Python Random Module | Usage Guide (With Examples)
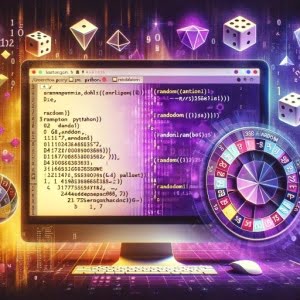
Ever felt puzzled by the concept of randomness in Python? You’re not alone. Just as the outcome of a dice roll adds an element of unpredictability to a board game, Python’s random
module introduces a similar sense of chance into your code.
This comprehensive guide is designed to help you navigate the labyrinth of Python’s random
module. From the basic usage to advanced techniques, we’ll explore it all, helping you to master randomness in Python.
TL;DR: How Can I Generate a Random Number in Python?
You can utilize Python’s
random
module to generate random numbers. Here’s a straightforward example:
import random
number = random.randint(1, 10)
print(number)
# Output:
# (A random integer between 1 and 10 will be printed)
The code above imports the random
module and then uses the randint
function to generate a random integer between 1 and 10. The resultant number is then printed to the console.
Intrigued? There’s a lot more to Python’s
random
module than meets the eye. Keep reading to delve deeper into the intricacies of generating randomness in Python!
Table of Contents
- Generating Random Numbers: The Basics
- Harnessing the Power of Python’s Random Module
- Exploring Alternatives: Numpy and Secrets
- Navigating Potential Pitfalls: Troubleshooting Python’s Random Module
- Understanding Randomness: The Heart of Python’s Random Module
- The Power of Randomness: Beyond Numbers
- Broadening Your Horizons: Delving Deeper into Randomness
- Decoding Randomness in Python: A Recap
Generating Random Numbers: The Basics
Python’s random
module is a powerful tool for generating random numbers. At its simplest, you can use it to generate a random integer within a specified range. Here’s how:
import random
# Generate a random integer between 1 and 100
random_number = random.randint(1, 100)
print(random_number)
# Output:
# (A random integer between 1 and 100 will be printed)
In the code block above, we first import the random
module. We then use the randint
function from the random
module to generate a random integer between 1 and 100. The resulting number is then printed to the console.
This basic use of the random
module is straightforward and easy to understand. However, it’s important to note that the randint
function generates pseudo-random numbers. This means that while the numbers appear random, they are generated by a deterministic process and will produce the same sequence of numbers if the random number generator is initialized with the same seed.
Despite this, for many applications, pseudo-random numbers are more than sufficient. They can be used to add a degree of unpredictability to your Python programs, be it for game development, simulations, or a wide range of other applications.
Harnessing the Power of Python’s Random Module
Beyond generating simple random integers, Python’s random
module provides a host of other functionalities. Let’s explore some of them.
Generating Random Floats
The random
module can generate random floating-point numbers between 0.0 and 1.0. Here’s how you can do it:
import random
# Generate a random float between 0.0 and 1.0
random_float = random.random()
print(random_float)
# Output:
# (A random float between 0.0 and 1.0 will be printed)
In the code block above, the random
function from the random
module is used to generate a random float between 0.0 and 1.0.
Choosing Random Elements from a List
Python’s random
module can also be used to pick a random element from a list. Here’s how:
import random
# Define a list
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
# Pick a random fruit
random_fruit = random.choice(fruits)
print(random_fruit)
# Output:
# (A random fruit from the list will be printed)
The choice
function randomly selects an element from the provided list.
Shuffling a List Randomly
The random
module can also shuffle the elements of a list in a random order. Here’s an example:
import random
# Define a list
numbers = [1, 2, 3, 4, 5]
# Shuffle the list
random.shuffle(numbers)
print(numbers)
# Output:
# (The list of numbers shuffled in a random order will be printed)
The shuffle
function rearranges the elements of the list in a random order. Each run of the code will most likely result in a different order of elements.
These advanced functionalities of the random
module allow for greater flexibility and complexity in generating randomness in Python.
Exploring Alternatives: Numpy and Secrets
Python’s random
module is a powerful tool, but it’s not the only game in town. Let’s delve into some alternative methods to generate random numbers in Python, specifically focusing on the numpy
library and the secrets
module.
Generating Random Numbers with Numpy
The numpy
library is a popular tool in the world of data science, and it includes functions for generating random numbers. Here’s how you can use it:
import numpy as np
# Generate a random float between 0.0 and 1.0
random_float = np.random.rand()
print(random_float)
# Output:
# (A random float between 0.0 and 1.0 will be printed)
In the code block above, the rand
function from the numpy.random
module is used to generate a random float between 0.0 and 1.0. numpy
provides more sophisticated random number generation capabilities, including the ability to generate arrays of random numbers, and to generate numbers according to different statistical distributions.
Cryptographically Secure Random Numbers with Secrets
For applications where security is paramount, such as cryptography, Python provides the secrets
module. This module generates random numbers that are cryptographically secure, making them suitable for generating passwords, tokens, or secret keys for encryption.
import secrets
# Generate a random integer between 1 and 100
secure_random_number = secrets.randbelow(101)
print(secure_random_number)
# Output:
# (A cryptographically secure random integer between 1 and 100 will be printed)
In the code block above, the randbelow
function from the secrets
module is used to generate a cryptographically secure random integer between 1 and 100.
While these alternative methods have their benefits, they also have their drawbacks. numpy
requires an additional library to be installed and may be overkill for simple applications. The secrets
module, while providing enhanced security, is slower than the random
module and may not be necessary for applications where security is not a concern.
While Python’s random
module is incredibly useful, it’s important to be aware of some common issues and considerations when using it.
