Numpy: Python’s Math Powerhouse | Ultimate Guide
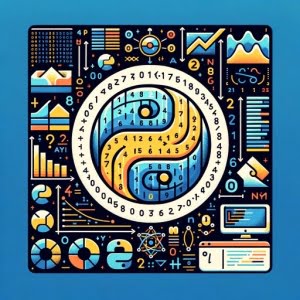
Ever wondered how Python handles complex mathematical operations? The secret lies in a powerful library known as Numpy. This numerical computation tool is Python’s answer to the need for high-performance mathematical, scientific, and engineering functionalities.
In this guide, we’ll delve into the basics of Numpy, exploring its uses and how you can get started. Whether you’re a data scientist, a machine learning enthusiast, or a Python developer looking to dive into numerical computations, this article is your stepping stone into the world of Numpy.
TL;DR: What is Numpy and How Do I Use It?
Numpy is a Python library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It’s a fundamental tool for scientific computing with Python, enabling you to perform complex mathematical operations with ease.
Here’s a simple example of how to use Numpy to create an array and perform a mathematical operation:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(np.sum(arr))
# Output:
# 15
In this example, we first import the Numpy library using the alias ‘np’. We then create a one-dimensional array with the values 1 through 5. The np.sum(arr)
function is used to calculate the sum of all elements in the array, which in this case outputs 15.
This is just a glimpse of the power of Numpy. Keep reading to learn more about its capabilities and how you can leverage them in your Python projects.
Table of Contents
- Exploring Numpy: Creating Arrays and Performing Basic Operations
- Numpy for Linear Algebra and Statistics
- Numpy vs. Pandas vs. SciPy: A Comparative Analysis
- Navigating Common Numpy Pitfalls
- Numpy’s Building Blocks: Arrays and Matrices
- Expanding Horizons: Numpy and Other Libraries
- Further Resources for Numpy Mastery
- Wrapping Up: The Power of Numpy
Exploring Numpy: Creating Arrays and Performing Basic Operations
Numpy’s core functionality revolves around its powerful n-dimensional array object, ndarray. Let’s dive into how you can create arrays using Numpy and perform basic operations on them.
Creating Arrays with Numpy
To create a Numpy array, you can use the np.array()
function and pass in a list of values. Here’s an example:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Output:
# array([1, 2, 3, 4, 5])
In this example, we’ve created a one-dimensional array with five elements. The print()
function outputs the array, where you can see the values we’ve inputted.
Basic Operations with Numpy Arrays
One of the benefits of using Numpy arrays is that you can perform mathematical operations on an element-wise basis. This means you can add, subtract, multiply, and divide the elements of two arrays without having to write any loops. Here’s how you can do it:
import numpy as np
arr1 = np.array([1, 2, 3, 4, 5])
arr2 = np.array([6, 7, 8, 9, 10])
# Addition
print(arr1 + arr2)
# Output:
# array([ 7, 9, 11, 13, 15])
# Subtraction
print(arr1 - arr2)
# Output:
# array([-5, -5, -5, -5, -5])
# Multiplication
print(arr1 * arr2)
# Output:
# array([ 6, 14, 24, 36, 50])
# Division
print(arr1 / arr2)
# Output:
# array([0.16666667, 0.28571429, 0.375 , 0.44444444, 0.5 ])
In this example, we’ve performed addition, subtraction, multiplication, and division on the arr1
and arr2
arrays. The operations are performed element-wise, which means the first elements of arr1
and arr2
are operated on together, then the second elements, and so on.
Common Numpy Functions
Numpy comes with a host of built-in functions to make your numerical computations even easier. Here are a few examples:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
# Sum of all elements in the array
print(np.sum(arr))
# Output:
# 15
# Mean of the array
print(np.mean(arr))
# Output:
# 3.0
# Maximum value in the array
print(np.max(arr))
# Output:
# 5
# Minimum value in the array
print(np.min(arr))
# Output:
# 1
In this example, we’ve used the np.sum()
, np.mean()
, np.max()
, and np.min()
functions to calculate the sum, mean, maximum, and minimum of the array, respectively. These are just a few examples of the many functions that Numpy provides to make your life easier when dealing with numerical data in Python.
