Python JSON Guide | Dumps(), Loads(), and More
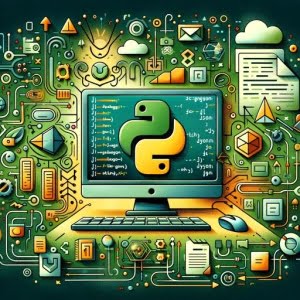
Struggling with handling JSON data in Python? You’re not alone. Many programmers find it challenging to work with JSON data due to its complex structure and syntax. But don’t worry! Like a skilled interpreter, Python can seamlessly translate JSON data into Python objects and vice versa.
In this comprehensive guide, we will explore how to work with JSON in Python. We will start with the basics and gradually delve into more advanced techniques. By the end of this article, you will have a solid understanding of how to handle JSON data in Python effectively.
So, if you’ve been wrestling with JSON in Python, this guide is for you!
TL;DR: How Do I Work with JSON in Python?
Python offers a built-in module called ‘json’ that is designed for encoding and decoding JSON data. This module makes it easy to convert JSON data into Python objects and vice versa.
Here’s a simple example to illustrate this:
import json
# A simple dictionary
data = {'name': 'John', 'age': 30}
# Convert the dictionary to JSON format
json_data = json.dumps(data)
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
In the above code, we first import the json module. We then define a simple dictionary, ‘data’. The json.dumps() function is used to convert this dictionary into a JSON formatted string, which is then printed out.
This is just the tip of the iceberg when it comes to handling JSON data in Python. For more detailed information and advanced usage scenarios, continue reading this guide. We’ll cover a range of topics from basic usage to handling complex JSON data and even alternative approaches.
Table of Contents
JSON Basics in Python
The ‘json’ module in Python is a handy tool that allows us to work with JSON data. Two of its key functions are ‘json.dumps()’ and ‘json.loads()’, which are used to convert Python objects into JSON format and vice versa.
json.dumps()
The ‘json.dumps()’ function takes a Python object as input and converts it into a JSON formatted string. Here’s a simple example:
import json
# A simple dictionary
data = {'name': 'John', 'age': 30}
# Convert the dictionary to JSON format
json_data = json.dumps(data)
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
In this code, we first define a dictionary named ‘data’. We then use the ‘json.dumps()’ function to convert this dictionary into a JSON formatted string, which is then printed out.
json.loads()
On the flip side, the ‘json.loads()’ function is used to convert a JSON formatted string into a Python object. Here’s how it works:
import json
# A JSON formatted string
json_data = '{"name": "John", "age": 30}'
# Convert the JSON string to a Python dictionary
data = json.loads(json_data)
print(data)
# Output:
# {'name': 'John', 'age': 30}
In this code, we define a JSON formatted string ‘json_data’. We then use the ‘json.loads()’ function to convert this string into a Python dictionary, which is then printed out.
These two functions form the backbone of working with JSON data in Python. They are simple to use, but they can also pose some challenges. For example, they may raise a ‘json.decoder.JSONDecodeError’ if the input is not a valid JSON. It’s important to handle such potential errors in your code to ensure its robustness.
Handling Complex JSON in Python
As we dive deeper into working with JSON in Python, we encounter more complex JSON data structures such as nested JSON objects and arrays. These require a more advanced understanding of the ‘json’ module.
Nested JSON Objects
Nested JSON objects are JSON objects that contain other JSON objects. Here’s an example of how to handle a nested JSON object in Python:
import json
# A nested JSON object
json_data = '{"employee": {"name": "John", "age": 30, "city": "New York"}}'
# Convert the JSON string to a Python dictionary
data = json.loads(json_data)
print(data)
# Output:
# {'employee': {'name': 'John', 'age': 30, 'city': 'New York'}}
In this example, the ‘json.loads()’ function is used to convert a nested JSON object into a Python dictionary. The resulting dictionary mirrors the structure of the original JSON object.
JSON Arrays
JSON arrays are equivalent to Python lists. Here’s how to handle a JSON array in Python:
import json
# A JSON array
json_data = '["apple", "banana", "cherry"]'
# Convert the JSON string to a Python list
data = json.loads(json_data)
print(data)
# Output:
# ['apple', 'banana', 'cherry']
In this example, the ‘json.loads()’ function is used to convert a JSON array into a Python list.
