AWK Delimiter Usage Guide | Field Separation Techniques
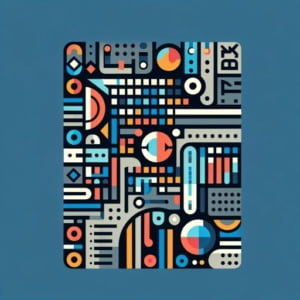
At IOFLOOD, parsing data from various file formats is a regular task. Often, we use awk
to handle files with different delimiters. To assist our bare metal hosting customers and fellow developers with data parsing tasks, we have crafted today’s article on how to specify and use delimiters in awk
.
In this guide, we’ll walk you through the process of using delimiters in AWK, from their basic usage to more advanced techniques. We’ll cover everything from specifying a simple delimiter with the -F option, handling multiple or complex delimiters, to alternative approaches and troubleshooting common issues.
Let’s dive in and start mastering AWK delimiters!
TL;DR: How Do I Use a Delimiter in AWK?
In AWK, you can specify a delimiter using the
-F
option and the basic syntax,awk -F '<delimiter>' '{print $<field_number>}' filename.txt
This allows AWK to split text data into fields, making it easier to process and analyze.
Here’s a simple example:
echo 'apple,banana,orange' | awk -F ',' '{print $1}'
# Output:
# 'apple'
In this example, we’re using AWK with the -F
option to specify a comma (,
) as the delimiter. The command echo 'apple,banana,orange'
outputs the string ‘apple,banana,orange’, which is then piped into AWK. The AWK command {print $1}
prints the first field, which is ‘apple’ in this case.
This is just a basic way to use a delimiter in AWK, but there’s much more to learn about handling text data with AWK. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- The Basics of AWK Delimiters
- Leveraging Multiple Delimiters in AWK
- Decoding Complex Delimiters
- Alternate AWK Delimiter Techniques
- Troubleshooting AWK Delimiters
- Understanding AWK Language
- The Role of Delimiters in Text Data
- Practical Uses of AWK Delimiters
- Exploring Related Topics
- Recap: AWK Delimiter Tips and Tricks
The Basics of AWK Delimiters
AWK uses the -F
option to specify a delimiter. A delimiter is a character or a sequence of characters that separates fields in a line of text. By default, AWK uses whitespace (spaces, tabs) as the delimiter. But with the -F
option, you can specify any character as the delimiter.
Let’s consider a simple example:
echo 'Hello-World-This-Is-AWK' | awk -F '-' '{print $1, $4}'
# Output:
# 'Hello AWK'
In this example, we’re using a hyphen (-
) as the delimiter. The echo
command outputs the string ‘Hello-World-This-Is-AWK’, which is then piped into AWK. The AWK command {print $1, $4}
prints the first and fourth fields, separated by the hyphen, which are ‘Hello’ and ‘AWK’ respectively.
The -F
option is incredibly useful when you’re working with structured text data, like CSV files. However, it’s important to be aware of potential pitfalls. For example, if your data contains the delimiter character within a field, AWK might split the field in unexpected ways. It’s always a good idea to understand your data and choose your delimiter carefully.
Leveraging Multiple Delimiters in AWK
AWK isn’t just limited to using a single character as a delimiter. It can handle multiple delimiters or even complex delimiters, which can be extremely useful when dealing with intricate text data structures.
Let’s look at an example where we use multiple delimiters:
echo 'apple-banana:orange' | awk -F '[-:]' '{print $2}'
# Output:
# 'banana'
In this example, we’ve defined two delimiters, a hyphen (-
) and a colon (:
), by enclosing them in square brackets. The string ‘apple-banana:orange’ is split into three fields: ‘apple’, ‘banana’, and ‘orange’. The AWK command {print $2}
prints the second field, which is ‘banana’.
This ability to specify multiple delimiters can be a powerful tool when dealing with complex text data. However, it’s essential to understand that AWK will treat each character in the -F
option as a separate delimiter. So, choose your delimiters wisely to ensure accurate data parsing.
