Pygame Guidebook: The Python Game Developer’s Toolkit
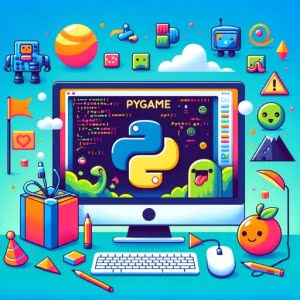
Are you looking to develop games in Python? Pygame is your answer. Like a Swiss army knife for game developers, Pygame provides all the tools you need to bring your game ideas to life.
This guide will walk you through everything you need to know about Pygame, from the basics to more advanced concepts.
Pygame offers a powerful yet accessible platform for game development. With Pygame, you can create simple 2D games, complex 3D environments, and everything in between.
So, let’s dive into the world of Pygame and start bringing your game ideas to life.
TL;DR: What is Pygame and how do I use it?
Pygame is a set of Python modules designed for writing video games. It provides functionalities such as creating windows, drawing shapes, handling input, and playing sounds. Here’s a basic example of how to create a window in Pygame:
import pygame
pygame.init()
win = pygame.display.set_mode((500, 500))
pygame.display.update()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
In this example, we first import the pygame module and initialize all imported pygame modules using pygame.init()
.
We then create a window with dimensions 500×500 using pygame.display.set_mode()
. The pygame.display.update()
function is used to update the entire display. The while True:
loop is used to keep the window open until the user closes it.
The for event in pygame.event.get():
loop is used to get events from the queue, like keyboard or mouse events. If the event type is pygame.QUIT
, which occurs when the user clicks the close button, we call pygame.quit()
to close the window.
Stay tuned for more detailed information and advanced usage scenarios. This is just the tip of the iceberg when it comes to Pygame’s capabilities!
Table of Contents
- Getting Started with Pygame
- Handling Events in Pygame
- Drawing Shapes with Pygame
- Advanced Pygame: Input Handling, Playing Sounds, and Using Sprites
- Exploring Alternatives to Pygame: Pyglet and Panda3D
- Comparing Pygame, Pyglet, and Panda3D
- Troubleshooting Common Pygame Issues
- Understanding Game Development Fundamentals with Pygame
- Expanding Your Pygame Horizons
- Wrapping Up: Mastering Pygame for Python Game Development
Getting Started with Pygame
Let’s start with the basics of Pygame. The first step in any Pygame program is to import the Pygame module and initialize it. Initialization is necessary as it loads the Pygame module and prepares it for use.
import pygame
pygame.init()
After initializing Pygame, the next step is to create a window where you can draw shapes, display text, and images. This is done using the pygame.display.set_mode()
function. Let’s create a window with dimensions 500×500.
win = pygame.display.set_mode((500, 500))
Handling Events in Pygame
In Pygame, an event is a response to user actions such as pressing a key, moving the mouse, or closing the window. Pygame has a function pygame.event.get()
that gets all the events from the queue. We use a loop to continuously check for these events.
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
In the above code, we create an infinite loop using while True:
. Inside this loop, we iterate over all the events in the event queue. If the event type is pygame.QUIT
, which happens when the user clicks the close button, we call pygame.quit()
to close the window.
Drawing Shapes with Pygame
Drawing shapes is a fundamental part of game development. Pygame provides several functions to draw shapes like rectangles, circles, and polygons. Let’s draw a simple rectangle on our window.
pygame.draw.rect(win, (255, 0, 0), (50, 50, 100, 100))
pygame.display.update()
In the above code, pygame.draw.rect()
is used to draw a rectangle. The function takes four arguments: the surface to draw on (our window win
), the color of the shape (a tuple of RGB values), and the rectangle to be drawn (a tuple of four values representing the x and y coordinates of the top left corner, and the width and height of the rectangle). After drawing the shape, we call pygame.display.update()
to make the drawn shape appear on the screen. The rectangle will be red as we have given the RGB values (255, 0, 0).
Advanced Pygame: Input Handling, Playing Sounds, and Using Sprites
As you become more comfortable with Pygame, you’ll start to explore more complex features. Let’s delve into handling keyboard and mouse input, playing sounds, and using sprites.
Handling Keyboard and Mouse Input
User input is an integral part of any game. Pygame provides an easy way to handle keyboard and mouse inputs. Let’s look at how to handle keyboard input.
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
print('Left key pressed')
elif event.key == pygame.K_RIGHT:
print('Right key pressed')
In the code above, we added a new event type pygame.KEYDOWN
to check if any key is pressed. If the left or right key is pressed, we print a corresponding message.