Reading and Writing JSON to/from a File
The ‘json.dump()’ and ‘json.load()’ functions are used to write and read JSON data to/from a file respectively. Here’s an example:
import json
# Write JSON data to a file
with open('data.json', 'w') as f:
json.dump({'name': 'John', 'age': 30}, f)
# Read JSON data from a file
with open('data.json', 'r') as f:
data = json.load(f)
print(data)
# Output:
# {'name': 'John', 'age': 30}
In this code, we first use the ‘json.dump()’ function to write a dictionary to a file in JSON format. We then use the ‘json.load()’ function to read the JSON data from the file and convert it into a Python dictionary.
Exploring Alternative JSON Tools in Python
While Python’s built-in ‘json’ module is powerful, there are alternative methods and third-party libraries that offer more features and flexibility when dealing with JSON data. Two notable examples are ‘simplejson’ and ‘pandas.read_json()’.
Simplejson: A Flexible JSON Handler
‘simplejson’ is a third-party library that offers more options for customizing the process of encoding and decoding JSON data. It also provides better error messages, which can be helpful for debugging.
Here’s an example of how to use ‘simplejson’:
import simplejson as json
# A simple dictionary
data = {'name': 'John', 'age': 30}
# Convert the dictionary to JSON format
json_data = json.dumps(data)
print(json_data)
# Output:
# '{"name": "John", "age": 30}'
In this example, we use ‘simplejson’ in the same way as the built-in ‘json’ module. However, ‘simplejson’ provides more options for customizing the encoding and decoding process.
Pandas: Handling JSON with DataFrames
The ‘pandas’ library offers a function called ‘read_json()’ that can convert a JSON string into a DataFrame. This can be particularly useful when dealing with large and complex JSON data.
Here’s an example of how to use ‘pandas.read_json()’:
import pandas as pd
# A JSON string
json_data = '[{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]'
# Convert the JSON string to a DataFrame
df = pd.read_json(json_data)
print(df)
# Output:
# name age
# 0 John 30
# 1 Jane 25
In this example, we convert a JSON string into a DataFrame using ‘pandas.read_json()’. This allows us to handle the JSON data in a tabular format, which can be more convenient for data analysis and manipulation.
Each of these alternative approaches has its advantages and disadvantages, and the best choice depends on your specific needs. ‘simplejson’ offers more flexibility and better error messages, while ‘pandas.read_json()’ allows for easy manipulation of JSON data in a tabular format. However, both require installing additional libraries, which may not be desirable in all situations. It’s recommended to start with Python’s built-in ‘json’ module and consider these alternatives as your needs become more complex.
Working with JSON in Python can sometimes lead to unexpected errors and challenges. Here, we’ll discuss some of the common issues you might encounter and provide solutions and tips to help you navigate these hurdles.
Handling json.decoder.JSONDecodeError
The json.decoder.JSONDecodeError
is raised when there’s an issue decoding a JSON document. This usually happens when the input is not a valid JSON.
import json
# An invalid JSON string
json_data = '{"name": "John" "age": 30}'
try:
data = json.loads(json_data)
except json.decoder.JSONDecodeError as e:
print(f'Invalid JSON: {e}')
# Output:
# Invalid JSON: Expecting property name enclosed in double quotes: line 1 column 15 (char 14)
In this example, we attempt to load an invalid JSON string, which raises a json.decoder.JSONDecodeError
. By using a try-except block, we can catch this error and provide a useful error message.
Dealing with Non-Serializable Types
Not all Python objects can be serialized to JSON. For instance, datetime
objects and set
data types are not serializable. Trying to serialize such objects will raise a TypeError
.
import json
from datetime import datetime
# A dictionary with a datetime object
data = {'name': 'John', 'age': 30, 'birthday': datetime(1990, 1, 1)}
try:
json_data = json.dumps(data)
except TypeError as e:
print(f'Non-serializable type: {e}')
# Output:
# Non-serializable type: Object of type datetime is not JSON serializable
In this example, we try to serialize a dictionary that contains a datetime
object, which raises a TypeError
. Again, using a try-except block allows us to catch the error and provide a useful message.
These are just a couple of the common issues you might encounter when working with JSON in Python. Always remember to handle potential errors in your code to ensure its robustness.
Understanding JSON and Python Data Structures
Before diving deeper into the technicalities of handling JSON in Python, it’s crucial to understand the basics of JSON and how it corresponds to Python data structures.
What is JSON?
