Print Last Column in AWK | Unix Command Line Guide
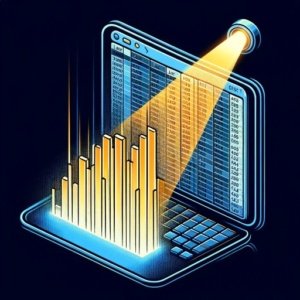
Recently, when developing software at IOFLOOD, we have had the need to consistently access the last column of a data field with AWK. Drawing from our experience, we have found a variety of methods to achieve the desired results. We have crafed today’s aricle with the goal to assist our dedicated hosting customers and fellow developers that may have similar questions.
This guide will walk you through using AWK to print the last column of a file. We’ll explore AWK’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering AWK!
TL;DR: How Do I Print the Last Column with AWK?
To print the last column of a file using AWK, you can use the following command:
awk '{print $NF}' filename
. This command will print the last column of the file named ‘filename’.
Here’s a simple example:
awk '{print $NF}' data.txt
# Output:
# (Expected last column data from data.txt)
In this example, we use the AWK command to print the last column of the file ‘data.txt’. The $NF
in the command represents the last field (or column) in each record (or row) of the file.
This is a basic way to use AWK to print the last column, but there’s much more to learn about AWK’s capabilities. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
Basics of AWK Column Printing
AWK is a powerful programming language designed for text processing. Its name is derived from the surnames of its authors – Alfred Aho, Peter Weinberger, and Brian Kernighan. AWK is particularly useful for column-based data because it automatically splits each line of input into fields.
Using AWK to Print the Last Column
The $NF
variable in AWK represents the last field (or column) of the current record (or row). So, to print the last column of a file, you would use the following command:
awk '{print $NF}' example.txt
# Output:
# (Expected last column data from example.txt)
In this example, ‘example.txt’ is the file we’re reading from. The command awk '{print $NF}' example.txt
prints the last column of each row in the file.
Advantages and Potential Pitfalls
One of the main advantages of using AWK is its ability to handle data of any size, from small text files to large databases. It’s also a versatile tool that can be used for a wide range of tasks beyond just printing the last column of a file.
However, a potential pitfall of using AWK is that it can be a bit complex for beginners. It has its own syntax and can be quite different from other programming languages. But with practice, you can master AWK and use it to perform a variety of data processing tasks.
Remember, AWK is a powerful tool, but like any tool, it’s important to understand how to use it effectively. In the next section, we’ll dive into more complex uses of the AWK command.
Advanced Features with AWK
As you become more comfortable with AWK, you’ll find that it has a lot more to offer. Let’s take a deeper dive into some of the more complex uses of the AWK command.
Using Different Field Separators
AWK uses spaces and tabs as default field separators. But what if your data uses a different field separator, like a comma or a semicolon? You can specify a different field separator using the -F
option.
Here’s an example of how to print the last column of a CSV (Comma Separated Values) file:
awk -F',' '{print $NF}' data.csv
# Output:
# (Expected last column data from data.csv)
In this command, -F','
tells AWK to use a comma as the field separator. It then prints the last column of ‘data.csv’.
Handling Multi-line Records
AWK can also handle multi-line records. By default, AWK treats each line as a separate record. However, you can change the record separator using the RS
variable.
For example, let’s say we have a file where records are separated by a blank line. We can tell AWK to treat each paragraph as a separate record like this:
awk 'BEGIN {RS = ""} {print $NF}' paragraph.txt
# Output:
# (Expected last word from each paragraph in paragraph.txt)
In this command, BEGIN {RS = ""}
tells AWK to use a blank line as the record separator. It then prints the last field (in this case, the last word) of each paragraph in ‘paragraph.txt’.
Best Practices and Differences
While AWK is a powerful tool, it’s important to use it effectively. Remember to specify your field separators if they’re different from the default. Also, be aware of how AWK treats records and fields, and adjust your commands accordingly if you’re dealing with multi-line records.
