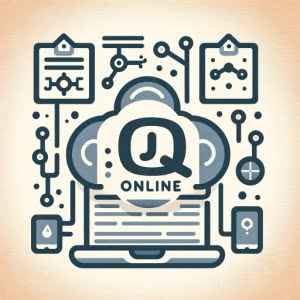
Do you find yourself wrestling with JSON data processing online? You’re not alone. Many developers find JSON data manipulation quite challenging, but there’s a tool that can make this process a breeze.
Like a Swiss Army knife for JSON data, jq is a powerful tool that can simplify your life. It’s a versatile utility that can help you manipulate JSON data in various ways, from simple data extraction to complex filtering and transformation.
This guide will walk you through the basics of using jq in an online context, from simple data manipulation to complex filtering and transformation. We’ll cover everything from making simple JSON manipulations using jq, handling different types of JSON data, to dealing with complex jq operations and even troubleshooting common issues.
So, let’s dive in and start mastering jq online!
TL;DR: How Do I Use jq Online?
You can use online jq tools like
jqplay.org
to process JSON data directly in your browser. Jq is a powerful resource that allows you to filter and transform JSON data, and an online tool simplifies the process.
Here’s a simple example:
# Input JSON data
{"name": "John", "age": 30}
# Filter using jq
.name
# Output
"John"
In this example, we have a JSON object with two properties: name
and age
. Using the jq filter .name
, we can extract the value of the name
property, which in this case is John
.
This is just a basic way to use jq online, but there’s much more to learn about processing JSON data with jq. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with jq Online
If you are new to jq online, don’t worry. We’ve got you covered. Here’s a step-by-step tutorial on how to use an online jq tool for processing JSON data.
Step 1: Accessing the jq Tool
The first step is to access an online jq tool. We recommend using jqplay.org
, which provides a user-friendly interface for processing JSON data.
Step 2: Inputting JSON Data
Once you’ve accessed the tool, you’ll see a section for inputting your JSON data. Here’s an example of JSON data you might input:
# Input JSON data
{"employees": [{"name": "John", "age": 30}, {"name": "Sara", "age": 25}]}
In this example, we have a JSON object that contains an array of employees, each with a name
and age
property.
Step 3: Using jq Filters
Now, let’s say we want to extract the names of all employees. We can do this using the jq filter .employees[].name
. Here’s how you would input this into the jq tool:
# Filter using jq
.employees[].name
Step 4: Viewing the Output
After inputting the filter, you can then view the output. Here’s what the output would look like for our example:
# Output
"John"
"Sara"
As you can see, the jq filter .employees[].name
has extracted the names of all employees from our JSON data.
Pros and Cons of Using jq Online
Using jq online has its benefits. It’s accessible from any device with an internet connection, it doesn’t require any installation, and it’s easy to use. However, for larger JSON data sets, an online tool might be slower than a local installation of jq. Moreover, if you’re dealing with sensitive data, you might want to avoid using an online tool due to privacy concerns.
Advanced jq Techniques for JSON Processing
Once you’ve grasped the basics of jq online, you can start exploring its more advanced features. Let’s delve into some of the more complex jq operations such as filters and pipes.
Advanced Filtering with jq
jq provides a wide array of filters that allow you to manipulate JSON data in different ways. For instance, you can use the map
function to apply a filter to an array of elements. Let’s see how this works with an example:
# Input JSON data
{"employees": [{"name": "John", "age": 30}, {"name": "Sara", "age": 25}]}
# Filter using jq
.employees | map(.age)
# Output
[30, 25]
In this example, we use the map
function to apply the .age
filter to each element in the employees
array. The result is a new array containing the ages of all employees.
Combining Filters with Pipes
You can also combine multiple filters using pipes. This allows you to perform more complex transformations on your JSON data. Here’s an example:
# Input JSON data
{"employees": [{"name": "John", "age": 30}, {"name": "Sara", "age": 25}]}
# Filter using jq
.employees | map(.name) | join(", ")
# Output
"John, Sara"
In this example, we first use the map
function to extract the names of all employees. We then use the join
function to combine these names into a single string, separated by commas.
