Numpy Concatenate: Mastering Array Joining in Python
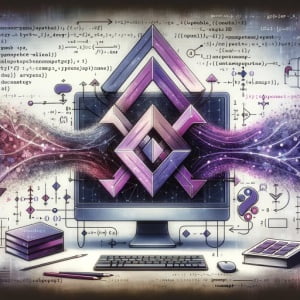
Are you struggling to join arrays in Python? Much like a master chef combining ingredients, numpy’s concatenate function can seamlessly blend your arrays. This guide is designed to help you navigate the intricacies of numpy concatenate, from basic usage to advanced techniques.
We’ll walk you through the process step by step, providing practical examples and clear explanations. Whether you’re a beginner or an experienced programmer, you’ll find valuable insights into how numpy concatenate works and how to use it effectively.
Stay tuned as we dive into the world of array joining in Python with numpy concatenate.
TL;DR: How Do I Concatenate Arrays in Numpy?
To concatenate arrays in numpy, you use the
numpy.concatenate()
function. Here’s a simple example:
import numpy as np
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
result = np.concatenate((array1, array2))
print(result)
# Output:
# array([1, 2, 3, 4, 5, 6])
In this example, we have two arrays, array1
and array2
. We use the numpy.concatenate()
function to join these two arrays end-to-end, resulting in a new array that includes all elements from both input arrays in their original order. The resulting array, result
, is then printed to the console.
This is just the tip of the iceberg. Continue reading for a deeper understanding and more advanced usage scenarios of numpy concatenate.
Table of Contents
- Understanding Numpy Concatenate: Basic Use
- Numpy Concatenate with Multidimensional Arrays
- Exploring Alternative Methods for Array Concatenation
- Troubleshooting Numpy Concatenate: Common Issues and Solutions
- Understanding Numpy’s Array Data Type and Axes Concept
- The Impact of Array Concatenation Beyond Coding
- Exploring Related Concepts
- Wrapping Up: Numpy Concatenate Unveiled
Understanding Numpy Concatenate: Basic Use
The numpy.concatenate()
function is a powerful tool in Python, especially when working with arrays. It allows you to join two or more arrays along an existing axis.
Let’s take a look at a basic example:
import numpy as np
# Define two one-dimensional arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Use numpy concatenate to join the arrays
result = np.concatenate((array1, array2))
print(result)
# Output:
# array([1, 2, 3, 4, 5, 6])
In this example, numpy.concatenate()
takes a tuple or list of arrays as its first argument. We passed in array1
and array2
as a tuple to the function. The function then returns a new array that contains all elements from array1
and array2
in the order they were input.
Advantages of Using Numpy Concatenate
The numpy.concatenate()
function is highly efficient when it comes to memory usage. It doesn’t create unnecessary copies of arrays during the concatenation process, making it a preferred choice for large-scale computations.
Potential Pitfalls
While numpy.concatenate()
is powerful, it’s important to note that all input arrays must have the same shape, except in the dimension corresponding to the axis (default is 0). If the arrays do not meet this requirement, you will encounter a ValueError.
Numpy Concatenate with Multidimensional Arrays
As you advance in your Python journey, you’ll often find yourself working with multidimensional arrays. Luckily, numpy.concatenate()
is well-equipped to handle this complexity.
Concatenating Along Different Axes
By default, numpy.concatenate()
joins arrays along the first axis (axis=0). However, you can specify a different axis by passing in the axis
parameter.
Let’s explore this with a code example:
import numpy as np
# Define two two-dimensional arrays
array1 = np.array([[1, 2, 3], [4, 5, 6]])
array2 = np.array([[7, 8, 9], [10, 11, 12]])
# Use numpy concatenate to join the arrays along the second axis
result = np.concatenate((array1, array2), axis=1)
print(result)
# Output:
# array([[ 1, 2, 3, 7, 8, 9],
# [ 4, 5, 6, 10, 11, 12]])
In this example, we’ve concatenated array1
and array2
along the second axis (axis=1). This means that the arrays are joined side-by-side, rather than top-to-bottom.
Understanding the Differences
The key difference when using the axis
parameter is how the arrays are joined. For axis=0
, arrays are joined vertically (top-to-bottom). For axis=1
, arrays are joined horizontally (side-by-side).
Best Practices
When using numpy.concatenate()
with multidimensional arrays, it’s crucial to ensure that your arrays have the same shape along the specified axis. If they don’t, you’ll encounter a ValueError. Always double-check the shapes of your arrays before concatenation to avoid this common pitfall.
