Python Sum() Function: Ultimate Usage Guide
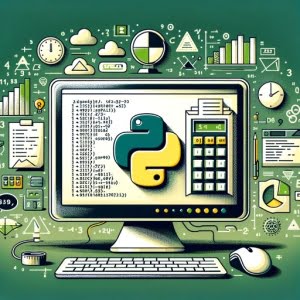
Struggling to add elements in Python? Like a seasoned accountant, Python’s sum function can easily tally up all your numbers. This guide will walk you through the sum function, from basic usage to advanced techniques.
The sum function is a built-in Python command that simplifies the process of adding numbers in a list, tuple, or any iterable object in Python. Whether you’re a beginner just starting out or an experienced programmer looking to refine your skills, understanding how to use the sum function can significantly boost your coding efficiency.
In this guide, we’ll explore the sum function in depth, providing practical examples and highlighting potential pitfalls. By the end, you’ll have a solid grasp of the Python sum function and how to use it effectively in your programming projects.
TL;DR: How Do I Use the Sum Function in Python?
The
sum()
function in Python adds up all the elements in an iterable. Here’s a simple example:
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total)
# Output:
# 15
In this example, we have a list of numbers from 1 to 5. We use the sum function to add all these numbers together, resulting in a total of 15. This showcases the basic usage of the sum function in Python, which is an essential tool for performing addition operations on iterable objects.
Continue reading for a comprehensive guide on the Python sum function, including more detailed usage and advanced scenarios.
Table of Contents
- Python Sum Function: A Beginner’s Guide
- Advanced Python Sum Function: Beyond the Basics
- Exploring Alternatives to Python Sum Function
- Troubleshooting Python Sum Function: Common Issues
- Understanding Python Iterables and Addition
- Python Sum in Data Analysis and Machine Learning
- Exploring Related Concepts
- Further Resources
- Wrapping Up: Python Sum Function
Python Sum Function: A Beginner’s Guide
The sum function in Python is a built-in function that adds all the elements in an iterable (like a list or tuple) and returns the total. It’s a straightforward and efficient way to perform addition operations in Python.
Let’s take a look at a simple example:
# Here is a list of numbers
digits = [4, 2, 9, 3, 5]
# Using the sum function to add all numbers in the list
total = sum(digits)
print(total)
# Output:
# 23
In this example, we have a list of numbers digits
. We use the sum
function to add all these numbers together, resulting in a total of 23. This is the basic usage of the sum
function in Python.
Advantages of Python Sum Function
The sum
function is a quick and efficient way to add numbers in an iterable. It’s built into Python, so you don’t need to import any additional modules or write any extra loops to use it.
Potential Pitfalls
While the sum
function is powerful, it’s important to note that it can only add numbers. If you try to use it on a list of strings, for example, you’ll get a TypeError
. Also, the sum
function can be slower than other methods when dealing with large datasets, so it’s important to consider the size and nature of your data when deciding whether to use sum
.
Advanced Python Sum Function: Beyond the Basics
As you become more comfortable with the sum function, you can start exploring its more advanced uses. The sum function isn’t limited to adding up numbers in a single list – it can also handle more complex data structures like a list of lists. Plus, it offers an optional start
parameter that you can use to specify a value to add to the sum.
Summing Nested Lists
Let’s say you have a list of lists, and you want to add up all the numbers across all the lists. Here’s how you can do it with the sum function:
# Here is a list of lists
nested_lists = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# Using the sum function with a generator expression to add all numbers
grand_total = sum(sum(sublist) for sublist in nested_lists)
print(grand_total)
# Output:
# 45
In this example, we use a generator expression (sum(sublist) for sublist in nested_lists)
to calculate the sum of each sublist, and then we use the sum function again to add up these sums. The result is the grand total of all the numbers across all the lists.
Using the Start Parameter
The sum function also accepts an optional start
parameter, which is added to the total sum. Here’s an example:
# Here is a list of numbers
numbers = [1, 2, 3, 4, 5]
# Using the sum function with a start parameter
total = sum(numbers, 10)
print(total)
# Output:
# 25
In this example, we specify a start value of 10. The sum function adds this start value to the sum of the numbers in the list, resulting in a total of 25.
Exploring Alternatives to Python Sum Function
While the sum function is a powerful tool for adding numbers in Python, it’s not the only way to do it. There are other methods that you can use, depending on your specific needs and the nature of your data. Let’s explore two of these alternatives: the reduce function from the functools module and list comprehension.
Using Reduce Function
The reduce function is a part of Python’s functools module, and it can be used to apply a particular function to all of the elements in a list. Here’s how you can use it to add numbers:
from functools import reduce
import operator
numbers = [1, 2, 3, 4, 5]
# Using the reduce function to add all numbers in the list
total = reduce(operator.add, numbers)
print(total)
# Output:
# 15
In this example, we import the reduce function from the functools module and the add function from the operator module. We then use reduce to apply the add function to all the numbers in the list, resulting in a total of 15.
List Comprehension
List comprehension is a concise way to create lists in Python. It can also be used to add elements in a list, although it’s a bit more complex than using the sum function. Here’s an example:
numbers = [1, 2, 3, 4, 5]
# Using list comprehension to add all numbers in the list
total = sum([num for num in numbers])
print(total)
# Output:
# 15
In this example, we use list comprehension to create a new list that contains all the numbers in the original list, and then we use the sum function to add these numbers together.