Potential Issues and Solutions
While jq is a powerful tool, it’s not without its quirks. For instance, you might encounter issues when dealing with special characters in your JSON data. Let’s say you have a JSON object with a property that contains a space, like "first name"
. To access this property with jq, you would need to use double quotes around the property name, like so:
# Input JSON data
{"employees": [{"first name": "John", "age": 30}, {"first name": "Sara", "age": 25}]}
# Filter using jq
.employees | map(".first name")
# Output
["John", "Sara"]
As you can see, by using double quotes around the property name, we can successfully access properties that contain special characters.
Exploring Alternative Methods for Online JSON Processing
While jq is a powerful tool for JSON processing, it’s not the only game in town. There are other methods you can use, especially if you’re comfortable with programming languages like JavaScript or Python. Let’s explore some of these alternatives.
JSON Processing with JavaScript
JavaScript is a language built for handling JSON. Let’s consider an example where we’re trying to extract the names of all employees, similar to what we did with jq:
// Input JSON data
let data = {"employees": [{"name": "John", "age": 30}, {"name": "Sara", "age": 25}]}
// Processing JSON data
let names = data.employees.map(employee => employee.name);
console.log(names);
// Output:
// ['John', 'Sara']
In this JavaScript code, we’re using the map
function to extract the names of all employees from our JSON data. The result is an array of names, just like with our jq example.
JSON Processing with Python
Python is another language that can handle JSON processing. Python’s json module is easy to use and very efficient. Here’s how you could extract employee names using Python:
# Input JSON data
import json
data = json.loads('{"employees": [{"name": "John", "age": 30}, {"name": "Sara", "age": 25}]}')
# Processing JSON data
names = [employee['name'] for employee in data['employees']]
print(names)
# Output:
# ['John', 'Sara']
In this Python code, we’re using a list comprehension to extract the names of all employees from our JSON data. Again, the result is an array of names.
Weighing the Pros and Cons
While JavaScript and Python offer robust solutions for JSON processing, they require more setup and familiarity with the programming language than jq. On the other hand, they offer more flexibility and integration with other parts of your codebase. Consider your specific needs and constraints when choosing your JSON processing tool.
While jq is a powerful tool for JSON processing, you might encounter some obstacles along the way. Let’s look at some common issues you may face when using jq online and how to resolve them.
Handling Special Characters in JSON Keys
One common issue is dealing with JSON keys that contain special characters like spaces or hyphens. In such cases, you need to wrap the key in double quotes. Let’s consider an example:
# Input JSON data
{"employee-data": [{"first name": "John", "age": 30}, {"first name": "Sara", "age": 25}]}
# Incorrect Filter
.employee-data | map(.first name)
# Correct Filter
.employee-data | map(".first name")
# Output
["John", "Sara"]
In this example, the incorrect filter results in an error because of the space in the first name
key. By wrapping the key in double quotes, we can correctly extract the first names of all employees.
Dealing with Large JSON Data Sets
Another issue might be the processing speed when dealing with large JSON data sets. While jq online tools are convenient, they might be slower for larger data sets due to network latency. In such cases, consider using a local installation of jq or processing the data in smaller chunks.
Best Practices and Optimization
To optimize your use of jq online, consider the following tips:
- Use Filters Efficiently: Rather than using multiple filters sequentially, try to accomplish your task with a single, efficient filter.
- Avoid Excessive Nesting: Deeply nested JSON structures can be difficult to navigate with jq. If possible, simplify your JSON structure.
- Watch Out for Special Characters: Remember to wrap keys with special characters in double quotes.
By keeping these considerations in mind, you can make your jq online experience smoother and more efficient.
Understanding JSON and jq Fundamentals
To effectively use jq online, it’s crucial to understand the basic concepts of JSON and jq. Let’s dive deeper into these fundamentals.
