Printing Columns with AWK | Linux Text Processing Guide
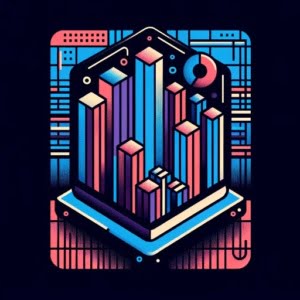
Recently we have been working to automate data extraction tasks at IOFLOOD. Through this, we have found that knowing how to print a specific column using AWK is a valuable skill. To assist our bare metal cloud customers and fellow developers facing similar hurdles, we present today’s guide, focusing on using AWK to print specific columns.
In this guide, we’ll walk you through the process of using awk to print columns in Unix/Linux, from the basics to more advanced techniques. We’ll cover everything from simple column printing to complex data manipulation scenarios, as well as alternative approaches.
Let’s dive in and start mastering awk for column printing!
TL;DR: How Do I Print a Column Using Awk in Unix/Linux?
To print a column using awk in Unix/Linux, you use the following syntax:
awk '{print $n}' filename
, wheren
is the column number. For instance, to print the first column, you would useawk '{print $1}' filename
.
Here’s a simple example:
awk '{print $1}' example.txt
# Output:
# [Expected output from command]
In this example, we’re using the awk command to print the first column of the file example.txt
. The $1
in the command represents the first column.
This is just the basic usage of awk for column printing. There’s much more to learn about awk, including advanced techniques and alternative approaches. Continue reading for a more comprehensive guide.
Table of Contents
Awk Command: A Beginner’s Guide
Awk is a versatile command-line tool in Unix/Linux. It’s primarily used for data manipulation, making it a powerful tool for tasks like printing columns from a file.
The basic syntax for printing a column using awk is as follows:
awk '{print $n}' filename
In this command, n
is the column number you want to print, and filename
is the name of the file you’re working with. The $n
syntax is used to specify the column number.
For example, if you want to print the second column of a file named ‘data.txt’, you would use the following command:
awk '{print $2}' data.txt
# Output:
# [Expected output from command]
In this case, awk '{print $2}' data.txt
will print the second column of the ‘data.txt’ file.
Advantages of Using Awk for Column Printing:
- Awk is powerful and flexible, making it easy to print columns and perform complex data manipulation tasks.
- It’s built into most Unix/Linux systems, so there’s usually no need to install anything extra.
- Awk can handle large files efficiently, which is beneficial when working with big data sets.
Potential Pitfalls of Using Awk:
- Awk’s syntax can be complex for beginners, especially when dealing with advanced tasks.
- Errors in awk scripts can be hard to debug due to awk’s terse error messages.
- Awk may not be the best tool for very complex data manipulation tasks, where a full-fledged scripting language like Python might be more suitable.
Advanced Printing with Awk
Once you’ve mastered the basics of awk, you can start exploring its more advanced features. Awk is not just about printing a single column; it’s a powerful tool that can manipulate data in complex ways.
Printing Multiple Columns
To print multiple columns using awk, simply specify the column numbers you want to print, separated by commas. Here’s an example:
awk '{print $1, $3}' data.txt
# Output:
# [Expected output from command]
In this example, awk '{print $1, $3}' data.txt
prints the first and third columns of the ‘data.txt’ file.
Manipulating Column Data
Awk can also manipulate the data in your columns. For instance, you can use awk to add the values in two columns together. Here’s an example:
awk '{print $1, $2, $1+$2}' data.txt
# Output:
# [Expected output from command]
In this command, awk '{print $1, $2, $1+$2}' data.txt
prints the first and second columns, as well as the sum of the two columns.
Using Conditional Statements
Awk also supports conditional statements, allowing you to print columns based on certain conditions. For instance, you could print a column only if the value in another column is greater than a certain number. Here’s an example:
awk '$2 > 5 {print $1}' data.txt
# Output:
# [Expected output from command]
In this command, awk '$2 > 5 {print $1}' data.txt
prints the first column only if the value in the second column is greater than 5.