Numpy for Linear Algebra and Statistics
Numpy isn’t just for creating arrays and performing basic operations. It also has powerful capabilities for more advanced mathematical operations, such as linear algebra and statistics. Let’s explore some of these features.
Linear Algebra with Numpy
Numpy provides a suite of functions in the numpy.linalg
module for linear algebra operations. This includes functions to calculate the determinant, solve linear equations, find eigenvalues and eigenvectors, and much more. Here’s an example of how you can use Numpy to solve a system of linear equations:
import numpy as np
# Coefficients of the equations
a = np.array([[3, 1], [1, 2]])
# Constants on the right side of the equations
b = np.array([9, 8])
# Solve the system of equations
x = np.linalg.solve(a, b)
print(x)
# Output:
# array([2., 3.])
In this example, we have a system of two linear equations: 3x + y = 9
and x + 2y = 8
. We represent the coefficients of the equations in a 2D array a
and the constants on the right side in the array b
. We then use the np.linalg.solve()
function to solve this system of equations, which gives us the solution [2., 3.]
.
Statistical Operations with Numpy
Numpy also provides a range of functions for statistical operations. You can calculate the mean, median, standard deviation, variance, and much more. Here’s an example of how you can use Numpy to calculate some basic statistics on an array of numbers:
import numpy as np
# An array of numbers
arr = np.array([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
# Calculate mean
print(np.mean(arr))
# Output:
# 5.5
# Calculate median
print(np.median(arr))
# Output:
# 5.5
# Calculate standard deviation
print(np.std(arr))
# Output:
# 2.8722813232690143
In this example, we have an array of numbers from 1 to 10. We then use the np.mean()
, np.median()
, and np.std()
functions to calculate the mean, median, and standard deviation of this array, respectively. These functions provide a quick and easy way to perform statistical operations on your data with Numpy.
Numpy vs. Pandas vs. SciPy: A Comparative Analysis
While Numpy is a powerful library for numerical computations, it’s not the only tool available in Python’s data science toolkit. Two other libraries, pandas and SciPy, also offer robust functionalities for data analysis and scientific computing. Let’s compare these three libraries and discuss when to use each one.
Numpy vs. Pandas
Pandas is a library built specifically for data manipulation and analysis. It’s built on top of Numpy, which means it uses Numpy arrays to store data. However, pandas provides more advanced data manipulation capabilities than Numpy. For example, pandas allows you to create DataFrames, which are like tables with labeled rows and columns. Here’s an example of how you can create a DataFrame using pandas:
import pandas as pd
# Creating a DataFrame
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 22]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
# 2 Peter 22
In this example, we’ve created a DataFrame with two columns, ‘Name’ and ‘Age’. Each row represents a different person. This kind of data structure is more intuitive for handling real-world data compared to Numpy arrays.
However, Numpy is generally faster than pandas for numerical computations. So if you’re working with numerical data and need to perform mathematical operations, Numpy might be the better choice.
Numpy vs. SciPy
SciPy is another library built on top of Numpy. It’s designed for scientific and technical computing, and it extends the capabilities of Numpy with more advanced functions for linear algebra, optimization, integration, interpolation, and other scientific computing tasks.
Here’s an example of how you can use SciPy to calculate the integral of a function:
from scipy import integrate
# Define the function
f = lambda x: x**2
# Calculate the integral of the function from 0 to 1
result, error = integrate.quad(f, 0, 1)
print(result)
# Output:
# 0.33333333333333337
In this example, we’ve used the integrate.quad()
function from SciPy to calculate the integral of the function f(x) = x^2
from 0 to 1. This kind of operation is not available in Numpy.
In summary, while Numpy is a powerful library for numerical computations, pandas and SciPy provide more advanced functionalities for data manipulation and scientific computing. Depending on the task at hand, you might find one library more suitable than the others.
