Numpy Append Function: From Basics to Advanced Usage
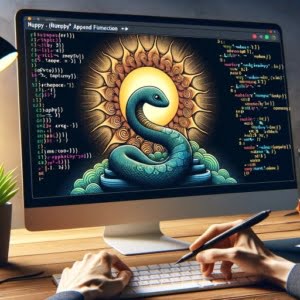
Are you grappling with appending data in Numpy arrays? Just like adding a new item at the end of a list in Python, the ‘append’ function in Numpy offers you the ability to attach new elements to an existing array.
This guide is designed to take you on a journey from the rudimentary to the more sophisticated usage of the ‘append’ function in Numpy.
Whether you’re a novice just dipping your toes in the pool of Numpy or a seasoned user looking to refine your skills, this guide has got you covered.
TL;DR: How Do I Use the Numpy Append Function?
To append data to a Numpy array, you use the ‘numpy.append()’ function. Here’s a simple example:
import numpy as np
arr = np.array([1, 2, 3])
new_arr = np.append(arr, [4, 5, 6])
print(new_arr)
# Output:
# array([1, 2, 3, 4, 5, 6])
In this example, we’ve created a Numpy array arr
with elements 1, 2, and 3. We then use the ‘numpy.append()’ function to add the elements 4, 5, and 6 to the end of the array, resulting in a new array new_arr
that contains all six elements.
For a deeper dive into the ‘append’ function, including its advanced usage and alternative approaches, continue reading.
Table of Contents
- Understanding Numpy Append: Basic Usage
- Numpy Append: Handling Different Shapes and Dimensions
- Exploring Alternatives to Numpy Append
- Troubleshooting Common Issues with Numpy Append
- Deep Dive into Numpy Arrays and the Append Operation
- The Role of Numpy Append in Data Manipulation
- Wrapping Up: Numpy Append Function in a Nutshell
Understanding Numpy Append: Basic Usage
The ‘numpy.append()’ function is a fundamental tool in the Numpy library, designed to add new elements to an existing Numpy array. It’s a simple, yet powerful tool that can significantly streamline your data manipulation tasks.
Let’s explore a basic usage of the ‘numpy.append()’ function with a simple example:
import numpy as np
arr = np.array([1, 2, 3])
new_arr = np.append(arr, [4, 5, 6])
print(new_arr)
# Output:
# array([1, 2, 3, 4, 5, 6])
In this example, we start with a Numpy array arr
containing the elements 1, 2, and 3. We then use the ‘numpy.append()’ function to add the elements 4, 5, and 6 to the end of arr
. The result is a new array new_arr
that contains all six elements.
One key advantage of the ‘numpy.append()’ function is its simplicity and straightforwardness. However, it’s important to note that the function creates a new array and does not modify the original array. This can potentially lead to memory issues if you’re working with large arrays and performing numerous append operations.
Numpy Append: Handling Different Shapes and Dimensions
As you become more proficient with Numpy, you’ll likely encounter situations where you need to append arrays of different shapes or dimensions. This is where understanding the ‘axis’ parameter in the ‘numpy.append()’ function becomes crucial.
Let’s look at an example where we append a 2D array to another 2D array along the second axis (axis = 1):
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
new_arr = np.append(arr1, arr2, axis=1)
print(new_arr)
# Output:
# array([[1, 2, 5, 6],
# [3, 4, 7, 8]])
In this example, we have two 2D arrays arr1
and arr2
. We append arr2
to arr1
along the second axis (axis=1). The result is a new 2D array new_arr
where the rows of arr2
are appended to the rows of arr1
.
It’s important to note that when using the ‘axis’ parameter, the arrays must have the same shape along all but the specified axis. Otherwise, a ValueError will be raised.
Understanding how to use the ‘axis’ parameter effectively can greatly expand your data manipulation capabilities with Numpy. However, always be mindful of the shapes of your arrays to avoid unexpected errors.
Exploring Alternatives to Numpy Append
While the ‘numpy.append()’ function is a powerful tool, it’s not the only method available for appending data to Numpy arrays. Two notable alternatives are the ‘numpy.concatenate()’ and ‘numpy.hstack()’ functions.