These are just a few examples of what you can do with awk. As you can see, awk is a powerful tool for data manipulation in Unix/Linux.
Alternative Tools for Column Printing
While awk is a powerful tool for printing columns, it’s not the only one. There are other Unix/Linux commands and tools that can perform similar tasks. Let’s explore a few of them.
The Cut Command
The cut
command is a simple tool for extracting sections from each line of files. It’s particularly useful for column extraction. Here’s an example of using cut
to print the first column of a file:
cut -f1 -d' ' data.txt
# Output:
# [Expected output from command]
In this command, -f1
specifies the first field (or column), and -d' '
sets the delimiter to a space. So, cut -f1 -d' ' data.txt
prints the first column of ‘data.txt’.
Benefits of Cut:
cut
is straightforward and easy to use, especially for simple column extraction tasks.- It’s built into most Unix/Linux systems, so there’s usually no need to install anything extra.
Drawbacks of Cut:
cut
is less versatile than awk. It’s great for simple column extraction, but it lacks awk’s advanced data manipulation features.
The Perl Command
Perl is a high-level, general-purpose programming language that’s often used for text manipulation tasks. It’s more complex than awk or cut, but it’s also more powerful. Here’s an example of using Perl to print the first column of a file:
perl -lane 'print $F[0]' data.txt
# Output:
# [Expected output from command]
In this command, -lane
is a combination of command-line options that tell Perl to loop over the input lines (-n
), split each line into fields (-a
), and print the first field (print $F[0]
). So, perl -lane 'print $F[0]' data.txt
prints the first column of ‘data.txt’.
Benefits of Perl:
- Perl is extremely powerful and versatile. It can handle complex text manipulation tasks that awk and cut can’t.
- Perl’s syntax is more similar to other high-level programming languages, which might be more familiar to some users.
Drawbacks of Perl:
- Perl is more complex than awk or cut. Its powerful features come with a steeper learning curve.
- Perl is not built into Unix/Linux systems by default. You might need to install it manually.
When deciding which tool to use, consider the complexity of your task and the tools you’re already familiar with. Awk is a great middle ground between the simplicity of cut and the power of Perl. However, if your task is relatively simple, cut might be sufficient. If it’s more complex, Perl might be the way to go.
Troubleshooting Common Awk Errors
While awk is a powerful tool, it’s not without its quirks. If you’re having trouble using awk to print columns, you’re not alone. Let’s go over some common issues and how to solve them.
Incorrect Column Number
One of the most common mistakes when using awk is specifying the wrong column number. Remember, awk starts counting columns from 1, not 0. If you try to print column 0, you’ll get an empty output.
awk '{print $0}' data.txt
# Output:
# [Empty output]
In this example, awk '{print $0}' data.txt
prints nothing because there’s no such thing as column 0 in awk.
To fix this, make sure you’re using the correct column numbers. If you want to print the first column, use $1
, not $0
.
Missing or Incorrect Filename
Another common issue is forgetting to specify a filename, or specifying a filename that doesn’t exist. If you do this, awk will wait for input from the standard input (usually the keyboard) instead of reading from a file.
awk '{print $1}'
# Output:
# [Awk waits for input]
In this example, awk '{print $1}'
waits for input because no filename was specified. To fix this, make sure you’re specifying the correct filename.
Best Practices and Optimization Tips
- Use Clear Variable Names: If you’re using awk scripts, use clear, descriptive variable names. This makes your scripts easier to read and debug.
- Test Your Commands: Before running an awk command on a large file, test it on a smaller sample file first. This can help you catch errors before they affect your data.
- Learn More About Awk: Awk has many features that aren’t covered in this guide. The more you learn about awk, the more effectively you can use it.
