Declaring and Constructing Java Classes: A How-To Guide
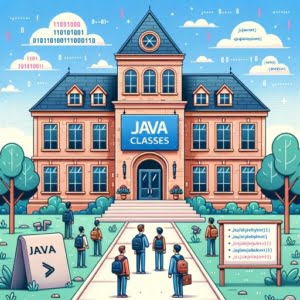
Are you finding it challenging to work with Java classes? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Think of Java classes as a blueprint – a blueprint that allows us to create objects in Java. These objects are the building blocks of any Java application, making classes an essential part of Java programming.
This guide will walk you through the process of creating and using classes in Java, from the basics to more advanced techniques. We’ll cover everything from declaring fields, creating methods, defining constructors to more advanced concepts such as inheritance, polymorphism, and encapsulation.
So, let’s dive in and start mastering Java classes!
TL;DR: How Do I Create a Class in Java?
To create a class in Java, you use the ‘class’ keyword followed by the name of the class, for example
public class MyClass
. The class body, enclosed between braces, can contain fields, methods, and constructors.
Here’s a simple example:
public class MyClass {
// fields, methods, and constructors go here
}
In this example, we’ve created a basic Java class named MyClass
. The class body is currently empty, but this is where you would define any fields, methods, or constructors that your class needs.
This is just the beginning of what you can do with Java classes. Continue reading to learn more about the different components of a Java class and how to use them effectively.
Table of Contents
- Creating a Basic Java Class
- Exploring Advanced Concepts in Java Classes
- Exploring Interfaces and Abstract Classes in Java
- Troubleshooting Common Issues with Java Classes
- Understanding Object-Oriented Programming and Java Classes
- Utilizing Java Classes in Larger Applications
- Wrapping Up: Mastering Java Classes
Creating a Basic Java Class
Creating a class in Java is quite straightforward. Let’s break it down into three main steps: declaring fields, creating methods, and defining constructors.
Declaring Fields
Fields are variables that hold data within a class. They represent the state of an object. Here’s how to declare a field in a Java class:
public class MyClass {
String myField;
}
In this example, we’ve declared a field myField
of type String
in our MyClass
.
Creating Methods
Methods in Java are blocks of code that perform a specific task. They are where the logic of the class is defined. Here’s a simple example of creating a method in a Java class:
public class MyClass {
String myField;
void myMethod() {
System.out.println('Hello, world!');
}
}
In this example, we’ve added a method myMethod
that prints ‘Hello, world!’ to the console.
Defining Constructors
A constructor in Java is a special method that is used to initialize objects. It is called when an object of a class is created. Here’s an example of defining a constructor in a Java class:
public class MyClass {
String myField;
MyClass() {
myField = 'Hello, world!';
}
void myMethod() {
System.out.println(myField);
}
}
In this example, we’ve added a constructor that initializes myField
with the string ‘Hello, world!’. Now, whenever an object of MyClass
is created, myField
will be initialized with this value.
These are the basic components of a Java class. In the next section, we’ll dive into more advanced concepts.
Exploring Advanced Concepts in Java Classes
Java classes are not just about declaring fields, creating methods, and defining constructors. They also include more advanced concepts such as inheritance, polymorphism, and encapsulation. Let’s delve deeper into these concepts.
Inheritance in Java Classes
Inheritance is a mechanism in Java that allows one class to inherit the fields and methods of another class. The class which inherits the properties of another class is known as the subclass (or derived class), and the class whose properties are inherited is known as the superclass (or base class).
Here’s a simple example of inheritance in Java:
public class MyBaseClass {
void myMethod() {
System.out.println('Hello from MyBaseClass!');
}
}
public class MyDerivedClass extends MyBaseClass {
}
MyDerivedClass obj = new MyDerivedClass();
obj.myMethod();
// Output:
// 'Hello from MyBaseClass!'
In this example, MyDerivedClass
is a subclass of MyBaseClass
and inherits its myMethod
method. When we create an object of MyDerivedClass
and call myMethod
, it prints ‘Hello from MyBaseClass!’.
Polymorphism in Java Classes
Polymorphism in Java is a concept by which we can perform a single action in different ways. We can process objects differently based on their data type. In other words, polymorphism allows us to define one interface and have multiple implementations.
Here’s an example of polymorphism in Java:
public class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
}
public class Cat extends Animal {
@Override
void sound() {
System.out.println('The cat says: Meow!');
}
}
public class Dog extends Animal {
@Override
void sound() {
System.out.println('The dog says: Woof!');
}
}
Animal myCat = new Cat();
Animal myDog = new Dog();
myCat.sound();
myDog.sound();
// Output:
// 'The cat says: Meow!'
// 'The dog says: Woof!'
