Java Enum with Custom Values: A Detailed Guide
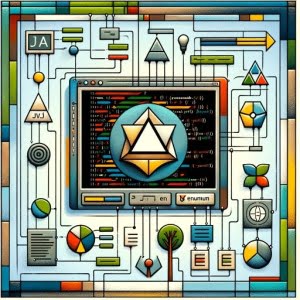
Are you finding it challenging to use enums with values in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling enums with values in Java, but we’re here to help.
Think of Java enums as a class that has a fixed set of constants. These constants can be assigned values, making enums a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of defining, assigning, and using values with enums in Java, from the basics to more advanced techniques, as well as alternative approaches.
Let’s get started and master Java enums with values!
TL;DR: How Do I Use Enums with Values in Java?
In Java, you can assign values to enums by defining a constructor and a private variable in the enum. For example an enum for Seasons of the year will have 4 constants:
public enum Season {WINTER, SPRING, SUMMER, FALL}
. This allows you to associate specific values with each enum constant.
Here’s a simple example:
public enum Day {
MONDAY(1),
TUESDAY(2);
private int value;
private Day(int value) {
this.value = value;
}
}
# Output:
# MONDAY = 1
# TUESDAY = 2
In this example, we’ve defined an enum Day
with two constants: MONDAY
and TUESDAY
. Each constant is assigned a specific value through the enum’s constructor. The private variable value
holds the value for each constant.
This is just a basic way to use enums with values in Java, but there’s much more to learn about using enums effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Defining and Using Enums with Values in Java
- Advanced Enums: Switch Statements, Collections, and Methods
- Alternative Techniques with Enums & Values
- Troubleshooting Enums with Values in Java
- Understanding Enums and Values in Java
- Applying Enums with Values in Larger Projects
- Wrapping Up: Mastering Java Enums with Values
Defining and Using Enums with Values in Java
Enums in Java are a type of class that have a fixed number of instances. These instances, also known as constants, can be assigned values. This can be done by defining a constructor and a private variable in the enum.
The constructor allows us to initialize the enum constants with specific values, while the private variable holds these values.
Let’s see how we can use this concept with a simple example:
public enum Day {
MONDAY(1),
TUESDAY(2),
WEDNESDAY(3),
THURSDAY(4),
FRIDAY(5),
SATURDAY(6),
SUNDAY(7);
private int value;
private Day(int value) {
this.value = value;
}
public int getValue() {
return this.value;
}
}
# Output:
# MONDAY = 1
# TUESDAY = 2
# WEDNESDAY = 3
# THURSDAY = 4
# FRIDAY = 5
# SATURDAY = 6
# SUNDAY = 7
In this example, we’ve created an enum Day
with seven constants, each representing a day of the week. Each day is assigned a value from 1 to 7 through the enum’s constructor. The private variable value
is used to store these values.
We’ve also added a method getValue()
that returns the value of a particular day. This is how we access the values assigned to the enum constants.
This is the basic use of enums with values in Java. It’s a simple and effective way to associate specific values with enum constants.
Advanced Enums: Switch Statements, Collections, and Methods
Now that we’ve covered the basics, let’s dive into some more complex uses of enums with values in Java. We’ll explore how to use enums in switch statements, collections, and methods.
Enums in Switch Statements
Enums can be used in switch statements to control program flow based on the enum constant.
public class Main {
public static void main(String[] args) {
Day day = Day.MONDAY;
switch(day) {
case MONDAY:
System.out.println("Start of the work week.");
break;
case FRIDAY:
System.out.println("End of the work week.");
break;
default:
System.out.println("Midweek day.");
break;
}
}
}
# Output:
# Start of the work week.
In this code, we’ve used the enum Day
in a switch statement. The output will depend on the value of day
.
Enums in Collections
Enums can also be used in collections like EnumSet and EnumMap.
import java.util.EnumSet;
public class Main {
public static void main(String[] args) {
EnumSet<Day> weekend = EnumSet.of(Day.SATURDAY, Day.SUNDAY);
System.out.println(weekend);
}
}
# Output:
# [SATURDAY, SUNDAY]
In this example, we’ve used EnumSet
to create a collection of enum constants. The EnumSet
weekend
contains the constants SATURDAY
and SUNDAY
.