Using ‘numpy.concatenate()’
The ‘numpy.concatenate()’ function can also be used to append data to a Numpy array. This function requires a tuple or list of arrays as its first argument, allowing you to concatenate along an existing axis.
Let’s look at an example:
import numpy as np
arr1 = np.array([1, 2, 3])
arr2 = np.array([4, 5, 6])
new_arr = np.concatenate((arr1, arr2))
print(new_arr)
# Output:
# array([1, 2, 3, 4, 5, 6])
In this example, we’ve concatenated arr1
and arr2
along the default axis (axis=0). The ‘numpy.concatenate()’ function is more flexible than ‘numpy.append()’ as it can handle multiple array inputs at once.
Using ‘numpy.hstack()’
The ‘numpy.hstack()’ function is another alternative. This function horizontally stacks arrays, effectively appending arrays along the second axis.
Let’s see how it works:
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([[5, 6], [7, 8]])
new_arr = np.hstack((arr1, arr2))
print(new_arr)
# Output:
# array([[1, 2, 5, 6],
# [3, 4, 7, 8]])
In this example, ‘numpy.hstack()’ horizontally stacks arr1
and arr2
, resulting in a new array new_arr
.
While these alternatives offer more flexibility, they can be slightly more complex to use than ‘numpy.append()’. The best method to use will depend on your specific use case and familiarity with these functions.
Troubleshooting Common Issues with Numpy Append
Like any function, using ‘numpy.append()’ can sometimes lead to unexpected issues. Understanding these potential pitfalls can help you troubleshoot and find solutions more effectively.
Shape Mismatch
One common issue is a shape mismatch. This typically occurs when you’re trying to append arrays of different shapes along a specific axis.
import numpy as np
arr1 = np.array([[1, 2], [3, 4]])
arr2 = np.array([5, 6])
try:
new_arr = np.append(arr1, arr2, axis=1)
except ValueError as e:
print(e)
# Output:
# all the input arrays must have same number of dimensions, but the array at index 0 has 2 dimension(s) and the array at index 1 has 1 dimension(s)
In this example, we tried to append a 1D array arr2
to a 2D array arr1
along the second axis. This results in a ValueError because the arrays have different dimensions.
The solution to this issue is to ensure that the arrays you’re appending have the same shape along all but the specified axis.
Type Mismatch
Another common issue is a type mismatch. This can occur when you’re trying to append arrays of different data types.
import numpy as np
arr1 = np.array([1, 2, 3])
arr2 = np.array(['4', '5', '6'])
new_arr = np.append(arr1, arr2)
print(new_arr)
# Output:
# array(['1', '2', '3', '4', '5', '6'], dtype='<U21')
In this example, we appended a string array arr2
to an integer array arr1
. The resulting array new_arr
contains all string elements, as Numpy converts all elements to strings to avoid a type mismatch.
It’s important to be aware of these potential issues when using the ‘numpy.append()’ function. Being mindful of the shapes and data types of your arrays can save you from unexpected results and errors.
Deep Dive into Numpy Arrays and the Append Operation
Before we delve further into the ‘numpy.append()’ function, it’s important to understand the fundamental concepts underlying this operation. This includes understanding Numpy arrays and their properties, as well as the ‘axis’ parameter and how Numpy handles different dimensions.
Numpy Arrays: A Quick Recap
Numpy arrays, also known as ‘ndarrays’, are the heart of the Numpy library. These arrays are homogeneous multidimensional containers of items of the same type and size. This means that all elements in a Numpy array have the same data type.
import numpy as np
arr = np.array([1, 2, 3])
print(arr)
print(type(arr))
# Output:
# array([1, 2, 3])
# <class 'numpy.ndarray'>
In this example, we create a Numpy array arr
with the elements 1, 2, and 3. When we print arr
, we see that it’s an instance of the ‘numpy.ndarray’ class.
