Data Types in Java: How to Define and Manage Variables
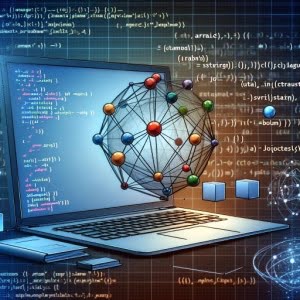
Ever felt overwhelmed by data types in Java? You’re not alone. Many developers, especially beginners, find understanding data types in Java a bit challenging. Think of Java’s data types as the building blocks – the raw materials that we use to construct our program.
Data types in Java are as essential as the ingredients needed to cook a meal. Without them, we can’t define the kind of data our variables can hold, leading to potential errors and inefficiencies.
In this guide, we’ll walk you through the different data types in Java, their uses, and how to work with them. We’ll start from the basics, covering primitive and non-primitive data types, and gradually move on to more advanced topics like type conversion, casting, and generics.
So, let’s dive in and start mastering data types in Java!
TL;DR: What Are Data Types in Java?
Java has two categories of data types, primitive and non-primitive. The primitive data types – includes
byte, short, int, long, float, double, char, boolean
. Non-primitive data types – includesString, Arrays, Classes, and Interfaces
Here’s a simple example of declaring an integer type variable in Java:
int myNum = 5;
System.out.println(myNum);
# Output:
# 5
In this example, we’ve declared a variable myNum
of type int
and assigned it the value 5
. When we print myNum
, it outputs 5
.
This is just a basic introduction to data types in Java, but there’s much more to learn about them, including their uses, type conversion, casting, and generics. Continue reading for a more detailed exploration.
Table of Contents
- Exploring Primitive and Non-Primitive Data Types in Java
- Type Conversion and Casting in Java
- Embracing Generics in Java
- Troubleshooting Common Issues with Data Types in Java
- The Importance and Fundamentals of Data Types in Java
- Applying Data Types in Larger Java Projects
- Exploring Related Topics in Java
- Wrapping Up: Mastering Data Types in Java
Exploring Primitive and Non-Primitive Data Types in Java
In Java, data types are divided into two main categories: primitive and non-primitive (also known as reference types).
Primitive Data Types in Java
Primitive data types in Java are the most basic data types. They include byte
, short
, int
, long
, float
, double
, char
, boolean
. Each of these data types has a predefined size and a specific value range.
Here’s an example of declaring and using variables of different primitive data types:
byte numByte = 10;
short numShort = 500;
int numInt = 10000;
long numLong = 150000L;
float numFloat = 5.75f;
double numDouble = 19.99d;
char myChar = 'A';
boolean myBool = true;
System.out.println(numByte);
System.out.println(numShort);
System.out.println(numInt);
System.out.println(numLong);
System.out.println(numFloat);
System.out.println(numDouble);
System.out.println(myChar);
System.out.println(myBool);
# Output:
# 10
# 500
# 10000
# 150000
# 5.75
# 19.99
# A
# true
In this example, we’ve declared variables of different primitive data types and assigned them appropriate values. When we print these variables, they output their respective values.
Non-Primitive Data Types in Java
Non-primitive data types, also known as reference types, include String
, Arrays
, Classes
, Interface
, and more. These data types reference a memory location where data is stored, rather than directly containing the values.
Here’s an example of declaring and using a String
and an Array
:
String greeting = "Hello, Java!";
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(greeting);
for (int number : numbers) {
System.out.println(number);
}
# Output:
# Hello, Java!
# 1
# 2
# 3
# 4
# 5
In this example, greeting
is a String
variable that holds a sequence of characters, and numbers
is an Array
that holds a sequence of int
values. When we print greeting
, it outputs Hello, Java!
. When we loop through numbers
and print each element, it outputs the numbers from 1
to 5
.
Understanding these basic data types in Java is the first step towards mastering Java programming.
Type Conversion and Casting in Java
As we delve deeper into Java’s data types, we come across the concept of type conversion, also known as casting. Casting is the process of converting a variable from one data type to another. In Java, there are two types of casting: implicit (automatic) and explicit.
Implicit Casting in Java
Implicit casting, also known as automatic type conversion, is performed by the Java compiler when we assign a smaller data type to a larger data type. In this case, there’s no data loss, and the operation is safe.
