Using Python’s randint for Random Number Generation
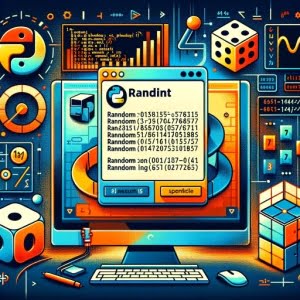
Python’s randint function is a powerful tool in your coding arsenal. It’s like a digital dice, capable of generating random numbers for a variety of applications.
This function can seem a bit confusing at first, but fear not! In this guide, we will dive deep into the ins and outs of using randint in Python. By the end of this guide, you will be able to confidently use the randint function in your own Python projects.
So, let’s roll the dice and start our journey into the world of Python’s randint function.
TL;DR: How Do I Use the randint Function in Python?
The randint function is part of Python’s random module, and it’s used to generate a random integer within a specified range. Here’s a simple example:
import random
number = random.randint(1, 10)
print(number)
# Output:
# (A random number between 1 and 10)
In this example, we’re using Python’s random.randint()
function to generate a random number between 1 and 10. The import random
line at the beginning is necessary because randint is part of the random module in Python. The function random.randint(1, 10)
then generates a random integer within the range of 1 and 10.
If you’re interested in learning more about the randint function, including its more advanced uses and potential issues you might encounter, keep reading for a comprehensive exploration.
Table of Contents
- Understanding Python’s randint Function
- Expanding the Range of randint
- Using randint in Loops
- Exploring Alternatives to randint
- Common Issues and Solutions with randint
- Best Practices with randint
- Understanding Random Number Generation in Python
- Real-World Applications of Python’s randint
- Further Reading: Probability Theory and Statistical Analysis
- Wrapping Up: Python’s randint Function
Understanding Python’s randint Function
Python’s randint()
is a function that belongs to the random
module. It is used to generate a random integer within a defined range. The function takes two parameters: the start and end of the range, inclusive.
Using randint: A Simple Example
Let’s look at a simple code example to understand how randint()
works:
import random
number = random.randint(1, 10)
print(number)
# Output:
# (A random number between 1 and 10)
In this example, import random
is used to import the random module, which contains the randint function. Next, random.randint(1, 10)
is used to generate a random integer between 1 and 10, inclusive. The result is then stored in the variable number
, which is printed out.
Parameters and Return Value
The randint(a, b)
function takes two parameters:
a
: The lower limit of the range (inclusive).b
: The upper limit of the range (inclusive).
The function returns a random integer N
such that a <= N <= b
.
Understanding the Output
The output of the randint()
function is a random integer within the specified range. In our example, the output is a random number between 1 and 10. Each time you run the code, you might get a different number because the selection is random.
By understanding the basics of Python’s randint()
function, you can start to harness the power of random number generation in your coding projects.
Expanding the Range of randint
Python’s randint()
function isn’t limited to small ranges. In fact, you can generate random integers in any range you like. For example, if you’re simulating a lottery draw, you might need to generate numbers between 1 and 1000:
import random
lottery_number = random.randint(1, 1000)
print(lottery_number)
# Output:
# (A random number between 1 and 1000)
In this example, we’ve expanded the range of randint()
to generate a random number between 1 and 1000. This demonstrates the flexibility of the randint()
function.
Using randint in Loops
One of the powerful ways to use randint()
is within loops. This allows you to generate multiple random numbers at once. For instance, if you need to generate a list of 5 random numbers between 1 and 10, you can use a for loop with randint()
:
import random
random_numbers = [random.randint(1, 10) for _ in range(5)]
print(random_numbers)
# Output:
# (A list of 5 random numbers between 1 and 10)
In this code, we’re using a for loop to generate a list of 5 random numbers. The random.randint(1, 10)
function is called 5 times, once for each iteration of the loop, generating a new random number each time. The result is a list of 5 random integers.
These examples demonstrate how you can use Python’s randint()
function in more complex ways to suit your needs. By adjusting the range and using loops, you can generate a variety of random number sequences.
Exploring Alternatives to randint
While randint()
is a powerful tool for generating random integers, Python offers other functions and modules for random number generation. These include the random()
function, the uniform()
function, and the NumPy module.
The random Function
The random()
function is another part of Python’s random module. Unlike randint()
, random()
generates a random floating-point number between 0.0 and 1.0.
import random
random_number = random.random()
print(random_number)
# Output:
# (A random floating-point number between 0.0 and 1.0)
The random()
function doesn’t take any arguments and returns a random float in the range [0.0, 1.0).
The uniform Function
The uniform(a, b)
function generates a random floating-point number between a
and b
. It’s similar to randint()
, but it works with floating-point numbers.
import random
random_number = random.uniform(1.0, 10.0)
print(random_number)
# Output:
# (A random floating-point number between 1.0 and 10.0)
In this example, random.uniform(1.0, 10.0)
generates a random float between 1.0 and 10.0.
The NumPy Module
NumPy, a powerful library for numerical computation in Python, also provides functions for random number generation. For instance, numpy.random.randint()
generates random integers in a similar way to Python’s randint()
.
import numpy
random_number = numpy.random.randint(1, 10)
print(random_number)
# Output:
# (A random integer between 1 and 10)
In this example, numpy.random.randint(1, 10)
generates a random integer between 1 and 10, just like Python’s randint()
function.
These alternative methods offer more flexibility and options for random number generation in Python. Depending on your specific needs, you might find one of these methods more suitable than randint()
.
