Python ‘Main’ Function: Syntax and Usage Guide
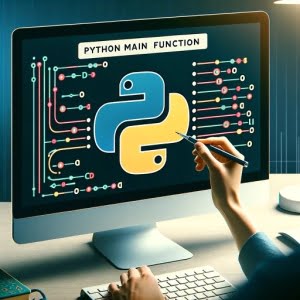
Ever wondered about the role of the main function in Python? Just like the director of a movie, the main function in Python orchestrates the flow of your program. It’s the entry point that sets the stage for the execution of your script.
But what exactly is this main function, and why is it so crucial in Python programming? In this guide, we will demystify the main function in Python, exploring its purpose, and how to use it effectively.
Whether you’re a beginner just starting out with Python or an intermediate developer looking to refine your skills, this guide offers insights that will enhance your understanding of Python programming.
TL;DR: What is the main function in Python and how do I use it?
The main function in Python is the entry point of a script. It’s defined using
def main():
. Here’s a simple example:
def main():
print('Hello, World!')
if __name__ == '__main__':
main()
# Output:
# 'Hello, World!'
In this example, we define a function named main
that prints ‘Hello, World!’. The if __name__ == '__main__':
line checks if the script is being run directly or being imported. If the script is run directly, it calls the main
function and prints ‘Hello, World!’.
Dive deeper into this guide for a comprehensive understanding and more advanced usage scenarios of the main function in Python.
Table of Contents
- Getting Started with the Python Main Function
- Advantages and Potential Pitfalls
- Implementing the Main Function in Larger Scripts
- Handling Command-line Arguments with argparse
- Exploring Alternative Approaches
- Navigating Common Errors and Obstacles
- The __name__ Attribute and Python Script Execution
- Exploring Further: The Main Function in Larger Projects
- Wrapping Up: Python Main Function Recap
Getting Started with the Python Main Function
Before we dive into the complexities, let’s start with the basics of defining and calling the main function in Python. We use the def
keyword to define the main function, like so:
def main():
print('This is the main function')
In this example, we’ve defined a function named main
that prints ‘This is the main function’ when called. However, defining the function is not enough. We need to call it for it to execute.
To call the main function, we use if __name__ == '__main__':
. Here’s how we can do it:
def main():
print('This is the main function')
if __name__ == '__main__':
main()
# Output:
# 'This is the main function'
In this code block, if __name__ == '__main__':
is checking if the script is being run directly or being imported. If the script is run directly, it calls the main
function and prints ‘This is the main function’.
Advantages and Potential Pitfalls
Using the main function in Python has its advantages. It helps keep your code organized, makes it more readable, and allows you to control the flow of your program. However, if not used correctly, it can lead to potential pitfalls. For example, if you forget to call the main function using if __name__ == '__main__':
, it won’t execute when the script is run directly.
Implementing the Main Function in Larger Scripts
As your Python scripts grow in complexity, the main function becomes increasingly important. It serves as the entry point of your script, allowing you to control the flow of your program. Here’s an example of how you can structure a larger Python script around the main function:
def helper_function():
return 'Hello from helper function!'
def main():
message = helper_function()
print(message)
if __name__ == '__main__':
main()
# Output:
# 'Hello from helper function!'
In this example, we have a helper_function
that returns a message. The main
function calls this helper_function
, stores its output in the message
variable, and then prints this message
. When the script is run directly, the main
function is called due to if __name__ == '__main__':
, resulting in the message from helper_function
being printed.
Handling Command-line Arguments with argparse
Python’s argparse
module makes it easy to write user-friendly command-line interfaces. It can handle positional arguments, optional arguments, and even sub-commands. Here’s an example of how you can use argparse
in the main function to handle command-line arguments:
import argparse
def main():
parser = argparse.ArgumentParser()
parser.add_argument('--name', help='Your name')
args = parser.parse_args()
print(f'Hello, {args.name}!')
if __name__ == '__main__':
main()
# Command:
# python script.py --name 'Python Developer'
# Output:
# 'Hello, Python Developer!'
In this code, we import argparse
and define a main
function. Inside the main
function, we create a parser object and add an argument --name
. When we run the script with a --name
argument, it prints a personalized greeting.
Exploring Alternative Approaches
While the main function is a fundamental part of Python programming, there are alternative methods and related concepts that can provide additional flexibility and functionality. Let’s delve into some of these alternatives.
Leveraging the __name__
Attribute
The __name__
attribute is a special built-in variable in Python. When a script is run directly, its __name__
attribute is set to '__main__'
. However, when the script is imported as a module, the __name__
attribute is set to the name of the script/module. Here’s an example:
print(__name__)
# Output when the script is run directly:
# '__main__'
# Output when the script is imported as a module:
# 'script_name'
This behavior of the __name__
attribute allows us to control the execution of our code based on whether the script is being run directly or imported as a module.
Using sys.argv
for Command-line Arguments
Before argparse
, there was sys.argv
. It’s a list in Python, which contains the command-line arguments passed to the script. The first item in this list, sys.argv[0]
, is always the script’s name. Here’s an example:
import sys
def main():
print(f'Hello, {sys.argv[1]}!')
if __name__ == '__main__':
main()
# Command:
# python script.py 'Python Developer'
# Output:
# 'Hello, Python Developer!'
In this code, we import the sys
module and define a main
function. The main
function prints a personalized greeting using sys.argv[1]
, which is the first command-line argument.