In this example, Cat
and Dog
classes extend Animal
class and override its sound
method. When we create myCat
and myDog
objects of type Animal
but instantiate them as Cat
and Dog
, and then call sound
, it produces different sounds.
Encapsulation in Java Classes
Encapsulation in Java is a mechanism of wrapping the data (variables) and code acting on the data (methods) together as a single unit. In encapsulation, the variables of a class will be hidden from other classes, and can be accessed only through the methods of their current class.
Here’s an example of encapsulation in Java:
public class EncapsulatedClass {
private String hiddenField;
public String getHiddenField() {
return hiddenField;
}
public void setHiddenField(String hiddenField) {
this.hiddenField = hiddenField;
}
}
EncapsulatedClass obj = new EncapsulatedClass();
obj.setHiddenField('Hello, world!');
System.out.println(obj.getHiddenField());
// Output:
// 'Hello, world!'
In this example, hiddenField
is private and can only be accessed through the getHiddenField
and setHiddenField
methods of EncapsulatedClass
. This is a basic example of encapsulation in Java.
These advanced concepts are key to mastering Java classes and object-oriented programming in Java.
Exploring Interfaces and Abstract Classes in Java
While regular classes are powerful tools in Java, there are situations where alternative approaches such as interfaces and abstract classes may be more appropriate. Let’s dive into these concepts.
Understanding Interfaces in Java
Interfaces in Java are a blueprint of a class. They are used when we need to ensure that certain methods are present in a class.
Here’s a simple example of an interface in Java:
interface MyInterface {
void myMethod();
}
public class MyClass implements MyInterface {
@Override
public void myMethod() {
System.out.println('Hello from MyClass!');
}
}
MyClass obj = new MyClass();
obj.myMethod();
// Output:
// 'Hello from MyClass!'
In this example, MyClass
implements MyInterface
and is therefore required to implement the myMethod
method. When we create an object of MyClass
and call myMethod
, it prints ‘Hello from MyClass!’.
Delving into Abstract Classes
Abstract classes in Java are classes that contain one or more abstract methods, which are methods declared without an implementation. They cannot be instantiated, and require subclasses to provide implementations for the abstract methods.
Here’s an example of an abstract class in Java:
abstract class MyAbstractClass {
abstract void myMethod();
}
public class MyClass extends MyAbstractClass {
@Override
public void myMethod() {
System.out.println('Hello from MyClass!');
}
}
MyClass obj = new MyClass();
obj.myMethod();
// Output:
// 'Hello from MyClass!'
In this example, MyClass
extends MyAbstractClass
and is therefore required to provide an implementation for the myMethod
method. When we create an object of MyClass
and call myMethod
, it prints ‘Hello from MyClass!’.
In conclusion, interfaces and abstract classes can be used as alternative approaches to regular classes in situations where you need to ensure that certain methods are implemented, or when you want to provide a common interface for multiple classes.
Troubleshooting Common Issues with Java Classes
Working with Java classes can sometimes lead to unexpected issues, especially when dealing with more complex concepts like inheritance and polymorphism. Let’s discuss some common problems and how to resolve them.
Issues with Inheritance
One common issue with inheritance is the super
keyword’s misuse, which is used to access/call the parent class members (fields and methods).
Let’s consider an example:
public class ParentClass {
String message = 'Hello from ParentClass!';
}
public class ChildClass extends ParentClass {
String message = 'Hello from ChildClass!';
void printMessage() {
System.out.println(message); // This will print 'Hello from ChildClass!'
System.out.println(super.message); // This will print 'Hello from ParentClass!'
}
}
ChildClass obj = new ChildClass();
obj.printMessage();
// Output:
// 'Hello from ChildClass!'
// 'Hello from ParentClass!'
In this example, both ParentClass
and ChildClass
have a field named message
. When we call printMessage
on an object of ChildClass
, it prints both messages. The super
keyword is used to refer to the message
field in ParentClass
.
Polymorphism Pitfalls
A common issue with polymorphism is misunderstanding which methods are accessible via an object reference. Remember, only the methods defined in the reference type can be called directly, even if the actual object has more methods due to overriding or additional methods in the subclass.
Here’s an example to illustrate this:
public class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
}
public class Cat extends Animal {
@Override
void sound() {
System.out.println('The cat says: Meow!');
}
void purr() {
System.out.println('The cat purrs.');
}
}
Animal myCat = new Cat();
myCat.sound(); // This will work
myCat.purr(); // This will NOT work
// Output:
// 'The cat says: Meow!'
// Error: The method purr() is undefined for the type Animal
In this example, myCat
is of type Animal
but is an instance of Cat
. While we can call the sound
method, we can’t call the purr
method directly on myCat
because purr
is not a method in the Animal
class.
