AWS-SDK NPM Package | Quick Start Guide for Node.js
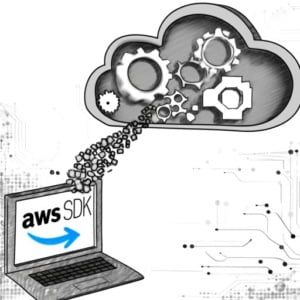
Looking to expand your Node.js Projects with cloud services? AWS, a popular choice, can be tricky to integrate into Node.js, especially when learning to configure the AWS SDK with npm. Well have no fear, as today’s article plans to address these topics and more.
When developing software for IOFLOOD, utilizing the AWS SDK efficiently is essential for scalable applications. To support other cloud developers and the customers utilizing our bare metal cloud servers, we’ve compiled this comprehensive guide on utilizing the AWS SDK with npm.
This guide will walk you through installing, configuring, and effectively using the AWS SDK in your Node.js applications. Whether you’re looking to leverage AWS S3 for storage, invoke AWS Lambda functions, or manage your AWS resources more efficiently, the AWS SDK npm package is an indispensable tool in your development arsenal.
Let’s dive in and unlock the potential of AWS in your Node.js applications!
TL;DR: How Do I Use the AWS SDK with npm for My Node.js Project?
To integrate AWS services into your Node.js project, start by installing the AWS SDK package using npm with the command
npm install aws-sdk
. This sets the stage for leveraging the vast array of AWS services directly from your Node.js application.
Here’s a quick example to illustrate how you can begin:
const AWS = require('aws-sdk');
AWS.config.update({region: 'us-east-1'});
// Example: Create an S3 instance and list buckets
const s3 = new AWS.S3();
s3.listBuckets((err, data) => {
if (err) console.log(err, err.stack);
else console.log(data);
});
# Output:
# { Buckets: [...], Owner: ... }
In this example, we start by requiring the aws-sdk
module and setting the AWS region. Then, we create an instance of the S3 service and use it to list all S3 buckets available under our AWS account. This simple operation demonstrates the ease with which you can start interacting with AWS services using the AWS SDK in your Node.js projects.
Eager to dive deeper into the AWS SDK’s capabilities and discover how to utilize more advanced features? Keep reading for a comprehensive guide that will take your Node.js applications to the next level.
Table of Contents
The Basics: AWS SDK and npm
Embarking on your journey with AWS in a Node.js environment begins with a fundamental step: installing the AWS SDK. The SDK is a treasure trove of tools that allow developers to interact with AWS services seamlessly. Let’s break down the process into manageable steps, ensuring you have everything you need to get started.
Installing the AWS SDK
The first step is as straightforward as it gets. Open your terminal and run the following command:
npm install aws-sdk
This command fetches the AWS SDK package from npm and installs it in your project, making it available for use.
Configuring AWS Credentials
With the AWS SDK installed, the next crucial step is configuring your AWS credentials. This allows your application to authenticate with AWS services.
Create a credentials file at ~/.aws/credentials
on macOS or Linux, or %UserProfile%\.aws\credentials
on Windows, and add the following content:
[default]
aws_access_key_id = YOUR_ACCESS_KEY_ID
aws_secret_access_key = YOUR_SECRET_ACCESS_KEY
Replace YOUR_ACCESS_KEY_ID
and YOUR_SECRET_ACCESS_KEY
with your actual AWS credentials. This file is read by the AWS SDK to authenticate your requests.
Performing Your First AWS Operation
Now that you have the AWS SDK installed and your credentials configured, let’s perform a basic operation: creating an S3 bucket. This example demonstrates how to use the SDK to create a new bucket.
const AWS = require('aws-sdk');
// Set the region
AWS.config.update({region: 'us-east-1'});
// Create S3 service object
const s3 = new AWS.S3({apiVersion: '2006-03-01'});
// Create the parameters for calling createBucket
var bucketParams = {
Bucket : 'my-nodejs-bucket'
};
// Call S3 to create the bucket
s3.createBucket(bucketParams, function(err, data) {
if (err) {
console.log("Error", err);
} else {
console.log("Success", data.Location);
}
});
# Output:
# 'Success' '/my-nodejs-bucket'
In this code snippet, we start by requiring the aws-sdk
module and setting the AWS region. We then create an S3 service object, specifying the API version. The createBucket
method is called with parameters defining the bucket name. Upon successful creation, the location of the new bucket is logged to the console.
This simple yet effective operation marks your first step into managing AWS resources using the AWS SDK in a Node.js project. It’s a demonstration of how straightforward it is to start building with AWS services using the SDK, setting a solid foundation for more complex operations as you advance.
