Enum Java: Mastering the Enum Data Type in Java
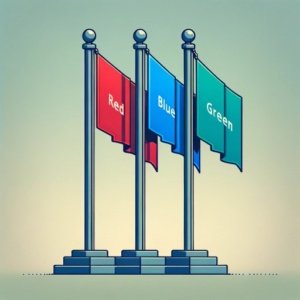
Are you finding it challenging to navigate enums in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling enums in Java, but we’re here to help.
Think of Java’s enums as a switchboard – they can control the flow of your code in a simple and effective way, providing a versatile tool for various tasks.
In this guide, we’ll walk you through the concept of enums in Java, their usage, benefits, and more. We’ll cover everything from the basics of enums to more advanced techniques, as well as alternative approaches.
Let’s get started and master enums in Java!
TL;DR: What is Enum in Java and How to Use It?
Enum in Java is a special data type that enables a variable to be a set of predefined constants, defined with the code
public enum sampleSet{listOfValues}
. The variable must be equal to one of the values that have been predefined for it. It’s a powerful tool for managing fixed sets of constants in your code.
Here’s a simple example:
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY;
}
In this example, we’ve defined an enum named Day
with seven constants representing the days of the week. This Day
enum can now be used in our code to represent a day, and it can only be one of these seven predefined constants.
This is just a basic introduction to enums in Java. There’s much more to learn about how to use them effectively, including more advanced techniques and alternative approaches. Continue reading for a deeper understanding and more advanced usage scenarios.
Table of Contents
Declaring and Using Enums in Java
Enums in Java are declared using the enum
keyword. They are primarily used when we know all possible values at compile time, such as choices on a menu, rounding modes, command line flags, etc. It’s a special data type that enables a variable to be a set of predefined constants. The variable must be equal to one of the values that have been predefined for it.
Let’s look at a simple example of declaring and using enums in Java:
public enum Season {
SPRING, SUMMER, AUTUMN, WINTER;
}
public class EnumTest {
Season season;
public EnumTest(Season season) {
this.season = season;
}
public void tellItLikeItIs() {
switch (season) {
case SPRING:
System.out.println("Time for rebirth!");
break;
case SUMMER:
System.out.println("Fun in the sun!");
break;
case AUTUMN:
System.out.println("Gather and harvest!");
break;
case WINTER:
System.out.println("Stay warm inside!");
break;
default:
System.out.println("Is it a season?");
break;
}
}
public static void main(String[] args) {
EnumTest spring = new EnumTest(Season.SPRING);
spring.tellItLikeItIs();
EnumTest winter = new EnumTest(Season.WINTER);
winter.tellItLikeItIs();
}
}
// Output:
// Time for rebirth!
// Stay warm inside!
In this example, we’ve declared an enum Season
with four constants representing the seasons. We then use this Season
enum in our EnumTest
class to print out a different message based on the season.
One key advantage of using enums in Java is that they are type-safe, meaning that you can’t assign any value other than the predefined enum constants to an enum variable. They also have many built-in methods that make it easy to use and manipulate enum constants.
However, enums in Java aren’t without their potential pitfalls. One thing to remember is that enums define a single instance for each of their constants, so they can’t be instantiated like a class. Also, because they are instances of an enum type, you can’t extend another class or enum.
Advanced Enum Usage in Java
As we delve deeper into the world of enums in Java, you’ll find that they can do much more than just define a set of constants. Enums in Java can have constructors, methods, and attributes, making them much more flexible and powerful than their counterparts in many other languages.
Constructors, Methods, and Attributes in Enums
Let’s start with an example that demonstrates these features:
public enum Planet {
MERCURY (3.303e+23, 2.4397e6),
VENUS (4.869e+24, 6.0518e6),
EARTH (5.976e+24, 6.37814e6),
MARS (6.421e+23, 3.3972e6),
JUPITER (1.9e+27, 7.1492e7),
SATURN (5.688e+26, 6.0268e7),
URANUS (8.686e+25, 2.5559e7),
NEPTUNE (1.024e+26, 2.4746e7);
private final double mass; // in kilograms
private final double radius; // in meters
Planet(double mass, double radius) {
this.mass = mass;
this.radius = radius;
}
private double mass() { return mass; }
private double radius() { return radius; }
}
In this example, we’ve defined an enum Planet
with eight constants representing the planets in our solar system. Each enum constant is initialized with two parameters (mass and radius), which are passed to the enum’s constructor. The enum also includes two methods (mass()
and radius()
) to retrieve these values.
