Declaring an Array of Strings: Bash Scripting Syntax Guide
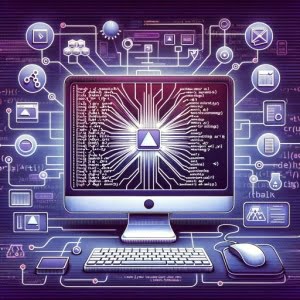
Are you finding it challenging to handle arrays of strings in Bash? You’re not alone. Many developers find themselves puzzled when it comes to handling arrays of strings in Bash, but we’re here to help.
Think of Bash’s arrays of strings as a well-organized library – allowing us to store and manipulate collections of strings, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with arrays of strings in Bash, from their creation, manipulation, and usage. We’ll cover everything from the basics of arrays to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Create and Use an Array of Strings in Bash?
In Bash, you can create an array of strings using parentheses
( )
and access elements using their index. For instance, to create an array with the strings “Hello” and “World”, you would usearr=("Hello" "World")
.
Here’s a simple example:
arr=("Hello" "World")
# Accessing elements
echo ${arr[0]} ${arr[1]}
# Output:
# 'Hello World'
In this example, we’ve created an array arr
with two elements, “Hello” and “World”. We then access these elements using their indices, 0
and 1
respectively, and print them out, resulting in the output ‘Hello World’.
This is just a basic way to create and use an array of strings in Bash. There’s much more to learn about manipulating arrays, handling complex operations, and alternative approaches. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Creating and Accessing Elements in Bash Arrays
Bash arrays allow you to store multiple values in a single variable. This is particularly useful when you need to group related data together. Let’s dive into the basics of creating and accessing elements in a Bash array of strings.
Creating a Bash Array
In Bash, you can create an array by simply assigning values to a variable using parentheses ( )
. Each value is separated by a space. Here’s an example:
# Creating an array
myArray=("Bash" "Array" "Of" "Strings")
# Printing the entire array
echo ${myArray[@]}
# Output:
# 'Bash Array Of Strings'
In this example, we’ve created an array myArray
with four elements: “Bash”, “Array”, “Of”, and “Strings”. The [@]
index is used to access all elements of the array, resulting in the output ‘Bash Array Of Strings’.
Accessing Array Elements
You can access an array element by referring to its index number. The index of the first element is 0
. For instance, to access the first element of our myArray
, we would use myArray[0]
.
Here’s how you can access individual elements:
# Accessing the first element
echo ${myArray[0]}
# Output:
# 'Bash'
In this example, we accessed the first element (“Bash”) of myArray
using myArray[0]
, resulting in the output ‘Bash’.
Bash arrays are incredibly versatile, allowing you to store multiple related values in a single variable. However, it’s important to remember that the indices start at 0
– a common pitfall for beginners.
Advanced Bash Array Operations
As you become more comfortable with Bash arrays, you can start to explore more complex operations. This includes adding and removing elements, looping through arrays, and sorting and filtering arrays. Let’s delve into these operations.
Adding and Removing Elements
You can add elements to a Bash array using the +=
operator. To remove elements, you can use the unset
command. Here’s an example:
# Adding an element
countries=("USA" "UK" "Canada")
countries+=("Australia")
# Printing the updated array
echo ${countries[@]}
# Output:
# 'USA UK Canada Australia'
# Removing an element
unset countries[1]
# Printing the updated array
echo ${countries[@]}
# Output:
# 'USA Canada Australia'
In this example, we first added “Australia” to our countries
array using +=
. We then removed the second element (“UK”) using unset
. The updated array is then printed out, showing the changes.
Looping Through Arrays
You can iterate through the elements of a Bash array using a for
loop. Here’s how:
# Looping through an array
for country in "${countries[@]}"
do
echo $country
done
# Output:
# 'USA'
# 'Canada'
# 'Australia'
In this example, we looped through the countries
array and printed each element on a new line.