Advanced AWS SDK Techniques
As you grow more comfortable with the basics of AWS SDK in your Node.js applications, it’s time to explore the more advanced features that can significantly enhance your projects. These features not only streamline your work but also open up new avenues for optimizing performance and managing complex AWS services efficiently.
Handling Asynchronous Calls with Promises
One of the most powerful aspects of Node.js is its non-blocking, asynchronous nature, which the AWS SDK supports beautifully through promises. Let’s dive into how you can leverage promises to handle asynchronous AWS service calls.
const AWS = require('aws-sdk');
AWS.config.update({region: 'us-east-1'});
const s3 = new AWS.S3();
async function listS3Buckets() {
try {
const data = await s3.listBuckets().promise();
console.log("Bucket List:", data.Buckets);
} catch (err) {
console.error("Error", err);
}
}
listS3Buckets();
# Output:
# 'Bucket List:' [{ Name: 'bucket1', CreationDate: '2020-01-01' }, { Name: 'bucket2', CreationDate: '2020-02-01' }]
In the above example, we transform the listBuckets
method call into a promise using .promise()
and then await its resolution within an asynchronous function. This approach not only makes the code cleaner but also ensures that you effectively handle the asynchronous nature of network requests to AWS services. It’s a fundamental technique for managing AWS resources in Node.js applications that require precise control over asynchronous operations.
Optimizing Performance with AWS SDK
Performance optimization is crucial in any application, and AWS SDK provides several tools and techniques to help you achieve it. One such technique is configuring the SDK to reuse connections, which can significantly reduce latency and improve the performance of your AWS service interactions.
const AWS = require('aws-sdk');
const https = require('https');
const agent = new https.Agent({
keepAlive: true
});
AWS.config.update({
httpOptions: { agent }
});
This code snippet demonstrates how to create an HTTPS agent that keeps connections alive. By passing this agent to the AWS SDK’s httpOptions
, you’re instructing the SDK to reuse connections for multiple requests, thereby reducing the overhead of establishing a new connection for each request. This optimization can lead to noticeable improvements in the responsiveness of your application, especially when it frequently interacts with AWS services.
Embracing these advanced techniques will not only bolster your Node.js applications but also sharpen your skills as a developer. As you delve deeper into the AWS SDK npm package, remember that the key to mastering its capabilities lies in experimenting with its vast array of features and integrating them into your projects in innovative ways.
Alternatives: Node.js and AWS
When you’ve mastered the AWS SDK for JavaScript, you might find yourself seeking even more control or efficiency for specific scenarios. This is where alternative approaches come into play, offering different methods to interact with AWS services directly from your Node.js applications. Let’s explore these alternatives, their use cases, and how they can complement or substitute the AWS SDK npm usage.
Direct API Calls: HTTP Requests
Sometimes, you might need to interact with AWS services in a way that’s not directly supported by the SDK, or you’re looking for a lighter footprint. Direct API calls using Node.js modules like axios
or the native https
module can be a powerful approach.
const https = require('https');
const options = {
hostname: 's3.amazonaws.com',
port: 443,
path: '/mybucket?max-keys=2',
method: 'GET',
headers: {
'Authorization': 'AWS YOUR_ACCESS_KEY_ID:YOUR_SIGNATURE'
}
};
const req = https.request(options, (res) => {
let responseBody = '';
res.on('data', (chunk) => {
responseBody += chunk;
});
res.on('end', () => {
console.log(JSON.parse(responseBody));
});
});
req.on('error', (e) => {
console.error(`problem with request: ${e.message}`);
});
req.end();
# Output:
# Lists the first two keys (objects) in the specified bucket
This example demonstrates how to make a GET request to the Amazon S3 service to list the first two objects in a bucket. By crafting a custom HTTP request, you gain fine-grained control over the request and response, allowing for precise interaction with AWS services. This method is particularly useful when you need to perform operations that are either not supported by the AWS SDK or when you’re optimizing for performance and resource usage.
Third-Party Libraries: Enhancing Functionality
The Node.js ecosystem is rich with third-party libraries that can enhance or simplify interactions with AWS services. Libraries such as aws-amplify
or serverless
offer higher-level abstractions and additional functionality not found in the AWS SDK.
For instance, aws-amplify
provides a comprehensive suite of tools for building scalable cloud-powered applications, including authentication, analytics, and offline data sync.
AWS CLI: Scripting and Automation
For certain tasks, especially those related to deployment or resource management, the AWS Command Line Interface (CLI) can be a powerful tool. It allows you to script and automate many AWS operations that you would otherwise perform manually.
