Javadoc Mastery: Your Guide to Java Documentation
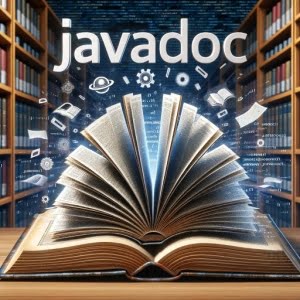
Are you finding it challenging to create professional documentation for your Java code? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled librarian, Javadoc is a handy utility that can seamlessly catalog your Java code for easy reference. These documentations can run on any system, even those without Java installed.
This guide will walk you through using Javadoc to create professional Java documentation. We’ll explore Javadoc’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Javadoc!
TL;DR: What is Javadoc and How Do I Use It?
Javadoc
is a tool included with theJava Development Kit (JDK)
that generates API documentation in HTML format from Java source code. To use Javadoc, you write comments in your source code, prepended with@
, that Javadoc then processes into documentation.
Here’s a simple example:
/**
* This method multiplies two integers.
* @param a the first integer
* @param b the second integer
* @return the product of a and b
*/
public int mult(int a, int b) {
return a * b;
}
In this example, we’ve used Javadoc comments to document a simple method that multiplies two integers. The comments are written in a specific format that Javadoc recognizes. The @param
tags are used to describe the parameters a
and b
, and the @return
tag is used to describe the return value of the method.
This is just a basic way to use Javadoc in Java, but there’s much more to learn about creating and managing professional documentation. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Javadoc for Beginners: Generating Your First Documentation
- Advanced Javadoc: Images, Links, and Tags
- Exploring Alternatives: Doxygen and Doclet
- Troubleshooting Javadoc: Overcoming Common Issues
- Documentation in Software Development: The Javadoc Perspective
- Javadoc in the Bigger Picture: Large Projects and Integration
- Wrapping Up: Javadoc
Javadoc for Beginners: Generating Your First Documentation
Javadoc is a tool that allows you to generate API documentation in HTML format directly from your Java source code. But how exactly do you use it? Let’s break it down.
Writing Javadoc Comments
To use Javadoc, you need to write special comments in your source code. These comments are written in a specific format that Javadoc recognizes. Here’s an example:
/**
* This method adds two integers.
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
In this example, we’re documenting a simple method that adds two integers. The comments are written in a specific format that Javadoc recognizes. The @param
tags are used to describe the parameters a
and b
, and the @return
tag is used to describe the return value of the method.
Generating the Documentation
Once you’ve written your Javadoc comments, you can use the javadoc
command to generate the documentation. Here’s how you can do it:
javadoc MyProgram.java
This command will generate an HTML file named MyProgram.html
that contains the API documentation for the MyProgram.java
file.
Benefits and Potential Pitfalls
Javadoc is a powerful tool that can significantly improve the readability and maintainability of your Java code. It allows you to document your code in a standardized format that other developers can easily understand. However, it’s important to remember that Javadoc is not a substitute for good coding practices. It’s still crucial to write clean, understandable code and use Javadoc to supplement your documentation.
Advanced Javadoc: Images, Links, and Tags
Once you’ve mastered the basics of Javadoc, it’s time to step up your game. Javadoc offers a range of advanced features that can help you create more comprehensive and useful documentation.
Including Images in Javadoc
Yes, you can include images in your Javadoc documentation. This can be particularly useful when you want to illustrate a complex concept or provide a visual aid. Here’s how you do it:
/**
* This class represents a simple 2D point.
* <img src="doc-files/Point.png" alt="Diagram of a Point">
*/
public class Point {
// ...
}
In this example, we’re including an image in the documentation for a Point
class. The image file (Point.png
) should be placed in a directory named doc-files
in the same directory as the source file.
Adding Links to Javadoc
You can also add links to other parts of your documentation or to external resources. This can be useful for cross-referencing related classes or methods. Here’s how you do it:
/**
* This method calculates the distance between this point and another point.
* @param other the other point
* @return the distance
* @see #Point(int, int)
* @see <a href="http://www.example.com">Example.com</a>
*/
public double distance(Point other) {
// ...
}
In this example, we’re using the @see
tag to add a link to the constructor of the Point
class and to an external website.
Using Tags in Javadoc
Javadoc supports a wide range of tags that can help you structure your documentation. We’ve already seen the @param
and @return
tags, but there are many more. For example, the @throws
tag can be used to document exceptions that a method can throw:
/**
* This method divides two integers.
