Pandas concat() Function: Guide to Merging DataFrames
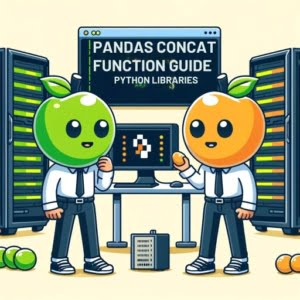
Integrating and combining datasets seamlessly is crucial for data analysis on our servers at IOFLOOD. The pandas concat function facilitates this process by enabling users to concatenate DataFrames in Pandas efficiently. We have formulated today’s article with practical examples and strategies to aid our customers in leveraging pandas concat effectively on their Customizable server solutions.
This comprehensive guide will walk you through the process of using concat
in Pandas, from basic use to advanced techniques. By the end of this article, you’ll be able to wield the concat
function with confidence, merging dataframes efficiently and effectively.
Let’s dive in to manipulating data frames in Panda!
TL;DR: How Do I Concatenate DataFrames in Pandas?
To concatenate dataframes in Pandas, you use the
concat()
function with the syntax,pd.concat([dataframe1, dataframe2])
Here’s a simple example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']})
result = pd.concat([df1, df2])
print(result)
# Output:
# A B
# 0 A0 B0
# 1 A1 B1
# 0 A2 B2
# 1 A3 B3
In this example, we created two dataframes, df1
and df2
, each with columns ‘A’ and ‘B’. We then used the concat()
function to merge these two dataframes. The resulting dataframe, result
, is a combination of df1
and df2
.
For a more detailed understanding and advanced usage scenarios, continue reading this guide.
Table of Contents
Understanding the Basics of concat()
The concat()
function in Pandas is a straightforward yet powerful method for combining two or more dataframes. At its simplest, it takes a list of dataframes and appends them along a particular axis (either rows or columns), creating a single dataframe.
Let’s look at an example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']})
result = pd.concat([df1, df2])
print(result)
# Output:
# A B
# 0 A0 B0
# 1 A1 B1
# 0 A2 B2
# 1 A3 B3
In this example, we’ve created two dataframes, df1
and df2
, each with two rows and two columns. The concat()
function takes these dataframes as a list ([df1, df2]
) and merges them. The result is a new dataframe that combines the rows of df1
and df2
.
While the concat()
function is a handy tool for dataframe merging, it’s important to be aware of its potential pitfalls. One common issue is the handling of indexes. In our example, the resulting dataframe maintains the original indexes of df1
and df2
, which may not be desirable in all cases. We’ll delve into how to manage this and other advanced features in the upcoming sections.
Leveraging Parameters in concat()
The concat()
function in Pandas offers a range of parameters that allow for more control over how dataframes are merged. Three of the most commonly used parameters are ‘axis’, ‘join’, and ‘keys’. Let’s explore each of these in detail.
Adjusting the Axis
The ‘axis’ parameter determines whether the dataframes are concatenated along the row axis (0) or the column axis (1). By default, concat()
merges dataframes vertically (row-wise). Here’s how to use ‘axis’ to concatenate dataframes horizontally (column-wise):
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'C': ['C0', 'C1'], 'D': ['D0', 'D1']})
result = pd.concat([df1, df2], axis=1)
print(result)
# Output:
# A B C D
# 0 A0 B0 C0 D0
# 1 A1 B1 C1 D1
In this example, df1
and df2
are merged side-by-side, resulting in a dataframe with four columns (‘A’, ‘B’, ‘C’, and ‘D’).
Choosing the Join Method
The ‘join’ parameter dictates how concat()
handles the merging of dataframes with non-matching indexes or columns. By default, it uses an ‘outer’ join, which includes all indexes or columns even if they don’t match. However, you can set ‘join’ to ‘inner’ to only include matching indexes or columns. Here’s an example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'B': ['B2', 'B3'], 'C': ['C2', 'C3']})
result = pd.concat([df1, df2], join='inner')
print(result)
# Output:
# B
# 0 B0
# 1 B1
# 0 B2
# 1 B3
In this case, only the ‘B’ column, which is present in both dataframes, is included in the result.
Using Keys for Hierarchical Indexing
The ‘keys’ parameter allows you to create a hierarchical index, which can be useful for tracking the original dataframes. Here’s how to use ‘keys’ in concat()
:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']})
result = pd.concat([df1, df2], keys=['df1', 'df2'])
print(result)
# Output:
# A B
# df1 0 A0 B0
# 1 A1 B1
# df2 0 A2 B2
# 1 A3 B3
In the output, ‘df1’ and ‘df2’ are used as keys to indicate the origin of each row.
