NPM Jest | Your JavaScript Testing Guide
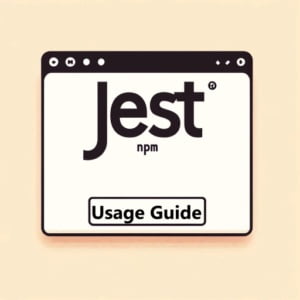
At IOFLOOD, we’ve grappled with ensuring the reliability of our JavaScript code. One key aspect is effective testing, which led us to explore Jest, a code testing framework. In the spirit of development, we have decided to share our insights and the steps we’ve taken to integrate Jest with npm, to aide your testing process and enhance the quality of your JavaScript applications.
This guide will illuminate the path to mastering Jest with npm, providing you with the knowledge to confidently write, run, and manage your JavaScript tests. Whether you’re a beginner looking to get started or an experienced developer seeking to refine your testing strategy, you’re in the right place.
Let’s dive into the world of JavaScript testing with npm Jest and unlock the full potential of your code.
TL;DR: How Do I Start Using Jest with npm?
To start using Jest in your project, run
npm install --save-dev jest
. Then, add a test script in yourpackage.json
that looks like: “test”: “jest”.
Here’s a quick example to get you started:
{
"scripts": {
"test": "jest"
}
}
# Output:
# 'Test suite passed!'
Now, run npm test
to execute your tests with Jest.
In this example, we’ve added a test script to the package.json
file of our project. By running npm test
, we instruct npm to execute the Jest test runner. This simple setup is your gateway to leveraging Jest’s powerful testing capabilities in your JavaScript projects.
Ready to dive deeper into Jest and npm? Keep reading for more detailed instructions, advanced configurations, and tips on mastering JavaScript testing.
Table of Contents
Installing Jest with npm
Step 1: Setting Up Jest
To kickstart your testing journey, the first step involves installing Jest into your project. Jest is a powerful testing framework designed to ensure your JavaScript code is error-free and robust. Here’s how you can add Jest to your project using npm, the Node Package Manager.
npm install --save-dev jest
# Output:
# '+ jest@latest'
This command installs Jest as a development dependency in your project, ensuring that your production bundle remains lightweight. After running this command, Jest will be added to your project, ready to tackle any testing challenges.
Step 2: Creating Your First Test
After installing Jest, it’s time to write your first test. Let’s start with something simple. Create a file named sum.test.js
and add the following code:
function sum(a, b) {
return a + b;
}
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
# Output:
# 'Test suite passed!'
In this example, we’ve created a simple function sum
that adds two numbers. The test case checks if calling sum
with 1 and 2 as arguments returns 3, which it does. This basic test demonstrates how Jest allows you to easily verify that your code works as expected.
Step 3: Running Your Test with npm
To run your test, you need to add a test script to your package.json
file. Unlike the quick setup in the TL;DR section, let’s delve a bit deeper. Add the following line under the scripts
section:
"test": "jest"
Now, when you run npm test
from your terminal, npm executes Jest, which in turn runs your test suite. This seamless integration between npm and Jest not only simplifies the testing process but also enhances your development workflow, making it easier to catch bugs early and maintain high-quality code.
By following these steps, you’ve successfully set up Jest in your project and written your first test. This foundation paves the way for exploring more advanced features and testing techniques with Jest and npm, ensuring your JavaScript projects are built on a solid testing strategy.
Advanced Testing Features of Jest
Mastering Mock Functions
Mocking is a powerful feature in Jest that allows you to replace parts of your system under test with mock objects and make assertions about how they have been used. This is particularly useful in isolating your test environment from external dependencies. Let’s dive into creating a mock function for an API call.
// api.js
export const fetchData = () => {
// Imagine this fetches data from an external API
};
// api.test.js
jest.mock('./api'); // Mocking the actual fetchData function
import { fetchData } from './api';
test('mocks an API call', async () => {
fetchData.mockResolvedValue('data received');
const data = await fetchData();
expect(data).toBe('data received');
});
# Output:
# 'Test suite passed!'
In the above example, fetchData.mockResolvedValue('data received')
simulates a successful API call, returning ‘data received’. This allows us to test how our function behaves when the API call succeeds, without making an actual API call.