Here’s an example of implicit casting:
int myInt = 9;
double myDouble = myInt;
System.out.println(myInt);
System.out.println(myDouble);
# Output:
# 9
# 9.0
In this example, we’ve assigned an int
value to a double
variable. Since the size of double
is larger than int
, the Java compiler automatically performs an implicit cast, converting myInt
from int
to double
before the assignment. When we print myDouble
, it outputs 9.0
, the double
equivalent of the int
value 9
.
Explicit Casting in Java
Explicit casting, on the other hand, needs to be performed manually by the programmer when assigning a larger data type to a smaller one. This operation could lead to data loss, so it needs to be done carefully.
Here’s an example of explicit casting:
ndouble myDouble = 9.78;
int myInt = (int) myDouble;
System.out.println(myDouble);
System.out.println(myInt);
# Output:
# 9.78
# 9
In this example, we’ve explicitly cast myDouble
to int
before assigning its value to myInt
. This operation truncates the decimal part of myDouble
, resulting in data loss. When we print myInt
, it outputs 9
, which is the int
equivalent of the double
value 9.78
.
Understanding type conversion and casting in Java is crucial as it helps us manipulate and use data efficiently in our Java programs.
Embracing Generics in Java
As we continue our journey into the depths of data types in Java, we encounter the concept of generics. Generics were introduced in Java 5 to provide compile-time type checking and to eliminate the risk of ClassCastException that was common while working with collections (arrays, lists, etc.).
Creating Type-Safe Classes with Generics
Generics allow us to create classes that work with different data types while still providing type safety. This means you can create a single class, and it can be used with any data type, saving you the effort of creating separate classes for each data type.
Here’s an example of a simple generic class in Java:
public class GenericClass<T> {
private T obj;
public void setObj(T obj) {
this.obj = obj;
}
public T getObj() {
return this.obj;
}
}
GenericClass<String> stringObj = new GenericClass<String>();
stringObj.setObj("Hello, Java!");
System.out.println(stringObj.getObj());
GenericClass<Integer> intObj = new GenericClass<Integer>();
intObj.setObj(123);
System.out.println(intObj.getObj());
# Output:
# Hello, Java!
# 123
In this example, GenericClass
is a generic class that can work with any data type. We’ve created two objects of GenericClass
, stringObj
and intObj
, specifying String
and Integer
as the data types, respectively. When we set and get the object using stringObj
, it works with String
values. Similarly, intObj
works with Integer
values. This demonstrates the flexibility and type safety provided by generics.
Implementing Generic Methods
Just like classes, we can also create generic methods that can be used with different data types. Here’s an example:
public static <T> void printArray(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
Integer[] intArray = {1, 2, 3, 4, 5};
String[] stringArray = {"Hello", "Java", "Generics"};
printArray(intArray);
printArray(stringArray);
# Output:
# 1
# 2
# 3
# 4
# 5
# Hello
# Java
# Generics
In this example, printArray
is a generic method that prints elements of any type of array. We’ve called printArray
with an Integer
array and a String
array, and it successfully prints the elements of both arrays.
Embracing generics in Java allows us to write more flexible and type-safe code, making it an important concept to master for any Java programmer.
Troubleshooting Common Issues with Data Types in Java
While working with data types in Java, you may encounter some common issues. These issues can often lead to errors or unexpected results in your code. Let’s discuss some of these problems and how to resolve them.
Type Mismatch Errors
One of the most common errors you might encounter is a type mismatch error. This error occurs when you try to assign a value of one data type to a variable of a different data type. For example:
int num = "Hello, Java!";
# Output:
# error: incompatible types: String cannot be converted to int
In this example, we’re trying to assign a String
value to an int
variable, which results in a type mismatch error. The solution is to ensure that the value you’re assigning matches the data type of the variable.
Overflow and Underflow
Another common issue when working with data types in Java is overflow and underflow. This happens when you assign a value that’s outside the range of the data type. For example:
byte num = 128;
# Output:
# error: incompatible types: possible lossy conversion from int to byte
In this example, we’re trying to assign the value 128
to a byte
variable. However, the maximum value a byte
can hold is 127
, so this results in an overflow error. To resolve this, either use a larger data type that can accommodate the value or ensure that the value is within the range of the data type.
Understanding these common issues and their solutions can help you avoid errors and write more robust Java code.