Remember, understanding the principles of Java classes, inheritance, and polymorphism can help you navigate and troubleshoot these common issues. Always consider the class hierarchy and the scope of variables and methods when working with Java classes.
Understanding Object-Oriented Programming and Java Classes
Object-Oriented Programming (OOP) is a programming paradigm that uses objects and classes. It simplifies software development and maintenance by providing some concepts:
- Object: An object is an instance of a class. It has a state and behavior. The state is stored in fields (variables), while the behavior is shown via methods (functions).
Class: A class is a blueprint or prototype from which objects are created. It represents a set of properties or methods that are common to all objects of one type.
Here’s a simple example of creating an object from a class in Java:
public class MyClass {
String myField = 'Hello, world!';
void myMethod() {
System.out.println(myField);
}
}
MyClass obj = new MyClass();
obj.myMethod();
// Output:
// 'Hello, world!'
In this example, MyClass
is a class with a field myField
and a method myMethod
. We create an object obj
from MyClass
and call myMethod
, which prints ‘Hello, world!’. The object obj
has a state (the value of myField
) and behavior (the ability to execute myMethod
).
The Importance of Classes in Java
In Java, everything is object-oriented, and classes are at the heart of it. They are the building blocks of your application. Classes in Java:
- Encapsulate complexity: You can hide complex implementation details behind simple class interfaces.
Promote code reusability: You can use the same class to create many objects, reducing code duplication.
Form the basis for inheritance: You can create new classes from existing ones through inheritance, promoting a hierarchical organization of classes.
Java classes are the backbone of Java’s Object-Oriented Programming. They encapsulate data and methods into a single unit, promoting better organization and reusability of code.
Utilizing Java Classes in Larger Applications
Java classes are not confined to small programs; they form the backbone of larger applications as well. The principles of Object-Oriented Programming (OOP) are applicable at all levels, from micro to macro. Understanding how to use classes effectively can significantly enhance your programming skills and open doors to more complex and robust applications.
Java Packages and Class Organization
Java packages are used for grouping related classes and interfaces together. They help in organizing your code and avoiding naming conflicts. For instance, you could have a package for all your entity classes, another for utility classes, and so on. This structure helps in maintaining a clean and organized codebase which is extremely beneficial when working on larger applications.
package com.myapp.entities;
public class MyClass {
// Class implementation
}
In this example, MyClass
is part of the com.myapp.entities
package. Organizing classes into packages is a common practice in large Java applications.
Exception Handling in Java
Exception handling is a powerful mechanism that allows you to handle runtime errors so that normal flow of the application can be maintained. Java provides several built-in classes for handling exceptions, and you can also create your own exception classes by extending the Exception
class.
public class MyClass {
void myMethod() {
try {
// Risky code
} catch (Exception e) {
// Handle exception
}
}
}
In this example, any exceptions that occur in the risky code block are caught and handled in the catch block. Proper exception handling is crucial in larger applications to ensure a smooth user experience.
Further Resources for Mastering Java Classes
If you’re interested in diving deeper into Java classes and related concepts, here are some resources to explore:
- Java’s Role in Web Development: What Is It Used For? – Understand Java’s usage and the fundamentals of its classes.
Java Collections: Overview – Explore the Java Collections Framework for storing and manipulating groups of objects.
Data Structures Usage in Java – Master using Java data structures for efficient data organization and manipulation.
Oracle’s Java Tutorials covers all aspects of Java programming, including classes and objects.
Baeldung’s Guide to Java Classes covers everything from basic class structure to more advanced topics.
GeeksforGeeks Java Programming Language section holds numerous articles on Java programming, classes, and objects.
Wrapping Up: Mastering Java Classes
In this comprehensive guide, we’ve explored the ins and outs of Java classes, a fundamental concept in Java and object-oriented programming.
We started with the basics, learning how to create a class in Java, including declaring fields, creating methods, and defining constructors. We then delved into more advanced concepts such as inheritance, polymorphism, and encapsulation, providing you with a deeper understanding of Java classes and their capabilities.
Along the way, we tackled common issues that you might encounter when using Java classes, providing solutions and best practices for each issue. We also looked at alternative approaches to regular classes, exploring interfaces and abstract classes, and their appropriate use cases.
Concept | Description |
---|---|
Regular Classes | Basic building blocks, encapsulate data and methods |
Interfaces | Blueprint of a class, ensures certain methods are implemented |
Abstract Classes | Cannot be instantiated, requires subclasses to provide implementations |
Whether you’re just starting out with Java or looking to level up your programming skills, we hope this guide has given you a deeper understanding of Java classes and their importance in object-oriented programming.
The ability to create and manipulate classes is a powerful tool in Java programming. With a solid understanding of classes, inheritance, polymorphism, and encapsulation, you’re well equipped to tackle more complex Java projects. Happy coding!