Sorting and Filtering Arrays
Bash doesn’t have built-in functions for sorting or filtering arrays. However, you can achieve these operations using other commands like sort
and grep
. Here’s an example of how to sort an array:
# Sorting an array
sortedCountries=($(for i in "${countries[@]}"; do echo $i; done | sort))
# Printing the sorted array
echo ${sortedCountries[@]}
# Output:
# 'Australia Canada USA'
In this example, we sorted the countries
array by piping the array elements to the sort
command. The sorted array is then stored in sortedCountries
and printed out.
These advanced operations can greatly enhance your ability to manipulate and utilize Bash arrays of strings. Remember, practice is key to mastering these techniques.
Alternative Techniques for Bash Arrays
While arrays are a powerful tool in Bash, they are not the only way to handle collections of strings. Bash also supports associative arrays and external tools like awk or sed. Let’s explore these alternatives.
Associative Arrays in Bash
Associative arrays, unlike regular arrays, use keys instead of numeric indices. This can be useful when you need to store data in a key-value pair format. Here’s an example:
# Creating an associative array
declare -A myAssociativeArray
myAssociativeArray["fruit"]="Apple"
myAssociativeArray["vegetable"]="Carrot"
# Accessing elements
echo ${myAssociativeArray["fruit"]}
# Output:
# 'Apple'
In this example, we created an associative array myAssociativeArray
and added two elements with keys “fruit” and “vegetable”. We then accessed the element with the key “fruit”, resulting in the output ‘Apple’.
Associative arrays can be more flexible than regular arrays, but they can also be more complex to use and understand.
Using External Tools: awk and sed
Bash also allows you to use external tools like awk or sed to handle arrays. These tools can provide more advanced features and flexibility, but they also come with a steeper learning curve. Here’s an example using awk:
# Using awk to split a string into an array
echo "Bash Array Of Strings" | awk '{split($0,a," "); print a[2]}'
# Output:
# 'Array'
In this example, we used awk to split a string into an array. The split
function in awk divides the string into an array a
using space as the delimiter. We then printed the second element of the array, resulting in the output ‘Array’.
While these tools can be very powerful, they can also be more complex and less straightforward than using Bash’s built-in array features. It’s important to weigh the benefits and drawbacks when deciding which approach to use.
Common Pitfalls with Bash Arrays
Working with Bash arrays can sometimes present challenges, especially when dealing with spaces in strings or handling empty or undefined elements. Let’s explore these common issues and their solutions.
Handling Spaces in Strings
When working with arrays, it’s important to remember that Bash treats spaces as delimiters. This means that if a string in your array contains spaces, Bash will interpret it as separate elements. Here’s an example:
# Creating an array with a string that contains spaces
arr=("Hello World")
# Printing the array
echo ${arr[@]}
# Output:
# 'Hello World'
In this example, even though the string “Hello World” contains a space, it’s treated as a single element in the array because we’ve used quotes around it. Without the quotes, Bash would interpret it as two separate elements.
Dealing with Empty or Undefined Elements
Another common issue is dealing with empty or undefined elements in your array. If you try to access an element that doesn’t exist, Bash won’t throw an error but will return an empty string. Here’s an example:
# Trying to access an undefined element
echo ${arr[2]}
# Output:
# ''
In this example, we tried to access the third element of arr
, which doesn’t exist. Instead of throwing an error, Bash simply returned an empty string.
These common pitfalls can make working with Bash arrays tricky, but with understanding and practice, you can avoid them. Remember, the key to mastering Bash arrays is understanding how Bash interprets and handles data.
Bash Arrays and Strings: The Fundamentals
To fully harness the power of Bash arrays, it’s crucial to understand the underlying concepts and theories. Let’s delve into the fundamentals of arrays and strings in Bash and their significance in Bash scripting.
Understanding Bash Arrays
In Bash, an array is a variable that can hold multiple values. Each value (also called an element) in an array is associated with a numeric index, starting with zero. The ability to store multiple related values under a single variable name makes arrays a powerful tool in Bash scripting.
Here’s an example of a Bash array:
# Creating a Bash array
fruits=("Apple" "Banana" "Cherry")
# Printing the array
echo ${fruits[@]}
# Output:
# 'Apple Banana Cherry'
In this example, we’ve created a Bash array fruits
that contains three elements. We then printed the entire array, resulting in the output ‘Apple Banana Cherry’.