Understanding the ‘Axis’ Parameter
In Numpy, the ‘axis’ parameter refers to the dimension of the array. For a 1D array, there’s only one axis (axis=0). For a 2D array, there are two axes: axis=0 (rows) and axis=1 (columns).
When you use the ‘numpy.append()’ function, the ‘axis’ parameter defines along which axis the arrays will be appended. If no axis is specified, the arrays will be flattened before the append operation.
Understanding these foundational concepts is key to mastering the ‘numpy.append()’ function and effectively manipulating data with Numpy.
The Role of Numpy Append in Data Manipulation
Appending data using Numpy doesn’t exist in isolation. It’s a fundamental operation that plays a crucial role in data manipulation and analysis. Whether you’re preprocessing data for machine learning algorithms or analyzing large datasets, the ‘numpy.append()’ function and its alternatives can significantly streamline your workflow.
Exploring Related Concepts
Once you’ve mastered the ‘numpy.append()’ function, there are many related concepts worth exploring. These include slicing, which allows you to extract specific portions of a Numpy array, and reshaping, which enables you to change the dimensions of your array.
import numpy as np
arr = np.array([1, 2, 3, 4, 5, 6])
# Slicing
print(arr[1:4])
# Output:
# array([2, 3, 4])
# Reshaping
reshaped_arr = arr.reshape((2, 3))
print(reshaped_arr)
# Output:
# array([[1, 2, 3],
# [4, 5, 6]])
In the first example, we slice the array arr
to extract the elements at indices 1, 2, and 3. In the second example, we reshape arr
into a 2D array with 2 rows and 3 columns.
Expanding Your Numpy Knowledge
The world of Numpy extends far beyond the ‘append’ function. There are numerous resources available online, including this blog post that provides full documentation on Numpy in Python, including how to install NumPy and set up your Python environment for scientific computing
To advance your knowledge and skills with Numpy, we have compiled an additional set of resources that you may find beneficial:
- Using np.where() in Python – A comprehensive guide on the “np.where” function and its role in conditional element selection in NumPy arrays.
Efficient Array Concatenation with NumPy – A practical approach on using “numpy concatenate” to create larger arrays from smaller ones.
Official Numpy Documentation – The official documentation of Numpy offering a detailed overview of the module and its functionalities.
Numpy Tutorial – A comprehensive tutorial from TutorialsPoint covering various aspects of Numpy and its practical applications in Python.
Numpy Guide on the official Python Wiki page for Numpy, providing an overview of its features and functionalities.
By diving deeper into Numpy and incorporating these resources into your learning journey, you will enhance your proficiency and capacity as a Python developer!
Wrapping Up: Numpy Append Function in a Nutshell
We’ve covered a lot of ground on our journey to mastering the ‘append’ function in Numpy. From the basics of adding elements to a Numpy array to handling different shapes and dimensions, we’ve delved deep into the mechanics of this essential function.
The ‘numpy.append()’ function allows you to add new elements to an existing Numpy array. It’s a straightforward tool, but it does create a new array and does not modify the original one, which could lead to memory issues if you’re working with large arrays and performing numerous append operations.
import numpy as np
arr = np.array([1, 2, 3])
new_arr = np.append(arr, [4, 5, 6])
print(new_arr)
# Output:
# array([1, 2, 3, 4, 5, 6])
We also explored how to append arrays of different shapes and dimensions by using the ‘axis’ parameter. Remember, when using the ‘axis’ parameter, the arrays must have the same shape along all but the specified axis.
Additionally, we discussed alternative methods to append data to Numpy arrays, such as ‘numpy.concatenate()’ and ‘numpy.hstack()’. These alternatives provide more flexibility but can be slightly more complex to use.
We highlighted common issues you might encounter when using ‘numpy.append()’, such as shape mismatch and type mismatch, and provided solutions and workarounds for these problems.
Mastering the ‘numpy.append()’ function is a significant step towards becoming proficient in data manipulation with Numpy. With this knowledge in hand, you’re well-equipped to handle a wide range of data manipulation tasks.
We encourage you to continue exploring Numpy and its many functions. There’s always more to learn in the world of data analysis and manipulation.