Python If Statement | Guide to Python Conditionals
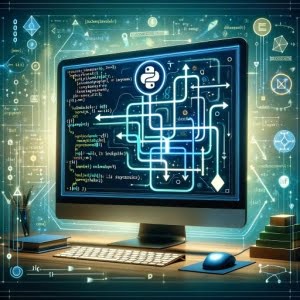
Imagine the Python if statement as a vigilant traffic cop, directing the flow of your code based on certain conditions. It’s a critical component of Python programming, allowing your code to make decisions and carry out different actions depending on various circumstances.
This comprehensive guide will walk you through everything you need to know about the Python if statement, from the basics to advanced usage. Whether you’re a beginner just dipping your toes into the programming world or an intermediate coder looking to solidify your understanding, there’s something in this guide for you.
So, buckle up and get ready to master the Python if statement!
TL;DR: How Do I Use an If Statement in Python?
In Python, you use the if statement to perform conditional operations. It’s a tool that allows your program to make decisions based on certain conditions. Here’s a straightforward example:
x = 10
if x > 5:
print('x is greater than 5')
# Output:
# 'x is greater than 5'
In this example, we’re setting a variable x
equal to 10. Then, we’re using an if statement to check if x
is greater than 5. Since 10 is indeed greater than 5, the condition is true, and the code within the if statement is executed, printing ‘x is greater than 5’ to the console.
This is just the tip of the iceberg when it comes to Python if statements. There’s much more to discover, including advanced usage scenarios and alternative approaches. So, don’t stop here, continue reading for a more detailed guide!
Table of Contents
- Understanding the Python If Statement: A Beginner’s Guide
- Diving Deeper: Logical Operators and Nested If Statements
- Elif and Else: Expanding the Power of If
- Troubleshooting Python If Statements: Common Issues and Solutions
- Unpacking Conditional Statements in Python
- Python If Statements in Real-World Projects
- Further Resources for Python Conditionals
- Python If Statement: The Final Recap
Understanding the Python If Statement: A Beginner’s Guide
The Python if statement is a fundamental building block in programming. It’s a conditional statement that allows code to be executed if certain conditions are met. Let’s break down how it works with a simple example.
y = 15
if y < 20:
print('y is less than 20')
# Output:
# 'y is less than 20'
In this example, we have a variable y
set to 15. The if statement checks if y
is less than 20. Since 15 is indeed less than 20, the condition is true, and the code within the if statement is executed, printing ‘y is less than 20’ to the console.
The basic structure of an if statement in Python is as follows:
if condition:
# code to be executed if the condition is true
The condition
is a test that the if statement performs. If the condition evaluates to True, then the code block under the if statement (indented with four spaces or a tab) is executed. If the condition evaluates to False, then the code block is skipped.
Pitfalls to Watch Out For
One common pitfall for beginners is forgetting the colon (:
) at the end of the if statement. This colon is necessary as it separates the condition from the code block that follows. Without it, you’ll encounter a syntax error.
Another pitfall is incorrect indentation. The code to be executed if the condition is true must be indented correctly. In Python, indentation isn’t just for readability; it’s a part of the syntax. Incorrect indentation can lead to unexpected behavior or errors.
Remember, mastering the Python if statement is a crucial step in your programming journey. Practice using it in different scenarios to solidify your understanding.
Diving Deeper: Logical Operators and Nested If Statements
As you get more comfortable with the Python if statement, you’ll find that it can be used in more complex scenarios. This includes the use of logical operators and nested if statements.
Logical Operators: And, Or, Not
Logical operators allow you to create more complex conditions in your if statements. Python supports three logical operators: and
, or
, and not
.
and
operator: Returns True if both conditions are true.or
operator: Returns True if at least one of the conditions is true.not
operator: Returns the opposite of the given condition.
Let’s see these operators in action with Python if statements:
x = 10
y = 5
if x > 5 and y > 5:
print('Both x and y are greater than 5')
else:
print('At least one of x or y is not greater than 5')
# Output:
# 'At least one of x or y is not greater than 5'
In this example, the and
operator is used in the if statement. The condition checks if both x
and y
are greater than 5. Since y
is not greater than 5, the condition evaluates to False, and the code within the else statement is executed.