Understanding and leveraging these parameters can greatly enhance your use of the concat()
function, providing you with more control over how your dataframes are merged.
Alternative Concatenation Approaches
While the concat()
function is a powerful tool for merging dataframes, Pandas provides other functions that offer different ways to combine dataframes. Two of these are the merge()
and join()
functions.
Merging DataFrames with merge()
The merge()
function combines dataframes based on a common column. Here’s an example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A1', 'A2'], 'C': ['C1', 'C2']})
result = df1.merge(df2, on='A', how='inner')
print(result)
# Output:
# A B C
# 0 A1 B1 C1
In this example, df1
and df2
are merged based on the common ‘A’ column. The ‘how’ parameter is set to ‘inner’, which means only the matching rows are included in the result.
Joining DataFrames with join()
The join()
function combines dataframes based on their indexes. Here’s an example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']}, index=['a', 'b'])
df2 = pd.DataFrame({'C': ['C0', 'C1'], 'D': ['D0', 'D1']}, index=['a', 'b'])
result = df1.join(df2)
print(result)
# Output:
# A B C D
# a A0 B0 C0 D0
# b A1 B1 C1 D1
In this case, df1
and df2
are joined based on their indexes, resulting in a dataframe that includes all columns from both dataframes.
Each of these functions has its advantages and disadvantages. The concat()
function is versatile and can handle simple concatenations quickly and efficiently. The merge()
function is powerful when you need to combine dataframes based on a common column, while the join()
function is ideal when you want to combine dataframes based on their indexes.
Function | Advantages | Disadvantages |
---|---|---|
concat() | Versatile, handles simple concatenations | May require additional steps to handle complex merges |
merge() | Powerful for merging on common columns | Can be complex to use for new users |
join() | Ideal for merging on indexes | Limited to index-based merging |
In conclusion, the method you choose for concatenating dataframes depends on your specific needs and the nature of your data. It’s beneficial to familiarize yourself with all these functions to enhance your data manipulation skills in Pandas.
Handling Errors with Pandas concat()
While concat()
is a powerful function, it’s not without its quirks. Let’s discuss some common issues you may encounter while using it, along with their solutions and workarounds.
Dealing with ‘ValueError’
One common issue is the ‘ValueError’, which often arises when trying to concatenate dataframes with different column names. Here’s an example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'C': ['C0', 'C1'], 'D': ['D0', 'D1']})
try:
result = pd.concat([df1, df2])
except ValueError as e:
print(e)
# Output:
# Frames have different column names
In this case, the solution is to ensure that the dataframes share the same column names or to use the ignore_index=True
parameter to reset the index in the resulting dataframe.
Handling Different Indexes
Another common issue is dealing with dataframes that have different indexes. If you concatenate such dataframes without specifying the ‘axis’ parameter, the resulting dataframe may not be what you expect. Here’s an example:
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']}, index=['a', 'b'])
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']}, index=['c', 'd'])
result = pd.concat([df1, df2])
print(result)
# Output:
# A B
# a A0 B0
# b A1 B1
# c A2 B2
# d A3 B3
In this case, the resulting dataframe maintains the original indexes from df1
and df2
, which may not be desirable. To avoid this, you can use the ignore_index=True
parameter to reset the index in the resulting dataframe.
Understanding these common issues and their solutions can help you to use the concat()
function more effectively and avoid potential pitfalls.
Understanding Pandas DataFrames
Before diving deeper into the concat()
function, it’s important to understand the basics of Pandas DataFrames and the concept of concatenation.
What is a DataFrame?
In Pandas, a DataFrame is a two-dimensional labeled data structure with columns potentially of different types. You can think of it like a spreadsheet or an SQL table. Here’s a simple example of a DataFrame:
import pandas as pd
df = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
print(df)
# Output:
# A B
# 0 A0 B0
# 1 A1 B1
This DataFrame, df
, has two columns (‘A’ and ‘B’) and two rows. Each cell contains a string.
What is Concatenation?
