Decoding Java Errors with Explanations and Solutions
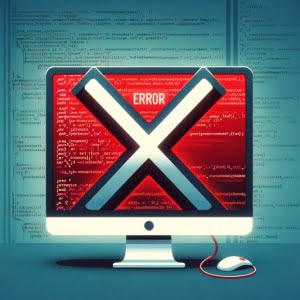
Ever felt like you’re wrestling with a Java error that’s hindering your progress? You’re not alone. Many developers find themselves puzzled when it comes to handling Java errors, but we’re here to help.
Think of Java errors as a maze – they might seem daunting at first, but with the right guidance, you can navigate through them with ease. Java errors provide clues that can guide you towards the solution, much like a skilled detective deciphering a complex case.
This guide will walk you through the most common Java errors, their causes, and solutions. We’ll cover everything from the basics of Java errors to more advanced issues, as well as best practices for error handling.
Let’s dive in and start mastering Java errors!
TL;DR: What are some common Java errors and how can I fix them?
Common Java errors include
NullPointerException
,ArrayIndexOutOfBoundsException
, andClassNotFoundException
. Each of these errors is caused by a specific issue in your code, such as accessing a null object or an array element that doesn’t exist. The solutions involve checking your code for these issues and correcting them.
Here’s a simple example:
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length());
}
}
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’re trying to access the length()
method on a null string, which results in a NullPointerException
.
This is just a basic introduction to Java errors, but there’s much more to learn about identifying, understanding, and troubleshooting them. Continue reading for more detailed information and advanced error handling techniques.
Table of Contents
- Unraveling Basic Java Errors
- Tackling Advanced Java Errors
- Mastering Error Handling in Java
- Troubleshooting Java Errors: Best Practices & Considerations
- The Backbone of Java Errors: Fundamentals and Hierarchy
- Beyond Basic Error Handling: Building Robust Applications
- Wrapping Up: Mastering Java Error Handling
Unraveling Basic Java Errors
Java, like any other programming language, has its fair share of common errors that beginners often encounter. Let’s decode some of these errors and understand how to fix them.
1. NullPointerException
A NullPointerException
occurs when you try to use a reference that points to no location in memory (null) as though it were referencing an object. In essence, it’s trying to handle something that doesn’t exist.
Here’s an example:
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length());
}
}
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this case, we’re trying to access the length()
method on a null string, which is not possible. The solution is to ensure that the object isn’t null before trying to use it.
2. ArrayIndexOutOfBoundsException
An ArrayIndexOutOfBoundsException
is thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
Here’s an example:
public class HelloWorld {
public static void main(String[] args) {
MissingClass obj = new MissingClass();
obj.printMessage();
}
}
# Output:
# Error: Could not find or load main class HelloWorld
# Caused by: java.lang.NoClassDefFoundError: MissingClass
In this example, we’re trying to create an instance of MissingClass
and call the printMessage
method on it. However, MissingClass
doesn’t exist in our classpath, leading to a java.lang.NoClassDefFoundError
.
3. ClassNotFoundException
A ClassNotFoundException
is thrown when an application tries to load in a class through its string name but no definition for the class with the specified name could be found.
Here’s an example:
public class Main {
public static void main(String[] args) {
try {
Class.forName("com.unknown.UnknownClass");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
# Output:
# java.lang.ClassNotFoundException: com.unknown.UnknownClass
In this case, we’re trying to load a class that doesn’t exist. The solution is to ensure that the class you’re trying to load is available in your classpath.
These are just a few examples of the basic errors you might encounter when starting your journey with Java. Understanding these errors and knowing how to fix them is the first step towards becoming proficient in Java.
Tackling Advanced Java Errors
As you progress with Java, you’ll encounter more complex errors. Let’s explore some of these advanced errors and understand how to address them.
1. ConcurrentModificationException
A ConcurrentModificationException
is thrown when one thread is modifying a collection while another thread is iterating over it.
Let’s look at an example:
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("One");
list.add("Two");
for (String item : list) {
if (item.equals("Two")) {
list.remove(item);
}
}
}
}
# Output:
# Exception in thread "main" java.util.ConcurrentModificationException
In this case, we’re removing an item from the list while iterating over it, which is not allowed. The solution is to use an Iterator
to safely remove items from the list while iterating.
2. StackOverflowError
A StackOverflowError
typically occurs when your recursion depth is too high or you have infinite recursion.
Here’s an example:
public class Main {
public static void recursivePrint(int num) {
if (num == 0) return;
else recursivePrint(++num);
}
public static void main(String[] args) {
recursivePrint(1);
}
}
# Output:
# Exception in thread "main" java.lang.StackOverflowError
In this case, we’ve created an infinite recursion, because the termination condition for the recursion is never met. The solution is to ensure you have a valid termination condition for your recursion.
Understanding these advanced errors will help you write more robust and reliable Java code. Remember, every error is an opportunity to learn and improve your coding skills.