Both of these methods offer their own advantages. The reduce function is versatile and can be used with any function, not just addition. List comprehension, on the other hand, is a powerful tool that can simplify your code and make it more readable. However, both methods can be slower than the sum function when dealing with large datasets, so it’s important to consider the size and nature of your data when choosing which method to use.
Troubleshooting Python Sum Function: Common Issues
While the Python sum function is a useful tool, you might encounter some issues when using it. One of the most common errors is a TypeError, which occurs when you try to sum non-numeric data types, such as strings. Let’s explore this issue and its solution.
TypeError: Unsupported Operand Type
Here’s an example of a TypeError that you might encounter when using the sum function:
# Here is a list of strings
words = ['Hello', 'World']
try:
total = sum(words)
except TypeError as e:
print(f'Error: {e}')
# Output:
# Error: unsupported operand type(s) for +: 'int' and 'str'
In this example, we try to use the sum function on a list of strings, which results in a TypeError. The error message tells us that we’re trying to use the +
operator (which is what the sum function uses internally) on an integer and a string, which is not allowed.
How to Sum a List of Strings
If you have a list of strings and you want to join them together, you can use the join
method, which is a string method in Python. Here’s how you can do it:
# Here is a list of strings
words = ['Hello', 'World']
# Using the join method to join the strings
total = ' '.join(words)
print(total)
# Output:
# Hello World
In this example, we use the join
method to join the strings in the list, with a space (' '
) as the separator. The result is a single string that contains all the words in the list, separated by spaces.
Remember, the Python sum function is a powerful tool, but like all tools, it has its limitations. Understanding these limitations and knowing how to work around them is a key part of becoming a proficient Python programmer.
Understanding Python Iterables and Addition
To fully grasp the power of the Python sum
function, it’s crucial to understand two fundamental concepts: Python’s iterable objects and the concept of addition in Python.
Python Iterables: Lists, Tuples, and More
An iterable in Python is any object capable of returning its elements one at a time. Common examples of iterables include lists, tuples, and strings. When you use the sum
function, you typically pass in an iterable, like a list of numbers:
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total)
# Output:
# 15
In this example, numbers
is a list of integers, which is an iterable. The sum
function goes through each number in the list one by one, adding them up to calculate the total.
Addition in Python: More Than Just Numbers
Addition in Python isn’t limited to just numbers. You can also add strings, lists, and other data types. However, when you’re using the sum
function, you’re typically adding numbers. If you try to use sum
with a list of strings, you’ll get a TypeError:
words = ['Hello', 'World']
try:
total = sum(words)
except TypeError as e:
print(f'Error: {e}')
# Output:
# Error: unsupported operand type(s) for +: 'int' and 'str'
In this example, we tried to use sum
on a list of strings, which resulted in a TypeError. This is because sum
is designed to work with numbers, not strings. If you want to join strings together, you should use the join
method instead.
Understanding Python’s iterable objects and the concept of addition in Python will give you a deeper understanding of the sum
function and its capabilities.
Python Sum in Data Analysis and Machine Learning
The Python sum
function is more than just a simple tool for adding numbers. It has wider applications in fields like data analysis and machine learning, where addition operations are frequently used.
Python Sum Function in Data Analysis
In data analysis, you often need to perform calculations on large datasets. The sum
function can be used to quickly add up numbers in a dataset, such as the total sales in a month or the total number of website visits.
Python Sum Function in Machine Learning
In machine learning, the sum
function can be used in various algorithms, like calculating the sum of squared errors in linear regression or the sum of weights in neural networks.
Exploring Related Concepts
If you’re interested in further exploring Python’s capabilities in numerical operations, you might want to check out Python’s statistics module and the NumPy library.
Python’s Statistics Module
The statistics module provides functions to mathematical statistics of numeric data. This includes functions to calculate measures of central location, dispersion, and more.
NumPy Library
NumPy is a powerful library for numerical computing in Python. It offers a wide range of mathematical functions beyond addition, including operations on multi-dimensional arrays and matrices.
Further Resources
For a deeper understanding of Python’s numerical capabilities, consider checking out the following resources:
- Python Built-In Functions: Mastering Python’s Arsenal – Discover the role of Python’s built-in functions in enhancing code efficiency and productivity.
Filtering Data in Python with the filter() Function – Dive into functional programming and condition-based filtering with “filter”.
Identifying Data Types in Python with the type() Function – Explore dynamic typing, object introspection, and type validation.
Python’s Official Documentation – Dive into the comprehensive Python library with this detailed documentation.
NumPy’s Official Documentation – Expand your understanding of the NumPy library for numerical computing.
Python for Data Analysis – Strengthen your data analysis skills in Python with this book by Wes McKinney.
Wrapping Up: Python Sum Function
The Python sum
function is a powerful built-in tool for adding numbers in an iterable. It’s quick, efficient, and easy to use, making it an essential part of any Python programmer’s toolkit.
Throughout this guide, we’ve explored the basic usage of the sum
function, along with more advanced techniques like summing nested lists and using the start
parameter. We’ve also discussed common issues you might encounter, such as TypeError when trying to sum a list of strings, and how to solve them.
In addition to the sum
function, we’ve also looked at alternative methods for adding elements in Python, including the reduce
function from the functools module and list comprehension. These methods offer their own advantages, and understanding when to use each one can make your code more efficient and readable.
Remember, the best method for adding elements in Python depends on your specific needs and the nature of your data. By understanding the different methods and their strengths and weaknesses, you can choose the most effective tool for the task at hand.