Integrating the AWS CLI with Node.js can be achieved through child processes. Here’s a simple example of invoking the AWS CLI from within a Node.js application:
const { exec } = require('child_process');
exec('aws s3 ls', (error, stdout, stderr) => {
if (error) {
console.error(`exec error: ${error}`);
return;
}
console.log(`stdout: ${stdout}`);
console.error(`stderr: ${stderr}`);
});
# Output:
# Lists all S3 buckets
This code snippet executes the aws s3 ls
command using the Node.js child_process
module, listing all S3 buckets associated with the configured AWS account. This approach is invaluable for scripting and automation tasks, allowing you to leverage the full power of the AWS CLI within your Node.js applications.
Exploring these alternative approaches can provide you with a more versatile and nuanced toolkit for interacting with AWS services in your Node.js projects. Whether you’re looking for direct control, additional functionality, or automation capabilities, there’s likely an alternative method that suits your needs.
When integrating AWS services into your Node.js projects using the aws-sdk npm package, you’re likely to encounter some common hurdles. Understanding how to troubleshoot these issues is crucial for maintaining a smooth development workflow. Let’s explore some of the typical challenges and how to overcome them.
Credential Configuration Issues
One of the most frequent stumbling blocks involves AWS credentials. Misconfigured or missing credentials can lead to errors that prevent your application from communicating with AWS services.
const AWS = require('aws-sdk');
AWS.config.getCredentials(function(err) {
if (err) console.log(err.stack);
// credentials not loaded
else console.log("Access key:", AWS.config.credentials.accessKeyId);
});
# Output:
# 'Access key: AKIDEXAMPLE'
In this example, we’re checking whether the AWS SDK can successfully retrieve the configured credentials. If there’s an error, it’s logged to the console, indicating that the credentials are not correctly loaded. This simple check can save you time by ensuring your credentials are set up correctly before proceeding with other AWS SDK operations.
Handling SDK Errors Gracefully
Errors can occur during AWS service operations for various reasons, such as network issues or incorrect API usage. Handling these errors gracefully is essential for robust application behavior.
const s3 = new AWS.S3();
s3.listBuckets((err, data) => {
if (err) console.log("Error", err);
else console.log("Success", data.Buckets);
});
# Output:
# 'Error' { code: 'InvalidAccessKeyId', message: 'The AWS Access Key Id you provided does not exist in our records.', ... }
This code snippet demonstrates handling an error when listing S3 buckets. If there’s an error (e.g., an invalid access key ID), it’s logged to the console with a descriptive message. Understanding and logging such errors can help you quickly diagnose and fix issues in your application.
Debugging Tips for Smooth Development
Debugging issues with the AWS SDK can sometimes be challenging due to the asynchronous nature of Node.js and the complexity of AWS services. Here are a few tips:
- Use the AWS SDK logging feature: Enabling logging can provide you with detailed information about the requests made to AWS services and the responses received.
- Simplify your code: Break down complex operations into smaller, manageable pieces. This makes it easier to identify where things are going wrong.
- Check the AWS status page: Sometimes, the issue might not be with your code but with AWS itself. The AWS status page can provide information on any ongoing service disruptions.
By familiarizing yourself with these troubleshooting techniques and considerations, you can navigate the challenges of using the aws-sdk npm package more effectively, ensuring a smoother development experience in your Node.js projects.
AWS SDK and npm: Core Concepts
To effectively leverage the aws-sdk
npm package in your Node.js projects, a solid understanding of its architecture and the role of npm in Node.js development is essential. This knowledge not only aids in utilizing the SDK more efficiently but also in troubleshooting and optimizing your applications.
Understanding the AWS SDK Architecture
The AWS SDK for JavaScript (Node.js) is designed to provide developers with an easy-to-use, consistent, and powerful set of tools for working with AWS services. It abstracts the complexity of directly interacting with AWS’s web services APIs, offering a more intuitive and developer-friendly way to access AWS resources.
const AWS = require('aws-sdk');
AWS.config.update({region: 'us-east-1'});
const ec2 = new AWS.EC2();
ec2.describeInstances({}, function(err, data) {
if (err) console.log(err, err.stack); // an error occurred
else console.log(data); // successful response
});
# Output:
# Displays information about all EC2 instances
In this example, we’re using the aws-sdk
package to interact with Amazon EC2. By calling describeInstances
, we retrieve information about all EC2 instances. This demonstrates the SDK’s ability to simplify complex AWS service operations into manageable, high-level method calls.
npm: The Backbone of Node.js Development
npm, or Node Package Manager, plays a pivotal role in Node.js development. It’s not only a package manager but also a powerful tool for managing dependencies, scripts, and versioning in Node.js projects.