Enums in Methods
Finally, let’s see how we can use enums in methods.
public class Main {
public static void printDayValue(Day day) {
System.out.println(day + " = " + day.getValue());
}
public static void main(String[] args) {
printDayValue(Day.MONDAY);
}
}
# Output:
# MONDAY = 1
In the above code, we’ve created a method printDayValue()
that takes an enum constant as a parameter and prints the constant along with its value.
These examples showcase the versatility of enums with values in Java, and how they can be used in various scenarios to make your code more efficient and readable.
Alternative Techniques with Enums & Values
Java provides several alternative approaches to using enums with values. Two of these include the EnumSet
and EnumMap
classes.
Leveraging EnumSet
EnumSet
is a specialized set implementation for use with enum types. It’s compact and efficient for enum constants.
import java.util.EnumSet;
public class Main {
public static void main(String[] args) {
EnumSet<Day> workDays = EnumSet.range(Day.MONDAY, Day.FRIDAY);
System.out.println(workDays);
}
}
# Output:
# [MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY]
In this example, we’ve created an EnumSet
named workDays
that includes the range of enum constants from MONDAY
to FRIDAY
. EnumSet
provides a high-performance set implementation for enum types.
Utilizing EnumMap
EnumMap
is a specialized map implementation for use with enum type keys. It maintains an order that corresponds to the order of the enum constants.
import java.util.EnumMap;
public class Main {
public static void main(String[] args) {
EnumMap<Day, String> dayMood = new EnumMap<>(Day.class);
dayMood.put(Day.MONDAY, "Sleepy");
dayMood.put(Day.FRIDAY, "Excited");
System.out.println(dayMood);
}
}
# Output:
# {MONDAY=Sleepy, FRIDAY=Excited}
In this code, we’ve created an EnumMap
named dayMood
that associates a String
mood with each Day
. EnumMap
provides a high-performance map implementation for enum keys.
These alternative approaches offer flexibility when working with enums. They can be more efficient and provide additional functionality compared to traditional methods. However, they may also be more complex and less familiar to some developers. Therefore, it’s important to consider the trade-offs and choose the approach that best fits your specific needs.
Troubleshooting Enums with Values in Java
Even with a good understanding of enums, you might encounter some common obstacles or errors. Let’s discuss some of these and their solutions.
Enum Constant Not Initialized
One common error is trying to access an enum constant before it’s been initialized. This can result in a NullPointerException
.
public enum Day {
MONDAY(1),
TUESDAY(2);
private int value;
public static final Day DEFAULT_DAY = MONDAY;
private Day(int value) {
this.value = value;
}
}
# Output:
# Exception in thread "main" java.lang.ExceptionInInitializerError
# Caused by: java.lang.NullPointerException
In this example, we tried to initialize DEFAULT_DAY
with MONDAY
before MONDAY
was fully constructed. This results in a NullPointerException
.
The solution is to ensure that enum constants are fully initialized before they are used.
Enum Constants Not Unique
Another common error is defining enum constants that are not unique. This can result in a IllegalArgumentException
.
public enum Day {
MONDAY(1),
TUESDAY(1);
private int value;
private Day(int value) {
this.value = value;
}
}
# Output:
# Exception in thread "main" java.lang.IllegalArgumentException: duplicate key: 1
In this example, we tried to assign the same value to two different enum constants. This results in a IllegalArgumentException
.
The solution is to ensure that each enum constant is assigned a unique value.
Best Practices and Optimization
When using enums with values in Java, it’s important to follow certain best practices for optimal performance and readability.
- Ensure Enum Constants Are Unique: Each enum constant should be unique to avoid confusion and potential errors.
