Core Java Explained: Your Guide to Java Fundamentals
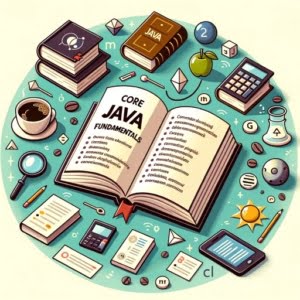
Are you finding the world of Java programming a bit intimidating? Don’t worry, you’re not alone. Many developers find themselves puzzled when they first encounter Core Java, but we’re here to help.
Think of Core Java as the foundation stone of your Java programming journey. It forms the base of any Java application, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of understanding Core Java, from basic concepts to more advanced topics. We’ll cover everything from data types, operators, control statements, to classes, objects, and more.
So, let’s dive in and start mastering Core Java!
TL;DR: What is Core Java?
Core Java refers to the fundamental parts of the Java programming language that form the basis of any Java application. This includes
data types, operators, control statements, classes, objects,
and more.
Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve created a basic Java program that prints ‘Hello, World!’ to the console. This involves using a class (HelloWorld
), a method (main
), and a statement (System.out.println("Hello, World!");
), which are all fundamental concepts in Core Java.
This is just a basic introduction to Core Java, but there’s much more to learn about its powerful and flexible features. Continue reading for a more detailed exploration of Core Java.
Table of Contents
- Core Java Basics: Data Types, Operators, and Control Statements
- Diving Deeper: Object-Oriented Programming in Core Java
- Advanced Core Java: Exception Handling, Multithreading, and Collections
- Troubleshooting Core Java: Common Issues and Solutions
- The Java Programming Language: A Brief History
- The Heart of Java: Core Java
- Core Java in Action: Real-World Applications and Further Learning
- Wrapping Up: Embarking on the Core Java Journey
Core Java Basics: Data Types, Operators, and Control Statements
When starting with Core Java, it’s essential to understand its basic building blocks. These include data types, operators, and control statements.
Data Types in Java
Data types are the most basic unit of any programming language. In Java, we have two main types of data: primitive (e.g., int, char, boolean) and non-primitive (e.g., String, Arrays, Classes).
Here’s an example of how we use these in a Java program:
public class DataTypes {
public static void main(String[] args) {
int num = 5; // int is a primitive data type
String str = "Hello"; // String is a non-primitive data type
System.out.println(num);
System.out.println(str);
}
}
# Output:
# 5
# Hello
In this example, num
is a variable of type int
(a primitive data type), and str
is a variable of type String
(a non-primitive data type). We then print these variables to the console.
Operators in Java
Operators are symbols that perform operations on one or more operands (variables and/or values). Java has several types of operators, including arithmetic (+
, -
, *
, /
, %
), relational (==
, !=
, “, =
), and logical (&&
, ||
, !
).
Here’s an example of operators in action:
public class Operators {
public static void main(String[] args) {
int a = 5;
int b = 10;
System.out.println(a + b); // addition operator
System.out.println(a == b); // equality operator
}
}
# Output:
# 15
# false
In this example, we first use the +
operator to add a
and b
together. We then use the ==
operator to check if a
and b
are equal.
Control Statements in Java
Control statements control the flow of execution in a Java program. They include if
, else
, switch
, for
, while
, and do-while
statements.
Here’s an example of a control statement:
public class ControlStatements {
public static void main(String[] args) {
int a = 5;
if (a > 0) {
System.out.println("a is positive");
} else {
System.out.println("a is not positive");
}
}
}
# Output:
# a is positive
In this example, we use an if
statement to check if a
is greater than 0. If it is, we print ‘a is positive’. If not, we print ‘a is not positive’.
Diving Deeper: Object-Oriented Programming in Core Java
As you become more comfortable with the basics of Core Java, it’s time to explore its object-oriented programming (OOP) concepts. These include classes, objects, inheritance, and polymorphism, which are fundamental to developing robust and scalable Java applications.
Classes and Objects in Java
In Java, a class is a blueprint from which individual objects are created. Let’s look at an example:
public class Dog {
String breed;
int age;
String color;
void barking() {
System.out.println("Woof!");
}
}
public class TestDog {
public static void main(String args[]) {
Dog myDog = new Dog();
myDog.barking();
}
}
# Output:
# Woof!
In this example, Dog
is a class that includes properties like breed
, age
, and color
, and a method barking()
. We then create an object myDog
from the Dog
class in the TestDog
class and call the barking()
method.