The aws-sdk
npm package is hosted on the npm registry, making it easily accessible to developers. Installing the SDK via npm ensures that you’re working with the latest version, and it handles any dependencies the SDK might have.
npm install aws-sdk
This command installs the AWS SDK into your project, adding it as a dependency in your package.json
file. This simplicity underscores npm’s importance in modern development workflows, facilitating easy integration of external libraries and tools like the AWS SDK.
Understanding the architecture of the AWS SDK and the role of npm in Node.js development provides a strong foundation for building sophisticated, cloud-powered applications. These fundamentals are the first step towards mastering the integration of AWS services into your Node.js projects, paving the way for advanced features and optimizations.
Best Practices for AWS SDK package
As your Node.js applications grow in complexity and scale, integrating AWS services using the AWS SDK npm package becomes an increasingly strategic endeavor. This section explores how to extend the use of AWS SDK beyond basic operations, focusing on structuring projects for scalability, automating deployments, and leveraging AWS for monitoring and management.
Structuring Projects for Microservices
Adopting a microservices architecture can significantly enhance the scalability and maintainability of your Node.js applications. AWS offers a variety of services that support microservices, such as AWS Lambda for serverless functions, Amazon ECS for container management, and Amazon API Gateway for creating RESTful APIs.
const AWS = require('aws-sdk');
const lambda = new AWS.Lambda();
var params = {
FunctionName: 'myFunction',
Payload: JSON.stringify({ key1: 'value1', key2: 'value2', key3: 'value3' })
};
lambda.invoke(params, function(err, data) {
if (err) console.log(err, err.stack); // an error occurred
else console.log('Function result: ', data.Payload); // successful response
});
# Output:
# 'Function result: ' "Your function execution result"
This example demonstrates invoking an AWS Lambda function from a Node.js application using the AWS SDK. By structuring your application to leverage serverless functions, you can build highly scalable and efficient microservices.
Integrating with CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) are essential practices for modern application development. AWS services such as AWS CodeBuild and AWS CodePipeline can be integrated into your Node.js projects to automate the build, test, and deployment processes.
Leveraging AWS Tools for Monitoring and Management
Effective monitoring and management are crucial for maintaining the health and performance of your applications. AWS CloudWatch provides comprehensive monitoring services, allowing you to collect and track metrics, collect and monitor log files, and set alarms.
Further Resources for AWS SDK npm Mastery
To deepen your understanding and skills in using the AWS SDK npm package for Node.js, consider exploring the following resources:
- AWS SDK for JavaScript Developer Guide: A comprehensive guide to using the AWS SDK for JavaScript.
AWS JavaScript in the Node.js Environment: Focuses on the basics of using AWS SDK for JavaScript in a Node.js environment.
Building Serverless Applications with Node.js and AWS Lambda: A tutorial on building serverless applications using AWS Lambda and Node.js.
These resources provide a wealth of information and practical examples to help you effectively leverage the AWS SDK npm package in your Node.js projects, ensuring your applications are scalable, maintainable, and efficiently integrated with AWS services.
Recap: AWS SDK for Node.js
In this comprehensive guide, we’ve navigated the essentials of integrating AWS services into your Node.js projects using the AWS SDK npm package. From the initial setup to advanced features, we’ve covered a broad spectrum to empower your cloud-based applications.
We began with the basics, illustrating how to install the AWS SDK using npm and configure your AWS credentials. This foundational step sets the stage for all subsequent interactions with AWS services from your Node.js environment.
Next, we delved into performing basic AWS operations, such as listing S3 buckets, demonstrating the simplicity and power of the AWS SDK. We then explored more advanced techniques, including handling asynchronous calls with promises and optimizing performance, which are crucial for building scalable and efficient applications.
Our journey also took us through alternative approaches for interacting with AWS services, such as direct API calls, third-party libraries, and the AWS CLI. These methods provide flexibility and control, catering to various development needs and scenarios.
Approach | Flexibility | Control | Use Case |
---|---|---|---|
AWS SDK npm | High | Moderate | General Node.js projects |
Direct API Calls | Moderate | High | Custom solutions |
Third-Party Libraries | High | Moderate | Enhanced functionality |
AWS CLI | Low | High | Scripting and automation |
Whether you’re just starting out with the AWS SDK npm package or looking to enhance your existing Node.js applications, this guide has equipped you with the knowledge to leverage AWS services effectively. The blend of basic and advanced topics ensures a well-rounded understanding, preparing you for the challenges and opportunities of cloud development.
With the AWS SDK npm package, the potential for creating powerful, cloud-enabled Node.js applications is immense. Embrace these capabilities, and continue exploring and experimenting to unlock the full potential of AWS services in your projects. Happy coding!