Playing Sounds
Sound effects and music can greatly enhance your game. Pygame allows you to play sounds with minimal effort. First, you need to initialize the mixer module, then load and play the sound.
pygame.mixer.init()
jump_sound = pygame.mixer.Sound('jump.wav')
jump_sound.play()
In the above code, we first initialize the mixer module using pygame.mixer.init()
. We then load the sound ‘jump.wav’ using pygame.mixer.Sound()
and store it in jump_sound
. To play the sound, we simply call jump_sound.play()
.
Using Sprites
Sprites are 2D bitmaps used to create characters, objects, and backgrounds in games. Pygame provides the Sprite
class to create and manage sprites.
class Player(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.image.load('player.png')
self.rect = self.image.get_rect()
player = Player()
In the code above, we create a Player
class that inherits from pygame.sprite.Sprite
. In the __init__
method, we load an image for the sprite and get its rectangular area. We then create an instance of the Player
class.
Exploring Alternatives to Pygame: Pyglet and Panda3D
While Pygame is a powerful tool for game development in Python, there are other libraries that you might consider depending on your needs. Two of the most notable alternatives are Pyglet and Panda3D.
Pyglet: A Windowing and Multimedia Library
Pyglet is a Python library for creating games and other visually-rich applications. It supports windowing, user interface event handling, and more. Here’s a simple example of creating a window with Pyglet:
import pyglet
window = pyglet.window.Window()
pyglet.app.run()
In the code above, we first import the pyglet module. We then create a window and start the application event loop with pyglet.app.run()
. The window will remain open until the user closes it.
Panda3D: A 3D Game Engine for Python
Panda3D is a game engine, a framework for 3D rendering and game development for Python and C++ programs. Here’s a simple example of setting up a 3D environment with Panda3D:
from panda3d.core import Point3
from direct.showbase.ShowBase import ShowBase
class MyApp(ShowBase):
def __init__(self):
ShowBase.__init__(self)
self.environ = self.loader.loadModel('models/environment')
self.environ.reparentTo(self.render)
self.environ.setScale(0.25, 0.25, 0.25)
self.environ.setPos(-8, 42, 0)
app = MyApp()
app.run()
In the code above, we first import the necessary modules from Panda3D. We then create a class MyApp
that inherits from ShowBase
. In the __init__
method, we load a 3D model of an environment, set its scale and position, and then make it a child of the render parent, which means it will be rendered in the scene.
Comparing Pygame, Pyglet, and Panda3D
While Pygame, Pyglet, and Panda3D all provide robust tools for game development in Python, they each have their strengths and weaknesses. Pygame is known for its simplicity and ease of use, making it a great choice for beginners. Pyglet, on the other hand, provides more advanced features like windowing and multimedia handling. Panda3D is the most advanced of the three, offering full-fledged 3D rendering and game development tools, but it also has a steeper learning curve.
Choosing between these libraries depends on your specific needs and your comfort level with Python. If you’re just starting out, Pygame could be a great choice. If you’re looking to create more complex games with advanced graphics and sound, Pyglet or Panda3D might be more suitable.
Troubleshooting Common Pygame Issues
Like any library, Pygame isn’t without its quirks. Let’s explore some common issues you might encounter when using Pygame and how to resolve them.
Issue with Event Handling
One common issue is handling multiple events simultaneously. For example, if you press two keys at the same time, your game may not respond to both. Here’s a solution:
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
print('Left key pressed')
if keys[pygame.K_RIGHT]:
print('Right key pressed')
In this code, pygame.key.get_pressed()
returns a list of boolean values representing the state of each key. If a key is pressed, the corresponding value in the list is True.
Drawing Shapes Incorrectly
Another common issue is drawing shapes incorrectly, such as drawing outside the window boundaries. To avoid this, always check the shape’s coordinates and dimensions before drawing.
Issues with Playing Sounds
Playing sounds can sometimes cause problems, such as the sound not playing or playing incorrectly. Make sure the sound file is in the correct format (WAV or OGG) and the file path is correct. Also, remember to initialize the mixer module before playing sounds.
pygame.mixer.init()
sound = pygame.mixer.Sound('sound.wav')
if pygame.mixer.get_init() is not None:
sound.play()
else:
print('Mixer not initialized')
In this code, we first check if the mixer module is initialized using pygame.mixer.get_init()
. If it’s initialized, we play the sound. If not, we print a message.
Remember, troubleshooting is a normal part of game development. Don’t get discouraged if you encounter issues. With patience and practice, you’ll overcome them and become a more skilled Pygame developer.