Nested If Statements
A nested if statement is an if statement that is contained inside another if statement. It allows for more specific conditions to be checked within broader conditions. Here’s an example:
x = 10
if x > 0:
print('x is positive')
if x > 5:
print('x is also greater than 5')
# Output:
# 'x is positive'
# 'x is also greater than 5'
In this example, the outer if statement checks if x
is greater than 0. Since x
is indeed positive, the code within this if statement is executed. This code includes another if statement (the nested if statement) that checks if x
is also greater than 5. Since x
is 10, this condition is also true, and ‘x is also greater than 5’ is printed to the console.
In conclusion, logical operators and nested if statements can greatly expand the functionality of Python if statements, allowing you to handle more complex conditions and scenarios.
Elif and Else: Expanding the Power of If
Python provides two additional keywords – elif
and else
– that can be used along with the if
statement. These keywords allow for more complex decision-making structures, enhancing the power of your code.
The Elif Statement
The elif
keyword is Python’s way of saying “if the previous conditions were not true, then try this condition”. It’s short for ‘else if’, and can be used to check multiple conditions after the initial if
statement. Let’s see it in action:
x = 20
if x < 10:
print('x is less than 10')
elif x < 20:
print('x is less than 20 but not less than 10')
elif x == 20:
print('x is equal to 20')
# Output:
# 'x is equal to 20'
In this example, the elif
statement allows us to check additional conditions if the original if
condition is not met. Since x
is not less than 10, the code skips to the next elif
statement. As x
is not less than 20 either, it proceeds to the final elif
statement. Here, since x
is indeed equal to 20, ‘x is equal to 20’ is printed to the console.
The Else Statement
The else
keyword catches anything that isn’t caught by the preceding conditions. It’s your last resort, executing a block of code if none of the if
or elif
conditions are met. Here’s an example:
x = 30
if x < 20:
print('x is less than 20')
elif x == 20:
print('x is equal to 20')
else:
print('x is greater than 20')
# Output:
# 'x is greater than 20'
In this example, since x
is neither less than 20 nor equal to 20, both the if
and elif
conditions fail. The code then proceeds to the else
statement, and ‘x is greater than 20’ is printed to the console.
In conclusion, the elif
and else
statements in Python provide additional flexibility when using the if
statement, allowing you to handle a wider range of conditions and make your code more efficient and easier to read.
Troubleshooting Python If Statements: Common Issues and Solutions
Even with the best understanding of Python if statements, you’re bound to run into some common issues. Let’s explore these problems and their solutions, along with some best practices for optimization.
Syntax Errors
One of the most common issues you might encounter is a syntax error. This could be due to forgetting the colon (:
) at the end of the if statement, or incorrect indentation. Remember, Python uses indentation to determine the grouping of statements.
x = 10
if x > 5
print('x is greater than 5')
# Output:
# SyntaxError: invalid syntax
In the above example, we forgot to include the colon at the end of the if statement, resulting in a syntax error. To fix this, simply add a colon at the end of your if statement.
Logical Errors
Another common issue is logical errors, where your code runs without any errors, but it doesn’t produce the expected result. This can often be due to incorrect conditions in your if statement.
x = 10
if x < 5:
print('x is greater than 5')
# Output:
# (No output)
In the above example, we’re checking if x
is less than 5, but then printing that x
is greater than 5. The condition is false, so nothing is printed. To fix this, make sure your conditions match the logic you want to implement.
Optimization Tips
When using Python if statements, it’s a good practice to start with the most likely condition. This makes your code more efficient as Python evaluates conditions in order and stops as soon as it finds a true condition. This can save processing time, especially when dealing with large datasets.
In conclusion, while Python if statements can be a source of common issues for beginners, understanding these issues and their solutions can help you write better, more efficient code.
Unpacking Conditional Statements in Python
In Python, conditional statements are a type of control structure that allow your code to execute different blocks of code based on certain conditions. They form the backbone of any programming language, including Python, and are essential for creating dynamic and responsive programs.
