Python Deepcopy Syntax and Usage Guide (With Examples)
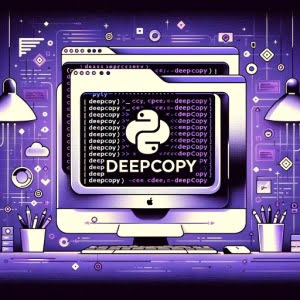
The deepcopy function in Python is a potent tool, often underutilized, that can simplify your management of mutable objects. Once you grasp its functionality, it can significantly bolster your Python programming prowess.
This guide is designed to equip you with a comprehensive understanding of Python’s deepcopy function, including its application and usage. By the conclusion of this article, you’ll be adept at utilizing Python’s deepcopy function in your projects and be able to engage in meaningful discussions about its implications with fellow Python enthusiasts.
TL;DR: What is Python’s deepcopy function?
Python’s deepcopy function is a method that creates a new and separate copy of an entire object or list with its own unique memory address. This means changes to the original object or list won’t affect the copied object or list and vice versa. It is particularly useful when working with mutable objects like lists, dictionaries, or custom objects in Python. For more advanced methods, background, tips, and tricks, continue reading the rest of the article.
Example:
import copy
# Original list
orig_list = [1, 2, [3, 4]]
# Deep copy of the list
deep_list = copy.deepcopy(orig_list)
# Change original list
orig_list[2][0] = 'a'
print('Original:', orig_list)
print('Deep Copy:', deep_list)
This code block demonstrates the basic usage of Python’s deepcopy function. It creates a deep copy of a list and then modifies the original list. The change is only reflected in the original list, not in the deep copy.
Table of Contents
Understanding Python’s Deepcopy
The deepcopy function in Python, as the name implies, generates a deep copy of an object. But what exactly does this entail?
In Python, a deep copy generates a new and independent copy of an entire object or list, complete with its unique memory address. Consequently, modifications to the original object or list do not impact the copied object or list, and vice versa. This function is a component of Python’s copy module.
Let’s consider a simple example to illustrate how deepcopy operates:
import copy
# Original dictionary
orig_dict = {'key1': 'value1', 'key2': {'key3': 'value3'}}
# Deep copy of the dictionary
deep_dict = copy.deepcopy(orig_dict)
# Change original dictionary
orig_dict['key2']['key3'] = 'new value'
print('Original:', orig_dict)
print('Deep Copy:', deep_dict)
In this example, after creating a deep copy of orig_dict
, we change the value of key3
inside the inner dictionary of orig_dict
. When we print both the original and the deep copied dictionary, the change is reflected only in the original dictionary and not in the deep copied one. This is because a deep copy creates copies for all nested objects, ensuring that any changes in the original object doesn’t affect the copied one.
When should you use deepcopy?
You should consider using deepcopy when you’re dealing with mutable objects and you desire to make changes to a copy of an object without affecting the original. This is especially handy when working with mutable Python objects such as lists, dictionaries, or custom objects.
Deepcopy vs Shallow Copy
Having delved into the concept of a deep copy in Python, it’s time to introduce its counterpart: the shallow copy.
A shallow copy, similar to a deep copy, creates a new object. However, in contrast to a deep copy, it doesn’t create a separate copy of inner objects. Instead, it simply replicates the references to the inner objects.
This implies that if you modify an inner object in the original object, the change will be mirrored in the shallow copy, and vice versa.
Deepcopy | Shallow Copy | |
---|---|---|
Creates a new object | Yes | Yes |
Creates a separate copy of inner objects | Yes | No |
Changes to the original object affect the copy | No | Yes |
Changes to the copy affect the original object | No | Yes |
Memory usage | High | Low |
Speed | Slow | Fast |
Let’s examine the following example to better understand this concept:
import copy
# Original list
orig_list = [1, 2, [3, 4]]
# Shallow copy of the list
shallow_list = copy.copy(orig_list)
# Change original list
orig_list[2][0] = 'a'
print('Original:', orig_list)
print('Shallow Copy:', shallow_list)
In this scenario, when we alter the original list, the change is also reflected in the shallow copy. This is because the shallow copy doesn’t possess its own separate copy of the inner list; it merely has a reference to the same inner list that the original list contains.
When should you use a deepcopy over a shallow copy?
With a deepcopy, you’re creating an entirely separate copy of an object, which can be safer if you aim to ensure that modifications to the copy don’t impact the original. However, creating a deepcopy can be slower and more memory-demanding, particularly for large objects, because a new object needs to be created and filled with copies of the data from the original object.
A shallow copy can be faster and less memory-consuming, as it’s merely creating a new object and copying references to the inner objects. But it can lead to unexpected behavior if not handled carefully, as modifications to the inner objects of the original object will affect the copy.
Advanced Usage of Deepcopy
While the fundamental usage of Python’s deepcopy function is relatively straightforward, there are advanced scenarios where a deeper understanding of deepcopy can be beneficial. Let’s delve into these scenarios.
Deepcopy and Custom Objects
One such scenario is when you’re working with custom objects, such as those created from your own classes. Python’s deepcopy function can handle these as well.
When you perform a deepcopy on a custom object, it creates a new object of the same class and populates it with deep copies of the original object’s attributes.
Consider the following example:
class MyClass:
def __init__(self, value):
self.value = value
import copy
# Original object
orig_obj = MyClass([1, 2, 3])
# Deep copy of the object
deep_obj = copy.deepcopy(orig_obj)
# Change original object
orig_obj.value[0] = 'a'
print('Original:', orig_obj.value)
print('Deep Copy:', deep_obj.value)
In this example, we create a deep copy of a custom object. When we modify the original object, the change is not reflected in the deep copy.
import copy
class MyClass:
def __init__(self, value):
self.value = value
# Original object
orig_obj = MyClass([1, 2, 3])
# Deep copy of the object
deep_obj = copy.deepcopy(orig_obj)
# Change original object
orig_obj.value[0] = 'a'
print('Original:', orig_obj.value)
print('Deep Copy:', deep_obj.value)
Potential Pitfalls with Deepcopy
While deepcopy is a powerful tool, it’s not without its potential pitfalls. We’ll go over a few of those here.