Understanding Game Development Fundamentals with Pygame
To fully harness the power of Pygame, it’s essential to grasp some fundamental game development concepts. Let’s delve into the game loop, event handling, and sprites.
The Game Loop
At the heart of every game is the game loop. It’s a continuous cycle that updates all elements of the game such as player inputs, updates the game state, and renders the game to the screen.
In Pygame, the game loop is implemented as a while
loop:
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# game logic here
pygame.display.update()
pygame.quit()
In this code, we have a running
variable that controls the game loop. Inside the loop, we handle events and update the game display. When the user closes the window, running
is set to False, and the game loop ends.
Event Handling in Pygame
Events are Pygame’s way of allowing the game to interact with the user. These can be key presses, mouse movements, or even system events.
In Pygame, events are handled using the pygame.event.get()
function inside the game loop:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
Here, pygame.event.get()
retrieves all events from the event queue. If the event type is pygame.QUIT
, the game loop ends.
Sprites and Sprite Groups
Sprites are 2D graphics that are the building blocks of your game. They can be characters, obstacles, backgrounds, etc. Pygame provides the Sprite
class to create and manage sprites.
class Player(pygame.sprite.Sprite):
def __init__(self):
super().__init__()
self.image = pygame.image.load('player.png')
self.rect = self.image.get_rect()
In this code, we create a Player
class that inherits from pygame.sprite.Sprite
. We load an image for the sprite and get its rectangular area.
Understanding these concepts will make working with Pygame a lot easier and more intuitive. It will allow you to create more complex and interactive games, and is a stepping stone to mastering game development with Pygame.
Expanding Your Pygame Horizons
As you become more comfortable with Pygame, you’ll likely want to start tackling larger projects and more complex games. There are several areas you can explore to take your Pygame skills to the next level.
Game Design Principles
Understanding game design principles can help you create more engaging and fun games. These principles cover a range of topics, from game mechanics and story development to player engagement and reward systems. Studying these principles can provide valuable insights into how to create successful games.
Creating Game Assets
Creating your own game assets, such as sprites, backgrounds, and sound effects, can give your game a unique look and feel. There are many tools available for creating 2D and 3D graphics, as well as sound editing software for creating your own sound effects and music.
Publishing Your Games
Once you’ve created a game, you might want to share it with others. There are many platforms available for publishing your games, from app stores to online game portals. You’ll need to learn about different publishing platforms, their requirements, and how to prepare your game for publication.
Further Resources for Mastering Pygame
To help you on your Pygame journey, here are some additional resources you might find useful:
- Exploring Essential Python Libraries – Explore a curated list of Python libraries to boost your coding productivity.
Managing Imports Across Directories in Python – Discover techniques for importing custom code and libraries efficiently.
Splitting Data for Machine Learning with sklearn: A Guide on the “train-test-split” technique for model evaluation.
Pygame Documentation – The official Pygame documentation is a good resource covering all library aspects.
Python Game Development Tutorials – This site offers a series of tutorials on game development with Pygame.
Real Python’s Game Development with Pygame tutorial provides a detailed guide to developing with Pygame, including handling graphics, sound, and user input.
Remember, mastering Pygame and game development is a journey. Don’t rush it. Take your time to understand each concept, practice regularly, and most importantly, have fun!
Wrapping Up: Mastering Pygame for Python Game Development
In this comprehensive guide, we’ve explored the world of game development using Pygame
, a powerful Python library.
We’ve covered everything from the basics of creating a window and handling events to more advanced topics like handling user input, playing sounds, and using sprites.
We’ve also tackled common issues in Pygame development, from event handling to drawing shapes and playing sounds, and provided solutions and workarounds to help you overcome these challenges. Moreover, we’ve explored alternative libraries for Python game development, such as Pyglet
and Panda3D
, giving you a broader view of the options available to you.
Here’s a quick comparison of Pygame and its alternatives:
Library | Strengths | Weaknesses |
---|---|---|
Pygame | Easy to use, great for beginners, good for 2D games | Not as powerful for 3D games |
Pyglet | Good for windowing and multimedia, powerful for 2D games | Less community support |
Panda3D | Powerful for 3D games, good for large projects | Steeper learning curve |
Remember, the best library for your game development journey depends on your specific needs, comfort level with Python, and the type of game you want to create. Whether you stick with Pygame or explore other libraries, the fundamental concepts of game development remain the same.
So, keep practicing, keep experimenting, and most importantly, keep having fun with your game development journey!