What is JSON?
JSON (JavaScript Object Notation) is a popular data format used for representing structured data. It’s easy to read and write, and is widely used for data interchange between a server and a web application. A JSON object contains key-value pairs, where each key is a string, and the value can be a string, number, boolean, null, array, or another JSON object.
Here’s a simple JSON object:
{
"name": "John",
"age": 30,
"isEmployed": true
}
In this JSON object, name
, age
, and isEmployed
are keys, and John
, 30
, and true
are their corresponding values.
What is jq?
jq is a command-line tool that allows you to process JSON data. It provides a flexible query language that allows you to parse, filter, and transform JSON data. With jq, you can extract specific data, combine data from multiple JSON objects, and even manipulate JSON data.
Here’s an example of using jq to extract the name
value from our JSON object:
echo '{"name": "John", "age": 30, "isEmployed": true}' | jq '.name'
# Output:
# "John"
In this command, we’re piping a JSON string into jq, and using the filter .name
to extract the name
value.
Understanding these fundamentals will give you a solid foundation for processing JSON data with jq online.
Expanding Your jq Horizons
jq is an incredibly versatile tool that can be applied in a variety of contexts beyond simple JSON processing tasks. Whether you’re working on large projects or complex online applications, jq can prove to be an invaluable asset.
jq in Large-Scale Projects
In large-scale projects, jq can be used to manage and manipulate large JSON data sets. For instance, you might use jq to filter and transform data from a large JSON API response, or to analyze and process JSON logs.
Here’s an example of how you might use jq to filter a large JSON log file:
cat large-log.json | jq 'select(.level == "error")'
# Output:
# Displays all log entries where the level is "error"
In this command, we’re using the cat
command to read a large JSON log file, and piping the output into jq. The jq filter select(.level == "error")
then selects all log entries where the level
is error
.
jq in Complex Online Applications
jq can also be used in complex online applications. For instance, you might use jq in a serverless function to process JSON data from an API request, or in a web application to filter and transform data for display.
Here’s an example of how you might use jq in a serverless function to process an API request:
echo '{"users": [{"name": "John", "age": 30}, {"name": "Sara", "age": 25}]}' | jq '.users | map(select(.age >= 30))'
# Output:
# [{"name": "John", "age": 30}]
In this command, we’re simulating an API request that returns a JSON object with a users
array. The jq filter .users | map(select(.age >= 30))
then selects all users who are 30 years old or older.
Further Resources for Mastering jq
If you’re looking to further your knowledge and expertise in using jq online, here are some resources that you might find helpful:
- jq Manual: This is the official manual for jq. It provides a comprehensive overview of jq’s features and functionality.
- jq Tutorial: This is an interactive tutorial that allows you to learn jq by doing. It covers everything from basic to advanced jq operations.
- Learn jq with DataCamp: This tutorial by DataCamp provides a practical introduction to jq with plenty of examples and exercises.
Wrapping Up:
In this comprehensive guide, we’ve delved into the world of jq, a powerful tool for JSON data processing. We’ve explored how jq can simplify your life, from basic data manipulation to complex filtering and transformation.
We began with the basics, learning how to use an online jq tool like jqplay.org
to process JSON data. We then ventured into more advanced territory, exploring complex jq operations such as filters and pipes. Along the way, we tackled common challenges you might face when using jq online, such as handling special characters in JSON keys and dealing with large JSON data sets, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to JSON processing, comparing jq with other methods like JavaScript and Python. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
jq | Powerful, flexible | May require troubleshooting for special characters |
JavaScript | Built for handling JSON | Requires more setup |
Python | Robust JSON module | Requires more setup |
Whether you’re just starting out with jq or you’re looking to level up your JSON processing skills, we hope this guide has given you a deeper understanding of jq and its capabilities.
With its balance of power and flexibility, jq is a powerful tool for JSON processing. Now, you’re well equipped to enjoy the benefits of efficient online JSON processing. Happy coding!