Python JSON Parser | json.loads, simplejson, demjson
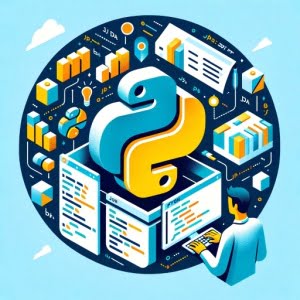
Are you finding it challenging to parse JSON in Python? You’re not alone. Many developers find themselves in a similar position, but Python, like a skilled linguist, is more than capable of understanding the language of JSON.
This guide will walk you through the process of parsing JSON in Python, from basic use to advanced techniques. We’ll explore Python’s built-in JSON module, explore alternatives, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Python JSON Parser!
TL;DR: How Do I Parse JSON in Python?
To parse JSON in Python, you can use the
json
module’sloads()
function, thesimplejson
library, or demjson.decode(). These functions allow Python to read JSON strings and convert them into Python objects.
Here’s a simple example:
import json
json_string = '{"key": "value"}'
parsed_json = json.loads(json_string)
print(parsed_json)
# Output:
# {'key': 'value'}
In this example, we import the json
module and use the loads()
function to parse a JSON string. The JSON string '{"key": "value"}'
is converted into a Python dictionary {'key': 'value'}
. The print()
function then outputs this dictionary.
This is a basic way to parse JSON in Python, but there’s much more to learn about handling JSON data in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Parsing JSON Strings with json.loads()
Python’s built-in json
module provides a simple and efficient way to parse JSON data. The json.loads()
function is one of the most commonly used tools in this module. The term ‘loads’ stands for ‘load string’, which is precisely what this function does – it takes a JSON formatted string and converts it into a Python object.
Let’s look at a simple code example:
import json
json_string = '{"name": "John", "age": 30, "city": "New York"}'
parsed_json = json.loads(json_string)
print(parsed_json)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we first import the json
module. We then define a JSON formatted string and use the json.loads()
function to convert this string into a Python dictionary. The print()
function is then used to output the Python dictionary.
The json.loads()
function is straightforward and efficient, making it an excellent tool for beginners. However, it’s important to note that this function expects a correctly formatted JSON string. If the string is not correctly formatted, the function will raise a json.JSONDecodeError
exception. So, always ensure your JSON data is correctly formatted when using json.loads()
.
Parsing Complex JSON Structures
As you gain experience with Python JSON parsing, you’ll inevitably encounter more complex JSON structures. These structures may include nested objects and arrays. Let’s delve into how Python handles these intricacies.
Consider this JSON string with nested objects:
json_string = '{"employee":{"name": "John", "age": 30, "city": "New York"}}'
parsed_json = json.loads(json_string)
print(parsed_json)
# Output:
# {'employee': {'name': 'John', 'age': 30, 'city': 'New York'}}
In this example, the JSON string contains a nested object. The json.loads()
function parses this complex structure seamlessly, creating a Python dictionary inside another dictionary.
Let’s look at another example with an array of objects:
json_string = '[{"name": "John", "age": 30, "city": "New York"}, {"name": "Jane", "age": 28, "city": "Chicago"}]'
parsed_json = json.loads(json_string)
print(parsed_json)
# Output:
# [{'name': 'John', 'age': 30, 'city': 'New York'}, {'name': 'Jane', 'age': 28, 'city': 'Chicago'}]
This time, the JSON string contains an array of objects. The json.loads()
function turns this into a Python list of dictionaries.
As you can see, Python’s json
module is capable of handling complex JSON structures. By understanding these advanced parsing techniques, you can handle any JSON data that comes your way.
Alternative JSON Parsing Methods
While Python’s built-in json
module is powerful, there are alternative methods to parse JSON in Python that you might find useful in certain scenarios. Let’s explore two of these methods: using the json.load()
function and employing third-party libraries like simplejson
.
Reading JSON from a File
The json.load()
function is similar to json.loads()
, but instead of parsing a string, it reads JSON data from a file. Here’s how it works:
import json
with open('data.json') as f:
data = json.load(f)
print(data)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we open a file called ‘data.json’ and use json.load()
to read the JSON data from the file. The data is then printed out as a Python dictionary.
Parsing With the simplejson Library
simplejson
is a third-party library that offers additional functionality beyond Python’s built-in json
module. It can be particularly useful when dealing with large or complex JSON data.
Here’s an example of how to use simplejson
:
import simplejson as json
json_string = '{"name": "John", "age": 30, "city": "New York"}'
data = json.loads(json_string)
print(data)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we import simplejson
under the alias json
and then use it just like we would the built-in json
module.