Concatenation is the process of joining two or more things end-to-end. In the context of dataframes, concatenation refers to the joining of two or more dataframes along a particular axis (either rows or columns).
df1 = pd.DataFrame({'A': ['A0', 'A1'], 'B': ['B0', 'B1']})
df2 = pd.DataFrame({'A': ['A2', 'A3'], 'B': ['B2', 'B3']})
result = pd.concat([df1, df2])
print(result)
# Output:
# A B
# 0 A0 B0
# 1 A1 B1
# 0 A2 B2
# 1 A3 B3
In this example, df1
and df2
are concatenated along the row axis (the default). The resulting dataframe, result
, includes all rows from both df1
and df2
.
By understanding Pandas DataFrames and the concept of concatenation, you can better comprehend the workings of the concat()
function and use it more effectively.
Real-World Data Tasks: Using concat()
DataFrame concatenation using concat()
is not just a technical skill, it’s a critical tool in the world of data analysis and machine learning. The ability to merge and manipulate dataframes effectively can significantly impact the quality of your data analysis and the accuracy of your machine learning models.
Consider a scenario where you’re working with a large dataset that’s been split across multiple CSV files. Using concat()
, you can easily merge these files into a single DataFrame for analysis. Or perhaps you’re doing feature engineering for a machine learning model, and you need to combine several features into a single DataFrame. Again, concat()
comes to the rescue.
# Combining multiple CSV files into a single DataFrame
df1 = pd.read_csv('file1.csv')
df2 = pd.read_csv('file2.csv')
df = pd.concat([df1, df2])
# Combining features for a machine learning model
feature1 = pd.DataFrame({'feature1': [0, 1, 2, 3]})
feature2 = pd.DataFrame({'feature2': [4, 5, 6, 7]})
features = pd.concat([feature1, feature2], axis=1)
In both of these examples, concat()
simplifies the process of combining data, making your data analysis or machine learning workflow smoother and more efficient.
Beyond dataframe concatenation, there are many other important concepts in data analysis and machine learning to explore, such as data cleaning, data visualization, and model tuning. Each of these topics is a deep well of knowledge in its own right, and mastering them can greatly enhance your data science skills.
If you’re interested in diving deeper into these topics, there are numerous resources available online. Websites like Kaggle, Coursera, and Medium offer a wealth of tutorials, courses, and articles on a wide range of data science topics. By continuing to learn and expand your skillset, you’ll be well on your way to becoming a proficient data scientist.
Further Resources for Pandas Library
If you’re interested in learning more ways to utilize the Pandas library, here are a few resources that you might find helpful:
- Data Analysis Made Simple: A Pandas Tutorial: Simplify your approach to data analysis with this easy-to-follow Pandas tutorial, ideal for beginners and intermediate users alike.
Reading CSV Files with Pandas: This article provides a comprehensive guide on how to read CSV files using Pandas in Python.
Resetting the Index of a Pandas DataFrame: This tutorial explains how to reset the index of a Pandas DataFrame.
pandas.concat() – pandas API Reference: Official documentation for the concat() function in Pandas, providing information on how to concatenate DataFrames.
Pandas concat() Function in Python: An article on GeeksforGeeks explaining how to use the concat() function in Pandas to combine DataFrames.
Pandas concat(): Joining DataFrames: A tutorial on DigitalOcean that demonstrates various examples of using the concat() function in Pandas.
Wrap Up: concat()
function in Pandas
Throughout this guide, we’ve explored the ins and outs of the concat()
function in Pandas, a powerful tool for merging dataframes. We started with the basics, demonstrating how to use concat()
to join two simple dataframes. We then dove into more advanced usage, discussing parameters like ‘axis’, ‘join’, and ‘keys’ that provide more control over the concatenation process.
Along the way, we highlighted some common issues that you might encounter when using concat()
, such as ‘ValueError’ and problems with different indexes. We provided solutions and workarounds for these issues, equipping you with the knowledge to handle any hiccups in your dataframe merging journey.
We also discussed alternative methods for concatenating dataframes, including the merge()
and join()
functions. Each of these methods has its own strengths and weaknesses, and the best one to use depends on your specific needs and the nature of your data.
Here’s a comparison table of the discussed methods for a quick recap:
Function | Advantages | Disadvantages |
---|---|---|
concat() | Versatile, handles simple concatenations | Requires additional steps for complex merges |
merge() | Powerful for merging on common columns | Can be complex for new users |
join() | Ideal for merging on indexes | Limited to index-based merging |
In conclusion, mastering dataframe concatenation in Pandas, represented by the concat()
function, is a valuable skill in data analysis and machine learning. By understanding and effectively using this function, you can manipulate your dataframes with ease, enhancing the quality of your data analysis and the accuracy of your machine learning models.