* @param a the dividend
* @param b the divisor
* @return the quotient
* @throws ArithmeticException if b is zero
*/
public int divide(int a, int b) {
if (b == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return a / b;
}
In this example, we’re using the @throws
tag to document that the divide
method can throw an ArithmeticException
if the divisor is zero.
Benefits and Potential Pitfalls
Using these advanced features can make your Javadoc documentation more informative and easier to navigate. However, it’s important to use them judiciously. Overuse of images, links, or certain tags can make your documentation cluttered and harder to read. Always keep your audience in mind and strive for clarity and simplicity.
Exploring Alternatives: Doxygen and Doclet
While Javadoc is the standard tool for generating Java documentation, it’s not the only game in town. There are other tools available that offer different features and capabilities. Let’s take a closer look at two of these alternatives: Doxygen and Doclet.
Doxygen: A Multi-Language Documentation Generator
Doxygen is a versatile documentation generator that supports multiple programming languages, including Java. It’s particularly useful if you’re working on a multi-language project or if you’re already familiar with Doxygen from working with other languages.
Here’s an example of how you might use Doxygen to document a Java method:
/**
* @brief This method adds two integers.
* @param a The first integer.
* @param b The second integer.
* @return The sum of a and b.
*/
public int add(int a, int b) {
return a + b;
}
This is similar to how you would document a method with Javadoc, but with a few differences. For instance, Doxygen uses the @brief
tag to provide a short summary of the method.
Doclet: Customizing Your Java Documentation
A Doclet is a program written in Java that specifies the content and format of the output generated by Javadoc. By creating your own Doclet, you can customize the appearance and structure of your Java documentation to suit your specific needs.
Here’s an example of how you might use a custom Doclet to generate Javadoc documentation:
javadoc -doclet com.mycompany.MyDoclet MyProgram.java
In this example, we’re using the -doclet
option to specify a custom Doclet (com.mycompany.MyDoclet
) that will be used to generate the documentation.
Javadoc vs. Doxygen vs. Doclet: A Comparison
Feature | Javadoc | Doxygen | Doclet |
---|---|---|---|
Language support | Java | Multiple languages | Java |
Customization | Limited | Extensive | Extensive |
Learning curve | Moderate | Steep | Steep |
While Javadoc is easy to use and perfectly suited for Java, Doxygen offers multi-language support and Doclet offers extensive customization options. The best tool for you depends on your specific needs and circumstances.
Remember, the goal is to create clear, comprehensive documentation that helps other developers understand your code. The tool you choose is just a means to that end.
Troubleshooting Javadoc: Overcoming Common Issues
As with any tool, you may encounter issues when using Javadoc. Let’s discuss some common problems and their solutions.
Formatting Issues
One common issue with Javadoc is formatting errors. These can occur if you don’t follow the exact syntax required by Javadoc. For example, forgetting to close a comment or using a tag incorrectly can result in a formatting error.
/**
* This method adds two integers.
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
public int add(int a, int b) {
return a + b;
}
# Output:
# Javadoc: Missing an '@return' tag.
In this example, the Javadoc comment is missing a closing */
, which results in a formatting error. The solution is to ensure that all Javadoc comments are properly formatted and closed.
Missing Documentation
Another common issue is missing documentation. This can occur if you forget to document a class, method, or field, or if your documentation is incomplete.
public class Point {
public int x;
public int y;
}
# Output:
# Javadoc: Class Point is missing documentation.
In this example, the Point
class and its fields (x
and y
) are missing Javadoc comments. The solution is to ensure that all parts of your code are thoroughly documented.
Tips for Using Javadoc Effectively
- Consistency is key. Try to follow a consistent style in your Javadoc comments. This will make your documentation easier to read and understand.
Don’t overdo it. While it’s important to document your code, not every line or variable needs a comment. Focus on documenting the parts of your code that aren’t immediately clear from the code itself.
Keep it up to date. Make sure to update your documentation when you update your code. Outdated documentation can be more confusing than no documentation at all.
Use the right tags. Javadoc supports a wide range of tags that can help you structure your documentation. Make sure to use the right tags for the right purposes.
Documentation in Software Development: The Javadoc Perspective
Documentation is the backbone of any software development project. It serves as a roadmap, guiding developers through the intricate maze of code, making it easier to understand, maintain, and upgrade. Javadoc plays a crucial role in this process, especially in the world of Java programming.