In the next section, we’ll explore alternative approaches to printing the last column of a file. Stay tuned!
Alternate Text Processing Tools
While AWK is a highly versatile tool, it’s not the only utility at your disposal for processing text data. Two other commands that you can use to print the last column of a file are ‘cut’ and ‘sed’.
Printing the Last Column with ‘cut’
The ‘cut’ command is another text processing utility that you can use to extract and print specific fields from a file. However, unlike AWK, ‘cut’ doesn’t have a built-in way to print the last column of a file. Instead, you need to specify the column number. Here’s an example:
cut -d',' -f3 data.csv
# Output:
# (Expected data from the third column of data.csv)
In this command, -d','
specifies the field separator, and -f3
tells ‘cut’ to print the third field. Remember, ‘cut’ can’t print the last column without knowing the total number of columns.
Printing the Last Column with ‘sed’
The ‘sed’ command, short for ‘stream editor’, is another powerful utility for text processing. While it’s primarily used for text substitution, you can also use it to print the last column of a file. Here’s how:
sed 's/.*,//' data.csv
# Output:
# (Expected last column data from data.csv)
In this command, s/.*,//
tells ‘sed’ to substitute everything up to the last comma with nothing, effectively printing the last column.
Advantages and Disadvantages
While ‘cut’ and ‘sed’ can be used to print the last column, they each have their limitations. ‘cut’ is straightforward and easy to use, but it can’t print the last column without knowing the total number of columns. On the other hand, ‘sed’ is more flexible and can print the last column, but it’s more complex and has a steeper learning curve.
AWK, ‘cut’, and ‘sed’ each have their strengths and weaknesses. Depending on your needs and the specifics of your data, one tool may be more suitable than the others. In the next section, we’ll discuss some common issues you might encounter when using these commands and how to troubleshoot them.
Troubleshooting Tips for AWK
While AWK is a powerful tool, it’s not without its quirks. Here, we’ll discuss some common issues you might encounter when using AWK to print the last column of a file, along with solutions and workarounds.
Dealing with Field Separator Issues
One common issue involves field separators. By default, AWK uses spaces and tabs as field separators. But what if your data uses a different field separator, like a pipe (|
)?
Here’s an example of a potential problem and how to solve it:
# AWK command without specifying the field separator
awk '{print $NF}' data.txt
# Output:
# (Incorrect last column data from data.txt)
# AWK command with the correct field separator
awk -F'|' '{print $NF}' data.txt
# Output:
# (Correct last column data from data.txt)
In the first command, AWK uses the default field separator and prints incorrect data. In the second command, we specify the correct field separator (|
), and AWK prints the correct last column data.
Handling Multi-line Records
Another common issue involves multi-line records. By default, AWK treats each line as a separate record. But what if your data has records that span multiple lines, like paragraphs in a text file?
Here’s an example of how to handle this situation:
# AWK command without handling multi-line records
awk '{print $NF}' paragraph.txt
# Output:
# (Incorrect last word from each line in paragraph.txt)
# AWK command with handling multi-line records
awk 'BEGIN {RS = ""} {print $NF}' paragraph.txt
# Output:
# (Correct last word from each paragraph in paragraph.txt)
In the first command, AWK treats each line as a separate record and prints the last word from each line. In the second command, we tell AWK to treat each paragraph as a separate record, and it prints the correct last word from each paragraph.
Remember, understanding your data and how AWK treats field separators and records is crucial to using AWK effectively. Stay tuned for more AWK insights in the next section.
The Fundamentals of AWK
AWK is a versatile text-processing language, designed for data manipulation and reporting. It’s part of the Unix operating system and is included in most Unix-like systems, such as Linux and macOS.
The Genesis of AWK
AWK was developed in the 1970s at Bell Labs by Alfred Aho, Peter Weinberger, and Brian Kernighan. Its name is an acronym derived from the first letter of each of the developers’ last names.