Initialize Enum Constants Before Use: Make sure enum constants are fully initialized before they are used to avoid
NullPointerException
.Use EnumSet and EnumMap for Efficiency:
EnumSet
andEnumMap
provide high-performance set and map implementations for enum types, and can be more efficient than regular sets and maps.Use Meaningful Names for Enum Constants: The names of enum constants should be meaningful and descriptive to improve code readability.
By following these best practices, you can avoid common errors and write efficient, readable code when using enums with values in Java.
Understanding Enums and Values in Java
Before we delve deeper into the use of enums with values in Java, it’s crucial to understand what enums are and why they are used.
What are Enums?
In Java, an enumeration (enum) is a special type of class that represents a group of constants (unchangeable variables, like final variables).
public enum Day {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
In this simple example, Day
is an enum and the days of the week are its constants.
Why Use Enums?
Enums increase code clarity and readability. They limit the possible values that can be assigned to a variable, reducing bugs and making your code more manageable.
What are Values in the Context of Enums?
Values in the context of enums are the specific values that each enum constant represents. These values are assigned to the constants at the time of their declaration.
public enum Day {
MONDAY(1),
TUESDAY(2);
private int value;
private Day(int value) {
this.value = value;
}
public int getValue() {
return this.value;
}
}
# Output:
# MONDAY = 1
# TUESDAY = 2
In this example, MONDAY
and TUESDAY
are enum constants, and 1
and 2
are their respective values. We’ve defined a constructor in the enum to assign these values to the constants.
Understanding these fundamentals of enums and values in Java is essential for using enums effectively in your code, and for understanding the rest of this guide.
Applying Enums with Values in Larger Projects
The use of enums with values can extend far beyond simple examples and small programs. In larger projects, enums with values can be a powerful tool to manage complex data structures, control program flow, and increase code readability.
For instance, in a large software application, you might use enums with values to represent user roles. Each role could be an enum constant, and the values could represent the different permissions associated with each role. This can greatly simplify the process of managing user permissions in your application.
Enums with values can also be useful in data analysis programs. Each enum constant could represent a specific type of data, and the values could represent properties of that data. This can make it easier to manage and analyze large datasets.
Exploring Related Topics
While enums with values are a powerful tool in Java, they are just one part of the language. There are many other topics and concepts that often accompany the use of enums in typical use cases.
Some of these related topics might include other types of enums, such as ordinal enums and enum methods, as well as other Java concepts like classes, interfaces, and exception handling.
Further Resources for Mastering Java Enums
Data types like enum are an integral building block of Java. To learn more about other primitive data types, Click Here for an in-depth Guide.
If you’re interested in learning more about enums and similar topics in Java, here are some external resources that offer more in-depth information:
- Java Integer Maximum Value – Understand the usage of Integer.MAX_VALUE for handling integer bounds checking.
Java Enum Basics – Learn how to declare and use enums for improved type safety and readability.
Java Enums Tutorial by Oracle provides an overview of enums in Java, including syntax, methods, and use cases.
Java Enum Types Tutorial by Baeldung dives deeper into the different types of enums in Java.
Enum Customized Value in Java Guide by GeeksforGeeks offers a deep dive into how to use and customize Enum values in Java.
Wrapping Up: Mastering Java Enums with Values
In this comprehensive guide, we’ve delved into the world of Java enums with values, a powerful feature in Java that allows you to associate specific values with each enum constant.
We began with the basics, learning how to define, assign, and use values with enums in Java. We then explored more advanced uses, such as using enums in switch statements, collections, and methods. We also looked at alternative approaches, including the use of EnumSet and EnumMap, and discussed common issues and best practices when using enums with values.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Use | Simple, easy to understand | Limited functionality |
Advanced Use | More functionality, versatile | More complex |
Alternative Approaches | More efficient, additional functionality | May be less familiar, more complex |
Whether you’re a beginner just starting out with Java enums, or an experienced developer looking to deepen your understanding, we hope this guide has provided you with a thorough understanding of how to use enums with values in Java.
The ability to use enums with values is a powerful tool in Java programming, allowing you to create more efficient, readable, and versatile code. Now, you’re well equipped to leverage this feature in your own projects. Happy coding!