Mastering Error Handling in Java
As your Java skills evolve, you’ll need to learn how to handle errors effectively. It’s not just about identifying and fixing errors, but also about preventing them and ensuring your code is robust and reliable. Here, we’ll discuss some best practices for error handling in Java, such as using try-catch blocks and throwing exceptions.
1. Using Try-Catch Blocks
A try-catch
block is used to handle exceptions. It allows the program to continue running even if an exception occurs.
Here’s an example:
public class Main {
public static void main(String[] args) {
try {
int result = 10 / 0;
System.out.println(result);
} catch (ArithmeticException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
# Output:
# An error occurred: / by zero
In this case, we’re dividing by zero, which is not allowed in mathematics and will throw an ArithmeticException
. However, because we’ve wrapped the code in a try-catch
block, the exception is caught, and an error message is printed instead of crashing the program.
2. Throwing Exceptions
Sometimes, it’s necessary to throw an exception to indicate that something has gone wrong. This can be done using the throw
keyword.
Here’s an example:
public class Main {
public static void checkAge(int age) {
if (age < 18) {
throw new ArithmeticException("Access denied - You must be at least 18 years old.");
} else {
System.out.println("Access granted - You are old enough!");
}
}
public static void main(String[] args) {
checkAge(15);
}
}
# Output:
# Exception in thread "main" java.lang.ArithmeticException: Access denied - You must be at least 18 years old.
In this case, we’re checking if a person is old enough to access a certain feature of our program. If they’re not old enough, we throw an ArithmeticException
with a custom message.
Mastering these error handling techniques is crucial for writing robust and reliable Java code. Remember, good error handling doesn’t just fix errors—it prevents them.
Troubleshooting Java Errors: Best Practices & Considerations
Dealing with Java errors involves more than just understanding the error and its cause. Effective troubleshooting requires a methodical approach and the use of the right tools. Let’s discuss some common issues you may encounter when dealing with Java errors, and share some tips and best practices for troubleshooting them.
1. Understanding Error Messages
Java error messages can sometimes be cryptic, especially if you’re new to the language. However, they’re also full of useful information that can help you understand what went wrong.
For example, consider this error message:
# Output:
# Exception in thread "main" java.lang.NullPointerException: Cannot invoke "Object.toString()" because "obj" is null
This error message tells you that you’re trying to call the toString()
method on an object (obj
) that is null. Understanding this can help you locate the error in your code and fix it.
2. Using Debugging Tools
Java offers several debugging tools that can help you troubleshoot errors. For example, the Java Debugger (JDB) is a command-line tool that allows you to step through your code, inspect variables, and evaluate expressions.
Here’s a simple example of how you can use JDB to debug a Java program:
# Compile the Java program with the -g option to generate all debugging info
javac -g Main.java
# Start the Java Debugger
jdb Main
In the debugger, you can then use commands like step
to step through your code, print
to print the value of a variable, or quit
to quit the debugger.
3. Best Practices for Troubleshooting Java Errors
When troubleshooting Java errors, it’s important to stay methodical. Start by understanding the error message, then try to reproduce the error in a controlled environment. Use debugging tools to inspect your code and understand what’s happening. And remember, Google is your friend! If you’re stuck, chances are someone else has encountered the same error and found a solution.
Remember, dealing with errors is a normal part of programming. Don’t get discouraged if you encounter an error. Instead, see it as a learning opportunity. With the right approach and tools, you can become a master at troubleshooting Java errors.
The Backbone of Java Errors: Fundamentals and Hierarchy
Before we delve deeper into handling Java errors, it’s crucial to understand how errors work in Java. This includes deciphering the difference between errors and exceptions, and comprehending the concept of the exception hierarchy in Java.
Errors vs Exceptions in Java
In Java, both errors and exceptions are subclasses of the Throwable
class. The significant difference between the two lies in the fact that errors are typically not handled directly in your code. They usually happen at a lower level, like a StackOverflowError
or an OutOfMemoryError
, that are beyond a programmer’s control. Exceptions, on the other hand, are events that occur during the execution of programs that disrupt the normal flow of instructions.
Here’s a simple example of an exception:
public class Main {
public static void main(String[] args) {
try {
int result = 10 / 0;
System.out.println(result);
} catch (ArithmeticException e) {
System.out.println("Caught an exception: " + e.getMessage());
}
}
}
# Output:
# Caught an exception: / by zero
In this case, we’re catching an ArithmeticException
which is an exception, not an error. The exception disrupts the normal flow of the program, but we’re able to handle it with a try-catch
block.
Understanding the Exception Hierarchy in Java
Java’s exception hierarchy plays a vital role in how errors and exceptions are handled. At the top of the hierarchy is the Throwable
class, with two subclasses: Error
and Exception
. The Exception
class further branches into RuntimeException
and other exceptions.
