Using Bash to Count Lines in a File: A File Handling Tutorial
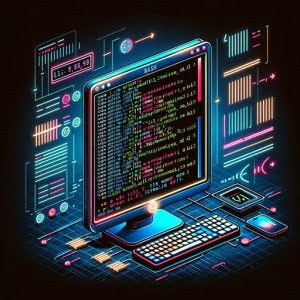
Are you finding it challenging to count lines in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling this task, but we’re here to help.
Think of Bash’s line counting capabilities as a powerful tool – allowing us to quickly tally up lines in a file, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of counting lines in Bash, from the basic ‘wc’ command to more advanced techniques. We’ll cover everything from the basics of line counting to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Count Lines in a File Using Bash?
To count lines in a file using Bash, you can use the
'wc -l'
command, with the syntax,wc -l filename.txt
. This command reads a file and returns the number of lines it contains.
Here’s a simple example:
wc -l filename.txt
# Output:
# 23 filename.txt
In this example, we use the ‘wc -l’ command on a file named ‘filename.txt’. The command returns the number of lines in the file, which in this case is 23.
This is a basic way to count lines in a file using Bash, but there’s much more to learn about this command and other related commands. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Bash Count Lines: The ‘wc -l’ Command
The ‘wc -l’ command is the most common and straightforward way to count lines in a file using Bash. ‘wc’ stands for ‘word count’, but this command can do more than just count words. It can also count lines, characters, and bytes.
The ‘-l’ option tells ‘wc’ to count lines. This is how you use it:
wc -l myfile.txt
# Output:
# 45 myfile.txt
In this example, ‘wc -l’ reads ‘myfile.txt’ and returns the number of lines it contains. In this case, it’s 45.
Advantages of ‘wc -l’
The ‘wc -l’ command is simple and easy to use. It’s built into most Unix-like systems, so you can expect to use it on any Linux distribution, macOS, and other similar systems.
Potential Pitfalls of ‘wc -l’
While ‘wc -l’ is a powerful tool, it’s important to note that it counts newline characters, not lines of text. This means that if a file doesn’t end with a newline character, ‘wc -l’ might give you a count that’s one less than you expect.
Here’s an example to illustrate this point:
echo -n 'Hello, World!' > myfile.txt
wc -l myfile.txt
# Output:
# 0 myfile.txt
In this case, even though ‘myfile.txt’ contains a string, ‘wc -l’ returns 0 because there’s no newline character in the file.
By understanding the basic use of ‘wc -l’, you can easily count lines in a file using Bash. However, there’s much more to learn about this command and other related commands. Continue reading for more advanced usage scenarios.
Advanced Line Counting with Bash
As you become more comfortable with Bash, you’ll find that the ‘wc -l’ command can be combined with other commands for more complex tasks. Let’s explore some intermediate uses of the ‘wc’ command.
Counting Lines with Specific Content
For instance, you might want to count the number of lines in a file that contain a specific word or pattern. You can do this by combining ‘wc -l’ with the ‘grep’ command, which searches for patterns in text.
grep 'pattern' myfile.txt | wc -l
# Output:
# 15
In this example, ‘grep’ searches ‘myfile.txt’ for lines containing ‘pattern’. It sends these lines to ‘wc -l’, which counts them. The result is the number of lines in ‘myfile.txt’ that contain ‘pattern’. In this case, it’s 15.
Counting Lines in Multiple Files
You can also use ‘wc -l’ with the ‘find’ command to count lines in multiple files. The ‘find’ command searches for files in a directory hierarchy. Here’s how you can use ‘find’ and ‘wc -l’ together:
find . -name '*.txt' -exec wc -l {} \;
# Output:
# 45 ./file1.txt
# 30 ./file2.txt
# 25 ./file3.txt
In this example, ‘find’ searches the current directory (.) for files ending in ‘.txt’. For each file it finds, it runs ‘wc -l’ to count the lines. The result is the number of lines in each text file in the current directory.
