AWK Data Manipulation Guide | Practical Examples in Unix
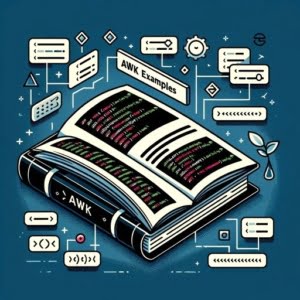
When it comes to automating data manipulation tasks for software at IOFLOOD, understanding how to use AWK helps tremendously. Drawing from our experience, AWK has proven to be an invaluable tool for extracting, transforming, and analyzing data. In today’s article, we’ll delve into practical AWK examples to aid our dedicated cloud service customers and fellow developers in harnessing AWK’s capabilities for seamless data manipulation.
This guide will walk you through practical examples of using AWK, from basic to advanced scenarios. We’ll explore AWK’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering AWK!
TL;DR: How Do I Use AWK for Data Manipulation?
AWK is a powerful command-line tool used for data manipulation in Unix-like operating systems. A common use case is to print specific fields from a file. For instance, to print the first field in a text file, you could use
awk '{print $1}' file.txt
.
Here’s a simple example:
echo -e 'apple
banana
cherry' > fruits.txt
awk '{print $1}' fruits.txt
# Output:
# apple
# banana
# cherry
In this example, we first create a file named fruits.txt
with three lines: apple, banana, and cherry. We then use the AWK command to print the first field (in this case, the entire line as there’s only one field) from the file. The output is the contents of the file printed line by line.
This is just a basic use of the AWK command, but there’s so much more you can do with it. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
AWK Basics: Simple Text Processing
AWK shines when it comes to simple text processing tasks. For instance, you can use it to print specific fields from a file. In AWK, a field is a unit of data separated from other fields by a delimiter, such as a space or a tab.
Consider the following example:
echo -e 'apple fruit
banana fruit
carrot vegetable' > food.txt
awk '{print $1}' food.txt
# Output:
# apple
# banana
# carrot
In this example, we create a file named food.txt
with three lines. Each line contains two fields separated by a space. We then use the AWK command to print the first field from each line in the file. The output is the first word of each line.
AWK is advantageous because it simplifies the process of handling and manipulating text data. However, it’s important to be aware of potential pitfalls. For instance, if the fields in your file are not consistently separated by the same delimiter, AWK might not behave as expected. In such cases, you may need to specify a different field separator using the -F
option in AWK.
Advanced Features of AWK
As you become more comfortable with AWK, you can start to explore its built-in variables and functions. These features allow you to perform more complex data manipulation tasks.
Let’s consider an example where we use the built-in variable NF
(Number of Fields) and the function length
.
echo -e 'apple fruit
banana fruit
carrot vegetable' > food.txt
awk '{print $1, $2, length($1), NF}' food.txt
# Output:
# apple fruit 5 2
# banana fruit 6 2
# carrot vegetable 6 2
In this example, we’re using AWK to print each line from the food.txt
file, along with the length of the first field and the total number of fields. The length($1)
function returns the length of the first field, and NF
returns the number of fields in each line.
This demonstrates how AWK’s built-in variables and functions can provide additional information about your data. But remember, AWK is a powerful tool with many more features to explore. As you continue to learn and experiment, you’ll discover even more ways to use AWK for data manipulation.
Exploring Alternatives to AWK
While AWK is a powerful tool for data manipulation, it’s not the only one in the toolbox. Other utilities like sed, grep, and Perl can also be used for similar tasks. Let’s take a look at how each of these alternatives can be used.
Sed for Stream Editing
Sed, short for Stream Editor, is a utility that parses and transforms text. It’s particularly useful for find-and-replace operations.
echo 'apple fruit' | sed 's/apple/pear/'
# Output:
# pear fruit
In this example, we’re using sed to replace ‘apple’ with ‘pear’. The ‘s’ command in sed stands for substitute, followed by the text to find and replace, separated by slashes.
Grep for Pattern Matching
Grep is a command-line utility used for searching text or files for lines that match a certain pattern. It’s an invaluable tool when you need to find specific strings in a file.
echo -e 'apple
banana
carrot' | grep 'a'
# Output:
# apple
# banana
Here, we’re using grep to print lines that contain the letter ‘a’. The output is the lines ‘apple’ and ‘banana’.
Perl for Advanced Programming
Perl is a high-level programming language often used for text manipulation. It’s more complex than AWK, sed, or grep, but it also offers more flexibility and functionality.
#!/usr/bin/perl
@fruits = ('apple', 'banana', 'cherry');
foreach $fruit (@fruits) {
print "$fruit
";
}
# Output:
# apple
# banana
# cherry
This Perl script creates an array of fruits and then prints each fruit on a new line.
Each of these tools has its own strengths and weaknesses. AWK is versatile and relatively easy to use, making it a great choice for many data manipulation tasks. However, for more complex tasks, you might find that Perl’s advanced features are more suitable. Similarly, for simple find-and-replace operations, sed might be the most efficient choice. And when you need to find lines that match a specific pattern, grep is your best bet.
Choosing the right tool depends on your specific needs and the nature of your data. It’s always a good idea to familiarize yourself with a range of tools so you can choose the best one for the job.
Troubleshooting Tips for AWK
As you start working with AWK, you may encounter a few common issues. Let’s discuss these potential problems and how to solve them.