What Are Conditional Statements?
Conditional statements evaluate a specific condition or expression. If the condition is true, a certain block of code is executed. If the condition is false, the code block is skipped, and the program moves on to the next part of the code.
In Python, the primary conditional statements are if
, elif
, and else
. We’ve already explored how these work in the sections above.
The Importance of Conditional Statements
Conditional statements are the building blocks of any non-trivial program. They allow your program to respond differently to different inputs, making it dynamic and flexible.
For example, consider a weather app. It might use a conditional statement to check the current temperature. If the temperature is below freezing, the app displays a warning about icy conditions. If it’s above a certain threshold, it might warn about heatstroke. In each case, the app is using conditional statements to decide what message to display.
temperature = 35 # current temperature
if temperature < 0:
print('Warning: Icy conditions ahead!')
elif temperature > 30:
print('Warning: Risk of heatstroke!')
else:
print('Temperature is in a safe range')
# Output:
# 'Warning: Risk of heatstroke!'
In this example, the app checks the current temperature and displays a warning based on the condition that’s met. This is a simple example, but it demonstrates how conditional statements can be used to create dynamic responses based on different conditions.
In conclusion, understanding conditional statements is crucial for programming in Python. They form the basis for decision-making in your code, allowing your program to react differently to different situations and inputs.
Python If Statements in Real-World Projects
The Python if statement is not just a theoretical concept. It’s a practical tool that you’ll find in almost every Python script or project. From simple scripts to complex machine learning algorithms, the humble if statement is a fundamental part of Python programming.
Consider a real-world project like a web scraper. This tool might use if statements to decide which web pages to scrape based on certain conditions. For example, it might only scrape pages that contain a certain keyword.
# pseudo code
web_pages = ['page1', 'page2', 'page3'] # a list of web pages
keyword = 'Python'
for page in web_pages:
if keyword in page:
# scrape the page
print(f'Scraping {page}')
else:
print(f'Skipping {page}')
# Output:
# 'Scraping page1'
# 'Skipping page2'
# 'Scraping page3'
In this pseudo code example, the scraper checks each page in a list of web pages. If the page contains the keyword ‘Python’, the scraper ‘scrapes’ the page. If not, it skips the page. This is a simple example, but it demonstrates how if statements can be used to control the flow of a larger program.
Further Resources for Python Conditionals
To extend your skills with Python conditionals, the following resources may serve as helpful tools:
- Python’s if-else Statements – Learn about making decisions and creating conditional logic with “if-else” statements in Python.
Python’s “and” Operator – A guide to combining boolean values using “and” to create compound conditional statements.
Tutorial on Conditional Statements by Real Python focuses on the use of conditional (if, elif, else) statements in Python.
The Official Tutorial on Control Flow by Python.org discusses how the walrus operator can be used in conjunction with other control flow tools.
Python ‘if’ Keyword – W3Schools offers a reference guide explaining the use of the ‘if’ keyword in Python.
By integrating these resources into your learning process and further exploring Python conditional statements, you can become a more effective and proficient Python developer.
Python If Statement: The Final Recap
Throughout this guide, we’ve taken a deep dive into the world of Python if statements. From the basic usage to more advanced scenarios, we’ve explored how this fundamental programming tool works and how it can be used effectively.
Here’s a quick recap of what we’ve covered:
- We learned that an if statement in Python tests a specific condition and executes a block of code only if the condition is true.
We delved into more complex uses of the Python if statement, including the use of logical operators and nested if statements.
We explored the
elif
andelse
statements in Python, which can be used in conjunction with theif
statement for more complex decision-making structures.We discussed common issues you might encounter when using Python if statements, along with their solutions and some best practices for optimization.
We also highlighted how Python if statements are used in real-world projects, demonstrating their practical application.
In conclusion, the Python if statement is a versatile and powerful tool in your programming toolbox. Whether you’re a beginner just starting out or an experienced programmer looking to brush up on your skills, understanding the Python if statement and its various uses is a key step in your coding journey.