Recursive References
A possible pitfall could occur when working with objects that have recursive references. Python’s deepcopy module handles this by maintaining a memo dictionary of objects already copied during the current copying process. This prevents infinite recursion which would otherwise happen in these situations.
Here is an example:
import copy
# Recursive list
rec_list = [0]
rec_list.append(rec_list)
# Deep copy of the recursive list
deep_list = copy.deepcopy(rec_list)
# Modifying original recursive list
rec_list[0] = 'a'
print('Original:', rec_list)
print('Deep Copy:', deep_list)
In this example, rec_list
is a recursive list that references itself. After creating a deep copy of this list, we modify the original list. When printing both lists, the change appears only in the original list, not in the deep copy.
Custom DeepCopy Methods
Another potential pitfall is when you’re dealing with objects that define their own __copy__()
or __deepcopy__()
method. Python’s deepcopy will use these methods if they are present to copy the object, and this might not always result in the required behavior.
Here’s an illustration:
import copy
class Foo:
def __init__(self, foo):
self.foo = foo
def __deepcopy__(self, memo):
return Foo(self.foo + "_deepcopy")
foo_instance = Foo("test")
foo_deepcopy = copy.deepcopy(foo_instance)
print('Original:', foo_instance.foo)
print('Deep Copy:', foo_deepcopy.foo)
In this example, the class Foo
has the custom __deepcopy__
method that changes the behaviour of the deep copy operation. After creating a deep copy of foo_instance
, we see that the string "_deepcopy"
is appended to the foo
attribute in the deep copied instance, whereas the foo
attribute in the original instance is unchanged.
Performance Considerations with Deepcopy
When using deepcopy, it’s important to keep in mind that creating a deep copy of an object can be slower and more memory-intensive than creating a shallow copy. This is because a new object needs to be created and populated with copies of the data from the original object. If you’re working with large objects or complex data structures, the performance impact can be significant.
Python’s Copy Module
While the deepcopy function is a key tool in managing mutable objects in Python, it’s part of a larger toolkit: the Python copy module. This module offers functions for creating copies of arbitrary Python objects, including both shallow and deep copies.
The copy module in Python provides two main functions: copy.copy()
and copy.deepcopy()
. We’ve already explored these functions in depth. However, the copy module also provides additional functionality that can be useful in certain scenarios.
For instance, the copy module provides the copy.error
exception, which is raised for class instances that have a __copy__()
or __deepcopy__()
method.
Let’s revisit the example of using the copy module to create a shallow copy of a list:
import copy
# Original list
orig_list = [1, 2, [3, 4]]
# Shallow copy of the list
shallow_list = copy.copy(orig_list)
# Change original list
orig_list[2][0] = 'a'
print('Original:', orig_list)
print('Shallow Copy:', shallow_list)
In this example, we create a shallow copy of a list using the copy.copy()
function. When we modify the original list, the change is reflected in the shallow copy because the shallow copy shares references to the inner objects with the original.
Beyond Deepcopy
Python’s deepcopy function and the copy module are indeed powerful tools, but their true power is realized when we understand them in the context of broader Python concepts.
Deepcopy is intrinsically linked to several other Python concepts. For instance, understanding Python’s data model, especially the distinction between mutable and immutable objects, is crucial for understanding when and why to use deepcopy.
Similarly, understanding Python’s memory management is key. When you create a deep copy of an object, Python allocates new memory for the copy, which can have implications for performance and memory usage in your programs.
Related Python Concepts | Description |
---|---|
Mutable vs Immutable Objects | Understanding the difference is crucial for knowing when to use deepcopy |
Python’s Data Model | Deepcopy is a part of Python’s data model |
Memory Management in Python | Deepcopy creates a new object with its own memory, affecting performance and memory usage |
Further Resources for Python Modules
Looking to delve deeper into the world of Python Modules? We’ve compiled a list of valuable resources to pave your learning pathway:
- Python Modules Tutorial: Getting Started – Explore the role of modules in simplifying large-scale Python projects.
Simplifying Data Hashing with Python hashlib – Learn how to generate and verify hashes using hashlib in Python.
Pickling and Unpickling in Python: A Quick Tutorial – Explore Python’s “pickle” module for object serialization.
Python Object Serialization and Deserialization with Pickle – Medium article that provides a guide on Python’s pickle module.
Modules for Serialization and Deserialization in Python – An overview of available Python serialization and deserialization modules.
Serialization Intro for Python – Machine Learning Mastery’s guide to understanding the concept of serialization in Python.
Invest time in these resources and continue your journey towards becoming a seasoned Python developer.
Conclusion
Python’s deepcopy function is a hidden gem that can significantly enhance your Python programming skills. It provides a powerful way to create separate copies of mutable objects, preventing unintentional modifications and leading to cleaner, bug-free code. Understanding when and how to use deepcopy is a crucial skill for any Python programmer.
But the journey doesn’t stop at deepcopy. The Python copy module, which houses the deepcopy function, is a treasure trove of tools for managing mutable objects. Mastering this module can lead to more efficient memory management and a deeper understanding of Python’s data model.
So, the next time you’re working with mutable objects in Python, remember the power of deepcopy and the copy module. Use them wisely, and you’ll be well on your way to becoming a Python pro.