Enums in Switch Statements
Enums can be used in switch statements to control program flow based on the enum constant. Here’s how you can use the Planet
enum in a switch statement:
public class EnumTest {
Planet planet;
public EnumTest(Planet planet) {
this.planet = planet;
}
public void tellItLikeItIs() {
switch (planet) {
case EARTH:
System.out.println("Earth is habitable.");
break;
case MARS:
System.out.println("Mars is the next destination.");
break;
default:
System.out.println("Other planets are currently uninhabitable.");
break;
}
}
public static void main(String[] args) {
EnumTest earth = new EnumTest(Planet.EARTH);
earth.tellItLikeItIs();
EnumTest mars = new EnumTest(Planet.MARS);
mars.tellItLikeItIs();
EnumTest jupiter = new EnumTest(Planet.JUPITER);
jupiter.tellItLikeItIs();
}
}
// Output:
// Earth is habitable.
// Mars is the next destination.
// Other planets are currently uninhabitable.
In this example, we’ve used the Planet
enum in a switch statement to print out a different message based on the planet. This demonstrates the power and flexibility of enums in Java, as they can be used to control program flow in a clear and type-safe way.
Alternative Approaches to Enums in Java
While enums are a powerful tool in Java, there are other ways to accomplish the same tasks. Two of these methods are through the use of classes or interfaces. Understanding these alternatives can help you make more informed decisions in your code design.
Using Classes
In some cases, you might find that a class can serve the same purpose as an enum. For instance, you could create a class with a private constructor and some public static final fields.
Here’s an example:
public class Season {
public static final Season SPRING = new Season("Spring");
public static final Season SUMMER = new Season("Summer");
public static final Season AUTUMN = new Season("Autumn");
public static final Season WINTER = new Season("Winter");
private String description;
private Season(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
}
In this example, we’ve created a Season
class that serves a similar purpose to an enum. However, this approach lacks some of the benefits of enums, such as type safety and built-in methods.
Using Interfaces
Another alternative to enums is interfaces. This can be particularly useful when you want to group related constants together.
Here’s an example:
public interface Season {
String SPRING = "Spring";
String SUMMER = "Summer";
String AUTUMN = "Autumn";
String WINTER = "Winter";
}
In this example, we’ve created an interface Season
with four constants. However, like the class approach, this lacks the type safety and built-in methods of enums.
Making the Right Decision
When deciding whether to use an enum, class, or interface, consider the needs of your program. If you need a fixed set of constants and the benefits of type safety and built-in methods, then enums are the way to go. If you need more flexibility or the ability to extend other classes, then a class or interface might be a better choice.
Troubleshooting Common Errors with Java Enums
While enums are powerful and flexible, like any other feature in programming, they can lead to errors if not used correctly. Let’s explore some common mistakes when using enums in Java, along with their solutions.
Error: Cannot Instantiate Enum
One common error is attempting to instantiate an enum using the new
keyword. Remember, enums are not classes and cannot be instantiated like one.
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY;
}
public class Test {
public static void main(String[] args) {
Day day = new Day(); // Error
}
}
In the above code, we are trying to instantiate the Day
enum, which leads to a compile-time error. Enums are instances of their own type and can’t be instantiated like classes.
Error: Null or Undefined Enum Constant
Another common error is referencing an enum constant that doesn’t exist, which will result in a NullPointerException
or IllegalArgumentException
.
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY;
}
public class Test {
public static void main(String[] args) {
Day day = Day.valueOf("HOLIDAY"); // Error
}
}
In the above code, we are trying to assign a non-existent enum constant HOLIDAY
to the day
variable, which leads to a runtime exception. Make sure to only reference constants that are defined in the enum.
Best Practices and Optimization Tips
While using enums in Java, it’s essential to follow best practices for clean, efficient code.
- Use Enums When Fixed Set of Constants: Enums are perfect when you have a fixed set of constants, like days of the week or planets in the solar system.
- Leverage Built-in Methods: Enums come with built-in methods like
values()
,valueOf()
,name()
, andordinal()
that can make your life easier. - Use Enums with Switch Statements: Enums can be used in switch statements for efficient and readable code.
- Avoid Instantiating Enums: Remember, enums are instances of their own type and can’t be instantiated like classes.
By following these guidelines, you can avoid common pitfalls and make the most of enums in Java.
Understanding Enums in the Context of Java
To fully grasp the concept of enums in Java, it’s crucial to understand where they fit in the broader context of the Java programming language. Enums are closely related to other fundamental concepts in Java, such as data types, classes, and interfaces.