The Quest for True Randomness
One common issue is the quest for truly random numbers. As mentioned earlier, the random
module generates pseudo-random numbers, which are deterministic and not truly random. This is generally not an issue for most applications, but in scenarios where true randomness is required, such as in cryptography, this can be a problem.
import random
random.seed(1)
print(random.random())
random.seed(1)
print(random.random())
# Output:
# 0.13436424411240122
# 0.13436424411240122
In the code block above, we seed the random number generator with a fixed value using random.seed()
. When we generate a random number after setting the seed, we get the same number each time. This illustrates that the numbers generated by the random
module are not truly random.
Pseudo-random vs True Random
While pseudo-random numbers generated by the random
module are sufficient for most applications, for some use cases, such as cryptography or simulations requiring high-quality randomness, true random numbers might be necessary. In such cases, Python’s secrets
module or third-party libraries that generate true random numbers could be alternatives to consider.
In conclusion, while Python’s random
module is a powerful tool for generating random numbers, it’s important to understand its limitations and potential issues. By being aware of these considerations, you can make more informed decisions when working with randomness in Python.
Understanding Randomness: The Heart of Python’s Random Module
Before we delve deeper into Python’s random
module, it’s important to understand the fundamental concepts of randomness and pseudo-randomness, and how they’re implemented in Python.
The Essence of Randomness
Randomness, in its pure form, refers to the concept of unpredictability. In the context of programming and Python, randomness is the ability to generate data, strings, or, more commonly, numbers, that cannot be predicted logically.
Pseudo-randomness: A Closer Look
In contrast, pseudo-random numbers, while appearing random, are generated in a predictable manner using a mathematical formula. This formula, or algorithm, is deterministic, meaning it will produce the same sequence of numbers if it is initialized with the same seed.
import random
random.seed(1)
print(random.random()) # Output: 0.13436424411240122
random.seed(1)
print(random.random()) # Output: 0.13436424411240122
In the code block above, we seed the random number generator with a fixed value using random.seed()
. When we generate a random number after setting the seed, we get the same number each time. This illustrates that the numbers generated by the random
module are not truly random, but pseudo-random.
Under the Hood: Python’s Random Module
Python’s random
module uses the Mersenne Twister algorithm, a pseudo-random number generator algorithm, to generate random numbers. This algorithm is known for its long period (the sequence of numbers it generates before repeating), high-quality randomness, and computational efficiency.
While the random
module’s numbers aren’t truly random, they are sufficient for most applications that require randomness, including simulations, games, and many more. Understanding the underlying principles of randomness and pseudo-randomness allows us to better appreciate the power and limitations of Python’s random
module.
The Power of Randomness: Beyond Numbers
Randomness plays a vital role in various applications, extending far beyond simply generating random numbers. Let’s explore the relevance of randomness in a few key areas.
Simulations and Games
In simulations and games, randomness can be used to mimic the unpredictability of real-world scenarios. Whether it’s simulating the roll of a dice in a digital board game or generating random events in a simulation, Python’s random
module can be a valuable tool.
Cryptography
In cryptography, randomness is essential for creating keys that are hard to predict. Python’s random
module, and more specifically the secrets
module, can be used to generate these cryptographically secure random numbers.
import secrets
# Generate a random 16-byte key for encryption
key = secrets.token_bytes(16)
print(key)
# Output:
# (A random 16-byte key will be printed)
In the code block above, the token_bytes
function from the secrets
module is used to generate a 16-byte key for encryption.
Broadening Your Horizons: Delving Deeper into Randomness
While we’ve covered a lot of ground, there’s still a lot more to explore when it comes to randomness. If you’re interested in delving deeper, you might want to explore concepts like probability distributions and Monte Carlo simulations. These topics offer a more advanced look at randomness and can provide you with more sophisticated tools for generating and working with random numbers.
Further Resources for Python Modules
Here are a few resources available online that can help you further your understanding of randomness and its implementation in Python:
- Python Modules: Your Key to Efficiency – Dive deep into importing modules and managing dependencies.
Generating Random Integers in Python: randint() – Learn how to generate random integers in Python with “randint” function.
Simplifying Random Data Generation in Python – Discover how to create random data and distributions in Python.
Python Random Seed Method – W3Schools’ reference guide on Python’s random.seed method for initializing random number generators.
Python Random Module Tutorial – A detailed video tutorial that introduces the Python random module.
Python’s Random Module: W3Schools Guide – An easy-to-follow guide by W3Schools that delves into the functionality and usage of Python’s random module.
Remember, the journey of learning never ends. Happy exploring!
Decoding Randomness in Python: A Recap
Throughout this comprehensive guide, we’ve explored the depths of Python’s random
module, uncovering its functions, common issues, and solutions. We’ve seen how to generate random integers and floats, select random elements from a list, and shuffle a list randomly. We also delved into the difference between pseudo-random and true random, and the implications of this difference.
import random
random_number = random.randint(1, 100) # Generates a random integer
random_float = random.random() # Generates a random float
random_element = random.choice(['apple', 'banana', 'cherry']) # Picks a random element
# Output:
# (Random integer, float, and element will be printed)
We also discussed alternative approaches to generating random numbers in Python, such as using the numpy
library for more sophisticated random number generation, and the secrets
module for generating cryptographically secure random numbers.
import numpy as np
random_float = np.random.rand() # Generates a random float
import secrets
secure_random_number = secrets.randbelow(101) # Generates a secure random number
# Output:
# (Random float and secure random number will be printed)
In conclusion, Python’s random
module, along with its alternatives, provides a versatile toolkit for handling randomness in Python. Whether you’re developing games, running simulations, or working on cryptography, understanding how to generate and work with random numbers in Python is a valuable skill.