JavaScript Object Notation (JSON) is a lightweight data-interchange format that is easy for humans to read and write and easy for machines to parse and generate. It is based on a subset of JavaScript Programming Language, Standard ECMA-262 3rd Edition – December 1999. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
JSON and Python Data Structures
Python represents data in several basic types like lists, dictionaries, strings, integers, floats, and booleans. These Python types correspond to JSON arrays, objects, strings, numbers, and booleans respectively.
Here’s a simple comparison:
Python | JSON |
---|---|
dict | object |
list, tuple | array |
str | string |
int, float | number |
True | true |
False | false |
None | null |
Understanding this correspondence is key to working with JSON in Python. It helps you to translate back and forth between Python and JSON, making it easier to handle and manipulate JSON data in your Python programs.
The Power of JSON in Data Handling
Handling JSON data in Python is not just about converting Python objects to JSON strings and vice versa. It’s a fundamental skill that has a wide range of applications in today’s data-driven world.
JSON in Web Scraping and APIs
Web scraping and APIs are two areas where JSON truly shines. Many modern web APIs return data in JSON format due to its lightweight nature and compatibility with JavaScript. Being able to handle JSON data in Python is crucial when working with such APIs.
import requests
import json
# Make a request to a web API that returns JSON data
response = requests.get('https://api.example.com/data')
# Convert the response to a Python dictionary
data = json.loads(response.text)
# Print the data
print(data)
# Output:
# This will vary depending on the API and the data it returns
In this example, we make a GET request to a hypothetical web API that returns JSON data. We then use the json.loads()
function to convert the response into a Python dictionary.
JSON in Data Analysis
JSON is also commonly used in data analysis. With the rise of big data, JSON has become a popular format for storing and exchanging large volumes of data. Python’s powerful data analysis libraries, such as pandas, can work with JSON data to provide valuable insights.
import pandas as pd
# A JSON string representing a dataset
json_data = '[{"name": "John", "age": 30}, {"name": "Jane", "age": 25}]'
# Convert the JSON string to a DataFrame
df = pd.read_json(json_data)
# Perform data analysis
# For example, calculate the average age
average_age = df['age'].mean()
print(average_age)
# Output:
# 27.5
In this example, we convert a JSON string into a pandas DataFrame. We then perform a simple data analysis operation: calculating the average age.
Further Resource for JSON in Python
While JSON is a powerful data format, it’s not the only one out there. Other formats like XML and CSV are also widely used. Understanding how to handle these formats in Python can further enhance your data handling skills. To expand your Python knowledge, we encourage you to explore these free online resources:
- Python json.dumps(): Serialize Data to JSON – Learn how to serialize Python objects to JSON using “json.dumps.”
Working with CSV Files in Python – An overview on how Python simplifies data import and export with CSV files.
Modify JSON Fields with Python – Discover how to modify fields in JSON data using Python.
Python Pretty Print JSON Guide – Learn how to format JSON output for readability in Python with pretty print.
Applications of JSON in Python – Explore the broad range of applications for JSON within Python programming.
Mastering JSON in Python: A Recap
We’ve taken a deep dive into working with JSON in Python, exploring everything from the basics to more advanced techniques. Let’s summarize key takeaways from our journey.
Python’s built-in ‘json’ module simplifies the process of handling JSON data. Key functions like json.dumps()
and json.loads()
allow us to convert Python objects into JSON strings and vice versa, making JSON data more manageable.
While Python simplifies JSON handling, we also discussed potential challenges such as json.decoder.JSONDecodeError
and issues with non-serializable types. We learned that using try-except blocks can help us handle these errors effectively.
Beyond Python’s built-in tools, we also explored alternative methods for handling JSON data, including the ‘simplejson’ library and ‘pandas.read_json()’. These alternatives offer more flexibility and features, but require installing additional libraries.
Method | Advantage | Disadvantage |
---|---|---|
json module | Built-in, easy to use | Limited features |
simplejson | More options, better error messages | Requires additional installation |
pandas.read_json() | Easy data manipulation in tabular format | Requires additional installation, more suitable for data analysis |
Understanding JSON in Python opens up a world of possibilities in areas like web scraping, APIs, and data analysis. It’s a fundamental skill in today’s data-driven world.
We hope this guide has helped you understand and master the process of working with JSON in Python. Remember, practice makes perfect, so keep experimenting with different JSON data and Python functions. Happy coding!