Both json.load()
and simplejson
provide valuable alternatives to json.loads()
. The best method to use depends on your specific needs. json.load()
is great for reading JSON data from files, while simplejson
can handle larger and more complex JSON data more efficiently.
Using demjson’s Decode Method
demjson
is another third-party library that provides an alternative way to parse JSON data. One unique feature of demjson
is its ability to decode non-standard JSON, meaning it can handle certain types of syntactic errors in your JSON strings better than the standard json
module.
Here’s how to use the demjson.decode()
function:
import demjson
json_string = '{"name": "John", "age": 30, "city": "New York"}'
data = demjson.decode(json_string)
print(data)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we import the demjson
module and use its decode()
function to parse a JSON string. The parsed data is then printed out as a Python dictionary.
Please note that non-standard JSON might not be a good practice to adopt, but if you find yourself in a circumstance where you have to deal with it, demjson.decode()
could be the tool you need to get the job done.
Remember, like the other methods discussed, the use of demjson.decode()
should be dictated by your specific needs and the nature of your JSON data.
Troubleshooting JSON Parsing Issues
As with any programming task, parsing JSON in Python can sometimes present challenges. Let’s discuss some common issues you may encounter and how to resolve them.
json.decoder.JSONDecodeError
One of the most common issues is the json.decoder.JSONDecodeError
. This error occurs when trying to parse a malformed JSON string. Consider the following example:
import json
json_string = '{"name": John}'
try:
parsed_json = json.loads(json_string)
except json.decoder.JSONDecodeError as e:
print(f'Error decoding JSON: {e}')
# Output:
# Error decoding JSON: Expecting property name enclosed in double quotes: line 1 column 10 (char 9)
In this example, we’re trying to parse a JSON string that’s missing double quotes around the value John
. This results in a json.decoder.JSONDecodeError
. To fix this error, ensure your JSON string is correctly formatted, with property names and string values enclosed in double quotes.
Dealing with Malformed JSON
Another common issue is dealing with malformed JSON. Malformed JSON can be a result of extra commas, missing brackets, or incorrect nesting. When dealing with malformed JSON, it’s essential to carefully check your JSON structure for any syntax errors.
Here’s an example of malformed JSON and how to fix it:
json_string = '{"name": "John",}'
try:
parsed_json = json.loads(json_string)
except json.decoder.JSONDecodeError as e:
print(f'Error decoding JSON: {e}')
# Output:
# Error decoding JSON: Expecting property name enclosed in double quotes: line 1 column 17 (char 16)
In this example, there’s an extra comma after the last item in the JSON string. Removing the extra comma resolves the issue.
By understanding these common issues and their solutions, you can ensure your Python JSON parsing tasks run smoothly.
Fix ChatGPT Invalid JSON Output
If you find yourself commonly dealing with malformed JSON, you may want an automated way to repair these strings before you load them. Regular expressions are a good way to fix malformed JSON, especially if the ways in which it is malformed are predictable.
When you ask ChatGPT (in my usage, GPT4) to give you JSON output, it makes some common mistakes, such as:
- Adds a trailing comma to the last item
- Doesn’t escape special characters like newlines inside of strings
- Doesn’t close the final item
- Uses the wrong number of backslashes to escape items
- Doesn’t escape double quotes inside of strings
I personally use some functions to clean up a variety of these problems on an as-needed basis. Since there can be unintended consequences to altering the text, I use a try-except process to progressively handle more possible issues only if loading the JSON fails after the previous processing step. We also use demjson.decode to attempt to load the json if json.loads() fails.
Here is the code I use to extract JSON sent to me by GPT4 / ChatGPT, and clean up some common problems with ChatGPT output JSON strings:
def try_robust_json_loads(text, exit_on_fail = False):
try:
reply_dict = json.loads(text)
return True, reply_dict
except json.JSONDecodeError:
print("json.loads failed, falling back to demjson.decode, for this text: \n" + text)
try:
reply_dict = demjson.decode(text)
return True, reply_dict
except demjson.JSONDecodeError as e:
print("Failed to parse JSON, attempting to clean the text...")