By combining ‘wc -l’ with ‘grep’ and ‘find’, you can perform more complex line counting tasks in Bash. But that’s not all – there are other commands and techniques you can use to count lines. Let’s explore some of them.
Alternative Methods for Counting Lines in Bash
While ‘wc -l’ is a versatile tool for counting lines in Bash, it’s not the only one. There are other commands and techniques that can accomplish the same task. Let’s explore some of them.
Count Lines with ‘awk’
‘awk’ is a powerful text-processing command in Unix-like systems. It can be used to count lines in a file. Here’s how:
awk 'END { print NR }' myfile.txt
# Output:
# 45
In this example, ‘awk’ reads ‘myfile.txt’. ‘END { print NR }’ tells ‘awk’ to print the number of records (lines) it has read once it reaches the end of the file. The result is the number of lines in ‘myfile.txt’, which in this case is 45.
Count Lines with ‘sed’
‘sed’, which stands for ‘stream editor’, is another command that can count lines in a file. Here’s an example:
sed -n '$=' myfile.txt
# Output:
# 45
In this example, ‘$=’ tells ‘sed’ to print the line number of the last line of ‘myfile.txt’, effectively giving us the total number of lines.
Decision-Making Considerations
While ‘wc -l’, ‘awk’, and ‘sed’ can all count lines in a file, the best command for the job depends on your specific needs. ‘wc -l’ is simple and easy to use, but it counts newline characters, not lines of text. ‘awk’ and ‘sed’ are more complex but also more powerful.
If you’re performing a simple line count, ‘wc -l’ is likely your best bet. If you need to process the text in some way, ‘awk’ or ‘sed’ might be more suitable. The key is to understand how each command works and to choose the right tool for the job.
Troubleshooting Common Bash Line Counting Issues
While counting lines in Bash is generally straightforward, you might encounter some common errors or obstacles. Let’s go over some of these potential issues and how to solve them.
Dealing with Non-Printable Characters
One common issue when counting lines in Bash is dealing with non-printable characters. These can sometimes cause the ‘wc -l’ command to return inaccurate results. Here’s an example:
echo -e 'Hello\x00World' | wc -l
# Output:
# 1
In this case, even though we have a string with a non-printable character (\x00), ‘wc -l’ still counts it as one line.
To handle such scenarios, you can use the ‘tr’ command to delete non-printable characters before counting lines:
echo -e 'Hello\x00World' | tr -d '\000' | wc -l
# Output:
# 1
File Without a Trailing Newline
As we’ve touched on before, ‘wc -l’ counts newline characters, not lines of text. This means that if a file doesn’t end with a newline character, ‘wc -l’ might give you a count that’s one less than you expect.
To ensure accurate line counting, you can add a newline to the end of a file using ‘echo’ or ‘printf’:
echo >> myfile.txt
wc -l myfile.txt
# Output:
# 46 myfile.txt
In this example, we add a newline to ‘myfile.txt’ before counting lines, ensuring an accurate count.
Best Practices and Optimization
When counting lines in Bash, it’s a good practice to understand what each command does and how it works. This will help you choose the right tool for the job and use it effectively.
Also, remember that Bash commands can be combined for more complex tasks. For instance, you can use ‘grep’ with ‘wc -l’ to count lines containing a specific pattern, or ‘find’ with ‘wc -l’ to count lines in multiple files.
Finally, always ensure your files end with a newline to get accurate results from ‘wc -l’. If you’re not sure, you can add a newline with ‘echo’ or ‘printf’ before counting lines.
The ‘wc’ Command: A Deep Dive
The ‘wc’ command, short for ‘word count’, is a staple in the Unix-like operating systems world. It’s a versatile command that can count words, characters, and lines in a file. But how does it work? Let’s take a closer look.
The Mechanics of ‘wc’
The ‘wc’ command reads a file and counts the newline characters (‘\n’), words (sequences of characters separated by whitespace), and bytes. It can also count characters if you use the ‘-m’ option, which is useful for multibyte characters.
