Pandas DataFrame Mastery: A Detailed Guide
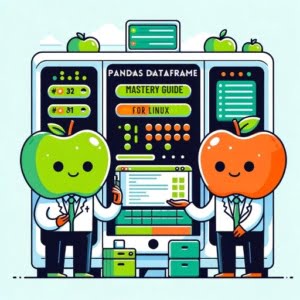
Creating and manipulating data structures is essential for data analysis on our servers at IOFLOOD. The pandas dataframe class offers a powerful tool for creating and working with tabular data. Join us as we explore how to create a Pandas DataFrame, providing step-by-step guidance and practical examples for our customers developing on their dedicated server.
This guide is designed to help you understand and master the use of pandas DataFrames. We’ll start from the basics and gradually move to more advanced manipulation techniques. Whether you’re just starting out with data manipulation in Python or looking to enhance your skills, this guide has got you covered.
So, let’s dive in and start our journey towards pandas DataFrame mastery.
TL;DR: How Do I Create a Pandas DataFrame?
To create a pandas
DataFrame
, you must first instantiate a dictionary of sample data objects, and then convert it with the syntax,df = pd.DataFrame(sampleData)
.
Here’s a simple example:
import pandas as pd
data = {'Name': ['John', 'Anna'], 'Age': [28, 24]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
In this example, we first import the pandas library as pd. We then create a dictionary with two keys, ‘Name’ and ‘Age’, each associated with a list of values. This dictionary is passed to the pd.DataFrame()
function to create a DataFrame. The print(df)
command then displays the DataFrame, with ‘Name’ and ‘Age’ as column headers and the corresponding values in the rows.
This is just the tip of the iceberg when it comes to pandas DataFrames. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Introduction to Pandas DataFrame
Pandas DataFrame is a two-dimensional labeled data structure, which can hold data of different types (like integers, strings, floating point numbers, Python objects, etc.) in columns. It’s similar to a spreadsheet or SQL table, or a dictionary of Series objects. DataFrame is generally the most commonly used pandas object.
Creating a Pandas DataFrame
Creating a DataFrame in pandas is as simple as creating a dictionary and passing it to the pd.DataFrame()
function. Here’s a basic example:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 35]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
# 2 Peter 35
In this example, the DataFrame df
has two columns, ‘Name’ and ‘Age’, each populated with the corresponding values from the dictionary data
. The index of the DataFrame is automatically assigned as integers from 0 to N-1, where N is the number of rows.
Adding, Deleting, and Modifying DataFrame Columns
Once you have a DataFrame, you can add, delete, and modify its columns. Here’s how:
# Adding a new column
ndf['Profession'] = ['Engineer', 'Doctor', 'Artist']
# Deleting a column
ndf = df.drop('Age', axis=1)
# Modifying a column
ndf['Name'] = ndf['Name'].str.upper()
In this example, we first add a new column ‘Profession’ to the DataFrame. The drop()
function is then used to delete the ‘Age’ column (note the axis=1
parameter indicating a column). Finally, we modify the ‘Name’ column to convert all names to uppercase.
Pandas DataFrame is a versatile and powerful tool for data manipulation. However, it’s important to be aware of potential pitfalls, like making sure your data types are consistent and handling missing or null values appropriately. These topics and more will be covered in the following sections.
Advanced Use of Pandas DataFrame
As you get more comfortable with pandas DataFrame, you’ll start to encounter situations that require more complex manipulations. These could involve merging or joining multiple DataFrames, reshaping or pivoting a DataFrame, among others. Let’s dive into some of these advanced operations.
Merging and Joining DataFrames
Merging is the process of combining two or more DataFrames based on a common column (or set of columns), similar to the JOIN operation in SQL.
# Creating two DataFrames
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2'],
'key': ['K0', 'K1', 'K2']})
df2 = pd.DataFrame({'C': ['C0', 'C1', 'C2'],
'D': ['D0', 'D1', 'D2'],
'key': ['K0', 'K1', 'K2']})
# Merging the DataFrames
merged = pd.merge(df1, df2, on='key')
print(merged)
# Output:
# A B key C D
# 0 A0 B0 K0 C0 D0
# 1 A1 B1 K1 C1 D1
# 2 A2 B2 K2 C2 D2
In this example, we merge df1
and df2
on the common column ‘key’. The resulting DataFrame merged
contains the combined data from df1
and df2
.
Reshaping and Pivoting DataFrames
Reshaping is the process of changing the structure (i.e., the number of rows and columns) of the DataFrame to make it suitable for further analysis. Pivoting is a specific kind of reshaping where we turn the unique values of a column into new columns in the DataFrame.