Why Documentation Matters
Documentation is like a user manual for your code. It explains what the code does, how it does it, and why it does it that way. This is vital for team projects where multiple developers need to understand each other’s code. It’s also essential for future-you who, believe it or not, will forget the details of the code you’re writing today.
/**
* This method calculates the factorial of a number.
* @param n the number
* @return the factorial of n
*/
public int factorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i;
}
return result;
}
# Output:
# The method takes an integer as input and returns its factorial.
In this code block, the Javadoc comments explain the purpose of the factorial
method, its parameters, and its return value. Without these comments, another developer (or future-you) would have to read and understand the code to figure out what it does.
The Concept of ‘Self-Documenting Code’
‘Self-documenting code’ is a term used to describe code that is written in a way that’s easy to understand without needing separate documentation. While it’s a good practice to write self-documenting code, it’s not a substitute for formal documentation. Think of self-documenting code as the plot of a novel, and Javadoc as the detailed notes in the margins that explain the plot, characters, and themes in depth.
Javadoc and self-documenting code go hand in hand. Javadoc comments can explain the why and the what, while self-documenting code can demonstrate the how. Together, they form a complete picture that makes your Java code easier to understand and maintain.
Javadoc in the Bigger Picture: Large Projects and Integration
Javadoc isn’t just for small projects or standalone classes. It’s designed to scale up and can be a powerful tool for managing documentation in larger projects. Moreover, it integrates smoothly with many popular development tools, making it a versatile part of your Java toolkit.
Javadoc for Large Projects
In larger projects, maintaining consistent and up-to-date documentation can become a challenge. Javadoc shines in this scenario. By embedding the documentation directly in the code, it ensures that the documentation is always just a keystroke away and evolves with your code.
/**
* This class represents a customer in our system.
* Each customer has a name and a unique ID.
*/
public class Customer {
private String name;
private String id;
// ...
}
In this example, the Customer
class might be one of hundreds in a large project. By using Javadoc, you can quickly generate a comprehensive overview of all classes and their relationships.
Integration with IDEs and Build Tools
Most Integrated Development Environments (IDEs) for Java, such as Eclipse and IntelliJ IDEA, have built-in support for Javadoc. They can generate Javadoc comments automatically, show the documentation inline while you’re coding, and even highlight syntax errors or missing tags.
Similarly, build tools like Maven and Gradle can generate Javadoc documentation as part of the build process. This ensures that your documentation is always up-to-date and matches the current state of your code.
Code Readability and Software Architecture
Good documentation goes hand in hand with readable code and solid software architecture. By using Javadoc consistently, you’re not just documenting your code; you’re also promoting good coding practices, improving code readability, and contributing to a robust software architecture.
Further Resources for Mastering Javadoc
To continue your journey in mastering Javadoc, consider exploring the following resources:
- Exploring Java Comments – Explore Java comment best practices for effective communication within your codebase.
Javadoc Comments Basics – Dive into Javadoc comments in Java for generating API documentation.
Oracle’s Official Javadoc Documentation – A comprehensive guide provided by the creators of Java.
Baeldung’s Guide to Javadoc is a practical guide that covers many aspects of Javadoc.
Java API Documentation by Stony Brook University covers various classes and packages in the Java SDK.
Wrapping Up: Javadoc
In this comprehensive guide, we’ve delved into the world of Javadoc, a powerful tool for creating professional Java documentation. We’ve explored how Javadoc can help catalog your Java code, making it easier for others (and future-you) to understand and maintain your code.
We started with the basics, learning how to write Javadoc comments and generate HTML documentation from our Java source code. We then moved on to more advanced features, such as including images and links in our documentation, and using a variety of tags to structure and enhance our documentation.
We also tackled common issues that you might encounter when using Javadoc, such as formatting errors and missing documentation, and provided solutions to help you overcome these challenges. Furthermore, we took a look at alternative tools for Java documentation, such as Doxygen and Doclet, giving you a broader perspective on the documentation landscape.
Here’s a quick comparison of these methods:
Method | Language Support | Customization | Learning Curve |
---|---|---|---|
Javadoc | Java | Limited | Moderate |
Doxygen | Multiple languages | Extensive | Steep |
Doclet | Java | Extensive | Steep |
Whether you’re just starting out with Javadoc or you’re looking to level up your Java documentation skills, we hope this guide has given you a deeper understanding of Javadoc and its capabilities.
With its balance of simplicity and power, Javadoc is an essential tool for any Java developer. It not only makes your code more understandable but also promotes good coding practices and contributes to a robust software architecture. Happy coding!