The Importance and Fundamentals of Data Types in Java
In any programming language, data types are fundamental. They define the type of data that a variable can hold, such as integers, floating-point numbers, characters, or booleans. But why are they so important?
Why Are Data Types Necessary in Programming?
Data types are crucial for a few reasons. Firstly, they help the compiler allocate the appropriate amount of memory for the data. For example, an int
in Java requires 4 bytes of memory, while a double
requires 8 bytes.
Secondly, data types define the operations that can be performed on the data. For instance, you can perform arithmetic operations on int
and double
, but not on boolean
or char
.
Lastly, data types provide a form of data validation. They ensure that the data stored in a variable is of the correct type, preventing errors and improving the robustness of the code.
How Do Data Types Work at a Fundamental Level?
At a fundamental level, data types work by reserving a specific amount of memory for a variable and defining the type of data that can be stored in that memory space.
Here’s an example of how data types work in Java:
int num = 5;
System.out.println(num);
# Output:
# 5
In this example, int
is the data type, num
is the variable, and 5
is the value. When we declare int num = 5;
, the Java compiler reserves 4 bytes of memory for the variable num
and stores the value 5
in that memory space. When we print num
, it retrieves the value from the memory and outputs 5
.
Understanding the importance and fundamentals of data types is crucial for mastering Java or any other programming language. It forms the basis of how we manipulate and use data in our programs.
Applying Data Types in Larger Java Projects
Understanding data types in Java is not just about knowing the difference between an int
and a String
. It’s about knowing how to use these data types to build larger, more complex Java applications. Whether you’re creating a simple calculator or a complex web application, data types are the building blocks of your code.
For example, in a banking application, you might use int
or double
to represent account balances, String
to represent user names, and boolean
to check if a user is logged in or not. By understanding how to use data types effectively, you can manipulate these values to perform tasks like calculating interest, validating user input, and controlling user access.
Exploring Related Topics in Java
Mastering data types in Java is just the beginning. There are other related topics that you can explore to deepen your understanding of Java programming. These include:
- Java Operators: These are special symbols that perform specific operations on one, two, or three operands, and then return a result.
Control Statements: These are used to control the flow of execution in a program, allowing the program to branch out in different directions based on a condition.
Object-Oriented Programming (OOP) Concepts: Java is an object-oriented programming language, and understanding OOP concepts like classes, objects, inheritance, and polymorphism is crucial for writing effective Java code.
Further Resources for Mastering Data Types in Java
Here are some resources that you can use to learn more about data types in Java and related topics:
- Mastering Java’s Applications: What Is Java Used For? – Learn about Java’s contribution to Internet of Things (IoT) projects.
Java Data Structures Guide – Explore essential data structures available in the Java programming language.
Java Fundamentals – Explore essential concepts and features of core Java programming.
Java Data Types – Official Oracle Documentation provides a comprehensive overview of data types in Java.
Java Operators – This tutorial from W3Schools covers Java operators in detail, with examples and exercises.
Object-Oriented Programming in Java – This course from Codecademy provides a thorough introduction to OOP concepts in Java.
Wrapping Up: Mastering Data Types in Java
In this comprehensive guide, we’ve journeyed through the world of data types in Java, a fundamental aspect of any Java program.
We began with the basics, understanding what data types are and how they work in Java. We learned about the different primitive and non-primitive data types, and how to declare and use variables of these types.
We then ventured into more advanced territory, delving into concepts like type conversion, casting, and generics. We learned how to perform implicit and explicit casting in Java and how to create type-safe classes and methods using generics.
Along the way, we also tackled some common issues that you might encounter when working with data types in Java, such as type mismatch errors and overflow/underflow. We provided solutions and tips to help you overcome these challenges and write more robust Java code.
Here’s a quick recap of the topics we’ve covered:
Topic | Description |
---|---|
Primitive Data Types | Basic data types like byte , short , int , long , float , double , char , boolean |
Non-Primitive Data Types | Reference types like String , Arrays , Classes , Interface |
Type Conversion and Casting | The process of converting a variable from one data type to another |
Generics | A feature that allows a type or method to operate on objects of various types |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up on your fundamentals, we hope this guide has given you a deeper understanding of data types in Java and their importance in programming.
With a solid grasp of data types, you’re now better equipped to write efficient, robust, and type-safe Java code. Happy coding!