The Power of Strings in Bash
In Bash, a string is a sequence of characters. Strings are one of the most fundamental and common types of data in scripting and programming languages. In the context of Bash arrays, strings are often the type of data that we store and manipulate.
Here’s an example of a string in Bash:
# Creating a string in Bash
string="Hello, World!"
# Printing the string
echo $string
# Output:
# 'Hello, World!'
In this example, we’ve created a string string
that contains the text ‘Hello, World!’. We then printed the string, resulting in the output ‘Hello, World!’.
The Significance of Arrays in Bash Scripting
Arrays play a crucial role in Bash scripting. They allow us to store and manipulate multiple related values under a single variable name. This can simplify our scripts and make them more efficient, especially when dealing with large amounts of data.
In conclusion, understanding the fundamentals of arrays and strings in Bash is key to mastering Bash scripting. By understanding these concepts, you’ll be better equipped to create and manipulate arrays in Bash, making your scripts more powerful and efficient.
Applying Bash Arrays in Larger Scripts
Bash arrays of strings aren’t just for simple scripts or small tasks. They can also be a powerful tool in larger Bash scripts or projects, providing a versatile and efficient way to handle data.
Bash Arrays in Large Projects
In larger Bash scripts or projects, arrays can be used to store and manipulate data on a larger scale. For instance, you might use an array to store the filenames in a directory, or the lines in a file. You can then manipulate this data as needed, such as sorting the filenames or filtering the lines.
Here’s an example of how you might use a Bash array in a larger script:
# Storing filenames in an array
files=(*)
# Looping through the files
echo "Files in directory:"
for file in "${files[@]}"
do
echo $file
done
# Output:
# 'Files in directory:'
# 'file1.txt'
# 'file2.txt'
# '...
In this example, we stored the filenames in the current directory in an array files
. We then looped through the array and printed each filename. This could be part of a larger script that performs operations on these files, such as renaming them or moving them to a different directory.
Related Topics: Associative Arrays and String Manipulation
Once you’ve mastered Bash arrays of strings, there are many related topics that you might find interesting. For instance, you could explore associative arrays in Bash, which allow you to use keys instead of numeric indices. This can be useful when you need to store data in a key-value pair format.
You might also be interested in string manipulation in Bash, which involves operations like splitting strings, replacing substrings, or changing the case of strings. These skills can be incredibly useful when working with arrays of strings.
Further Resources for Bash Array Mastery
If you’re interested in delving deeper into Bash arrays and related topics, here are some resources that you might find helpful:
- GNU Bash Manual: The official manual for Bash, which includes a detailed section on arrays.
- Bash Guide for Beginners: An in-depth guide to Bash scripting, including arrays and string manipulation.
- Advanced Bash-Scripting Guide: A guide to more advanced topics in Bash scripting, including associative arrays and external tools like awk and sed.
Wrapping Up: Mastering Bash Array of Strings
In this comprehensive guide, we’ve navigated the world of Bash arrays of strings, exploring their creation, manipulation, and various use cases.
We began with the basics, learning how to create a Bash array of strings and access its elements. We then ventured into more advanced territory, exploring operations such as adding and removing elements, looping through arrays, and even sorting and filtering arrays.
Along our journey, we also tackled common challenges you might face when working with Bash arrays, such as handling spaces in strings and dealing with empty or undefined elements. We provided solutions and workarounds for these issues to help you navigate these potential pitfalls.
We also explored alternative approaches to handling arrays of strings in Bash, including the use of associative arrays and external tools like awk and sed. These alternatives offer additional flexibility and functionality, but also come with their own set of challenges and considerations.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Bash Arrays | Moderate | Low | General Use |
Associative Arrays | High | Moderate | Key-Value Data |
External Tools (awk, sed) | High | High | Advanced Operations |
Whether you’re just starting out with Bash arrays of strings or you’re looking to level up your Bash scripting skills, we hope this guide has given you a deeper understanding of Bash arrays and their capabilities.
With their versatility and efficiency, Bash arrays of strings are a powerful tool for handling data in Bash scripting. Now, you’re well equipped to harness their power. Happy scripting!