Inheritance in Java
Inheritance is an important pillar of OOP in Java. It allows a class to inherit the properties and methods of another class. Here’s an example:
public class Animal {
public void eat() {
System.out.println("Animal eats");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("Dog barks");
}
}
public class TestDog {
public static void main(String args[]) {
Dog myDog = new Dog();
myDog.eat();
myDog.bark();
}
}
# Output:
# Animal eats
# Dog barks
In this example, Dog
is a subclass that inherits from the Animal
superclass. When we create an object of Dog
, it can access both its own method bark()
and the inherited eat()
method.
Polymorphism in Java
Polymorphism in Java is a concept by which we can perform a single action in different ways. Let’s see how it works:
public class Animal {
public void sound() {
System.out.println("Animal makes a sound");
}
}
public class Dog extends Animal {
public void sound() {
System.out.println("Dog barks");
}
}
public class TestAnimal {
public static void main(String args[]) {
Animal myAnimal = new Dog();
myAnimal.sound();
}
}
# Output:
# Dog barks
In this example, we have overridden the sound()
method in the Dog
class. Even though we create an Animal
object, it uses the sound()
method in Dog
because the object is a Dog
instance. This is polymorphism in action.
Advanced Core Java: Exception Handling, Multithreading, and Collections
As you progress in your Core Java journey, you’ll encounter more advanced concepts. These include exception handling, multithreading, and collections. Let’s dive into each of these topics.
Exception Handling in Java
Exception handling is a powerful mechanism in Java used to handle runtime errors, so that normal flow of the application can be maintained.
Here’s an example of how exception handling works in Java:
public class ExceptionHandling {
public static void main(String args[]) {
try {
int divideByZero = 5 / 0;
}
catch (ArithmeticException e) {
System.out.println("ArithmeticException => " + e.getMessage());
}
}
}
# Output:
# ArithmeticException => / by zero
In this example, we’re trying to divide by zero, which is not allowed and would usually stop the program. However, by using a try-catch
block, we’re able to catch the ArithmeticException
and print an error message. This way, the program can continue running even after the exception occurs.
Multithreading in Java
Multithreading is a feature that allows concurrent execution of two or more parts of a program for maximum utilization of CPU.
Here’s a simple example of multithreading in Java:
class MultithreadingDemo extends Thread {
public void run() {
try {
System.out.println ("Thread " + Thread.currentThread().getId() + " is running");
}
catch (Exception e) {
System.out.println ("Exception caught");
}
}
}
public class Test {
public static void main(String[] args) {
int num = 8; // number of threads
for (int i=0; i<num; i++) {
MultithreadingDemo thread = new MultithreadingDemo();
thread.start();
}
}
}
# Output (may vary):
# Thread 11 is running
# Thread 12 is running
# ...
In this example, we create a MultithreadingDemo
class that extends the Thread
class. The run()
method is overridden with our desired functionality. In the main
method, we create multiple threads that execute the run()
method concurrently.
Collections in Java
The Java Collections Framework provides a set of interfaces and classes to implement various data structures and algorithms. This includes the List
, Set
, and Map
interfaces, among others.
Here’s a basic example of a Java collection:
import java.util.*;
public class CollectionsDemo {
public static void main(String args[]) {
List<String> list = new ArrayList<String>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
System.out.println(list);
}
}
# Output:
# [Apple, Banana, Cherry]
In this example, we create an ArrayList
of String
objects and add several fruits to it. We then print out the entire list.
Troubleshooting Core Java: Common Issues and Solutions
Learning Core Java is a journey, and like any journey, it’s not without its obstacles. Here, we’ll discuss some common issues you might encounter while learning Core Java and offer some solutions and best practices.
Understanding Java Errors
Java errors can be intimidating at first, but they’re actually quite informative. They tell you what kind of error occurred and where it happened.
For instance, if you try to divide by zero, you’ll get an ArithmeticException
:
public class ExceptionHandling {
public static void main(String args[]) {
try {
int divideByZero = 5 / 0;
}
catch (ArithmeticException e) {
System.out.println("ArithmeticException => " + e.getMessage());
}
}
}
# Output:
# ArithmeticException => / by zero
In this example, we catch the ArithmeticException
and print out an error message. This helps us identify and fix the problem.
Debugging Java Code
Debugging is a crucial skill for any programmer. Java IDEs like IntelliJ IDEA and Eclipse have powerful debugging tools that can help you step through your code and find where things are going wrong.
When debugging, break points are your best friend. They allow you to pause your code at specific points and inspect the values of variables.
Java Best Practices
Following best practices can help prevent many common issues. Here are a few tips:
- Comment your code: Comments can help you and others understand what your code is doing, and why.
- Follow naming conventions: Java has standard naming conventions, like using camelCase for variables and methods, and PascalCase for classes.
- Keep your code DRY: DRY stands for ‘Don’t Repeat Yourself’. If you find yourself writing the same code multiple times, consider creating a method that can be called multiple times instead.