Here’s an example:
wc myfile.txt
# Output:
# 45 100 500 myfile.txt
In this example, ‘wc’ reads ‘myfile.txt’ and returns the number of lines, words, and bytes. The numbers represent the count of lines, words, and bytes, respectively.
‘wc’ Options
The ‘wc’ command has several options that modify its behavior:
- ‘-l’: Count lines
- ‘-w’: Count words
- ‘-c’: Count bytes
- ‘-m’: Count characters
You can use these options individually or combine them. For instance, ‘wc -lw’ counts lines and words.
Understanding Lines in Bash
In Bash, a ‘line’ is a sequence of characters that ends with a newline character (‘\n’). This is why ‘wc -l’ counts newline characters, not lines of text. If a file doesn’t end with a newline character, ‘wc -l’ will give you a count that’s one less than you expect.
To ensure accurate line counting, always make sure your files end with a newline. If you’re not sure, you can add a newline with ‘echo’ or ‘printf’ before counting lines.
By understanding the fundamentals of the ‘wc’ command and how Bash handles lines, you can count lines in Bash effectively and accurately.
Expanding Bash Line Counting to Larger Projects
Counting lines in a file is a fundamental task in Bash scripting. However, the ‘wc’ command’s true power shines when you apply it to larger scripts or projects. The ability to count lines, words, or characters can be incredibly useful in many scenarios, such as analyzing log files, parsing data, or tracking codebase changes.
Exploring Related Commands
In addition to ‘wc’, there are several related commands that you might find useful in your Bash scripting journey. These include ‘grep’ for pattern searching, ‘find’ for file searching, and ‘awk’ for text processing.
For instance, you might use ‘grep’ and ‘wc’ together to count the number of lines in a file that match a specific pattern. Here’s an example:
grep 'ERROR' logfile.txt | wc -l
# Output:
# 120
In this code block, ‘grep’ searches ‘logfile.txt’ for lines containing ‘ERROR’, and ‘wc -l’ counts these lines. The result is the number of lines in ‘logfile.txt’ that contain ‘ERROR’, which in this case is 120.
Further Resources for Bash Mastery
To deepen your understanding of Bash scripting and line counting, you might find the following resources helpful:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and detailed, making it an excellent resource for both beginners and experienced users.
Bash Guide for Beginners: This guide provides a gentle introduction to Bash scripting. It covers basic concepts and commands, including ‘wc’.
Advanced Bash-Scripting Guide: This guide delves into more advanced Bash scripting topics. It’s a great resource if you’re ready to take your Bash skills to the next level.
By understanding the basics of line counting in Bash and exploring related commands, you can tackle more complex tasks and projects with confidence.
Wrapping Up: Mastering Line Counting in Bash
In this comprehensive guide, we’ve delved into the nuts and bolts of counting lines in Bash, a fundamental yet powerful task in the world of scripting.
We started with the basics, demonstrating how to use the ‘wc -l’ command to count lines in a file. We then ventured into more advanced territories, exploring how to combine ‘wc -l’ with other commands like ‘grep’ and ‘find’ for more complex tasks. We also touched on alternative approaches like using ‘awk’ and ‘sed’ to accomplish the same task.
Along the way, we tackled common challenges you might encounter when counting lines in Bash, such as dealing with non-printable characters and files without a trailing newline. We provided solutions and best practices to help you overcome these obstacles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘wc -l’ | Simple and easy to use | Counts newline characters, not lines of text |
‘awk’ | Powerful and flexible | More complex than ‘wc -l’ |
‘sed’ | Can handle complex tasks | Might be overkill for simple line counting |
Whether you’re just starting out with Bash or looking to level up your scripting skills, we hope this guide has given you a deeper understanding of line counting in Bash and its applications.
Counting lines in a file is just the tip of the iceberg in Bash scripting. With the knowledge and skills you’ve gained from this guide, you’re well on your way to becoming a Bash scripting pro. Happy scripting!