Decoding Complex Delimiters
Sometimes, your data may require a sequence of characters or a pattern as a delimiter. AWK can handle this too. Let’s see an example:
echo 'apple::banana::orange' | awk -F '::' '{print $3}'
# Output:
# 'orange'
Here, we’re using a double colon (::
) as the delimiter. The string ‘apple::banana::orange’ is split into three fields: ‘apple’, ‘banana’, and ‘orange’. The AWK command {print $3}
prints the third field, which is ‘orange’.
Using complex delimiters can be handy, but remember that they can also make your AWK commands more complicated. Always test your commands thoroughly to ensure they’re working as expected.
Alternate AWK Delimiter Techniques
While the -F
option is a common way to specify delimiters in AWK, it’s not the only way. There are other methods to set delimiters that can offer more flexibility or better suit certain scenarios. Let’s explore two such alternatives: the BEGIN block and the split function.
Using the BEGIN Block to Set Delimiters
The BEGIN block in AWK allows you to set a delimiter before any input is processed. This can be particularly useful when you want to apply the same delimiter to multiple lines of input. Check out the following example:
echo -e 'apple banana
orange pear' | awk 'BEGIN {FS=" "} {print $2}'
# Output:
# 'banana'
# 'pear'
In this code, we use the BEGIN block to set the field separator (FS) to a space. The echo command outputs two lines of text, ‘apple banana’ and ‘orange pear’, which are piped into AWK. The AWK command {print $2}
prints the second field of each line, ‘banana’ and ‘pear’.
Splitting Fields with the split Function
AWK’s split function is another powerful tool for handling delimiters. It allows you to split a string into an array of fields based on a specified delimiter. Here’s an example:
echo 'apple:banana:orange' | awk '{split($0,a,":"); print a[3]}'
# Output:
# 'orange'
In this command, we use the split function to divide the input string into an array a
based on the colon delimiter. The AWK command print a[3]
then prints the third element of the array, which is ‘orange’.
The split function is especially useful when you need to manipulate fields individually or in complex ways. However, it can make your AWK commands more complicated, so it’s best used when necessary.
Both the BEGIN block and the split function offer unique advantages and potential drawbacks. Your choice of method will depend on your specific needs and the nature of your text data.
Troubleshooting AWK Delimiters
While AWK delimiters are a powerful tool, they can sometimes lead to unexpected results or errors. Let’s discuss some common issues you might encounter and how to resolve them.
Problem: Unexpected Field Splitting
One common issue is when AWK splits a field in an unexpected way. This usually happens when your data contains the delimiter character within a field. Let’s see an example:
echo 'apple,banana,orange,grape,fruit' | awk -F ',' '{print $3}'
# Output:
# 'orange'
In this example, we’re using a comma (,
) as the delimiter. But what if ‘orange,grape’ was supposed to be a single field? AWK would still split it into two fields, leading to unexpected results.
Solution: Choosing the Right Delimiter
The solution to this problem is to choose your delimiter carefully. Make sure it’s a character that doesn’t appear within your fields. If necessary, you can use multiple or complex delimiters to avoid this issue.
Problem: Missing Fields
Another common issue is missing fields. This can happen when you’re using a delimiter that doesn’t exist in your data. For instance:
echo 'apple banana orange' | awk -F ',' '{print $2}'
# Output:
# ''
Here, we’re using a comma (,
) as the delimiter, but our data doesn’t contain any commas. As a result, AWK doesn’t split the data into fields, and the second field is missing.
Solution: Checking Your Data
The solution to this problem is to check your data before processing it with AWK. Make sure your data contains the delimiter you’re using. If necessary, you can preprocess your data to replace or add the correct delimiters.
Working with AWK delimiters requires careful attention to your data and the delimiters you’re using. By understanding the potential pitfalls and how to avoid them, you can use AWK delimiters effectively and efficiently.
Understanding AWK Language
Before we dive deeper into the use of delimiters, it’s essential to understand the basics of the AWK programming language and its role in text processing.