AWK Syntax Errors
Syntax errors are common, especially for beginners. They occur when the AWK command does not follow the correct syntax.
awk '{print $1' file.txt
# Output:
# awk: {print $1
# awk: ^ syntax error
In this example, we’ve forgotten to close the curly brace. AWK throws a syntax error indicating the problem. The solution is to correct the syntax by adding the missing brace.
Unexpected Output
Sometimes, you might get output that you weren’t expecting. This usually happens when the data doesn’t match your assumptions.
echo -e 'apple
banana fruit
carrot vegetable' > food.txt
awk '{print $1}' food.txt
# Output:
# apple
# banana
# carrot
In this example, we’re trying to print the first field from each line in the file. However, the first line only has one field, so the output might not be what you expected if you assumed that all lines had two fields.
Tips for Using AWK
- Always double-check your syntax. If you encounter a syntax error, carefully review your command to ensure it follows the correct AWK syntax.
Test your AWK command on a small, representative sample of your data before running it on the entire dataset. This can help you catch and correct any errors or unexpected behavior.
Understand your data. Make sure you know how many fields each line has and what delimiter is being used.
Remember, troubleshooting is a normal part of working with any new tool. Don’t be discouraged by these challenges. With time and practice, you’ll become more proficient with AWK.
The Fundamentals of AWK
AWK, named after its creators Aho, Weinberger, and Kernighan, is a powerful tool designed for text processing. Its core functionality revolves around processing structured text data, making it a go-to utility for tasks involving data extraction, reporting, and text transformation.
Understanding AWK Syntax
The basic syntax of an AWK command is awk 'pattern {action}' file
, where:
pattern
is a condition that you specify. AWK will apply the action to lines that match this pattern.action
is what AWK does when it finds a line that matches the pattern. This could be printing the line, modifying it, or performing some calculation.file
is the file that AWK reads.
echo -e 'apple fruit
banana fruit
carrot vegetable' > food.txt
awk '/fruit/ {print $1}' food.txt
# Output:
# apple
# banana
In this example, the pattern is /fruit/
and the action is {print $1}
. AWK reads food.txt
, looks for lines that contain ‘fruit’, and prints the first field of those lines.
AWK’s Design and Philosophy
AWK’s design philosophy revolves around handling structured text data. It treats text as a sequence of records (lines) and fields (parts of a line), allowing you to easily manipulate text by specifying patterns and actions.
AWK also provides a range of built-in variables and functions that you can use to perform more complex operations. For instance, NF
gives you the number of fields in a line, and length
returns the length of a string.
Understanding these fundamentals will help you unlock AWK’s full potential. With this knowledge, you can start to see why AWK is such a powerful tool for data manipulation.
AWK in Real-World Applications
AWK isn’t just a theoretical tool – it has numerous practical applications in the real world. Let’s explore some of these scenarios to understand AWK’s relevance and versatility.
Log Analysis with AWK
AWK is a powerful tool for log analysis. With its text processing capabilities, you can easily extract, filter, and summarize data from log files.
echo -e 'ERROR: Disk full
INFO: User logged in
ERROR: File not found' > log.txt
awk '/ERROR/ {print $0}' log.txt
# Output:
# ERROR: Disk full
# ERROR: File not found
In this example, we’re using AWK to filter out lines containing ‘ERROR’ from a log file. This can be extremely useful when you’re troubleshooting issues and need to focus on error messages.
Data Extraction with AWK
AWK excels at extracting specific data from structured text files. For instance, you might use AWK to extract user names from a /etc/passwd file in a Unix-like system.
awk -F':' '{print $1}' /etc/passwd
# Output:
# (A list of user names)
In this example, we’re using AWK to print the first field (user names) from the /etc/passwd file. The -F':'
option tells AWK to use ‘:’ as the field separator.
Regular Expressions and Shell Scripting
AWK’s power is amplified when you combine it with regular expressions and shell scripting. Regular expressions allow you to match complex patterns in text, while shell scripting lets you automate and combine multiple commands.
awk '/[0-9]$/ {print $0}' file.txt
# Output:
# (Lines from file.txt that end with a number)
In this example, we’re using a regular expression (/[0-9]$/
) to match lines that end with a number.
Further Resources for Mastering AWK
Ready to dive deeper into AWK? Here are some resources that can help you further your understanding:
- GNU AWK User’s Guide: This comprehensive guide covers all aspects of AWK, from basic to advanced features.
The AWK Programming Language: This book by AWK’s creators provides an in-depth look at AWK’s design and capabilities.
AWK – A Tutorial: This tutorial provides a practical introduction to AWK with plenty of examples.
Remember, mastering a tool like AWK takes time and practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy AWKing!
Recap: Practical AWK examples
In this comprehensive guide, we’ve explored the world of AWK, a powerful tool for data manipulation in Unix-like operating systems.
We began with the basics, learning how to use AWK for simple text processing tasks. We then ventured into more advanced territory, exploring AWK’s built-in variables and functions, which allow for more complex data manipulation scenarios.
Along the way, we tackled common challenges you might face when using AWK, such as syntax errors and unexpected output, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to data manipulation, comparing AWK with other tools like sed, grep, and Perl. Here’s a quick comparison of these tools:
Tool | Versatility | Complexity | Use Case |
---|---|---|---|
AWK | High | Moderate | Data manipulation |
Sed | Moderate | Low | Find and replace |
Grep | Low | Low | Pattern matching |
Perl | High | High | Advanced text processing |
Whether you’re just starting out with AWK or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of AWK and its capabilities.
With its balance of versatility and ease of use, AWK is a powerful tool for data manipulation. Keep practicing, keep experimenting, and you’ll soon master the art of AWK. Happy AWKing!