Bash String Replacement: Shell Script Stream Editor Guide
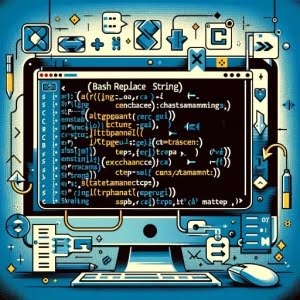
Are you finding it challenging to replace strings in Bash? You’re not alone. Many developers find themselves in a bind when it comes to this task, but Bash can make help with this process.
Think of Bash as a skilled surgeon, capable of performing precise text manipulations and complex text replacements with ease.
This guide will walk you through the process of replacing strings in Bash, from basic to advanced techniques. We’ll cover everything from using the sed
command, handling multiple occurrences, to dealing with special characters and whitespace.
So, let’s dive in and start mastering string replacement in Bash!
TL;DR: How Do I Replace a String in Bash?
You can use the
sed
command to replace a string in Bash. This syntax for this is,echo 'Original String' | sed 's/TargetWord/ReplacementWord'
. This command is a stream editor for filtering and transforming text.
Here’s a simple example:
echo 'Hello, World!' | sed 's/World/Universe/'
# Output:
# 'Hello, Universe!'
In this example, we use the echo
command to print ‘Hello, World!’, then pipe this output into sed
. The sed
command replaces ‘World’ with ‘Universe’, resulting in the output ‘Hello, Universe!’.
This is just a basic way to replace a string in Bash using the
sed
command. But there’s much more to learn about string replacement in Bash, including handling multiple occurrences, dealing with special characters, and more. Continue reading for a more detailed guide on string replacement in Bash.
Table of Contents
- Using the sed Command: A Beginner’s Guide
- Advanced String Replacement in Bash
- Exploring Alternative Methods for String Replacement in Bash
- Troubleshooting String Replacement in Bash
- Bash and String Manipulation: The Fundamentals
- Expanding Your Bash Skills: Beyond String Replacement
- Wrapping Up: Mastering String Replacement in Bash
Using the sed
Command: A Beginner’s Guide
The sed
command, short for ‘stream editor’, is a powerful tool in Bash for text manipulation. It’s particularly useful when you need to replace strings. Let’s delve into the sed
command and how to use it for string replacement in Bash.
A Simple sed
Command Example
Here’s a straightforward example of using the sed
command to replace a string in Bash:
echo 'The sky is blue.' | sed 's/blue/green/'
# Output:
# 'The sky is green.'
In this example, we first use the echo
command to print ‘The sky is blue.’ Then we pipe (|
) this output into the sed
command. The sed
command replaces ‘blue’ with ‘green’, resulting in the output ‘The sky is green.’
Understanding the sed
Command
The sed
command follows the syntax s/old/new/
, where ‘old’ is the string you want to replace, and ‘new’ is the string you want to replace it with. The ‘s’ stands for ‘substitute’.
While the sed
command is incredibly useful, it’s essential to be aware of its limitations. By default, sed
only replaces the first occurrence of the ‘old’ string on each line. If you have multiple occurrences of the ‘old’ string in a line and you want to replace all of them, you’ll need to use a different approach, which we’ll cover in the Intermediate Level section.
In the next section, we’ll delve into more complex string replacements, such as replacing multiple occurrences and using regular expressions. Stay tuned!
Advanced String Replacement in Bash
As your scripting needs become more complex, you might find yourself needing to replace multiple occurrences of a string or even using regular expressions for more advanced pattern matching. Let’s explore these advanced uses of the sed
command.
Replacing Multiple Occurrences
By default, the sed
command only replaces the first occurrence of a string in each line. But what if you want to replace all occurrences of a string? You can do this by adding a ‘g’ (for ‘global’) at the end of the sed
command.
Here’s an example:
echo 'Cats and dogs are pets. I love cats and dogs.' | sed 's/cats/animals/g'
# Output:
# 'Animals and dogs are pets. I love animals and dogs.'
In this code block, we use the ‘g’ option to replace all occurrences of ‘cats’ with ‘animals’. This results in both instances of ‘cats’ being changed to ‘animals’.
Using Regular Expressions with sed
Regular expressions (regex) are powerful tools for matching patterns in text. You can use regex with the sed
command to perform more complex string replacements.
For example, let’s say you want to replace all words that start with ‘a’ in a string. You can do this using regex in the sed
command, like so:
echo 'apple, ant, cat, alligator' | sed 's/a[^ ]*/<replaced>/g'
# Output:
# '<replaced>, <replaced>, cat, <replaced>'
In this example, the regular expression a[^ ]*
matches any word that starts with ‘a’. The sed
command then replaces these words with ”.
These examples showcase the versatility of the sed
command for string replacement in Bash. However, there are even more advanced techniques and alternative commands you can use, which we’ll explore in the next section.
Exploring Alternative Methods for String Replacement in Bash
While sed
is a powerful tool for string replacement, Bash offers other commands like awk
and tr
that can also be used for this purpose. Let’s explore these alternative methods and understand their benefits and potential drawbacks.
String Replacement with awk
awk
is a versatile scripting language designed for text processing. It can also be used for string replacement in Bash.
Here’s an example of how to use awk
to replace a string:
echo 'Hello, World!' | awk '{gsub(/World/, "Universe"); print}'
# Output:
# 'Hello, Universe!'
In this example, the gsub
function in awk
replaces ‘World’ with ‘Universe’. gsub
stands for ‘global substitution’, which means it replaces all occurrences of the specified string.
The benefit of using awk
is that it’s a full-fledged scripting language with powerful text processing capabilities. However, its syntax can be more complex than sed
, especially for beginners.
String Replacement with tr
tr
stands for ‘translate’. It’s a command-line utility in Unix-like operating systems that translates or deletes characters. It can be used to replace single characters in a string.