Understanding AWK’s Role
AWK operates on a line-by-line basis and automatically splits each line into fields. This makes it particularly useful for working with structured data, like tables or databases. It’s often used in data extraction, transformation, reporting, and validation.
Here’s an example of how AWK can be used to calculate the total size of files in a directory:
ls -l | awk '{total += $5} END {print total}'
# Output:
# (Total size of files in the directory)
In this example, ls -l
lists the files in the directory in long format, which includes the file size in bytes (the fifth field). The AWK command then adds up the sizes and prints the total.
AWK in the Broader Context
While AWK is a powerful tool on its own, it’s often used in combination with other Unix commands, like ‘grep’ for searching, ‘sort’ for sorting data, and ‘cut’ and ‘sed’ for data manipulation. This makes AWK an integral part of the Unix philosophy of designing small, simple tools that do one thing well and work well together.
In the next section, we’ll explore how AWK fits into larger scripts and projects, and suggest related commands or functions that often accompany AWK in typical use cases.
Larger Script Usages of AWK
While AWK shines in manipulating column-based data, its potential extends far beyond. In larger scripts or projects, AWK can be a valuable tool for data extraction, transformation, and reporting.
AWK and Shell Scripts
In Unix-like systems, AWK often finds its place within shell scripts, working alongside other commands to perform complex tasks. For instance, you can use AWK in a shell script to process log files, extract specific entries, calculate statistics, and generate reports.
Here’s an example of how AWK can be used in a shell script:
#!/bin/bash
# Print the number of users logged in
echo "Number of users logged in:"
who | awk '{print $1}' | sort | uniq | wc -l
# Output:
# (Number of unique users logged in)
In this script, the who
command lists the logged-in users. The AWK command extracts the usernames, which are then sorted and deduplicated using sort
and uniq
. Finally, wc -l
counts the number of unique users.
AWK and Other Commands
AWK often works in tandem with other Unix commands. For instance, ‘grep’ is commonly used with AWK for searching specific patterns in a file. ‘sort’ and ‘uniq’ are used for sorting and removing duplicates from AWK’s output. The power of AWK is amplified when combined with these other tools.
Further Resources for Mastering AWK
To deepen your understanding of AWK and its applications, here are some resources that you might find helpful:
- The GNU Awk User’s Guide – This is the official manual for GNU AWK. It’s comprehensive and has lots of examples.
Manipulating Data with Grep, Sed, & Awk – Master the basics of data manipulation using powerful scripting tools like Grep, Sed, and Awk.
Effective AWK Programming – This book from O’Reilly offers a more in-depth look at AWK programming.
Remember, mastering AWK, like any other tool, requires practice. So, roll up your sleeves, start writing some AWK scripts, and have fun exploring!
Recap: AWK Print Last Column
In this comprehensive guide, we’ve delved into the world of AWK, a powerful tool for text processing in Unix-like systems. We’ve explored how to use AWK to print the last column of a file, from basic use to advanced techniques.
We began with the basics, understanding the AWK command and its usage to print the last column of a file. We then ventured into more advanced territory, discussing different field separators and handling multi-line records.
Along the way, we tackled common challenges that you might encounter when using AWK, providing solutions and workarounds for each issue. We also explored alternative approaches, such as using ‘cut’ and ‘sed’ commands, and compared their effectiveness.
Here’s a quick comparison of these methods:
Method | Flexibility | Complexity |
---|---|---|
AWK | High | Moderate |
cut | Low | Low |
sed | High | High |
Whether you’re a beginner just starting out with AWK or an experienced user looking to deepen your understanding, we hope this guide has helped you master the AWK command to print the last column of a file.
With its balance of flexibility and power, AWK is a valuable tool in any developer’s toolkit. Now, you’re well equipped to harness its capabilities. Happy coding!