# Substitute special characters within quotes only (ignore escaped quotes)
cleaned_text = re.sub(r'(?<!\\)"(.*?)(?<!\\)"', lambda x: x.group(0).replace('\n', '\\n').replace('\r', '\\r').replace('\t', '\\t'), text, flags=re.S)
try:
reply_dict = json.loads(cleaned_text)
return True, reply_dict
except json.JSONDecodeError:
print("Failed to json.load, after cleaning, falling back to demjson.decode, for this text: \n" + cleaned_text)
try:
reply_dict = demjson.decode(cleaned_text)
return True, reply_dict
except demjson.JSONDecodeError as e:
print("Failed to parse JSON, attempting to escape double and quadruple backslashes, and correct incorrectly escaped double quotes...")
cleaned_text = re.sub(r'(?<!\\)\\\\\\\\(?!\\)', '\\\\\\\\\\\\\\\\', cleaned_text) # replace 4x \ with 8x \
# Replace any remaining '\\' followed by non-valid escape character with '\\\\'
cleaned_text = re.sub(r'(?<!\\)\\\\(?!\\+|["\\/bfnrt]|u[0-9A-Fa-f]{4})', r'\\\\\\\\', cleaned_text)
pattern = r'(?<!\\)\\\\\"(?!.+\"$)'
cleaned_text = re.sub(pattern, r'\"', cleaned_text)
cleaned_text = escape_quotes_json_lines(cleaned_text)
print("Updated text:")
print(cleaned_text)
try:
reply_dict = json.loads(cleaned_text)
return True, reply_dict
except json.JSONDecodeError as e:
print ("Failed to load json after cleaning: " + str(e))
if exit_on_fail:
print("Warning: JSON loading failed with error:", reply_dict + "\nExiting Program")
sys.exit(1)
else:
return False, e
def escape_quotes_json_lines(text):
pattern = r'^[^"\n]*?\"[^"\n]*?\"[^"\n]*?\"(.*)\"[^"\n]*$'
changed = True
while changed:
changed = False # Track if any changes occurred in this pass
matches = [match for match in re.finditer(pattern, text, re.MULTILINE)] # Find all matches
for match in reversed(matches): # This "reversed" safeguard is probably no longer necessary due to outer loop restarting logic
substring = match.group(1) # Extract the wanted substring, from after the third quote to before the final quote
new_substring = re.sub(r'(?<!\\)"', r'\\"', substring) # Escape unescaped quotes within the substring
if new_substring != substring:
text = text[:match.start(1)] + new_substring + text[match.end(1):] # Replace escaped substring back into the original text
# Mark that a change occurred and iterate outer loop
changed = True
break
return text
def extract_and_clean_json(text):
text = text.strip()
# Find the start and end of the JSON string
start = text.find('{') if '{' in text else text.find('[')
end = text.rfind('}') if '}' in text else text.rfind(']')
# Extract the JSON string
json_str = text[start:end+1]
# Attempt to load the JSON
try:
json.loads(json_str)
except json.JSONDecodeError:
print("Initial JSON loading failed, attempting to remove trailing commas.")
json_str = re.sub(r',\s*}', '}', json_str)
json_str = re.sub(r',\s*]', ']', json_str)
try:
json.loads(json_str)
print("Successfully cleaned the JSON string.")
except json.JSONDecodeError:
print("JSON loading failed again, attempting to escape special characters inside of double quotes.")
json_str = re.sub(r'(?<!\\)"(.*?)(?<!\\)"', lambda x: x.group(0).replace('\n', '\\n').replace('\r', '\\r').replace('\t', '\\t'), json_str, flags=re.S)
try:
json.loads(json_str)
print("Successfully cleaned the JSON string.")
except json.JSONDecodeError:
print("Failed to clean the JSON string. Passing back likely invalid JSON:")
print(json_str)
return json_str
def get_Token_String(usage):
tokenString = "Prompt: " + str(usage.prompt_tokens) + " Completion: " + str(
usage.completion_tokens) + " Total: " + str(usage.total_tokens)
return tokenString
def gpt_response(model, messages_reference, max_tokens, temperature):
# don't modify mutable arguments
messages = copy.deepcopy(messages_reference)
openai.api_key = GPT_API_KEY
response = openai.ChatCompletion.create(model=model, messages=messages, max_tokens=max_tokens, n=1, temperature=temperature)
messages.append({"role": "assistant", "content": response.choices[0].message.content})
content = response.choices[0].message.content
token_string = get_Token_String(response.usage)
return jsonify(reply=content, tokens=token_string, messages=messages)
# Example code snippet using the above functions:
response = gpt_response("gpt-4", messages, 1250, 0.2)
data = json.loads(response.data)
reply = data['reply']
print("Got GPT Reply: \n" + reply)
json_reply = extract_and_clean_json(reply)
success, dict_reply = try_robust_json_loads(json_reply, True)
# Example Output:
# Initial JSON loading failed, attempting to remove trailing commas.
# JSON loading failed again, attempting to escape special characters inside of double quotes.
# Successfully cleaned the JSON string.
You can see from this code that it can be rather involved to handle all of the possible ways that ChatGPT can send back malformed JSON. However, the ways that it misforms the JSON is fairly predictable and so with this code, we’re able to successfully clean up what it gives us nearly all of the time.