Understanding Awk Commands
Awk is not just a command; it’s a programming language in its own right. It’s designed for text processing and typically used as a data extraction and reporting tool. Awk shines when working with structured data, especially when it comes in the form of rows and columns.
Let’s take a closer look at the awk command:
awk '{print $n}' filename
In this command, awk
calls the awk program. The part inside the single quotes {print $n}
is an awk script, which tells awk what to do. The $n
is a field variable, which represents the nth field or column in the current record. filename
is the name of the file awk reads.
Here’s another example:
awk -F: '{print $1}' /etc/passwd
# Output:
# [Expected output from command]
This command prints the first field of each line in the /etc/passwd
file. The -F:
option tells awk to use :
as the field separator.
Awk is part of a broader ecosystem of Unix/Linux commands, all designed to manipulate data in some way. Commands like grep
, sed
, cut
, and sort
each have their own strengths, but awk is particularly powerful when you need to work with structured data.
Unix/Linux commands are built around a philosophy of small, composable tools. Each command does one thing well, and you can combine them to perform complex tasks. This is why learning awk and other Unix/Linux commands can be so powerful: once you understand the basics, you can combine commands in endless ways to manipulate your data.
Practical Usage of Awk
Awk’s ability to manipulate data makes it a valuable tool in larger scripts or projects. It’s not just about printing columns; awk can sort data, perform calculations, and even generate reports. It’s versatile enough to handle a variety of tasks in data processing and analysis.
Integrating Awk in Scripts
Awk can be used in shell scripts to process data files. For instance, you might have a script that uses awk to extract certain columns from a file, then uses other commands to process that data.
Here’s an example of how awk can be used in a script:
#!/bin/bash
# Use awk to extract the second column
awk '{print $2}' data.txt > output.txt
# Use sort to sort the output
sort output.txt > sorted.txt
# Output:
# [Expected output from command]
In this script, awk extracts the second column from ‘data.txt’ and writes it to ‘output.txt’. Then, sort sorts ‘output.txt’ and writes the result to ‘sorted.txt’.
Complementary Unix/Linux Commands
Awk often goes hand in hand with other Unix/Linux commands. Here are a few commands that are often used with awk:
- sort: Sorts lines in text files.
- grep: Searches for patterns in files.
- sed: Edits text in files.
- cut: Removes sections from lines of files.
These commands, combined with awk, form a powerful toolkit for text processing and data manipulation in Unix/Linux.
Further Resources for Mastering Awk
If you want to delve deeper into awk and related topics, here are some resources to check out:
- GNU Awk User’s Guide: This is the official manual for GNU awk, which covers all features of the language.
Unix for Poets: A classic guide to Unix text processing utilities, including awk.
AWK Command in Unix/Linux: Examples – Explore practical examples of using the AWK command in Unix/Linux for text processing and data extraction.
Recap: Column Printing with Awk
In this comprehensive guide, we’ve explored the ins and outs of using awk to print columns in Unix/Linux. We’ve covered everything from the basic usage of awk to more complex data manipulation scenarios, as well as alternative approaches.
We started with the basics, learning how to use awk to print a single column from a file. We then delved into more advanced usage, such as printing multiple columns, manipulating column data, and using conditional statements. We also discussed alternative tools for column printing, such as the cut and Perl commands.
Along the way, we addressed common issues that you might encounter when using awk, providing you with solutions and workarounds. We also provided some best practices and optimization tips to help you use awk more effectively.
Here’s a quick comparison of the methods we’ve discussed:
Method | Versatility | Learning Curve |
---|---|---|
Awk | High | Moderate |
Cut | Low | Low |
Perl | Very High | High |
Whether you’re just starting out with awk or you’re looking to level up your Unix/Linux skills, we hope this guide has given you a deeper understanding of awk and its capabilities.
With its balance of power and flexibility, awk is a valuable tool for data manipulation in Unix/Linux. Now, you’re well equipped to handle any column printing task that comes your way. Happy coding!