As with any powerful tool, using Numpy can sometimes present challenges, especially for beginners. Let’s discuss some common issues that you might encounter when using Numpy and how to solve them.
Dealing with Multidimensional Arrays
One common issue when working with Numpy is dealing with multidimensional arrays. The dimensions of an array are defined by its shape, which is a tuple of N positive integers that specify the sizes of each dimension. Here’s an example of a common error you might encounter when working with multidimensional arrays:
import numpy as np
# Create a 2D array with shape (2, 3)
arr = np.array([[1, 2, 3], [4, 5, 6]])
# Try to access an element with an invalid index
try:
print(arr[2, 3])
except IndexError as e:
print(f'IndexError: {e}')
# Output:
# IndexError: index 2 is out of bounds for axis 0 with size 2
In this example, we’ve tried to access the element at index [2, 3]
of a 2D array with shape (2, 3)
. However, since the indices in Python start at 0, the valid indices for this array are [0, 0]
to [1, 2]
. The index [2, 3]
is out of bounds, resulting in an IndexError
.
To avoid this error, always make sure that your indices are within the bounds of the array.
Understanding Data Types
Another common issue in Numpy is dealing with different data types. Numpy arrays can contain different data types, such as integers, floats, and strings. However, all elements in a Numpy array must be of the same data type. Here’s an example of a common error you might encounter when dealing with data types:
import numpy as np
# Try to create an array with mixed data types
try:
arr = np.array([1, 'two', 3.0])
except ValueError as e:
print(f'ValueError: {e}')
# Output:
# No error, but the resulting array has a dtype of '<U21'
print(arr)
# Output:
# array(['1', 'two', '3.0'], dtype='<U21')
In this example, we’ve tried to create a Numpy array with mixed data types: an integer, a string, and a float. However, since all elements in a Numpy array must be of the same data type, Numpy automatically converts all elements to strings, resulting in an array with a data type of <U21
, which represents a string of length 21 or less.
To avoid issues with data types, always make sure that all elements in your Numpy array are of the same data type. If you need to store elements of different data types, consider using a structured array or a pandas DataFrame.
Numpy’s Building Blocks: Arrays and Matrices
At the heart of Numpy’s functionality are arrays and matrices. These fundamental data structures are key to understanding how Numpy operates and why it’s so powerful in data analysis and machine learning.
Understanding Numpy Arrays
A Numpy array, also known as an ndarray, is a grid of values, all of the same type, and is indexed by a tuple of non-negative integers. The number of dimensions is the rank of the array, and the shape of an array is a tuple of integers giving the size of the array along each dimension.
Here’s an example of a one-dimensional Numpy array:
import numpy as np
# Create a one-dimensional Numpy array
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Output:
# array([1, 2, 3, 4, 5])
In this example, we’ve created a one-dimensional array with five elements. The print()
function outputs the array, where you can see the values we’ve inputted.
Matrices in Numpy
A matrix is a special kind of two-dimensional array where each data element is of the same size. So you can think of a matrix as an array of arrays. Here’s an example of a 2×3 matrix in Numpy:
import numpy as np
# Create a 2x3 Numpy matrix
matrix = np.array([[1, 2, 3], [4, 5, 6]])
print(matrix)
# Output:
# array([[1, 2, 3],
# [4, 5, 6]])
In this example, the matrix
variable holds a 2D Numpy array, or matrix, with two rows and three columns.
The Power of Arrays and Matrices in Data Analysis and Machine Learning
Arrays and matrices are powerful tools because they allow you to perform operations on entire sets of data all at once. This is much more efficient than performing operations on individual data elements one at a time.
In the context of data analysis and machine learning, arrays and matrices are essential because they allow you to organize and manipulate data in a structured way. For instance, a common use case in machine learning is to represent a dataset as a 2D array (or matrix), where each row represents a different sample (or observation), and each column represents a different feature.
By using Numpy’s arrays and matrices, you can perform complex mathematical operations on your data with just a few lines of code. This makes Numpy a must-have tool for any data scientist or machine learning practitioner.