Third-party Libraries: Click and Fire
There are also third-party libraries like Click and Fire that provide more advanced features for building command-line interfaces. These libraries offer decorators for defining commands and arguments, automatic help generation, and other handy features. However, they require an additional installation step and might be overkill for simple scripts.
# Example using Click
import click
@click.command()
@click.option('--name', default='Python Developer', help='Your name')
def main(name):
print(f'Hello, {name}!')
if __name__ == '__main__':
main()
# Command:
# python script.py --name 'Python Developer'
# Output:
# 'Hello, Python Developer!'
In this Click example, we define a main
function with a --name
option. When we run the script with a --name
argument, it prints a personalized greeting.
As with any programming concept, understanding the main function in Python and its intricacies can sometimes lead to errors. Here, we will address some common errors and obstacles you may encounter, along with their solutions.
Error: Calling the Main Function Incorrectly
A common error is calling the main function incorrectly. For example, you may forget to include parentheses when calling the main function, like so:
def main():
print('Hello, World!')
if __name__ == '__main__':
main # Incorrect! Should be main()
This will not call the main function, and ‘Hello, World!’ will not be printed. The correct way to call the main function is main()
, with parentheses.
Considerations: Structuring Your Python Script
When structuring your Python script, consider placing all function definitions at the top of your script and the main function call at the bottom. This way, all your functions are defined before they are called, which can help avoid errors.
Optimization: Using Command-line Arguments
If your script needs to take in user input, consider using command-line arguments. This can make your script more flexible and easier to use. Python’s built-in argparse
module or third-party libraries like Click and Fire can help with this.
Remember, practice and experience are key to mastering the use of the main function in Python. Don’t be discouraged by errors or obstacles – they are opportunities to learn and improve!
The __name__
Attribute and Python Script Execution
To fully grasp the concept of the main function in Python, it’s crucial to understand the __name__
attribute and the if __name__ == '__main__':
idiom.
Understanding the __name__
Attribute
In Python, __name__
is a special built-in variable. When a Python script is run directly, its __name__
attribute is set to '__main__'
. However, when the script is imported as a module, the __name__
attribute is set to the name of the script/module.
Here’s an example to illustrate this behavior:
print(__name__)
# Output when the script is run directly:
# '__main__'
# Output when the script is imported as a module:
# 'script_name'
The if __name__ == '__main__':
Idiom
The if __name__ == '__main__':
idiom is a common way to call the main function in a Python script. It checks if the script is being run directly or being imported. If the script is run directly, the code block under if __name__ == '__main__':
is executed.
Script Execution vs Import
When a Python script is run directly, it’s often to perform a specific task or start a program. On the other hand, when a script is imported as a module, it’s usually to use its functions, classes, or variables in another script.
The main function plays a crucial role in controlling what gets executed when a script is run directly versus when it’s imported. By placing code inside the main function, you can ensure that it only gets executed when the script is run directly.
Structuring a Python Script
A well-structured Python script often has function definitions at the top, the main function definition in the middle, and the main function call (if __name__ == '__main__': main()
) at the bottom. This structure ensures that all functions are defined before they are called, and it clearly separates the script’s reusable components (functions) from the script’s entry point (main function).
Exploring Further: The Main Function in Larger Projects
The main function’s utility extends beyond simple scripts—it plays a vital role in larger Python projects as well. It serves as the entry point of your application, providing a clear picture of the program’s flow and making the code easier to read and maintain.
Command-line Interfaces
In larger projects, the main function often interacts with command-line interfaces (CLI). CLI allows users to interact with the program through textual commands, making it an essential part of many Python applications. Here’s a simple example of a main function interacting with a CLI:
import argparse
def main():
parser = argparse.ArgumentParser()
parser.add_argument('--name', help='Your name')
args = parser.parse_args()
print(f'Hello, {args.name}!')
if __name__ == '__main__':
main()
# Command:
# python script.py --name 'Python Developer'
# Output:
# 'Hello, Python Developer!'
In this example, the main function uses the argparse
module to parse command-line arguments and prints a personalized greeting.
Modules and Packages
Modules and packages are another aspect where the main function comes into play. When you import a Python module, the interpreter executes all the code in the module, from top to bottom. However, code under the if __name__ == '__main__':
block is not executed, because the __name__
attribute of the imported module is not '__main__'
. This allows you to control which parts of your module are executed on import and which parts are executed when the module is run directly.
Further Learning Resources
This guide provides a comprehensive overview of the main function in Python. However, there’s always more to learn! For more in-depth information on related topics such as command-line interfaces, modules, and packages, consider checking out the Python documentation or other Python learning resources.
Wrapping Up: Python Main Function Recap
In this guide, we’ve journeyed through the fundamentals of the Python main function, its purpose, and its usage. We’ve learned that the main function is the entry point of a Python script, controlling the program’s flow and enhancing readability.
We’ve also explored common issues such as the incorrect calling of the main function and provided solutions to navigate these obstacles. Furthermore, we’ve delved into alternative approaches to handle command-line arguments and structuring Python scripts, including the use of the argparse
module and third-party libraries like Click and Fire.
To summarize, here’s a quick comparison of the methods discussed:
Method | Use | Complexity |
---|---|---|
if __name__ == '__main__': | Running code when the script is run directly | Beginner |
argparse | Handling command-line arguments | Intermediate |
sys.argv | Handling command-line arguments (alternative) | Intermediate |
Click, Fire | Handling command-line arguments (advanced) | Expert |
The Python main function is a powerful tool in any developer’s arsenal. Understanding it and its related concepts is crucial for mastering Python programming. As always, the key to learning is practice. So, roll up your sleeves and start coding!