Learning to troubleshoot and follow best practices will not only help you write better code, but also make your Core Java journey smoother and more enjoyable.
The Java Programming Language: A Brief History
Java, a class-based, object-oriented programming language, was developed by James Gosling at Sun Microsystems and released in 1995. It was designed to have as few implementation dependencies as possible, which led to its ‘Write once, run anywhere’ capability. This means that compiled Java code can run on all platforms that support Java without the need for recompilation.
Java quickly gained popularity and has since become one of the most used programming languages in the world, particularly for client-server web applications.
The Heart of Java: Core Java
Core Java, also known as ‘standard Java’, forms the foundation of the Java programming language. It includes fundamental features such as primitive data types, operators, control statements, classes, objects, inheritance, exception handling, and more.
Core Java is the part of Java that sets the syntax rules and basic programming constructs. It’s the part of Java that all other parts build upon. Without a solid understanding of Core Java, you won’t be able to understand more advanced Java features and libraries.
Here’s a simple example of a Core Java program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we have a class HelloWorld
with a method main
. The main
method is the entry point for any Java program. The System.out.println("Hello, World!");
line is a simple statement that prints ‘Hello, World!’ to the console.
This small program encompasses several fundamental Core Java concepts: classes, methods, and statements. As you dive deeper into Core Java, you’ll encounter more complex concepts, but they all build upon these basics.
Understanding Core Java is essential to becoming a proficient Java programmer. It’s the first step in your journey to mastering Java.
Core Java in Action: Real-World Applications and Further Learning
Having a good understanding of Core Java not only equips you with the knowledge of a powerful programming language, but it also opens the door to a variety of advanced concepts and real-world applications.
Core Java in Real-World Scenarios
Core Java is widely used in creating web applications, building robust backend systems, and even in developing Android applications. Its object-oriented nature allows for code reusability and modular design, which makes it a preferred choice for large-scale projects.
For instance, consider a bank application that has to manage millions of customers. With Core Java, you can create classes for ‘Customer’, ‘Account’, ‘Transaction’, etc., and use objects of these classes to perform operations like deposit, withdrawal, fund transfer, and more.
public class Customer {
private String name;
private Account account;
// methods for deposit, withdrawal, etc.
}
public class Account {
private double balance;
// methods to update balance
}
In this simplified example, we have a Customer
class and an Account
class. Each Customer
has an Account
, and we can create methods to perform various banking operations.
Exploring Java Frameworks and Libraries
Once you have a solid understanding of Core Java, you can explore various Java frameworks and libraries that can help you build complex applications more efficiently. These include Spring for enterprise applications, Hibernate for database connectivity, and Apache Commons for utility functions, among others.
Further Resources for Core Java Mastery
To deepen your understanding of Core Java and stay updated with the latest developments, here are a few resources you might find helpful:
- Java: A Solution for Various Tasks – Explore Java’s role in applications and development.
Understanding Java Data Types – Learn about primitive and reference data types and their usage.
Core Java Interview Prep Tips – Master answering core Java interview questions confidently and articulately.
Oracle’s Official Java Tutorials cover many topics in Core Java in detail.
Java Code Geeks offers a plethora of Java articles, tutorials, and resources for all levels.
Baeldung has a vast collection of in-depth tutorials on various Java topics, including Core Java, Spring, and more.
Wrapping Up: Embarking on the Core Java Journey
In this comprehensive guide, we’ve traversed the landscape of Core Java, the foundation of the Java programming language. From the basics to the advanced, we’ve explored the different facets of Core Java and how they come together to form robust and efficient Java applications.
We embarked on our journey with the fundamentals, understanding the basic building blocks of Core Java such as data types, operators, and control statements. We then ventured into the realm of object-oriented programming, delving into classes, objects, inheritance, and polymorphism. Our journey took us further into advanced concepts like exception handling, multithreading, and the Java Collections Framework.
Along the way, we tackled common challenges you might encounter when learning Core Java, providing you with troubleshooting tips and best practices to guide you. We also glanced at the real-world applications of Core Java, highlighting its importance in various domains from web applications to Android app development.
Concept | Importance | Complexity |
---|---|---|
Basic Concepts | Forms the foundation of Java programming | Beginner |
Object-Oriented Programming | Enables code reusability and modular design | Intermediate |
Advanced Concepts | Facilitates robust and efficient applications | Expert |
Whether you’re just dipping your toes into Core Java or you’re looking to solidify your understanding, we hope this guide has been a valuable resource in your journey to mastering Core Java.
With its robustness and versatility, Core Java is a powerful tool in the arsenal of any programmer. Now, you’re well equipped to harness the power of Core Java and apply it to your own projects. Happy coding!