Exploring Alternative Methods for Array Concatenation
While numpy.concatenate()
is a versatile function, numpy offers other functions for specific concatenation scenarios. These include numpy.hstack()
, numpy.vstack()
, and numpy.dstack()
.
Horizontal Stacking with numpy.hstack()
The numpy.hstack()
function is equivalent to concatenation along the second axis, except for 1-D arrays where it concatenates along the first axis. Let’s look at an example:
import numpy as np
# Define two one-dimensional arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Use numpy.hstack to join the arrays
result = np.hstack((array1, array2))
print(result)
# Output:
# array([1, 2, 3, 4, 5, 6])
In this scenario, numpy.hstack()
behaves similarly to numpy.concatenate()
. However, the key difference is how numpy.hstack()
handles 1-D arrays.
Vertical Stacking with numpy.vstack()
The numpy.vstack()
function is equivalent to concatenation along the first axis after 1-D arrays of shape (N,)
have been reshaped to (1,N)
. Here’s how it works:
import numpy as np
# Define two one-dimensional arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Use numpy.vstack to join the arrays
result = np.vstack((array1, array2))
print(result)
# Output:
# array([[1, 2, 3],
# [4, 5, 6]])
In this example, numpy.vstack()
joins the arrays vertically (top-to-bottom), similar to numpy.concatenate()
with axis=0
.
Depth Stacking with numpy.dstack()
The numpy.dstack()
function concatenates arrays along the third axis. This function is less common but can be useful in certain scenarios:
import numpy as np
# Define two one-dimensional arrays
array1 = np.array([1, 2, 3])
array2 = np.array([4, 5, 6])
# Use numpy.dstack to join the arrays
result = np.dstack((array1, array2))
print(result)
# Output:
# array([[[1, 4],
# [2, 5],
# [3, 6]]])
Here, numpy.dstack()
joins the arrays along a new third axis, creating a 3-D array.
Comparison of Methods
Function | Equivalent concatenate | Best Use Case |
---|---|---|
hstack | concatenate with axis=1 for 2-D arrays | When you want to join arrays horizontally |
vstack | concatenate with axis=0 | When you want to join arrays vertically |
dstack | concatenate with axis=2 | When you want to join arrays along a third axis |
While numpy.concatenate()
is a great all-rounder, these alternative functions can provide more intuitive syntax in certain scenarios. Choose the one that best fits your use case.
Troubleshooting Numpy Concatenate: Common Issues and Solutions
While numpy.concatenate()
is a powerful tool, you may encounter some issues when using it. Let’s discuss some of these potential pitfalls and how to resolve them.
ValueError: All Input Arrays Must Have Same Number of Dimensions
One common issue is encountering a ValueError with the message ‘all the input arrays must have the same number of dimensions’. This error occurs when the input arrays have different numbers of dimensions.
Let’s look at an example:
import numpy as np
# Define a one-dimensional array and a two-dimensional array
array1 = np.array([1, 2, 3])
array2 = np.array([[4, 5, 6], [7, 8, 9]])
try:
result = np.concatenate((array1, array2))
except ValueError as e:
print(f'ValueError: {e}')
# Output:
# ValueError: all the input arrays must have same number of dimensions
In this example, array1
is a one-dimensional array, while array2
is a two-dimensional array. When we try to concatenate these arrays using numpy.concatenate()
, we encounter a ValueError.
Resolving Dimension Mismatch
To resolve this issue, you can reshape array1
to match the number of dimensions in array2
. Here’s how you can do it:
# Reshape array1 to a two-dimensional array
array1 = array1.reshape(1, -1)
# Now we can concatenate the arrays without issues
result = np.concatenate((array1, array2))
print(result)
# Output:
# array([[1, 2, 3],
# [4, 5, 6],
# [7, 8, 9]])
By reshaping array1
to a two-dimensional array, we can successfully concatenate array1
and array2
using numpy.concatenate()
.
Remember, it’s vital to ensure that all input arrays have the same number of dimensions when using numpy.concatenate()
. This is one of the key considerations to keep in mind for successful array concatenation in numpy.
Understanding Numpy’s Array Data Type and Axes Concept
To fully grasp the numpy.concatenate()
function, it’s crucial to understand two fundamental concepts: numpy’s array data type and the concept of axes in numpy.
Numpy’s Array Data Type
In numpy, the primary data structure is the multidimensional array. This structure is a grid of values, all of the same type, and is indexed by a tuple of non-negative integers. The dimensions are called ‘axes’.