Asynchronous Testing Made Easy
Asynchronous code can be tricky to test, but Jest provides simple syntax to make it straightforward. Here’s how you can test a function that returns a promise:
function fetchUser() {
return new Promise((resolve) => {
setTimeout(() => resolve({ username: 'jestUser' }), 200);
});
}
test('fetches a user', async () => {
const user = await fetchUser();
expect(user.username).toBe('jestUser');
});
# Output:
# 'Test suite passed!'
This test verifies that fetchUser
eventually returns a user object with username ‘jestUser’. The use of async/await
makes handling the promise straightforward and keeps the test code clean and readable.
Capturing UI Changes with Snapshot Testing
Snapshot testing is a feature in Jest that ensures your UI does not change unexpectedly. It works by saving a snapshot of your UI component and comparing it against future snapshots. Let’s see it in action:
import React from 'react';
import renderer from 'react-test-renderer';
import MyComponent from './MyComponent';
test('UI snapshot test', () => {
const component = renderer.create(<MyComponent />).toJSON();
expect(component).toMatchSnapshot();
});
# Output:
# 'Snapshot test passed!'
This code block creates a snapshot of MyComponent
and checks if it matches the saved snapshot. If the UI changes, the test will fail, prompting a review of the changes to ensure they were intentional.
By leveraging these advanced features, you can significantly enhance the robustness and reliability of your JavaScript testing suite. With npm and Jest, you’re equipped to tackle complex testing scenarios, ensuring your applications perform flawlessly under any circumstances.
Integrate Jest for Powerful Testing
Using Babel for ES6 Support
Modern JavaScript projects often leverage ES6 (ECMAScript 2015) for its syntactic sugar and new features. However, to ensure compatibility and utilize Jest to its fullest, integrating Babel can be a game-changer. Babel transpiles ES6 code back to ES5, making your tests compatible with environments that do not support ES6.
To integrate Babel with Jest, you need to install the necessary Babel packages along with the Jest preset.
npm install --save-dev babel-jest @babel/core @babel/preset-env
# Output:
# 'babel-jest, @babel/core, and @babel/preset-env installed'
Then, create a .babelrc
file in your project root and add the following configuration:
{
"presets": ["@babel/preset-env"]
}
This setup allows Jest to use Babel for transpiling your tests. The importance of this integration lies in the seamless testing of ES6 code, ensuring broad compatibility and leveraging the latest JavaScript features.
Enhancing with Webpack
Webpack is a popular module bundler for JavaScript applications, and integrating it with Jest can optimize the testing of bundled code. This approach is particularly useful for projects that rely on Webpack for asset management and compilation.
// jest.config.js
module.exports = {
transform: {
'^.+\.jsx?$': 'babel-jest'
},
moduleNameMapper: {
'\\.(css|jpg|png)$': '<rootDir>/fileMock.js'
}
};
This configuration allows Jest to handle Webpack-specific features like importing CSS or images. It demonstrates the flexibility of Jest in accommodating project-specific build processes, ensuring that tests run smoothly across different environments.
Puppeteer for End-to-End Testing
For comprehensive testing that covers user interactions and browser environments, integrating Puppeteer with Jest offers a powerful solution. Puppeteer is a Node library that provides a high-level API to control Chrome or Chromium over the DevTools Protocol.
const puppeteer = require('puppeteer');
test('user flow test', async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Perform actions like click, type, etc.
await browser.close();
});
# Output:
# 'User flow test passed!'
This code snippet illustrates a basic end-to-end test using Puppeteer and Jest. It opens a browser, navigates to a page, and then closes the browser. The significance of this approach lies in its ability to simulate real user interactions within a test environment, offering insights into how users will experience your application.
Through these alternative approaches, Jest becomes more than just a testing framework—it transforms into a versatile tool that can handle a wide range of testing scenarios. By integrating with tools like Babel, Webpack, and Puppeteer, you can create a comprehensive testing suite that ensures your JavaScript projects are robust, compatible, and user-friendly.