Expanding Horizons: Numpy and Other Libraries
Numpy, while powerful on its own, can be combined with other Python libraries to perform even more complex data analysis and machine learning tasks. Let’s explore how Numpy can be used in conjunction with other libraries for these advanced applications.
Numpy and Matplotlib for Data Visualization
Matplotlib is a popular Python library for creating static, animated, and interactive visualizations. Numpy arrays can be used as input data for these visualizations. Here’s an example of how you can use Numpy and Matplotlib to plot a sine wave:
import numpy as np
import matplotlib.pyplot as plt
# Create an array of x values from 0 to 2*pi
x = np.linspace(0, 2*np.pi, 100)
# Create an array of y values representing the sine of x
y = np.sin(x)
# Create a plot using matplotlib
plt.plot(x, y)
plt.show()
# Output:
# A plot of a sine wave
In this example, we first create an array of x values from 0 to 2*pi using the np.linspace()
function. We then create an array of y values representing the sine of x using the np.sin()
function. Finally, we create a plot of y vs. x using Matplotlib’s plt.plot()
function and display it using plt.show()
.
Numpy and Scikit-Learn for Machine Learning
Scikit-learn is a Python library for machine learning that provides simple and efficient tools for data mining and data analysis. It’s built on top of Numpy, and many of its functions require Numpy arrays as input. Here’s an example of how you can use Numpy and scikit-learn to train a linear regression model:
import numpy as np
from sklearn.linear_model import LinearRegression
# Create an array of x values and reshape it to the format expected by scikit-learn
x = np.array([1, 2, 3, 4, 5]).reshape((-1, 1))
# Create an array of y values
y = np.array([2, 4, 6, 8, 10])
# Create a linear regression model
model = LinearRegression()
# Train the model
model.fit(x, y)
# Make a prediction
y_pred = model.predict([[6]])
print(y_pred)
# Output:
# array([12.])
In this example, we first create arrays of x and y values representing our data. We then create a LinearRegression model and train it on our data using the model.fit()
function. Finally, we use the trained model to make a prediction on a new x value using the model.predict()
function.
Further Resources for Numpy Mastery
For a deeper understanding of Numpy and how it integrates with other Python libraries, consider checking out the following resources:
- numpy.append() Function: Appending Arrays in Python – Learn how to use the “numpy append” function to add elements to an existing NumPy array.
Official Numpy Documentation – The official documentation of Numpy providing a comprehensive overview and specifications of the module.
Python Data Science Handbook – A book by O’Reilly Media dedicated to data science in Python, including extensive coverage of Numpy.
Coursera Python Numpy Courses – A selection of courses offered by Coursera specifically focusing on Python’s Numpy module.
edX Numpy Learning Resources – A collection of learning materials and courses on Numpy offered by edX.
Wrapping Up: The Power of Numpy
In this comprehensive guide, we’ve explored the power of numpy
, Python’s numerical powerhouse. From creating simple arrays to performing complex mathematical operations, numpy
is a versatile tool that supercharges Python’s capabilities for scientific computing.
We started with the basics, learning how to create numpy
arrays and perform elementary operations. We then delved into more advanced uses, exploring how numpy
can be used for linear algebra and statistics. We also compared numpy
with other Python libraries like pandas
and SciPy
, highlighting when and why you might choose one over the other.
Here’s a quick comparison of these three libraries:
Numpy | Pandas | SciPy | |
---|---|---|---|
Purpose | Numerical computations | Data manipulation and analysis | Scientific computing |
Key Features | Powerful n-dimensional arrays, mathematical functions | DataFrames, data cleaning functions | Advanced mathematical functions, integration with Numpy |
Best For | Mathematical operations on arrays | Handling and analyzing structured data | More advanced mathematical computations |
Remember, the best tool depends on your specific needs. While numpy
is widely useful for mathematical computations, pandas
excels in data manipulation and SciPy
offers more advanced scientific computing capabilities.
Whether you’re a data scientist, a machine learning enthusiast, or a Python developer, mastering numpy
can be a game-changer. We hope this guide has given you a solid foundation to start harnessing the power of numpy
in your own projects.