Here’s an example of how to use tr
to replace a character:
echo 'Hello, World!' | tr 'W' 'U'
# Output:
# 'Hello, Uorld!'
In this example, tr
replaces ‘W’ with ‘U’.
The advantage of tr
is its simplicity, especially for single character replacements. However, it’s not as flexible as awk
or sed
when it comes to complex string replacements or pattern matching.
In conclusion, while sed
is a powerful tool for string replacement in Bash, awk
and tr
offer alternative approaches that might be more suitable depending on your specific needs. It’s important to understand the strengths and weaknesses of each tool to make an informed decision.
Troubleshooting String Replacement in Bash
As with any programming task, you might encounter a few bumps along the way when replacing strings in Bash. Let’s discuss some common issues and their solutions.
Dealing with Special Characters
Special characters like *
, .
, ?
, and others have special meanings in regular expressions. If you want to replace a string that includes these characters, you need to escape them using a backslash (\
).
Here’s an example:
echo 'Hello, World!' | sed 's/\./!/'
# Output:
# 'Hello, World!'
In this example, we replace the period (.
) with an exclamation point (!
). Notice how we use \.
instead of .
in the sed
command.
Handling Whitespace
Whitespace characters like spaces and tabs can also cause issues when replacing strings. To handle whitespace, you can use the [[:space:]]
character class in your sed
command.
Here’s an example:
echo 'Hello, World!' | sed 's/[[:space:]]/
/g'
# Output:
# 'Hello,
World!'
In this example, we replace all spaces with newline characters (). The
[[:space:]]
character class matches any whitespace character.
Remember, troubleshooting is a normal part of the coding process. Don’t get discouraged if you run into issues. Instead, use these challenges as opportunities to learn and improve your Bash scripting skills.
Bash and String Manipulation: The Fundamentals
To effectively use Bash for string replacement, it’s important to understand the fundamentals of Bash and string manipulation. Let’s dive into these concepts.
What is Bash?
Bash, or the ‘Bourne Again SHell’, is a command-line interpreter for Unix-like operating systems. It allows users to interact with the system and run commands. Bash is known for its flexibility and powerful scripting capabilities, which includes string manipulation.
Understanding Strings in Bash
In Bash, a string is a sequence of characters enclosed in quotes. You can manipulate these strings in various ways, such as extracting substrings, concatenating strings, and of course, replacing strings.
Here’s an example of a string in Bash:
echo 'This is a string in Bash.'
# Output:
# 'This is a string in Bash.'
In this example, ‘This is a string in Bash.’ is a string. We use the echo
command to print the string.
Regular Expressions and Pattern Matching
Regular expressions (regex) are a powerful tool for matching patterns in text. They’re used in many programming languages, including Bash. You can use regex to identify and replace specific patterns in a string.
For example, the regex a[^ ]*
matches any word that starts with ‘a’. You can use this regex in the sed
command to replace all words that start with ‘a’, as we saw in the Intermediate Level section.
Understanding these fundamental concepts is key to mastering string replacement in Bash. With this knowledge, you can write more effective Bash scripts and tackle more complex text manipulation tasks.
Expanding Your Bash Skills: Beyond String Replacement
While mastering string replacement is an essential skill in Bash scripting, it’s just the tip of the iceberg. Bash scripting offers a wide array of functionalities that are crucial for tasks like shell scripting and text processing.
Delving into Shell Scripting
Shell scripting is a powerful aspect of Bash that allows you to automate tasks. String replacement is a common operation in shell scripts, especially when dealing with text files or command outputs. Mastering string replacement in Bash will undoubtedly boost your efficiency in shell scripting.
Text Processing in Bash
Bash is a potent tool for text processing. With commands like awk
, sed
, grep
, and cut
, you can manipulate text files in numerous ways. String replacement is a fundamental part of text processing, making it a vital skill to learn.
Exploring Related Concepts
There are numerous related concepts that you can explore to further enhance your Bash scripting skills. For instance, file handling in Bash is a valuable skill that allows you to read from or write to files. Regular expressions, on the other hand, are a powerful tool for pattern matching and text manipulation.
Further Resources for Mastering Bash
To deepen your understanding of Bash and its capabilities, here are some resources you might find useful:
- GNU Bash Manual: This is the official manual for Bash. It provides an in-depth look at Bash and its features.
Advanced Bash-Scripting Guide: This guide goes beyond the basics and delves into more advanced topics in Bash scripting.
Bash Academy: This interactive resource provides a hands-on approach to learning Bash.
By exploring these resources and mastering string replacement in Bash, you’ll be well on your way to becoming a Bash scripting expert.
Wrapping Up: Mastering String Replacement in Bash
In this comprehensive guide, we’ve delved into the process of replacing strings in Bash, exploring the various techniques and commands that make this task possible.
We began with the basics, demonstrating how to use the sed
command to replace strings in Bash. We then moved onto more advanced usage, exploring how to replace multiple occurrences of a string and how to use regular expressions for more complex pattern matching. Along the way, we tackled common issues like handling special characters and whitespace, providing you with solutions to these challenges.
We also explored alternative approaches to string replacement, introducing you to other powerful commands like awk
and tr
. Each of these commands has its strengths and potential drawbacks, and understanding these can help you choose the best tool for your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
sed | Powerful, versatile | Only replaces first occurrence by default |
awk | Full-fledged scripting language | More complex syntax |
tr | Simple, great for single character replacements | Not as flexible for complex string replacements |
Whether you’re just starting out with Bash or you’re looking to level up your scripting skills, we hope this guide has given you a deeper understanding of string replacement in Bash. With these skills in your toolkit, you’re well equipped to tackle a wide range of text manipulation tasks in Bash. Happy scripting!