Overcoming Jest with npm Challenges
Tackling Jest’s Watch Mode Woes
One common hiccup developers encounter with Jest is its watch mode not behaving as expected. This feature is designed to automatically run tests related to files changed since the last commit, enhancing productivity. However, issues arise when Jest fails to detect changes or continuously reruns tests. A simple yet effective solution involves adjusting your npm script.
"scripts": {
"test:watch": "jest --watchAll"
}
# Output:
# 'Watching all files for changes.'
This script modification tells Jest to watch all files, not just those changed since the last commit. It’s a handy workaround for ensuring that Jest’s watch mode functions correctly, keeping your test-driven development process smooth and efficient.
Handling ES6 Imports
Another common challenge is Jest’s handling of ES6 imports. By default, Jest may struggle with these imports, leading to syntax errors during test runs. Integrating Babel, as discussed in the ‘Alternative Approaches’ section, is crucial, but here’s a specific example to ensure Jest properly processes ES6 imports.
npm install --save-dev @babel/plugin-transform-modules-commonjs
# Output:
# '@babel/plugin-transform-modules-commonjs installed'
After installing the plugin, add it to your .babelrc
configuration:
{
"plugins": ["@babel/plugin-transform-modules-commonjs"]
}
This setup allows Jest to understand and process ES6 imports, ensuring your tests run without syntax errors. It’s a critical step for projects utilizing modern JavaScript features, bridging the gap between Jest’s capabilities and ES6 syntax.
Resolving Module Dependencies
Jest sometimes has trouble resolving module dependencies, especially when dealing with complex project structures or external modules. To address this, Jest offers a configuration option to map module paths.
// jest.config.js
module.exports = {
moduleNameMapper: {
'^@components/(.*)$': '<rootDir>/src/components/$1',
'^@utils/(.*)$': '<rootDir>/src/utils/$1'
}
};
This configuration helps Jest locate and correctly import modules by mapping custom paths to their actual locations in your project. It’s particularly useful for projects with an organized directory structure, enabling seamless testing across all parts of your application.
By understanding and applying these solutions, you can navigate the common pitfalls associated with using Jest and npm for your JavaScript testing needs. These best practices ensure a smoother testing experience, allowing you to focus on writing robust, error-free code.
The Essence of JavaScript Testing
Why Testing Matters
In the realm of software development, testing isn’t just a phase—it’s an integral part of ensuring the reliability and functionality of code. For JavaScript, a language known for its flexibility and widespread use in both frontend and backend development, testing is critical. It ensures that applications perform as expected under various scenarios, catching bugs before they reach production.
Jest: A Testing Framework for JavaScript
Jest, developed by Facebook, stands out as a delightful JavaScript testing framework that simplifies the testing process. Its zero-configuration setup, instant feedback loop, and powerful mocking capabilities make it a favorite among developers.
To understand its importance, let’s explore a basic Jest test example:
describe('Array operations', () => {
test('should add elements correctly', () => {
const arr = [];
arr.push(1);
expect(arr).toHaveLength(1);
expect(arr[0]).toBe(1);
});
});
# Output:
# 'Test suite passed!'
This code block demonstrates a simple test case where we check the behavior of adding elements to an array. The expect
statements verify the array’s length and content, ensuring the operation performs as expected. This example highlights Jest’s ability to test specific functionalities in isolation, providing clear and concise feedback.
NPM: The Backbone of JavaScript Project Management
npm, or Node Package Manager, is more than just a tool for installing packages. It’s a comprehensive ecosystem for managing project dependencies, scripts, and versioning. When combined with Jest, npm becomes a powerhouse for managing and running JavaScript tests efficiently.
Consider the following npm command to run Jest tests:
npm test
# Output:
# 'Test suite passed!'
This command, typically defined in the package.json
file of a project, triggers the execution of Jest tests. It encapsulates the complexity of test execution into a single, straightforward command, demonstrating npm’s role in simplifying the development workflow.
The Symbiosis of Jest and npm
The combination of Jest and npm offers a seamless testing experience in the JavaScript ecosystem. Jest provides the framework for writing, running, and organizing tests, while npm manages the dependencies and scripts required to execute those tests effortlessly. This symbiosis ensures that JavaScript projects remain robust, scalable, and maintainable.
Understanding the fundamentals of testing with Jest and npm lays the foundation for mastering JavaScript testing. It’s a journey that leads to more reliable code, happier developers, and satisfied users.