JSON’s Relevance in Python
JSON, short for JavaScript Object Notation, is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. It is a text format that is completely language-independent but uses conventions that are familiar to programmers of the C family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
In Python, JSON is a built-in package that allows us to work with JSON data. We can parse JSON data, modify it, and even create JSON data structures from scratch. But before we dive into the specifics of these operations, let’s understand the structure of JSON and how it maps to Python data types.
JSON Structure and Python Equivalents
JSON is built on two structures:
- A collection of name/value pairs. In various languages, this is realized as an object, record, struct, dictionary, hash table, keyed list, or associative array.
An ordered list of values. In most languages, this is realized as an array, vector, list, or sequence.
In JSON, these take on the form of Objects and Arrays. Here’s how JSON structures map to Python data types:
- JSON Objects are represented as Python Dictionaries (
dict
). - JSON Arrays are represented as Python Lists (
list
). - JSON Strings are represented as Python Strings (
str
). - JSON Numbers are represented as Python Numbers (
int
for integers,float
for floating-point numbers). - JSON
true
andfalse
are represented as PythonTrue
andFalse
respectively. - JSON
null
is represented as PythonNone
.
Here’s an example of a JSON object and its Python equivalent:
# JSON Object
{ "name":"John", "age":30, "city":"New York" }
# Python Dictionary
{'name': 'John', 'age': 30, 'city': 'New York'}
In this example, the JSON object is a collection of name-value pairs, which is represented as a Python dictionary.
Understanding this mapping between JSON structures and Python data types is fundamental to parsing and working with JSON data in Python.
JSON Real-World Applications
Parsing JSON in Python is not just an academic exercise. It’s a skill with real-world implications in various fields, particularly web development and data analysis.
Web Development
In web development, APIs often use JSON to transmit data. When you make an API request from a Python application, the response you receive is often in JSON format. Being able to parse this JSON data is crucial to accessing the information you need.
Data Analysis
In the field of data analysis, JSON is commonly used to store and transmit structured data. Being able to parse JSON data allows you to access, analyze, and visualize this data in Python, using libraries like pandas and matplotlib.
Exploring Related Concepts
Once you’ve mastered JSON parsing in Python, there are many related concepts to explore. For instance, you might want to learn more about working with APIs in Python, or delve into data serialization and how it relates to JSON.
Further Resources
Ready to take your Python JSON parsing skills to the next level? Here are some resources that can help:
- Python JSON Syntax Overview explores Python’s “json.loads” and “json.dumps” functions for JSON operations.
Python CSV Module Basics dives into the world of comma-separated values (CSV) and its applications.
Python Base64 Encoding is a quick tutorial on Python’s base64 encoding for binary data representation.
Official Python JSON Module Documentation helps guide you to understanding and using the JSON module effectively.
Real Python’s Guide to JSON Data Handling explores managing JSON data in Python.
Python JSON Formatting Guide – A helpful tutorial on how to effectively format JSON using Python.
These resources provide in-depth tutorials and examples that can help deepen your understanding of JSON parsing in Python.
Wrapping Up:
In this comprehensive guide, we’ve explored the ins and outs of parsing JSON in Python. We’ve covered how to use Python’s built-in json
module, and we’ve delved into the json.loads()
function, which is integral to converting JSON formatted strings into Python objects.
We began with the basics, learning how to parse simple JSON strings using json.loads()
. We then ventured into more advanced territory, exploring how to parse complex JSON structures with nested objects and arrays. Along the way, we tackled common challenges you might face when parsing JSON in Python, such as the json.decoder.JSONDecodeError
and issues with malformed JSON, providing you with solutions and workarounds for each issue.
We’ve further introduced advanced techniques to handle malformed JSON data through the use of regular expressions (regex), with an extensive code example on how to repair commonly malformed JSON produced by ChatGPT / GPT4. Automated repair processes, such as removing trailing commas, escaping special characters inside of strings, adjusting doubled or quadrupled backslashes, or correcting wrongly escaped quotes are implemented.
We also looked at alternative approaches to parsing JSON in Python, comparing the use of Python’s built-in json.load()
function and the third-party libraries simplejson
and demjson
with the json.loads()
function. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
json.loads() | Simple, efficient | Can only parse strings |
json.load() | Can read JSON data from a file | Requires a file |
simplejson | Can handle larger and more complex JSON data | Requires installation of an additional library |
demjson.decode() | Can handle non-standard JSON and syntax errors | Requires installation of an additional library and can normalize bad practice |
Whether you’re just starting out with Python JSON parsing or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of JSON parsing in Python and its real-world applications.
With the ability to parse JSON data efficiently, you’re well equipped to handle a wide range of data manipulation tasks in Python. Happy coding!