Here’s a simple representation of the hierarchy:
Throwable
|
|--- Error
|
|--- Exception
|
|--- RuntimeException
|
|--- Other exceptions
Understanding this hierarchy is crucial because it affects how exceptions are caught. A catch
block for a superclass exception will catch exceptions of that class and all its subclasses. Therefore, the order of catch
blocks matters. You should start from the most specific (subclass) exception and proceed to the most general (superclass) exception.
In-depth understanding of these fundamentals equips you with the knowledge to effectively handle and troubleshoot Java errors and exceptions.
Beyond Basic Error Handling: Building Robust Applications
As you advance in your Java journey, you’ll find that error handling extends beyond fixing individual errors. It’s about building robust and reliable applications that can handle unexpected situations gracefully. Let’s discuss the importance of error handling in larger applications and how it relates to concepts like robustness and reliability.
The Role of Error Handling in Robust Applications
In a small program, an unhandled error might cause a minor inconvenience. But in a large application, it could lead to a system crash, data loss, or other serious consequences. That’s why robust applications need effective error handling.
Effective error handling ensures that your application can handle unexpected situations gracefully. It allows your application to recover from errors, or at least fail safely. In other words, it contributes to the robustness and reliability of your application.
Logging and Automated Testing: Allies in Error Handling
Error handling goes hand in hand with logging and automated testing. Logging helps you monitor your application and spot errors that might otherwise go unnoticed. Automated testing, on the other hand, helps you catch errors before they reach production.
Here’s an example of how you can use Java’s built-in logging API to log errors:
import java.util.logging.Logger;
public class Main {
private static final Logger LOGGER = Logger.getLogger(Main.class.getName());
public static void main(String[] args) {
try {
int result = 10 / 0;
} catch (ArithmeticException e) {
LOGGER.severe("An error occurred: " + e.getMessage());
}
}
}
# Output (in the log):
# SEVERE: An error occurred: / by zero
In this example, we’re logging an ArithmeticException
instead of just printing it to the console. This log entry could then be monitored and analyzed to help troubleshoot and prevent future errors.
Further Resources for Mastering Java Error Handling
To delve deeper into Java error handling and related topics, consider exploring the following resources:
- Core Java Essentials – Explore the fundamentals of core Java programming.
Throwing Exceptions in Java: How-To Guide – Explore how to throw exceptions in Java.
Exploring NoClassDefFoundError in Java – Master resolving NoClassDefFoundError in Java applications.
Oracle’s Java Tutorials: Exceptions – Guide on handling exceptions in Java, straight from the creators of Java.
Baeldung’s Guide to Java Logging covers various logging frameworks and best practices.
JUnit 5 User Guide – The official user guide for JUnit 5, a popular framework for automated testing in Java.
Remember, mastering error handling is a journey. Keep learning, keep experimenting, and you’ll become more proficient at handling and preventing Java errors.
Wrapping Up: Mastering Java Error Handling
In this comprehensive guide, we’ve dissected the complex world of Java errors. From understanding the basics to tackling advanced errors, we’ve navigated the maze that Java errors can often seem to be.
We started with the basics, decoding common Java errors like NullPointerException
, ArrayIndexOutOfBoundsException
, and ClassNotFoundException
. We then ventured into more complex territory, discussing advanced errors such as ConcurrentModificationException
and StackOverflowError
. Along the way, we’ve provided practical examples to illustrate these errors and their solutions.
Further, we’ve delved into expert-level error handling techniques, discussing the use of try-catch blocks and the throwing of exceptions. We’ve also shed light on best practices for troubleshooting Java errors, emphasizing the importance of understanding error messages and using debugging tools.
We also took a step back to look at the fundamentals of errors in Java, discussing the difference between errors and exceptions and the concept of the exception hierarchy in Java. Finally, we’ve touched upon the importance of error handling in building robust applications, and suggested related topics like logging and automated testing for further exploration.
Here’s a quick recap of the common Java errors we’ve discussed:
Error | Common Cause | Solution |
---|---|---|
NullPointerException | Accessing a null object | Ensure object isn’t null before using it |
ArrayIndexOutOfBoundsException | Accessing an array element outside its bounds | Ensure index is within array bounds |
ClassNotFoundException | Trying to load a class that doesn’t exist | Ensure class is available in classpath |
ConcurrentModificationException | Modifying a collection while iterating over it | Use Iterator to safely modify collection during iteration |
StackOverflowError | Excessive recursion depth or infinite recursion | Ensure valid termination condition for recursion |
Whether you’re a beginner just starting out with Java, or an intermediate developer looking to level up your error handling skills, we hope this guide has given you a deeper understanding of Java errors and how to handle them.
Remember, encountering errors is part and parcel of programming. Don’t be discouraged when you encounter a Java error. Instead, see it as a learning opportunity. Keep learning, keep experimenting, and you’ll become a master at handling and preventing Java errors. Happy coding!