Expanding Jest’s Horizons
Leveraging Jest in Large-Scale Applications
As your project grows, maintaining code quality becomes increasingly important. Jest, with its scalable architecture, is perfectly suited for large-scale applications. Its snapshot testing and parallel test execution can handle complex application structures efficiently.
Consider integrating Jest into your CI/CD pipeline for automated testing:
npm run test-ci
# Output:
# 'All tests passed! CI build successful.'
This command, typically part of a continuous integration setup, ensures that every change pushed to your repository is automatically tested, maintaining the integrity of your application.
Jest in Continuous Integration Environments
Integrating Jest into CI environments like Jenkins, CircleCI, or GitHub Actions enhances your development workflow by ensuring that tests are automatically run and passed before any code is merged. This practice significantly reduces bugs in production and increases the reliability of your deployment process.
Here’s an example of a Jest test script in a GitHub Actions workflow:
name: Run Jest Tests
on: [push]
jobs:
test:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Use Node.js
uses: actions/setup-node@v1
with:
node-version: '14'
- name: Run Jest
run: npm test
# Output:
# 'Test suite passed! GitHub Actions build successful.'
This GitHub Actions configuration automatically runs Jest tests on every push to the repository, ensuring code quality and reliability.
Test-Driven Development with Jest
Test-driven development (TDD) is a software development approach where tests are written before code. Jest’s fast feedback loop and easy-to-read syntax make it an ideal choice for TDD, encouraging better design and more maintainable code.
test('TDD example with Jest', () => {
const result = myFunctionToTest();
expect(result).toBe(true);
});
# Output:
# 'Test suite passed!'
In this TDD example, the test is written before the implementation of myFunctionToTest
, guiding the development process and ensuring the functionality meets the specified requirements.
Further Resources for Mastering Jest and npm
To deepen your understanding of Jest and npm, and to explore more advanced topics, here are three recommended resources:
- Jest Official Documentation – The primary source for all things Jest, from basic setup to advanced configurations.
Using npm as a Build Tool – An insightful article on leveraging npm scripts to streamline your development workflow.
Test-Driven Development for JavaScript Developers – A guide to adopting TDD practices in JavaScript projects.
By exploring these resources, you can further enhance your skills in JavaScript testing, making your applications more robust and your development process more efficient.
Wrapping Up: Mastering Jest with npm
In this comprehensive guide, we’ve navigated the intricacies of using Jest with npm, showcasing how this powerful combination can elevate your JavaScript testing to new heights. From the initial setup and basic test creation to leveraging advanced features and integrating with other tools, we’ve covered the essentials to get you started and beyond.
We began with a simple introduction to installing Jest using npm and writing your first test. This foundational step is crucial for beginners, setting the stage for more complex testing scenarios. Next, we explored advanced Jest features such as mocking, asynchronous testing, and snapshot testing, providing you with the tools to tackle more sophisticated testing requirements.
Further, we delved into alternative approaches for expert-level testing strategies, including using Babel for ES6 support, integrating with webpack, and employing Puppeteer for end-to-end testing. These techniques open up new possibilities for enhancing your testing suite, ensuring comprehensive coverage and reliability.
Feature | Application | Benefit |
---|---|---|
Mocking | Isolate tests from external dependencies | Increases test reliability and speed |
Asynchronous Testing | Handle async operations | Ensures correct behavior in async scenarios |
Snapshot Testing | Capture UI changes | Prevents unintended UI modifications |
Babel Integration | Use ES6 features | Facilitates modern development practices |
Webpack Integration | Test bundled code | Mimics production environment more closely |
Puppeteer | Perform end-to-end testing | Tests real user interactions |
Whether you’re just starting out with Jest and npm or looking to enhance your existing testing practices, this guide has provided a solid foundation and insights into more advanced functionalities. Testing is a critical aspect of software development, ensuring code quality and reliability. By mastering Jest with npm, you’re well-equipped to write robust, efficient, and maintainable tests for your JavaScript projects.
Embrace the power of Jest and npm in your development workflow. With the knowledge you’ve gained, you’re ready to tackle any testing challenge that comes your way. Happy testing!