Enums as a Special Data Type
In Java, an enum is a special type of data type, also known as an enumerated type. It’s used to define a variable that can only assign certain predefined values. This makes enums a type of restricted class.
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY;
}
In this example, Day
is an enum that can only take one of the seven predefined constants. This ensures type safety as you can’t assign any value other than the predefined constants to a Day
variable.
Enums and Classes
While enums are not classes, they share many similarities. Like classes, enums can have attributes, methods, and constructors. However, unlike classes, enums have a fixed set of instances (the enumerated constants), and you can’t create new instances of an enum.
public enum Planet {
MERCURY (3.303e+23, 2.4397e6),
VENUS (4.869e+24, 6.0518e6),
EARTH (5.976e+24, 6.37814e6),
MARS (6.421e+23, 3.3972e6),
JUPITER (1.9e+27, 7.1492e7),
SATURN (5.688e+26, 6.0268e7),
URANUS (8.686e+25, 2.5559e7),
NEPTUNE (1.024e+26, 2.4746e7);
private final double mass; // in kilograms
private final double radius; // in meters
Planet(double mass, double radius) {
this.mass = mass;
this.radius = radius;
}
private double mass() { return mass; }
private double radius() { return radius; }
}
In this example, Planet
is an enum that has attributes (mass
and radius
), a constructor, and methods (mass()
and radius()
). This demonstrates the class-like behavior of enums in Java.
Enums and Interfaces
Enums can also implement interfaces in Java, which allows them to be used in a polymorphic way, just like classes.
public enum Day implements Weekday {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY;
@Override
public boolean isWeekend() {
return (this == SATURDAY || this == SUNDAY);
}
}
public interface Weekday {
boolean isWeekend();
}
In this example, the Day
enum implements the Weekday
interface, which includes a method isWeekend()
. This method is implemented in the Day
enum to check if a day is a weekend.
By understanding enums in the context of these related concepts, you can gain a deeper understanding of their role and use in Java.
Applying Enums in Larger Java Projects
Enums in Java are not just for simple programs or scripts. They are a powerful tool that can be utilized in larger, more complex Java projects. Whether you’re building a web application, a desktop application, or a backend service, enums can help you write cleaner, safer, and more efficient code.
For instance, in a web application, you might use enums to represent the different roles a user can have, such as ADMIN, USER, and GUEST. In a game, you might use enums to represent the different states a game can be in, such as STARTING, RUNNING, PAUSED, and ENDED.
Enums and Related Concepts
When working with enums in Java, you’ll often encounter related concepts. For instance, enums often go hand-in-hand with switch statements, which provide a clean and efficient way to handle different enum constants.
Enums can also be used in conjunction with collections (like EnumSet and EnumMap) and annotations, which can further enhance their power and flexibility.
Further Resources for Mastering Java Enums
To continue your journey in mastering enums in Java, here are some additional resources that offer in-depth information and advanced usage scenarios:
- Beginner’s Guide to Java Primitive Data Types – Discover how Java handles numbers and text via primitive data types.
Enum with Values in Java – Understand how to define enums with custom values and behaviors in Java.
Java Double – Learn about the Java double data type for representing double-precision floating-point numbers.
Java Enum Tutorial by JournalDev covers basic to advanced topics like EnumSet, EnumMap, and more.
Java Enum Types by Oracle – The official Oracle documentation of enum types in Java, along with several examples.
Java Enums by Baeldung offers a deep dive into enums, including their structure, and usage in switch statements.
Wrapping Up: Enum Data Type in Java
In this comprehensive guide, we’ve dived deep into the world of enums in Java, a powerful tool for defining a set of predefined constants in an efficient and type-safe way.
We started with the basics, learning how to declare and use enums in Java. We then moved on to more advanced topics, such as using constructors, methods, and attributes in enums, and utilizing enums in switch statements. Along the way, we tackled common challenges you might encounter when using enums, providing solutions and best practices to guide you.
We also explored alternative approaches to enums, comparing them with classes and interfaces. This gave you a broader understanding of how enums fit into the Java ecosystem and when to use each approach.
Here’s a quick comparison of these methods:
Method | Type Safety | Flexibility | Built-in Methods |
---|---|---|---|
Enums | High | Moderate | Yes |
Classes | High | High | No |
Interfaces | Low | High | No |
Whether you’re a beginner just starting out with enums or an experienced developer looking to deepen your understanding, we hope this guide has been a valuable resource in mastering enums in Java.
Enums are a powerful tool in Java, bringing type safety, efficiency, and clarity to your code. With the knowledge you’ve gained from this guide, you’re now well-equipped to use enums effectively in your Java projects. Happy coding!