# Creating a DataFrame
df = pd.DataFrame({'date': ['2020-01-01', '2020-01-02', '2020-01-03']*2,
'city': ['New York', 'New York', 'New York', 'Chicago', 'Chicago', 'Chicago'],
'temp': [32, 30, 31, 20, 21, 23],
'humidity': [80, 85, 90, 70, 75, 80]})
# Pivoting the DataFrame
pivoted = df.pivot(index='date', columns='city')
print(pivoted)
# Output:
# temp humidity
# city Chicago New York Chicago New York
# date
# 2020-01-01 20 32 70 80
# 2020-01-02 21 30 75 85
# 2020-01-03 23 31 80 90
In this example, we pivot the DataFrame df
on the ‘date’ column, with the unique values in ‘city’ as new columns. The resulting DataFrame pivoted
has a multi-index column and shows the ‘temp’ and ‘humidity’ values for each ‘city’ on each ‘date’.
These are just a few examples of the advanced manipulations you can perform with pandas DataFrames. As with any tool, the key to mastering pandas DataFrame is practice. Try these operations on your own datasets and see what you can discover!
Alternative Tools for Data Manipulation
While pandas DataFrame is a powerful tool for data manipulation in Python, it’s not the only one. Other methods and libraries can also be used to manipulate data, each with its own strengths and weaknesses. Let’s explore some of these alternatives.
NumPy Arrays
NumPy, which stands for ‘Numerical Python’, is another library in Python that provides support for arrays. NumPy arrays are used for storing and manipulation of numerical data.
import numpy as np
# Creating a NumPy array
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Output:
# [1 2 3 4 5]
In this example, we create a one-dimensional NumPy array. NumPy arrays are faster and more compact than Python lists and are better for mathematical operations.
SQL Databases
SQL databases can be used for data manipulation in Python through libraries like sqlite3 or SQLAlchemy. SQL databases provide robust and scalable options for data storage and manipulation.
import sqlite3
# Connecting to a SQLite database
conn = sqlite3.connect('example.db')
# Creating a table
conn.execute('''CREATE TABLE stocks
(date text, trans text, symbol text, qty real, price real)''')
# Inserting data into the table
conn.execute("INSERT INTO stocks VALUES ('2006-01-05','BUY','RHAT',100,35.14)")
# Committing the changes and closing the connection
conn.commit()
conn.close()
# Output:
# (No output, but a new SQLite database 'example.db' is created with a table 'stocks' and one row of data)
In this example, we create a SQLite database, define a table ‘stocks’, and insert a row of data into the table. SQL databases are powerful tools for data manipulation, especially for large datasets.
Third-Party Libraries
There are also several third-party libraries in Python for data manipulation, like Dask and Vaex. These libraries are designed to work with large datasets, even those that don’t fit in memory.
Each of these methods has its own advantages and disadvantages, and the best one to use depends on the specific requirements of your project. For small to medium-sized datasets, pandas DataFrame is often the most convenient option. For larger datasets or for more complex mathematical operations, you might want to consider NumPy, SQL databases, or third-party libraries.
Method | Advantages | Disadvantages |
---|---|---|
Pandas DataFrame | Easy to use, powerful, flexible | Can be slow with large datasets |
NumPy Arrays | Fast, compact, good for mathematical operations | Less flexible, only for numerical data |
SQL Databases | Robust, scalable, good for large datasets | More complex, requires knowledge of SQL |
Third-Party Libraries | Can handle very large datasets | Can be more complex, may require additional installation |
Remember, the best tool is the one that suits your needs. Don’t be afraid to experiment and find the one that works best for you!
Errors and Solutions for DataFrames
As you work with pandas DataFrame, you may encounter a few common issues. These can range from data type mismatches to dealing with missing data. Let’s discuss these problems and their solutions.
Data Type Mismatches
Data type mismatches can occur when you’re trying to perform an operation that’s not compatible with the data type of a DataFrame column.
# Creating a DataFrame with mixed data types
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 'three']})
# Attempting to perform a numerical operation
try:
df['A'] = df['A'] + 1
except Exception as e:
print(f'Error: {e}')
# Output:
# Error: can only concatenate str (not "int") to str
In this example, we try to add 1 to each value in column ‘A’, but encounter an error because one of the values is a string. To solve this issue, we can convert the data type of the column to numeric using the pd.to_numeric()
function, which can handle errors by either raising, ignoring, or coercing them.
Handling Missing Data
Missing data is a common issue in data analysis. Pandas represents missing values as NaN
(Not a Number). You can handle missing data in several ways, such as ignoring it, removing it, or filling it with a specific value or a computed value (like mean, median, etc.).
# Creating a DataFrame with missing values
import numpy as np
df = pd.DataFrame({'A': [1, np.nan, 3]})
# Filling missing values with the mean of the column
df['A'].fillna(df['A'].mean(), inplace=True)
print(df)
# Output:
# A
# 0 1.0
# 1 2.0
# 2 3.0
In this example, we fill the missing value in column ‘A’ with the mean of the non-missing values in the same column.
Remember, the best way to handle these issues depends on the specific requirements of your project. Always consider the implications of your choices on your data analysis.
Key Concepts of Pandas DataFrames
To effectively use pandas DataFrame, it’s important to understand the basics of the pandas library and the DataFrame object, as well as the underlying data structures that they rely on.