AWK is a powerful and versatile programming language designed for text processing and data extraction. It’s named after its creators: Alfred Aho, Peter Weinberger, and Brian Kernighan.
AWK shines when it comes to processing structured text data. It can read a file line by line, split each line into fields, and perform actions on each field. This makes it an excellent tool for tasks such as data extraction, report generation, and automation of complex text transformations.
The Role of Delimiters in Text Data
Delimiters play a crucial role in text data processing. They define the boundaries between different pieces of data, or ‘fields’. In a CSV file, for example, the comma character is a delimiter that separates each field.
echo 'apple,banana,orange' | awk -F ',' '{print $2}'
# Output:
# 'banana'
In this example, we use a comma (,
) as the delimiter to separate the fields ‘apple’, ‘banana’, and ‘orange’. The AWK command {print $2}
prints the second field, which is ‘banana’.
By understanding and effectively using delimiters, you can accurately and efficiently parse and process text data with AWK. Whether you’re dealing with simple or complex data structures, mastering delimiters will empower you to handle them with ease.
Practical Uses of AWK Delimiters
AWK delimiters are not just theoretical concepts; they have practical applications in real-world scenarios. The ability to parse and process text data efficiently can be a game-changer in areas like data analysis and log file processing.
AWK Delimiters in Data Analysis
Data analysis often involves dealing with large datasets, usually in structured formats like CSV or TSV files. AWK delimiters can split this data into manageable fields, making it easier to analyze and extract insights.
cat data.csv | awk -F ',' '{sum += $5} END {print sum}'
# Output:
# Total of all values in the fifth column
In this example, we’re summing all the values in the fifth column of a CSV file. The AWK command adds up the values in the fifth field ($5
) for each line and prints the total sum at the end.
AWK Delimiters in Log File Processing
Log files are another area where AWK delimiters prove useful. Log files often contain structured text data that can be parsed using AWK to extract valuable information or debug issues.
cat server.log | awk -F ' ' '{print $1, $7}'
# Output:
# IP addresses and request URIs from a web server log
In this example, we’re extracting the IP addresses and request URIs from a web server log. The AWK command prints the first and seventh fields, which correspond to the IP address and request URI.
Exploring Related Topics
Once you’ve mastered AWK delimiters, you might want to explore related topics like regular expressions in AWK or text processing with other tools. Regular expressions can provide even more power and flexibility in handling text data. Other tools like sed or grep also offer unique capabilities for text processing.
Further Resources for AWK Delimiter Mastery
If you’re interested in delving deeper into AWK and its applications, here are some resources that you may find helpful:
- The GNU Awk User’s Guide – A comprehensive guide to AWK from the creators of GNU Awk.
Data Processing with AWK – A blog post discussing various data processing tasks with AWK.
Effective AWK Programming – A book by Arnold Robbins, a recognized expert in AWK.
These resources will provide you with a deeper understanding of AWK and its capabilities, helping you become more proficient in handling text data.
Recap: AWK Delimiter Tips and Tricks
In this comprehensive guide, we’ve delved into the world of AWK delimiters, exploring their usage from the basics to advanced techniques. We’ve seen how AWK delimiters can be a powerful tool in text data processing, enabling you to dissect and analyze structured text data effectively.
We started with the basics, learning how to use the -F
option to specify a simple delimiter in AWK. We then moved onto more advanced topics, such as handling multiple or complex delimiters and alternative approaches like the BEGIN block and the split function.
Along the way, we addressed common issues you might encounter when using AWK delimiters, such as unexpected field splitting and missing fields, and provided solutions for each problem. We also discussed the importance of understanding your data and choosing your delimiters wisely to avoid potential pitfalls.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
-F Option | Moderate | Low |
Multiple/Complex Delimiters | High | Moderate |
BEGIN Block | Moderate | Moderate |
split Function | High | High |
Whether you’re just starting out with AWK or you’re looking to enhance your text processing skills, we hope this guide has given you a deeper understanding of AWK delimiters and their capabilities.
With the ability to handle simple to complex text data structures, mastering AWK delimiters will empower you to handle text data more efficiently. Happy coding!