Here’s a simple example of a numpy array:
import numpy as np
# Create a numpy array
array = np.array([1, 2, 3])
print(array)
# Output:
# array([1, 2, 3])
In this example, array
is a one-dimensional array with three items. The array only has one axis (axis 0), and this axis has three elements.
The Concept of Axes in Numpy
In numpy, the term ‘axes’ refers to the dimensions of the array. A 2-dimensional array has two axes: the first running vertically downwards across rows (axis 0), and the second running horizontally across columns (axis 1).
Let’s look at a 2-dimensional array:
# Create a two-dimensional numpy array
array = np.array([[1, 2, 3], [4, 5, 6]])
print(array)
# Output:
# array([[1, 2, 3],
# [4, 5, 6]])
In this example, array
is a two-dimensional array with two axes. The first axis has two elements (two rows), and the second axis has three elements (three columns).
Understanding the array data type and the concept of axes is fundamental to mastering numpy’s concatenate function. With this knowledge, you can effectively manipulate and combine arrays in a variety of ways.
The Impact of Array Concatenation Beyond Coding
Array concatenation, especially with numpy’s concatenate function, plays a significant role in data manipulation and machine learning. By joining arrays, you can create larger and more complex data structures, enabling you to handle more sophisticated tasks.
Array Concatenation in Data Manipulation
In data manipulation, you often need to combine different datasets into a single, more comprehensive dataset. With numpy concatenate, you can easily join arrays of data, making it a crucial tool for data preprocessing in Python.
Array Concatenation in Machine Learning
In machine learning, you frequently need to combine features from different sources into a single feature vector. Numpy concatenate is a common tool for this task, allowing you to create a unified view of your data.
Exploring Related Concepts
Beyond array concatenation, numpy offers a wealth of functionality for array manipulation. These include array splitting, which is the opposite of concatenation, and array reshaping, which allows you to change the number of dimensions and the size along each axis of your arrays.
Here’s a simple example of array splitting:
import numpy as np
# Create a numpy array
array = np.array([1, 2, 3, 4, 5, 6])
# Split the array into three equally sized subarrays
result = np.split(array, 3)
print(result)
# Output:
# [array([1, 2]), array([3, 4]), array([5, 6])]
In this example, we use the numpy.split()
function to split array
into three equally sized subarrays.
Further Resources for Numpy Mastery
For more advanced numpy topics and resources, we recommend checking out IOFlood’s Article, Discovering the Magic of Numpy. This resource offers a comprehensive documentation and resources for mastering the numpy’s library, providing you with the knowledge to further enhance your data manipulation and machine learning tasks.
We have also gathered and additional set of resources that you may find beneficial:
- Python NumPy append() Guide – Master the art of array concatenation and appending values to NumPy arrays for dynamic data handling.
Reshaping Arrays with numpy.reshape() in Python – Learn about the “numpy reshape” function and its significance in transforming array dimensions.
Numpy Arrays Tutorial – An interactive tutorial from LearnPython.org exploring the functionalities of Numpy arrays.
Numpy Questions – A collection of StackOverflow questions and answers focused on Numpy.
Python Numpy Articles on GeeksForGeeks, centered around the usage of Numpy in Python.
By utilizing these curated resources, you can develop robust programming skills, particularly in handling array-oriented operations.
Wrapping Up: Numpy Concatenate Unveiled
In this guide, we delved into the depths of the numpy.concatenate()
function, a powerful tool for joining arrays in Python. We explored its basic usage with simple examples and ventured into more advanced techniques involving multidimensional arrays and different axes.
We also tackled common issues that you may encounter when using numpy.concatenate()
, such as dimension mismatches, and offered solutions to these problems. This knowledge will help you avoid common pitfalls and use the function more effectively.
In addition, we introduced alternative methods for array concatenation, including numpy.hstack()
, numpy.vstack()
, and numpy.dstack()
. These functions offer more specific and intuitive syntax for certain concatenation scenarios. Here’s a quick comparison:
Function | Equivalent concatenate | Best Use Case |
---|---|---|
hstack | concatenate with axis=1 for 2-D arrays | When you want to join arrays horizontally |
vstack | concatenate with axis=0 | When you want to join arrays vertically |
dstack | concatenate with axis=2 | When you want to join arrays along a third axis |
We also touched on the broader implications of array concatenation in fields like data manipulation and machine learning, highlighting the relevance of numpy.concatenate()
beyond coding.
Whether you’re a beginner or an experienced programmer, we hope this guide has provided you with a deeper understanding of numpy concatenate and its applications. Happy coding!