The Pandas Library
Pandas is a powerful, open-source data analysis and manipulation library for Python. It provides data structures and functions needed to manipulate structured data, including functions for reading and writing data in a variety of formats.
import pandas as pd
In this line of code, we import the pandas library and use ‘pd’ as an alias. This is a common convention in the Python community and it allows us to access pandas functions using the prefix ‘pd’.
The DataFrame Object
The DataFrame is one of the main data structures in pandas. It’s a two-dimensional table of data with rows and columns. Each column in a DataFrame is a Series object, and rows consist of elements inside Series.
# Creating a simple DataFrame
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 35]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
# 2 Peter 35
In this example, we create a DataFrame from a dictionary. The keys of the dictionary become the column labels and the values become the data of the DataFrame.
Indexing and Data Types
Each row and column in a DataFrame has an index. By default, pandas assigns integer labels to the rows, which start from 0 and increment by 1 for each row. DataFrames can contain data of different types: integers, floats, strings, Python objects, etc.
# Displaying the index and data types of the DataFrame
print(df.index)
print(df.dtypes)
# Output:
# RangeIndex(start=0, stop=3, step=1)
# Name object
# Age int64
# dtype: object
In this example, we display the index and data types of the DataFrame. The index is a RangeIndex
object that starts at 0 and stops at 3 (exclusive), stepping by 1. The data types of the columns are displayed with the dtypes
attribute: ‘Name’ is of type ‘object’ (which typically means ‘string’ in pandas), and ‘Age’ is of type ‘int64’.
Understanding these basics will help you effectively manipulate data using pandas DataFrames.
Practical Uses of Pandas DataFrames
Pandas DataFrame is not just a tool for data manipulation. Its relevance extends to a wide range of areas in data analysis, machine learning, and more. Let’s explore how you can leverage the power of pandas DataFrame beyond the basics.
Pandas DataFrame in Data Analysis
Data analysis involves inspecting, cleaning, transforming, and modeling data to discover useful information and support decision-making. Pandas DataFrame provides a host of functionalities that make these tasks easier.
# Descriptive statistics with pandas DataFrame
import pandas as pd
# Assuming df is a pandas DataFrame
df.describe()
# Output:
# (Summary statistics for numerical columns in the DataFrame)
In this example, we use the describe()
function to generate descriptive statistics that summarize the central tendency, dispersion, and shape of a dataset’s distribution.
Pandas DataFrame in Machine Learning
Machine learning involves training a model using data, so that it can make predictions or decisions without being explicitly programmed. Pandas DataFrame is often used to preprocess data before it’s fed into a machine learning algorithm.
# Splitting a DataFrame into features and labels
# Assuming 'label' is the column we want to predict
features = df.drop('label', axis=1)
labels = df['label']
In this example, we split a DataFrame into features (the input) and labels (the output), which can then be used to train a machine learning model.
Further Resources for Mastering Pandas DataFrame
If you’re looking to further your understanding of pandas DataFrame, here are some resources that you might find helpful:
- Leveraging Pandas for Efficient Data Analysis: Discover how to leverage Pandas for more efficient data analysis, with tips and strategies outlined in this resourceful guide.
Grouping and Aggregating Data in Pandas using the groupby() Function: This tutorial explores how to use the groupby() function in Pandas to group data and perform aggregation operations on a DataFrame in Python.
Creating an Empty DataFrame in Pandas: This guide provides examples and explanations of different ways to create an empty DataFrame in Pandas, giving you a head start when initializing your data structure.
Pandas Documentation: The official documentation is always a great place to start. It’s comprehensive and includes plenty of examples.
Python for Data Analysis: This book by Wes McKinney, the creator of pandas, provides an in-depth introduction to using pandas for data analysis.
DataCamp’s Pandas Tutorial: This tutorial provides a practical introduction to pandas DataFrame with real-world examples.
Recap: Mastering Pandas DataFrames
Throughout this guide, we’ve taken a deep dive into the world of pandas DataFrames
. We started with the basics, learning how to create a DataFrame and manipulate its columns. We then moved on to more advanced topics, exploring complex manipulations like merging, joining, reshaping, and pivoting DataFrames.
We also discussed common issues you might encounter when working with DataFrames, such as data type mismatches and handling missing data, and provided solutions for each. The key is to understand your data and the specific requirements of your project. With practice, troubleshooting these issues becomes second nature.
Beyond pandas DataFrames
, we also explored alternative methods for data manipulation in Python. These include NumPy arrays
, SQL databases
, and third-party libraries
. Each method has its own strengths and weaknesses. The best one to use depends on your specific needs and the size and complexity of your dataset.
Method | Best Used For |
---|---|
Pandas DataFrame | Small to medium-sized datasets, complex data manipulations |
NumPy Arrays | Numerical operations, large datasets |
SQL Databases | Large datasets, robust and scalable solutions |
Third-Party Libraries | Very large datasets, out-of-memory computations |
In conclusion, pandas DataFrames
offer a powerful and flexible tool for data manipulation in Python. Whether you’re a beginner just starting out or an expert looking to hone your skills, mastering pandas DataFrames
can significantly enhance your data analysis capabilities and open up new opportunities for exploration and discovery.