Common Issues and Solutions with randint
While using Python’s randint()
function, you might encounter certain issues. Let’s discuss some common problems and their solutions.
Forgetting to Import the Random Module
One of the most common mistakes is forgetting to import the random module. Without importing it, Python won’t recognize the randint()
function, leading to an error.
number = randint(1, 10)
print(number)
# Output:
# NameError: name 'randint' is not defined
In this example, we forgot to import the random module, so Python raises a NameError
because it doesn’t recognize randint()
. The solution is to import the random module at the beginning of your code.
import random
number = random.randint(1, 10)
print(number)
# Output:
# (A random number between 1 and 10)
By importing the random module with import random
, we can now use the randint()
function without any issues.
Using Incorrect Range Values
Another common mistake is using incorrect values for the range. Remember, the first parameter should be less than or equal to the second. If it’s greater, Python will raise a ValueError
.
import random
number = random.randint(10, 1)
print(number)
# Output:
# ValueError: empty range for randrange() (10,1, -9)
In this example, we tried to generate a random number between 10 and 1, which is an empty range. The solution is to ensure the first parameter is less than or equal to the second.
Best Practices with randint
When using randint()
, always import the random module at the beginning of your code. Ensure the range values are correct, with the first value less than or equal to the second. By following these best practices, you can avoid common issues and use randint()
effectively in your Python projects.
Understanding Random Number Generation in Python
Random number generation is a fundamental concept in programming that has a variety of applications, from game development to data analysis. Python’s random
module, which includes the randint()
function, is a powerful tool for generating these random numbers.
The Role of Python’s Random Module
Python’s random
module provides a suite of functions for generating random numbers. These functions include randint()
, random()
, uniform()
, and many others. Each function generates a random number in a different way or within a different range.
import random
random_integer = random.randint(1, 10)
random_float = random.random()
random_uniform = random.uniform(1.0, 10.0)
print(random_integer, random_float, random_uniform)
# Output:
# (A random integer between 1 and 10, a random float between 0.0 and 1.0, a random float between 1.0 and 10.0)
In this code, we’re using three functions from Python’s random
module to generate different types of random numbers. Each function provides a unique way to generate random numbers, making the random
module a versatile tool for random number generation in Python.
The Importance of Randomness in Programming
Randomness plays a crucial role in many areas of programming. For instance, in game development, randomness can be used to create unpredictable gameplay elements. In data analysis, random sampling can help ensure a representative sample of data. By understanding how to generate random numbers in Python, you can harness the power of randomness in your own programming projects.
Real-World Applications of Python’s randint
Python’s randint()
function isn’t just for academic exercises—it has practical applications in real-world scenarios. Let’s explore some of these applications.
Simulations
In simulations, randint()
can be used to generate random inputs. For example, in a weather simulation, randint()
could generate random temperatures or wind speeds.
import random
random_temperature = random.randint(-10, 40)
print('Random Temperature:', random_temperature, '°C')
# Output:
# Random Temperature: (A random number between -10 and 40) °C
In this code, we’re using randint()
to generate a random temperature between -10 and 40 degrees Celsius.
Games
In games, randint()
can be used to create unpredictable elements, making the game more exciting. For instance, in a dice game, randint()
could be used to generate the dice roll.
import random
dice_roll = random.randint(1, 6)
print('Dice Roll:', dice_roll)
# Output:
# Dice Roll: (A random number between 1 and 6)
In this code, we’re using randint()
to simulate a dice roll, generating a random number between 1 and 6.
Data Analysis
In data analysis, randint()
can be used to generate random samples from a larger dataset. This can ensure a more representative sample and more accurate analysis.
Further Reading: Probability Theory and Statistical Analysis
If you’re interested in the theory behind random number generation, consider studying probability theory. And if you want to apply random number generation in data analysis, look into statistical analysis in Python. Both topics will provide a deeper understanding of the power and potential of Python’s randint()
function.
Further Resources for Python Modules
For a more profound understanding of Python Modules, we have gathered several insightful resources for you:
- Python Modules Fundamentals Covered – Dive deep into Python’s module caching and reload mechanisms.
Implementing Queues in Python – Dive into various queue types, including FIFO and LIFO, in Python.
Simplifying Random Data Generation in Python – Learn how to add randomness to your Python programs with “random.”
Python’s Random Module – Learn about the random module and generating random numbers with this Programiz guide.
How to Create Random Numbers in Python – A Medium article that delves into generating random numbers in Python.
Python’s Random Tutorial – A tutorial by Real Python covering topics related to generating random numbers in Python.
Explore these resources, and you’ll be taking another stride towards expertise in Python and taking your coding abilities to the next level.
Wrapping Up: Python’s randint Function
Python’s randint()
function is a powerful tool in the random
module, providing a straightforward way to generate random integers within a specified range. From simple applications to more complex scenarios, randint()
offers a reliable solution for random number generation.
While randint()
is generally easy to use, common issues include forgetting to import the random
module and using incorrect range values. These can be easily avoided by following best practices such as always importing necessary modules and ensuring correct parameter values.
Beyond randint()
, Python offers other methods for random number generation. These include the random()
and uniform()
functions in the random
module, and the numpy.random.randint()
function in the NumPy module. Each method has its unique advantages and can be more suitable depending on your specific needs.
Random number generation is a fundamental aspect of programming with diverse applications. By mastering Python’s randint()
function and understanding other random number generation methods, you can harness the power of randomness in your Python projects.